Arduino Workshop - Chapter 5 - Interrupts
Summary
TLDRThis script introduces the concept of interrupts in microcontroller programming, contrasting them with the polling method for handling button inputs. It explains how interrupts allow the microcontroller to be notified of changes without constant checking, making them more efficient for complex programs. The video covers the basics of setting up interrupts in Arduino, including defining interrupt service routines (ISRs) and the importance of keeping ISRs short to avoid interfering with other tasks. It also provides practical tips and best practices for using interrupts effectively.
Takeaways
- 🔄 Polling is a method where a program frequently checks the state of an input like a button, but it's inefficient for complex programs.
- 🛠️ Interrupts provide a more efficient way to handle input changes; they allow the microcontroller to be notified of state changes without constant checking.
- 👮♂️ An interrupt is a hardware feature that can monitor an input pin and 'interrupt' the microcontroller to signal a change.
- 📬 The analogy of expecting a letter from the postman illustrates how interrupts allow you to be notified instantly of an event, freeing you to do other tasks.
- ⚠️ There are three types of interrupts: change, rising, and falling, each triggered under different conditions of signal transition.
- 🛂 The Interrupt Service Routine (ISR) is a specific function run when an interrupt condition is met, and it should be kept as short as possible.
- 🚫 ISRs cannot return values or take parameters, and certain functions like delay() do not work inside them due to their interrupt-based nature.
- 🔁 The 'volatile' modifier is used for variables changed inside an ISR to indicate they can change at any time, independent of the main program flow.
- 🔌 Only certain Arduino pins have interrupt capabilities, and not all chips support all types of interrupts on all pins.
- 🔄 When using interrupts, attachInterrupt is used to set up the interrupt on a specific pin with a designated ISR to be executed upon change, rising, or falling.
- 🔧 In practice, using interrupts can bypass the need for continuous polling in the main loop, making the program more efficient and responsive.
Q & A
What is the primary method discussed in the script for detecting button presses in simple programs?
-The primary method discussed is polling, which involves constantly checking the state of the button at a high frequency to detect presses or releases.
Why is polling not efficient for complex programs?
-Polling is inefficient for complex programs because it requires the microcontroller to frequently check the button state, even when it is often the same, which interrupts other tasks and processes.
What is an interrupt in the context of microcontrollers?
-An interrupt is a hardware feature on a microcontroller that can monitor the state of an input pin and can interrupt the microcontroller's current task to signal a change in state.
How do interrupts differ from polling in terms of efficiency?
-Interrupts are more efficient because they allow the microcontroller to perform other tasks and only check the button state when there is an actual change, rather than constantly polling.
What are the three basic types of interrupts mentioned in the script?
-The three basic types of interrupts are change, rising, and falling. Change interrupt triggers on either a rising or falling signal, while rising and falling interrupts trigger specifically on transitions from low to high or high to low, respectively.
What is an Interrupt Service Routine (ISR)?
-An ISR is a specific function that runs when an interrupt condition is met, allowing the microcontroller to respond to the interrupt event.
Why should the code in an ISR be kept as short as possible?
-The code in an ISR should be short to minimize the time the microcontroller is occupied with the ISR, ensuring it can quickly return to its main tasks and not interfere with other processes that require precise timing.
Why are the delay and Millie's functions not suitable for use inside ISRs?
-Delay and Millie's functions are not suitable for ISRs because they are based on interrupt functions, and only one interrupt can be executed at a time, which would conflict with the ISR's operation.
What is the purpose of declaring a variable as 'volatile' inside an ISR?
-The 'volatile' modifier is used to declare a variable that can be changed at any time by an interrupt service routine, ensuring the main program is aware of potential changes made within the ISR.
Which pins on an Arduino board are typically interrupt-capable?
-Typically, digital pins 2 and 3 on an Arduino board are interrupt-capable, but this can vary depending on the board model, and a quick Google search can provide specific information for different boards.
How can you determine which pins on an Arduino board support interrupts?
-You can determine which pins support interrupts by searching online for the specific Arduino board model along with terms like 'interrupt pins' or checking the board's datasheet or documentation.
Outlines
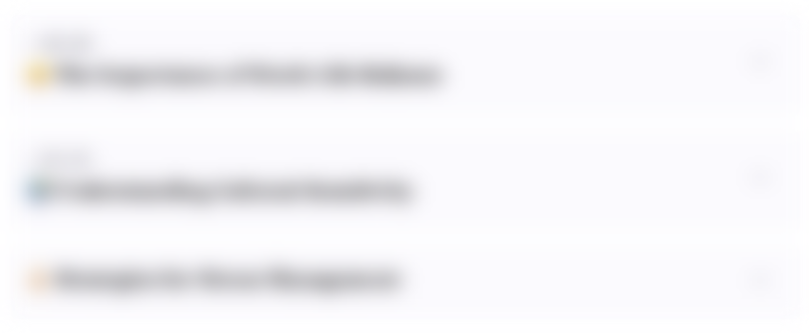
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنMindmap
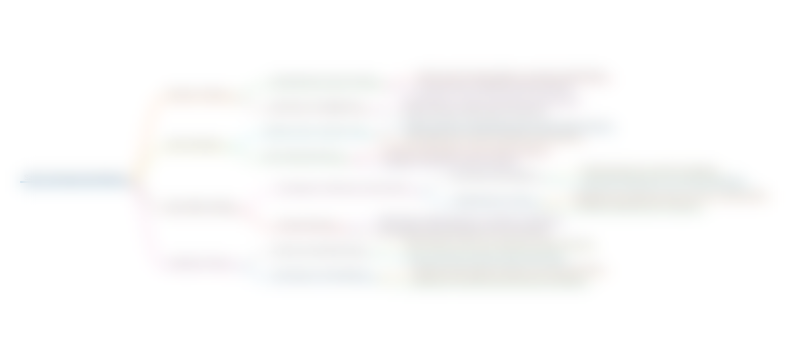
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنKeywords
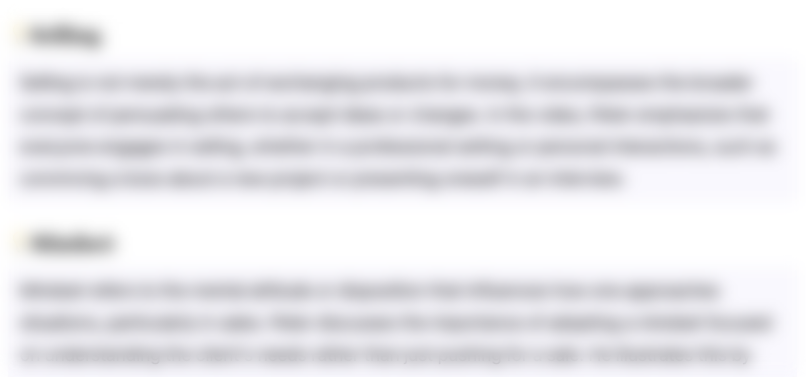
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنHighlights
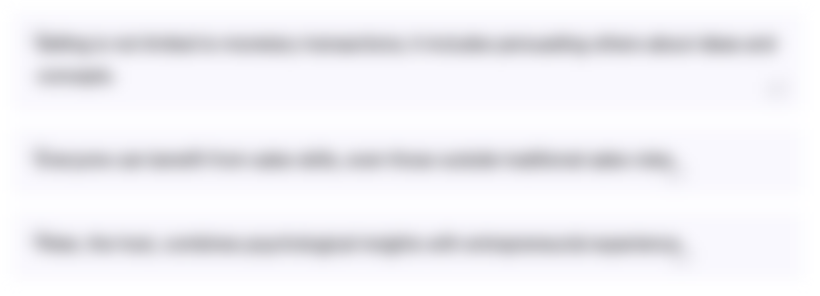
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنTranscripts
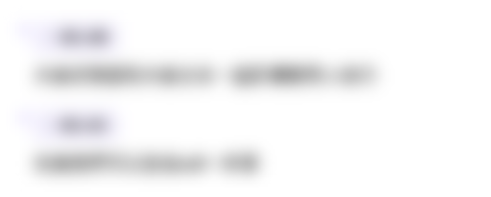
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنتصفح المزيد من مقاطع الفيديو ذات الصلة
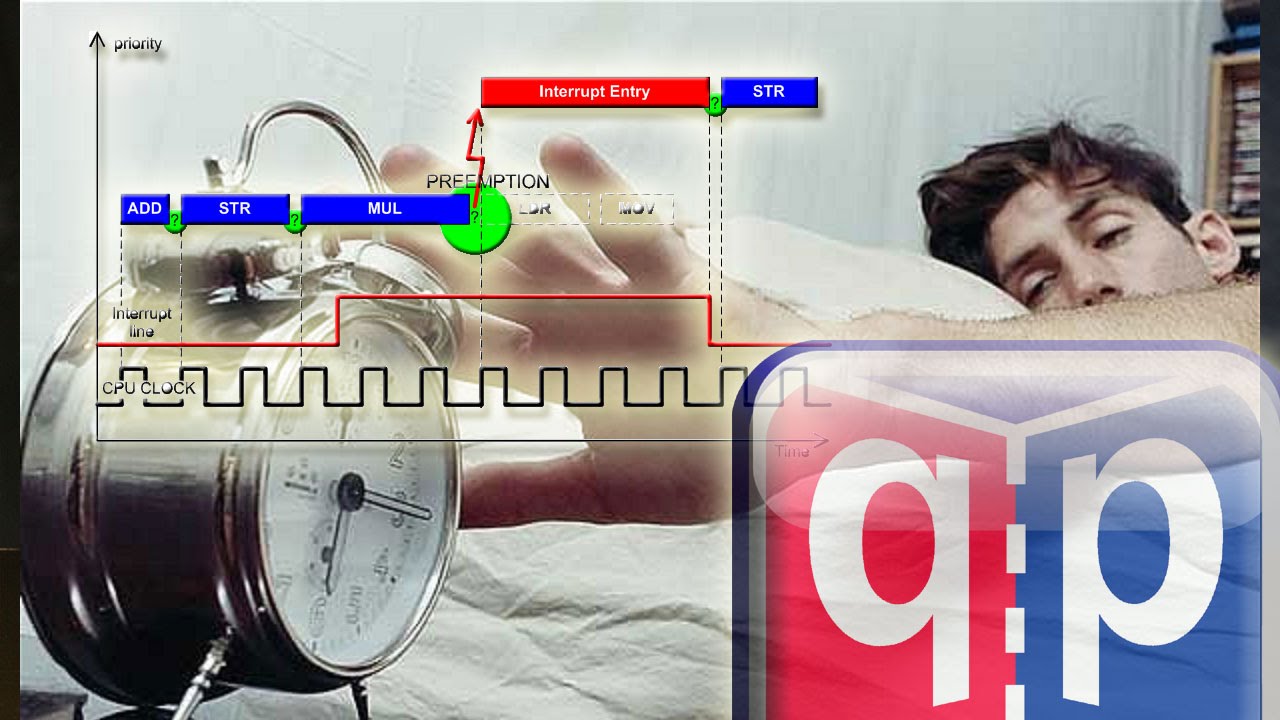
#16 Interrupts Part-1: What are interrupts, and how they work
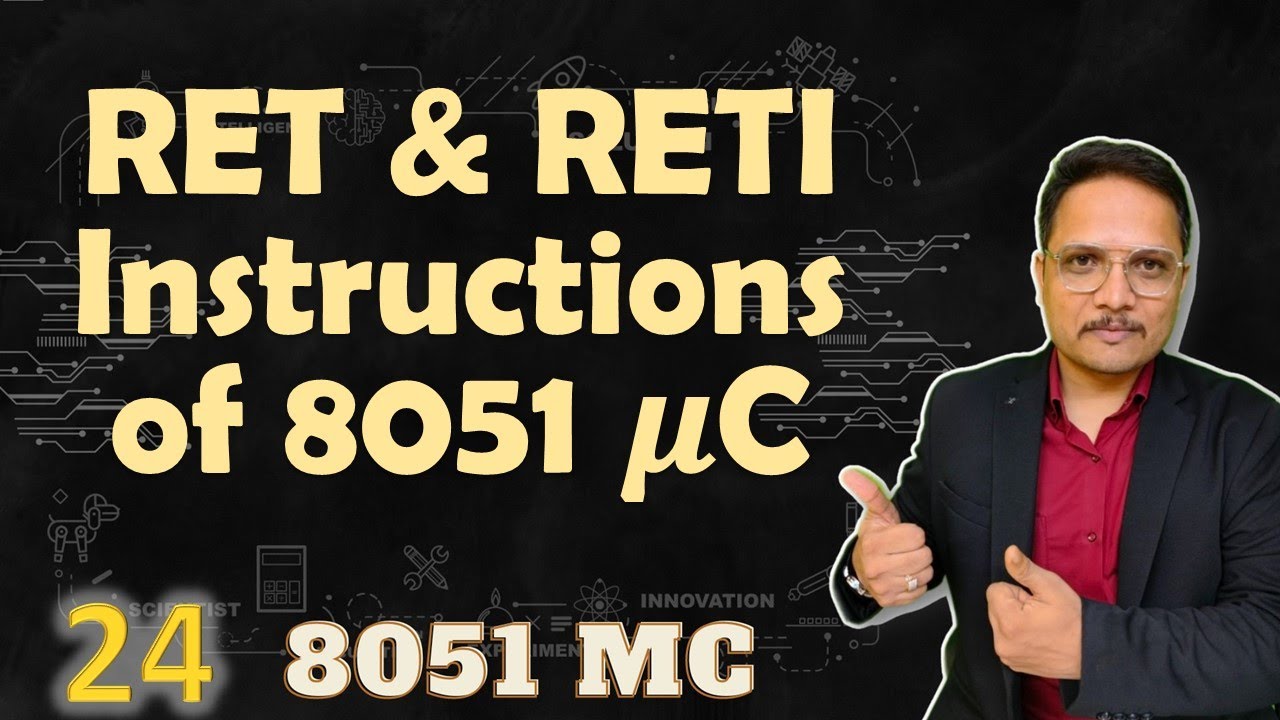
RET & RETI Instructions of 8051 Microcontroller: Comparison and Explanation | Instructions of 8051
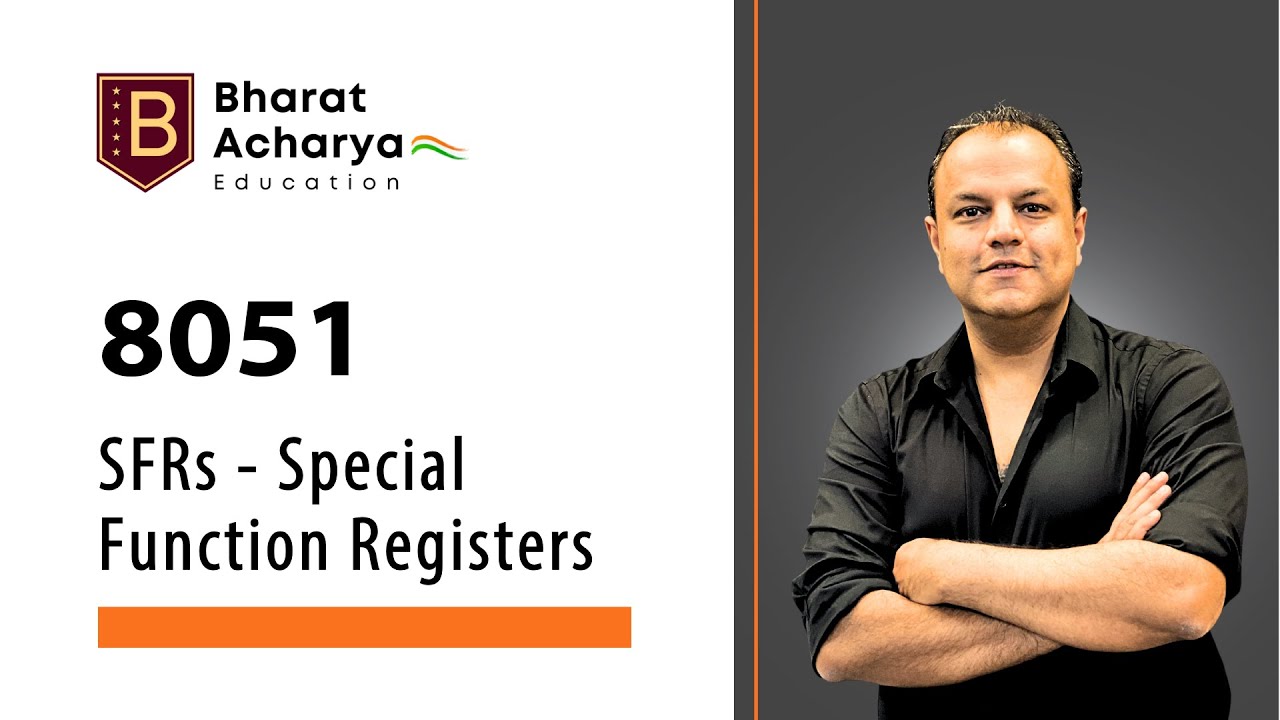
8051 | SFRs - Special Function Registers | Bharat Acharya Education
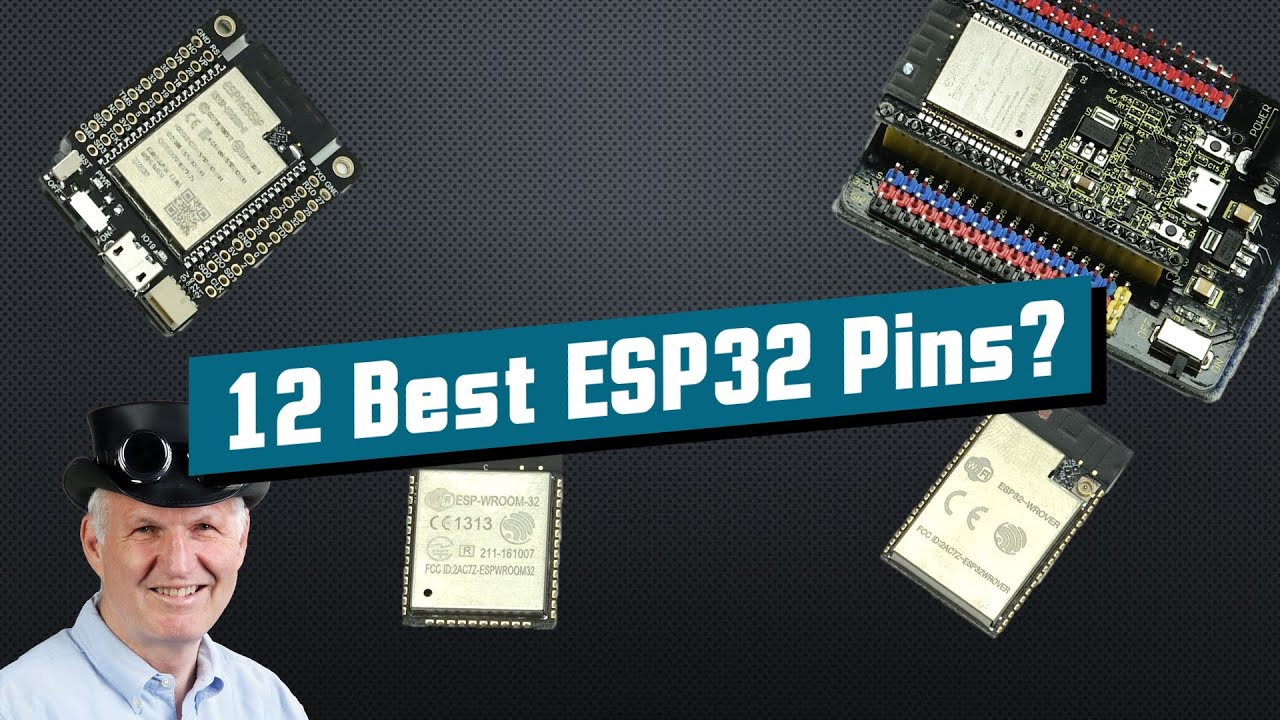
#363 Which ESP32 pins are safe to use?

PIC16F877A a basic Introduction
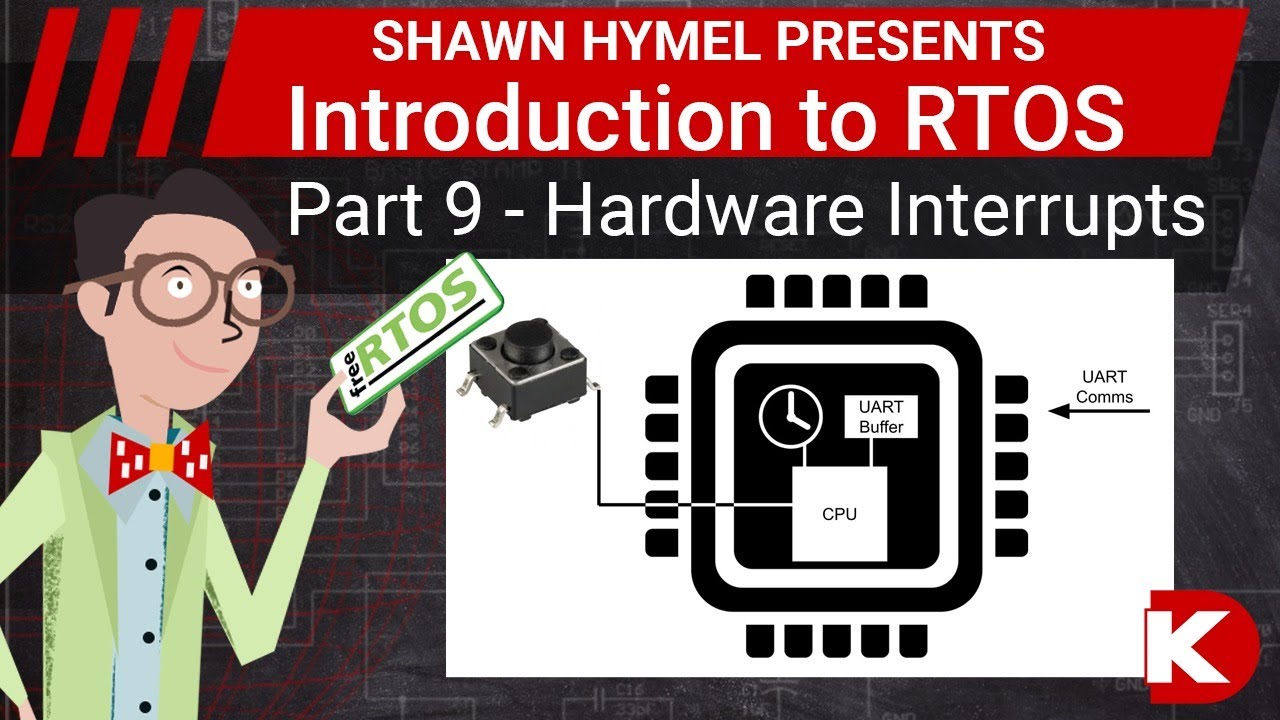
Introduction to RTOS Part 9 - Hardware Interrupts | Digi-Key Electronics
5.0 / 5 (0 votes)