#32 Spring Security | Custom Configuration
Summary
TLDRThis video tutorial delves into customizing Spring Security configurations beyond the default settings. It guides viewers through creating a config class to define beans and override the default security filter chain. The presenter demonstrates disabling CSRF protection, enforcing authentication for all requests, and toggling between form login and HTTP basic authentication. The tutorial also touches on making the application stateless for session management, providing a foundation for more advanced security implementations like JWT in future videos.
Takeaways
- 😀 The video discusses configuring a Spring Security application with custom settings beyond the default configurations.
- 🔒 It explains how to disable the default Spring Security configuration and set up a custom security filter chain.
- 🛠️ The presenter demonstrates creating a config class to customize Spring Security settings, emphasizing the use of the @Configuration annotation.
- 📝 The video covers changing application properties, such as usernames and passwords, and the importance of modifying the security filter chain.
- 🔄 The process of disabling CSRF (Cross-Site Request Forgery) protection in Spring Security is detailed, showing both lambda and imperative syntax.
- 🚫 It illustrates how to enforce authentication for every HTTP request, ensuring no page can be accessed without proper credentials.
- 🔑 The video guides through enabling form login with customizer and handling HTTP basic authentication for tools like Postman.
- 🔄 The presenter discusses the implications of disabling CSRF and making the HTTP session stateless using session management configurations.
- 📱 The script touches on the challenges of form login in a stateless session and how it requires credentials with every request.
- 🛑 The video shows how to disable the default logout functionality when implementing custom security configurations.
- 🔍 Finally, the script provides insights into the builder pattern for configuring HTTP security settings in a readable and sequential manner.
Q & A
What is the default configuration provided by Spring Security when it is first implemented?
-When Spring Security is first implemented, it provides a default configuration that includes a basic security setup. This includes a default user with a preset username and password, and a simple login form for authentication.
How can one customize the security settings in a Spring Security application?
-To customize the security settings in a Spring Security application, one needs to create a configuration class annotated with `@Configuration` and use the `@EnableWebSecurity` annotation to override the default security configuration. This allows the developer to define their own beans and security configurations.
What is the purpose of the `SecurityFilterChain` in Spring Security?
-The `SecurityFilterChain` in Spring Security is responsible for defining the sequence of security filters that are applied to the application's HTTP requests. Customizing this filter chain allows developers to control the security mechanisms applied to different parts of the application.
How can one disable CSRF (Cross-Site Request Forgery) protection in Spring Security?
-To disable CSRF protection in Spring Security, one can use the `HttpSecurity` object's `csrf()` method with a customizer that disables CSRF, either by using lambda syntax for a cleaner code or by using the imperative style with a custom `CsrfConfigurer`.
What does the `authorizeRequests()` method do in Spring Security configuration?
-The `authorizeRequests()` method in Spring Security configuration is used to specify the authorization rules for HTTP requests. It allows developers to define which requests should be authenticated, which can be done using lambda expressions or an `AuthorizationManager` object.
How can one implement form-based authentication in Spring Security?
-Form-based authentication can be implemented in Spring Security by using the `formLogin()` method on the `HttpSecurity` object. This method can be customized with default settings using `withDefaults()` or by specifying custom configurations.
What is HTTP Basic authentication and how is it enabled in Spring Security?
-HTTP Basic authentication is a simple authentication scheme built into the HTTP protocol, where credentials are sent in the HTTP headers. In Spring Security, it can be enabled by calling the `httpBasic()` method on the `HttpSecurity` object, which can also be customized with default settings.
Why might one want to disable the default logout functionality in a customized Spring Security setup?
-One might want to disable the default logout functionality in a customized Spring Security setup because when implementing custom authentication mechanisms, the default logout may not work as expected. Developers may need to implement their own logout logic that integrates with their custom authentication system.
What is the session creation policy and how does it relate to making a session stateless in Spring Security?
-The session creation policy in Spring Security determines when and how sessions are created. To make a session stateless, one can set the session creation policy to 'stateless' using the `sessionManagement()` method on the `HttpSecurity` object. This means that the server does not need to maintain session information between requests.
How can one make HTTP requests stateless to avoid CSRF issues in Spring Security?
-To make HTTP requests stateless and avoid CSRF issues, one can configure the session management in Spring Security to be stateless by setting the session creation policy to 'stateless'. This requires passing credentials with every request, effectively making each request independent of any session state.
What is the builder pattern mentioned in the script and how does it simplify Spring Security configuration?
-The builder pattern mentioned in the script is a method of constructing an object step by step, often used in configurations. In Spring Security, it simplifies the configuration by allowing developers to chain method calls on a single object, making the code more readable and easier to manage.
Outlines
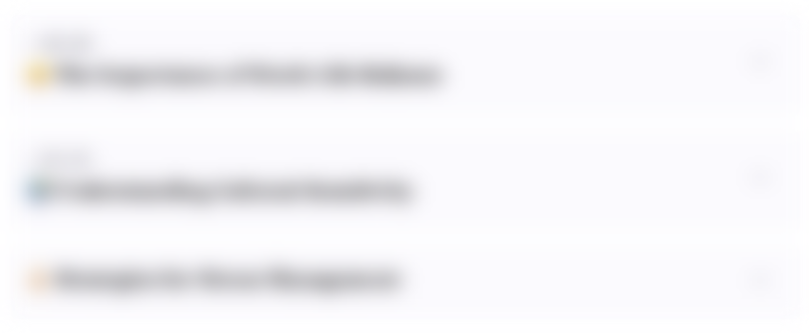
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنMindmap
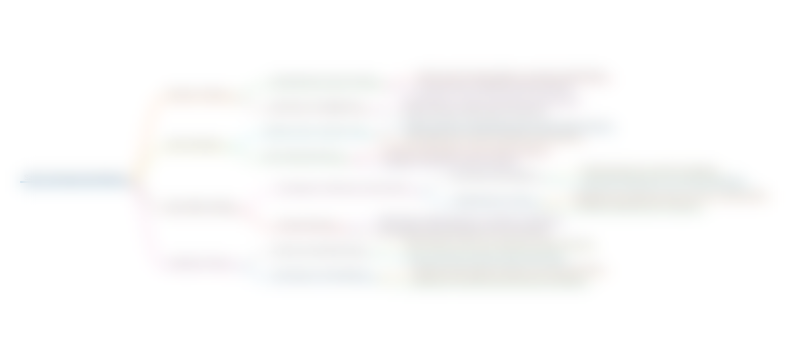
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنKeywords
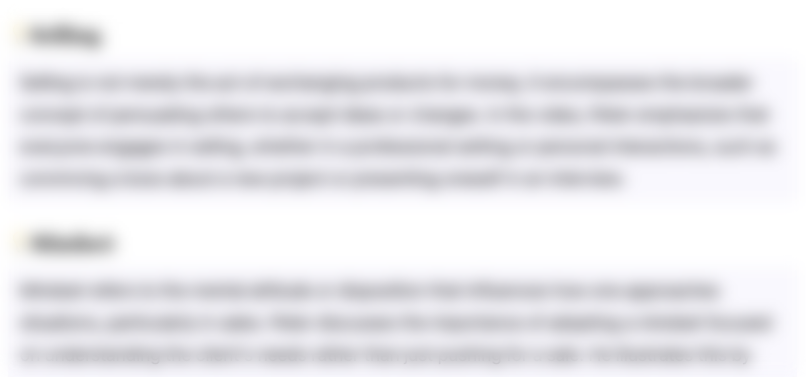
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنHighlights
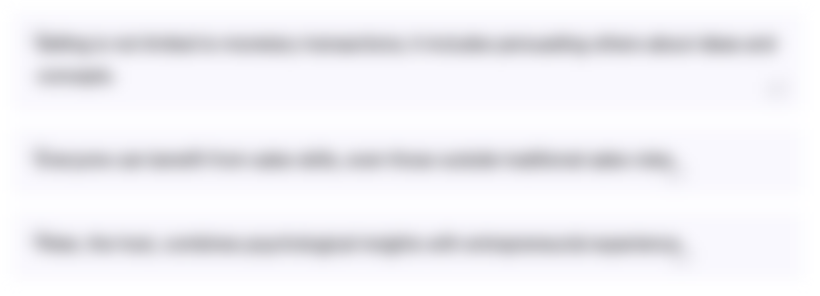
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنTranscripts
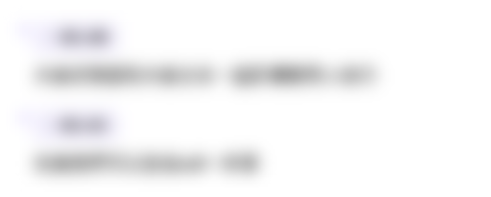
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنتصفح المزيد من مقاطع الفيديو ذات الصلة

#30 Spring Security | Custom Login

CORSO COMPLETO SPRING #2 | Convention over configuration
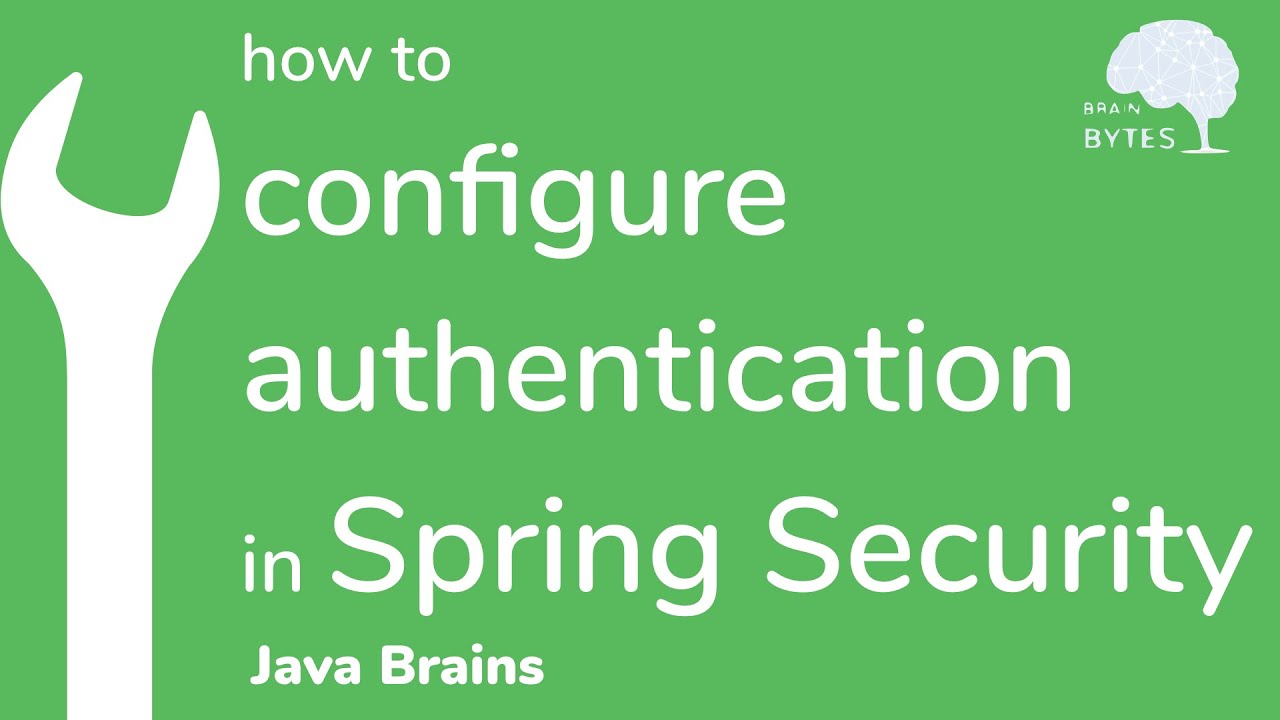
How to configure Spring Security Authentication - Java Brains

Security Misconfiguration - 2023 OWASP Top 10 API Security Risks
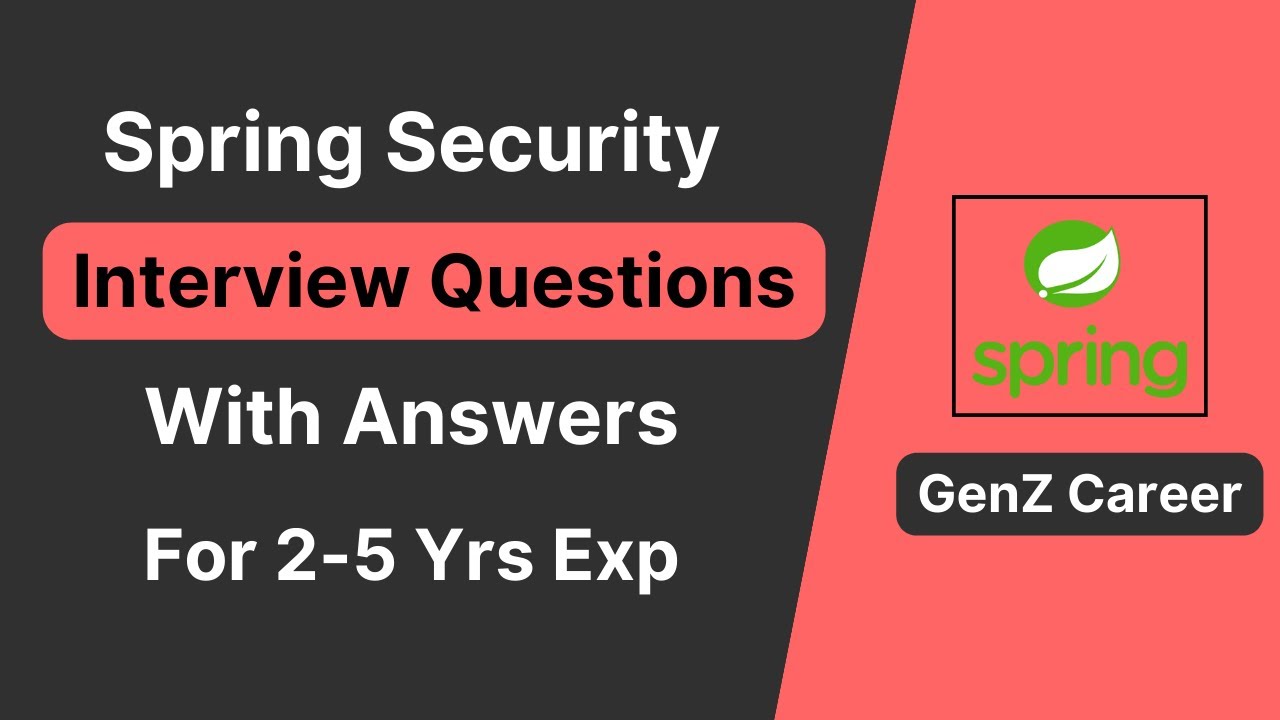
Top Spring Security Interview Questions and Answers
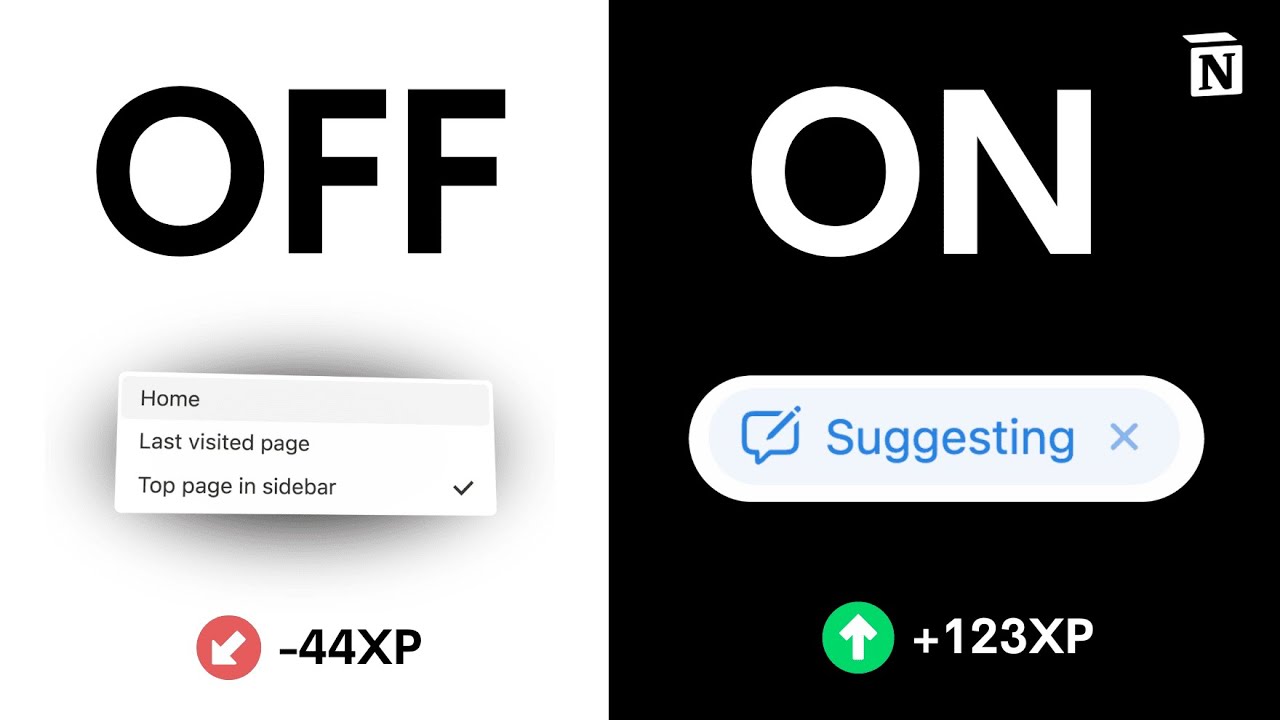
Best Notion Setup Tips & Tracks for Beginners (2024)
5.0 / 5 (0 votes)