Introduction to Plugin Architecture in C#
Summary
TLDRIn this Rock Coding YouTube tutorial, Anton teaches viewers about plugin architecture in C#, focusing on extending applications at runtime. He explains how plugins work using examples like Visual Studio and WordPress, then dives into creating a plugin system for an ASP.NET Core application. Anton demonstrates building a server, a plugin development kit (PDK), and a custom middleware to load and unload plugins dynamically. He also addresses challenges like unloading plugins and avoiding memory leaks, providing practical insights into plugin development in C#.
Takeaways
- 📘 Plugin architecture in C# allows for extending applications at runtime, similar to how extensions work in Visual Studio, VS Code, or WordPress plugins.
- 🔌 The presenter introduces a 'Plugin Development Kit (PDK)' which is a specialized SDK for creating plugins, including components like 'IPluginEndpoint' and 'PathAttribute'.
- 🏗️ The server project is designed to be extended with plugins, and it references the PDK to incorporate HTTP context functionalities.
- 🛠️ Custom middleware is created to handle plugin loading and execution, enabling dynamic addition of endpoints to an ASP.NET Core application.
- 🔄 The process of loading and unloading plugins involves using reflection to instantiate types and execute methods based on the plugin's assembly.
- 🧩 The concept of 'Assembly Load Context' is highlighted as a way to manage the scope of assembly loading, allowing for the possibility of unloading assemblies.
- 🗑️ Unloading plugins is not immediate and requires the use of garbage collection to clear references and finalize the unloading process.
- 🔄 The presenter demonstrates the use of a weak reference to an assembly load context to check if an assembly has been successfully unloaded.
- 🛑 The importance of managing references carefully is emphasized to avoid preventing assemblies from being unloaded due to lingering references.
- 🚫 The script mentions that certain operations, like serialization with the JSON serializer, can inadvertently leak references and affect the unloading process.
- 🔧 The video concludes with recommendations for plugin development, such as aiming for pure functions and being cautious of side effects that could impact the plugin lifecycle.
Q & A
What is plugin architecture in the context of C#?
-Plugin architecture in C# refers to the ability to extend an application at runtime, similar to how extensions or plugins add functionality to applications like Visual Studio or WordPress.
Why is plugin architecture useful in application development?
-Plugin architecture is useful because it allows developers to add new features and functionality to an application without modifying the core application code, providing flexibility and extensibility.
What is the role of a Plugin Development Kit (PDK) in creating plugins?
-A Plugin Development Kit (PDK) provides the necessary components and interfaces for developers to create plugins. It includes elements like 'IPluginEndpoint' and 'PathAttribute' to define entry points and routing for plugins.
How does the 'PathAttribute' in the PDK contribute to plugin development?
-'PathAttribute' in the PDK is used to specify the endpoint path for a plugin, allowing the server to recognize and route requests to the appropriate plugin functionality.
What is the purpose of the 'EndpointPDK' in the server project?
-The 'EndpointPDK' in the server project references the framework and provides the ability to include HTTP context within the plugin, enabling the plugin to interact with the server's HTTP requests and responses.
Can you explain the custom middleware mentioned in the script for handling plugins?
-The custom middleware, referred to as 'Plugin Middleware', is used to dynamically load and execute plugin code during runtime. It matches incoming requests to plugin endpoints and activates the corresponding plugin functionality.
What is the significance of unloading assemblies in a plugin architecture?
-Unloading assemblies is important for updating or removing plugins without restarting the entire application. It allows for dynamic changes to the application's functionality.
Why is it challenging to unload a plugin in a C# application?
-Unloading a plugin can be challenging due to reference leaks where parts of the plugin may still be referenced by the application, preventing the garbage collector from unloading the assembly.
What is the role of 'AssemblyLoadContext' in managing plugin assemblies?
-'AssemblyLoadContext' provides a way to load and unload assemblies independently from the main application domain. It allows for creating a separate scope for plugin assemblies, facilitating their dynamic loading and unloading.
How can you ensure that a plugin is properly unloaded from an application?
-To ensure a plugin is properly unloaded, you can use techniques like forcing garbage collection, waiting for finalizers to run, and using weak references to check if the assembly has been collected.
What are some potential issues with using the 'System.Text.Json' serializer in plugins?
-The 'System.Text.Json' serializer can cause reference leaks because it caches the types it serializes, which can prevent the plugin assembly from being unloaded if the serializer holds references to plugin types.
Outlines
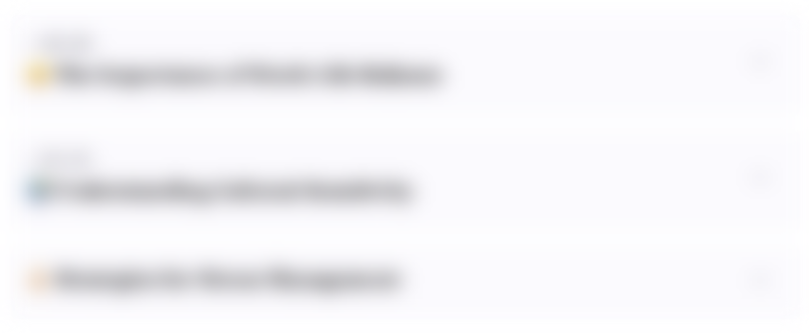
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنMindmap
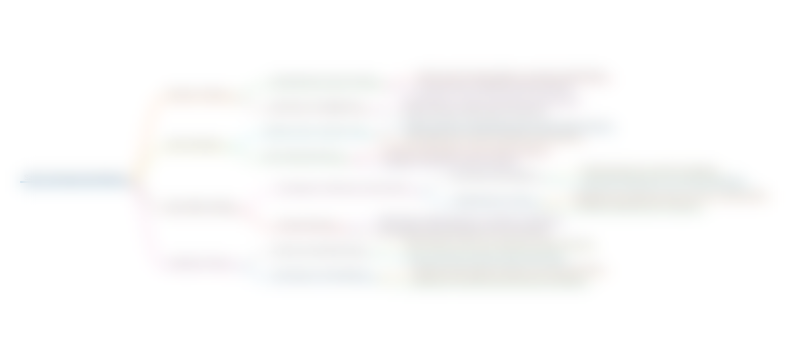
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنKeywords
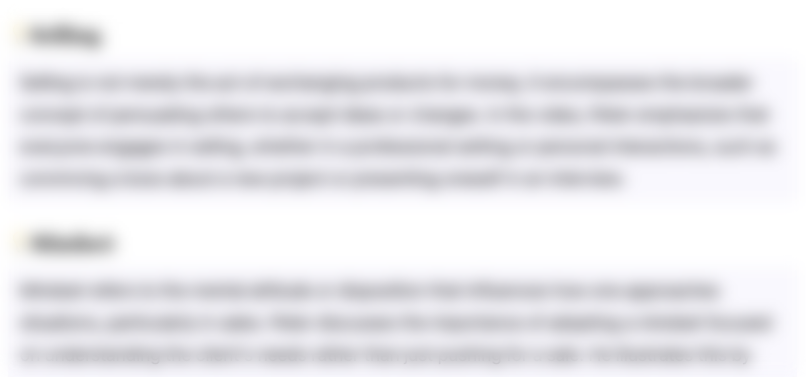
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنHighlights
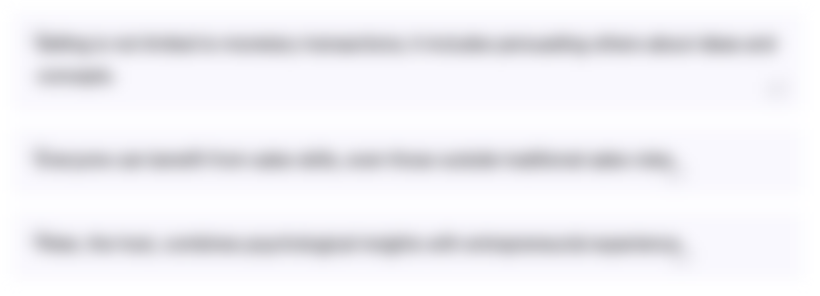
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنTranscripts
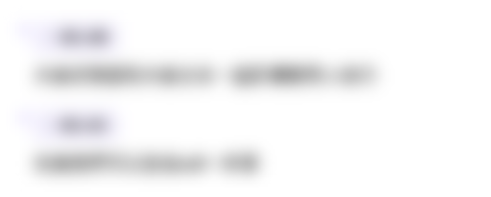
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنتصفح المزيد من مقاطع الفيديو ذات الصلة
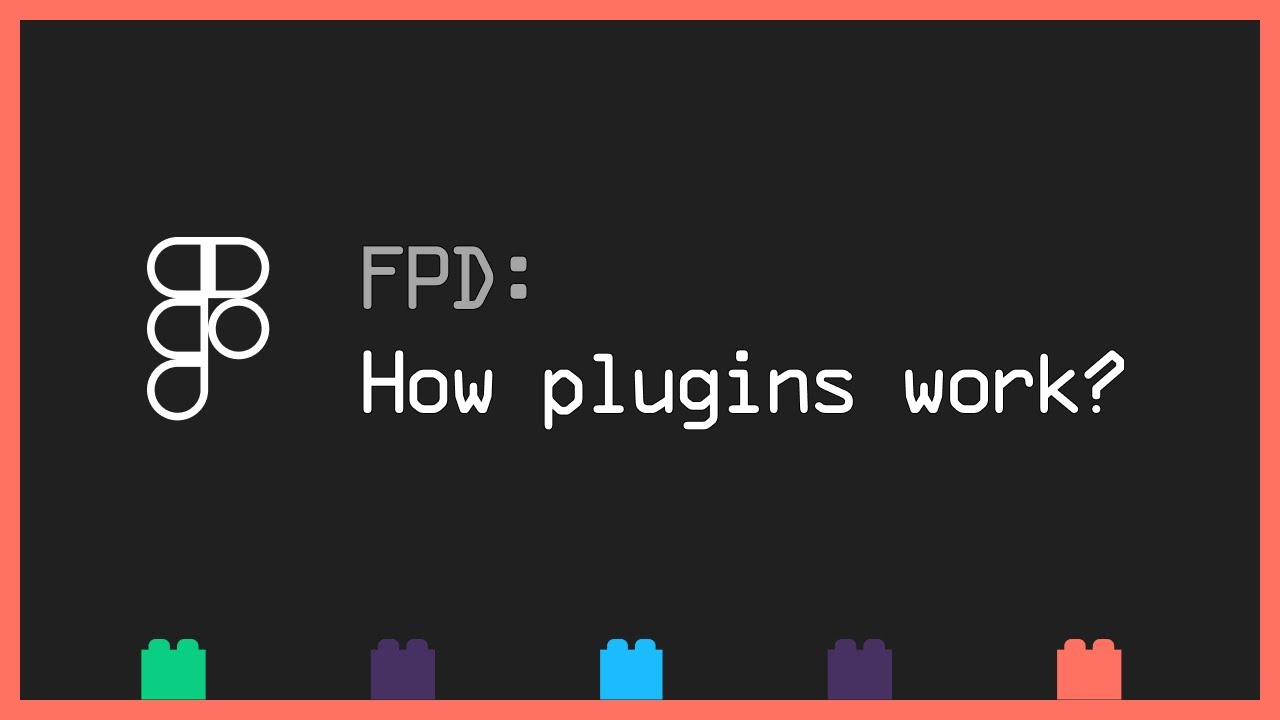
Figma plugin development: How plugins work?

Custom Fleet Plugins for Your Kotlin Codebase | Vitaly Bragilevsky

Plugin - Part 1 | What is Plugin? | Quick Introduction to Plugin | Dynamic CRM
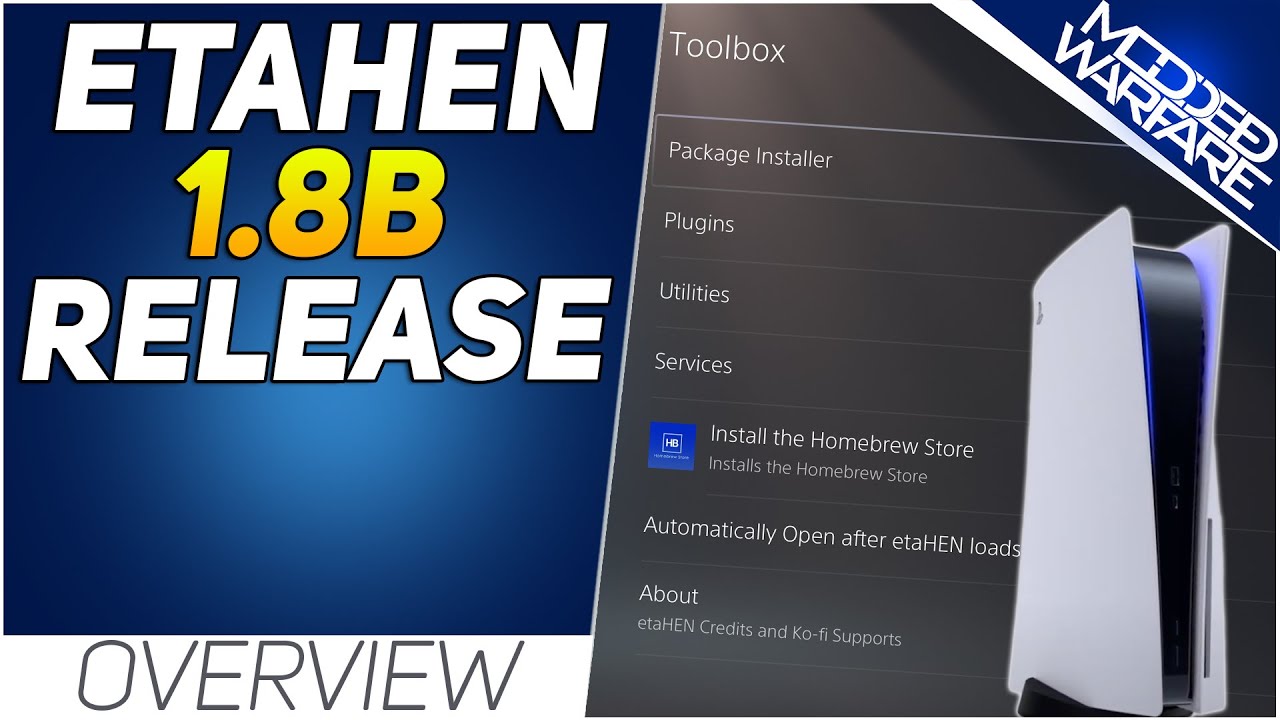
etaHEN 1.8B & New Itemzflow Released for PS5!
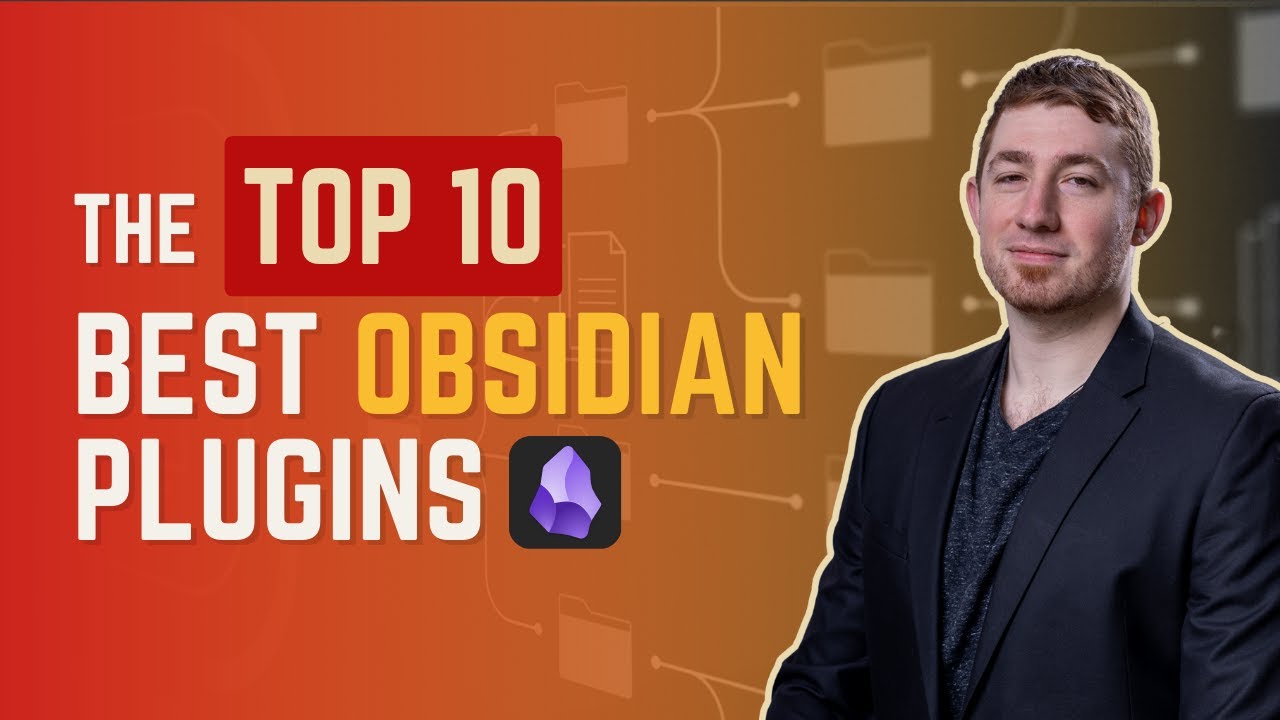
🏆️ TOP 10 BEST Obsidian Plugins 🔌️

Ultimate Solution for Zero Latency Monitoring Without DSP (Works with any Interface & any Daw)
5.0 / 5 (0 votes)