#83 User Input using BufferedReader and Scanner in Java
Summary
TLDRThis video script delves into Java's methods for taking user input, contrasting the traditional `System.in.read` method, which returns ASCII values, with the more modern and user-friendly `Scanner` class introduced in Java 1.5. It explains the complexities of handling `IOException` and the importance of closing resources like `BufferedReader` to prevent data leaks. The script provides a step-by-step guide on using both `BufferedReader` and `Scanner` for input, highlighting the simplicity and efficiency of the latter.
Takeaways
- 📝 System.out.println is a method that belongs to the PrintStream class, which is used for outputting text to the console.
- 🔍 The System class contains a static PrintStream object named 'out', which is used to call the println method without creating an object.
- ❓ To take input from the user in Java, one can use the System.in object, which is of type InputStream and has a 'read' method.
- 🔢 The read method returns an int value representing the ASCII value of the character entered by the user, not the actual number.
- 💡 To convert the ASCII value to an actual number, subtract 48 from the read value, e.g., 'int num = read - 48;'.
- 🚫 The read method can only read one character at a time, which may not be suitable for larger numbers or strings.
- 🔄 Java provides the BufferedReader class for reading multiple characters at once, which can be used with an InputStreamReader.
- 📚 BufferedReader requires the user to import the java.io package and pass an InputStreamReader object to its constructor.
- 🔑 When using BufferedReader, the readLine method returns a string, which can be converted to an integer using Integer.parseInt.
- 🛠 Scanner class, introduced in Java 1.5, simplifies input by providing methods like nextInt() that automatically parse the input into the desired data type.
- 🔌 Both BufferedReader and Scanner need to be closed after use to free up system resources, preventing resource leaks and allowing others to use the resources.
Q & A
What is the purpose of the 'println' method in Java?
-The 'println' method in Java is used to print text to the console. It is a method of the PrintStream class, accessed through the 'out' object which is a static variable inside the System class.
How does Java handle user input from the console?
-Java uses the 'in' object of the InputStream class to handle user input from the console. The 'read' method of the InputStream class returns an int value representing the ASCII value of the input character.
What is the issue with using 'System.in.read' for taking user input?
-The 'System.in.read' method returns the ASCII value of the input character, not the actual character or number. This can be problematic when trying to read numerical input directly.
How can you convert the ASCII value returned by 'System.in.read' to an actual number?
-To convert the ASCII value to an actual number, you can subtract 48 from the ASCII value. This works because the ASCII value for '0' is 48, and the values for other digits increase by 1 from there.
What is the BufferedReader class used for in Java?
-The BufferedReader class is used for reading text from an input stream, such as the console. It allows for reading lines of text and can handle larger inputs compared to 'System.in.read'.
Why do we need to import the java.io package to use BufferedReader?
-The java.io package contains the BufferedReader class, along with other I/O classes like IOException. Importing this package is necessary to use these classes in your Java program.
How does the BufferedReader work with the InputStreamReader?
-The BufferedReader is constructed with an InputStreamReader object, which in turn is constructed with an InputStream object, such as 'System.in'. This setup allows the BufferedReader to read from the console input stream.
What is the advantage of using BufferedReader over 'System.in.read'?
-BufferedReader allows for reading an entire line of text as a String, making it easier to handle multi-character inputs. It also provides methods to convert the String to other data types, like integers, without dealing with ASCII values.
What is the Scanner class in Java and how is it used for input?
-The Scanner class, introduced in Java 1.5, is a more modern way to handle user input. It can read different types of input directly, such as integers or strings, without the need for manual conversion from ASCII values.
Why is it important to close resources like BufferedReader or Scanner after use?
-Closing resources like BufferedReader or Scanner is important to free up system resources. It prevents resource leaks and ensures that the resources are available for other parts of the program or other programs to use.
Outlines
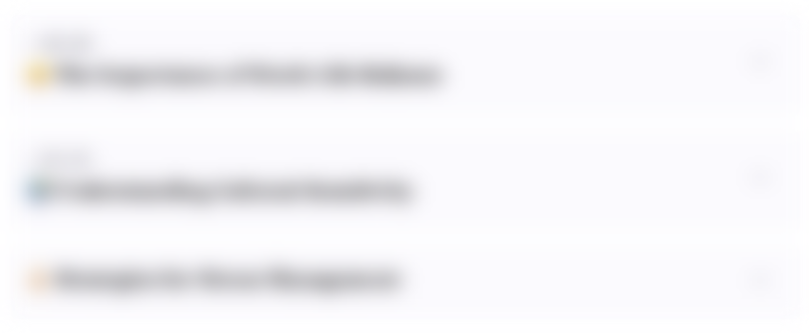
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنMindmap
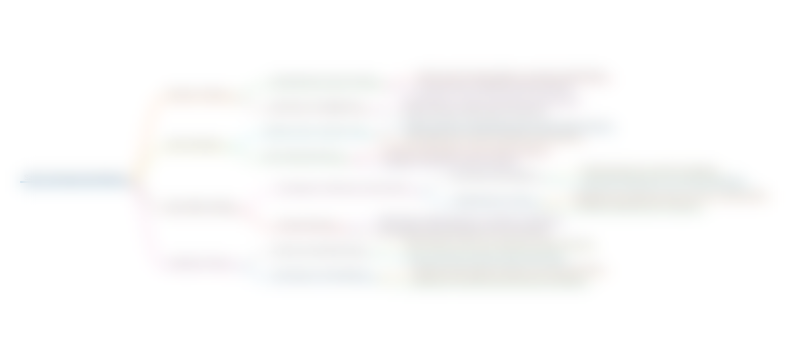
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنKeywords
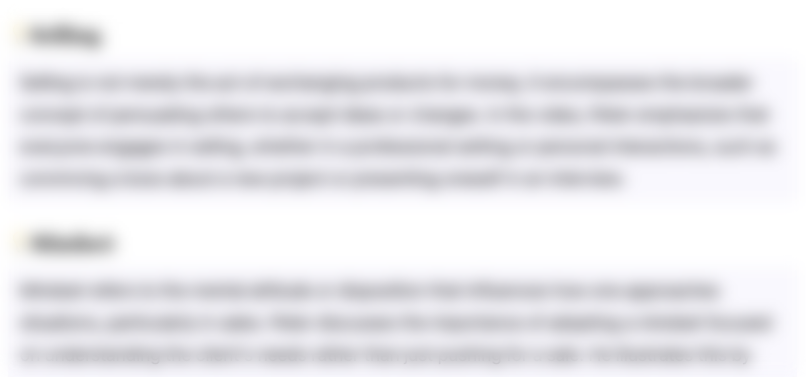
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنHighlights
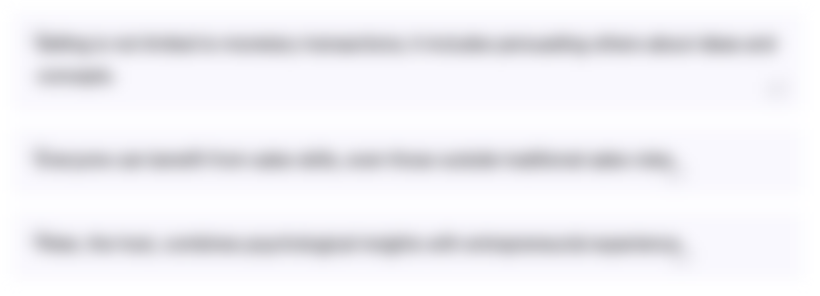
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنTranscripts
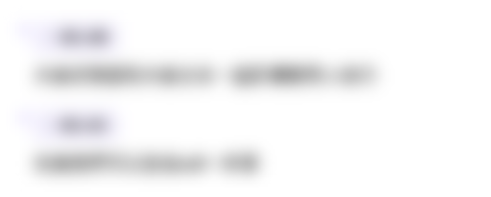
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنتصفح المزيد من مقاطع الفيديو ذات الصلة

Capítulo 4 - Inserção de elementos em um vetor
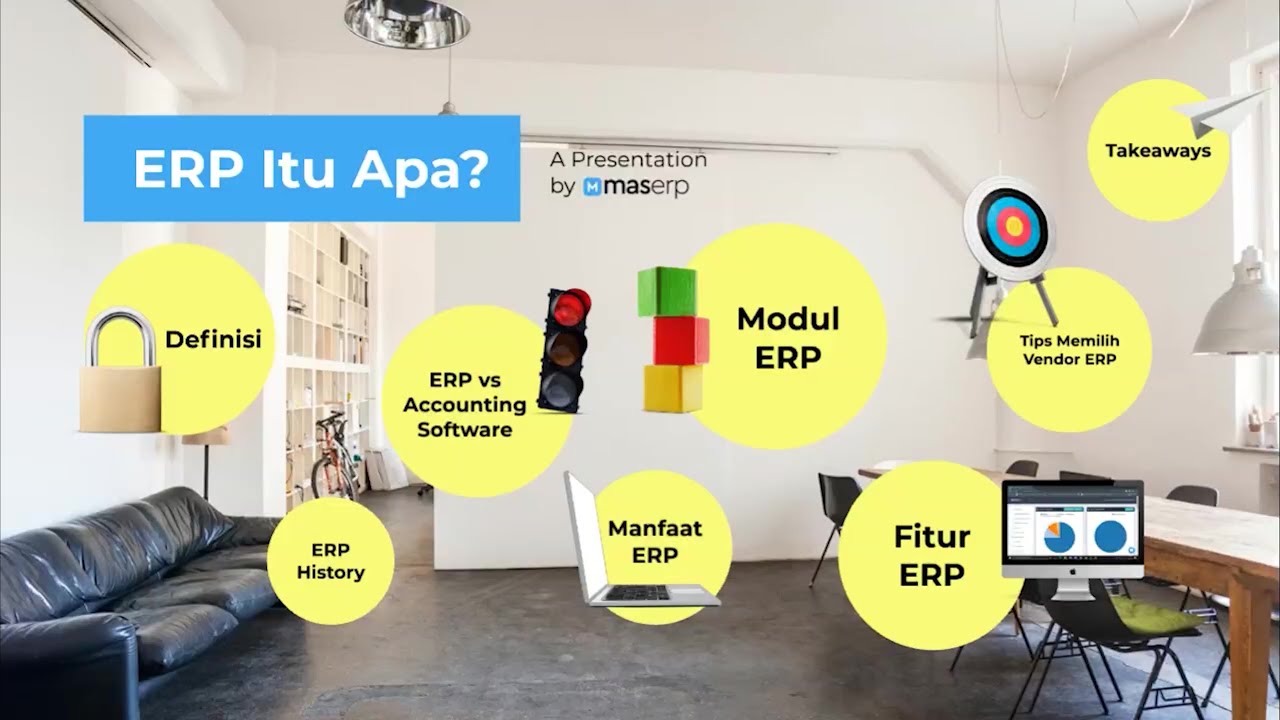
Apa Itu ERP? History, Manfaat, Fitur dan Tips Memilih Vendor ERP!
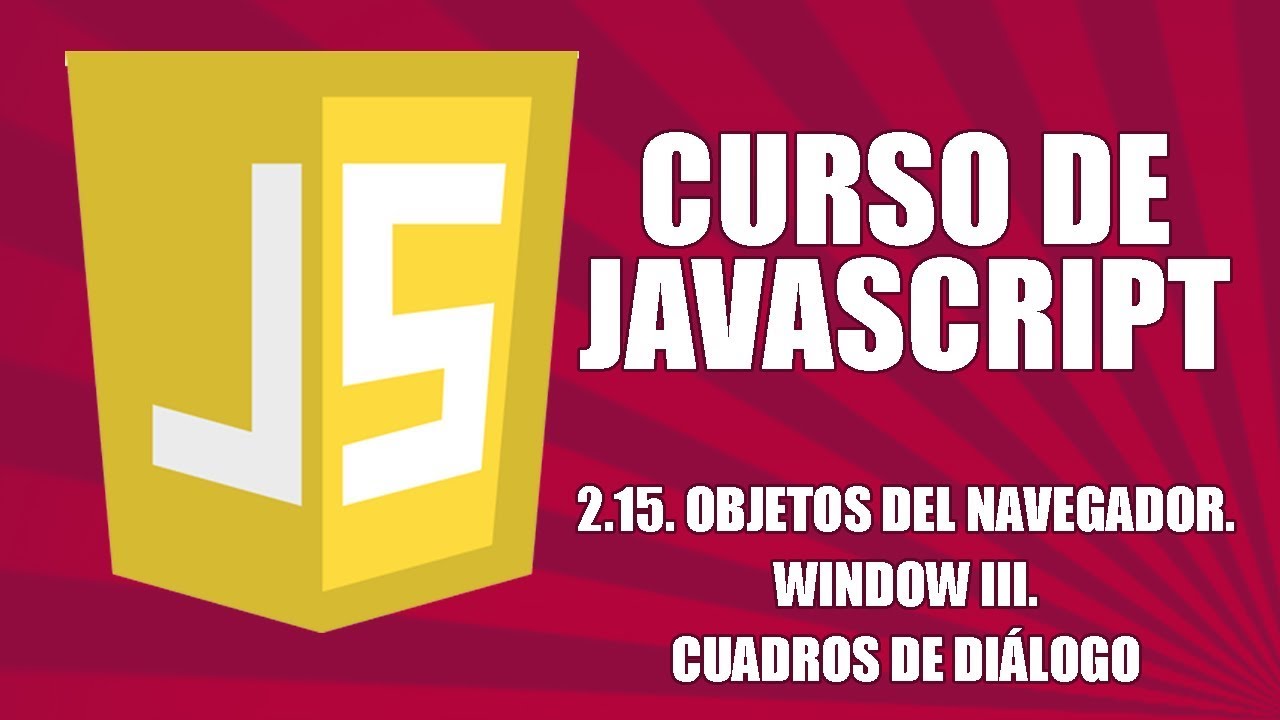
Curso de Javascript - 2.15. Objetos del navegador (BOM). Window III. Cuadros de diálogo

What Is Hashing? | What Is Hashing With Example | Hashing Explained Simply | Simplilearn

Keystroke-Level Model - Introduction

Packages
5.0 / 5 (0 votes)