#71 What is Annotation in Java
Summary
TLDRThis video script delves into the concept of annotations in Java programming, highlighting their role as metadata to provide extra information to compilers or runtime environments without altering code behavior. The script uses the example of method overriding to demonstrate how annotations like '@Override' can help prevent bugs by making developers' intentions explicit to the compiler. It also touches on various types of annotations used in Java and their applications in frameworks like Hibernate and Spring, emphasizing the importance of solving logical issues at compile time rather than at runtime.
Takeaways
- 📝 Annotations are used to provide extra information to the compiler or runtime without changing the code's functionality.
- 🔄 The script discusses method overriding, where a subclass method has the same name as a superclass method, but with a different implementation.
- 💡 The importance of meaningful method names is highlighted, as long or complex names can lead to bugs if not properly overridden.
- 🐛 Bugs in programming are logical problems that result in unexpected outputs, which can be difficult to debug, especially in large codebases.
- 🛠️ The '@Override' annotation in Java helps to indicate the compiler that a method is intended to override a superclass method, catching errors at compile time.
- 🔍 Using annotations like '@Override' can assist in debugging by providing compiler feedback if the intended override is not correctly implemented.
- 🚫 The '@Deprecated' annotation is used to mark a class or method as deprecated, suggesting it should not be used as it may be removed in future versions.
- 🏷️ Annotations can be applied at different levels: class, method, or variable, and have different retention policies depending on their use case.
- 🛑 The '@SuppressWarnings' annotation is used to hide warnings in the code, which can be useful when certain warnings are not relevant.
- 🔄 Annotations play a significant role in frameworks like Hibernate and Spring, where they drive much of the framework's behavior.
- 🔑 Creating custom annotations allows developers to set specific retention policies, determining when the annotation is relevant, such as at compile time or runtime.
Q & A
What does the term 'annotation' mean in the context of programming?
-In programming, 'annotation' refers to metadata that can be added to the source code to provide additional information to the compiler or runtime environment without altering the code's behavior.
Why is it important to use annotations when writing code?
-Annotations are important because they can help communicate your intentions to the compiler, which can then provide errors or warnings if something is amiss, helping to catch bugs early in the development process.
Can you provide an example of how annotations are used in Java for method overriding?
-In Java, the '@Override' annotation is used to indicate that a method is intended to override a method from a superclass. If the method does not properly override a method from the superclass, the compiler will generate an error.
What is the purpose of the '@Override' annotation in Java?
-The '@Override' annotation in Java is used to explicitly state that a method is overriding a method from a superclass. It helps the compiler check for errors in method overriding, ensuring that the method signature matches a method in the superclass.
What happens if you forget to use the '@Override' annotation when you intend to override a method?
-If you forget to use the '@Override' annotation when overriding a method, the compiler will not check for errors related to method overriding, which could lead to runtime errors or bugs if the method signature is incorrect.
How do annotations help in debugging complex codebases with many classes?
-Annotations can help in debugging by providing explicit information to the compiler about the programmer's intentions. If there is a mismatch, the compiler can generate errors that guide the programmer to the source of the problem, making it easier to debug, especially in complex codebases.
What is the difference between a compile-time and a runtime issue in programming?
-A compile-time issue is an error that is detected by the compiler before the code is executed, such as syntax errors or type mismatches. A runtime issue, on the other hand, occurs during the execution of the program, such as null pointer exceptions or division by zero errors.
Why is it better to solve problems at compile time rather than at runtime?
-Solving problems at compile time is better because it prevents the execution of potentially faulty code, which can lead to crashes or incorrect behavior at runtime. It also allows for earlier detection and correction of issues, reducing the risk of bugs reaching production.
Can annotations be used for purposes other than method overriding in Java?
-Yes, annotations in Java can serve various purposes, such as marking a class as deprecated, indicating that a method is part of a framework's API, or providing metadata for reflection, among others.
What is the role of the 'deprecated' annotation in Java?
-The 'deprecated' annotation in Java is used to mark a class, method, or field as deprecated, indicating that it is no longer recommended for use and may be removed in future versions of the Java language or library.
How can annotations be used in the context of frameworks like Hibernate or Spring?
-In frameworks like Hibernate or Spring, annotations are extensively used to configure the behavior of the framework without requiring extensive XML configuration. They can be applied at the class, method, or field level to define aspects such as transaction management, dependency injection, and data access.
Outlines
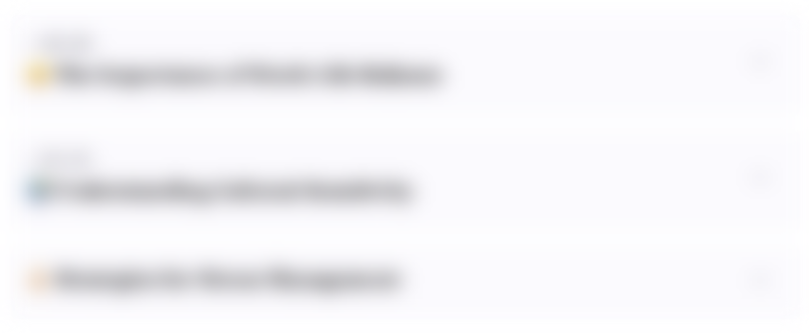
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنMindmap
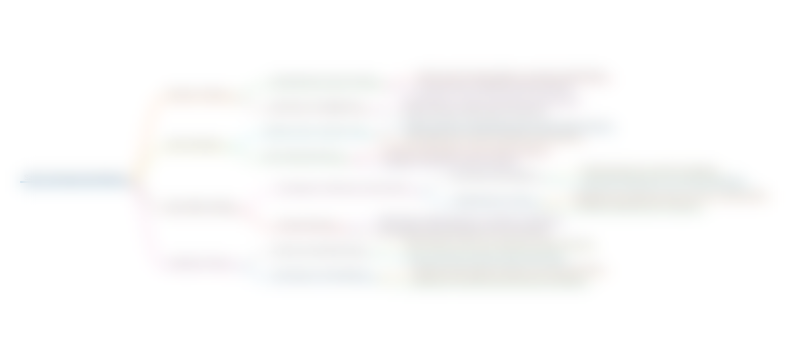
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنKeywords
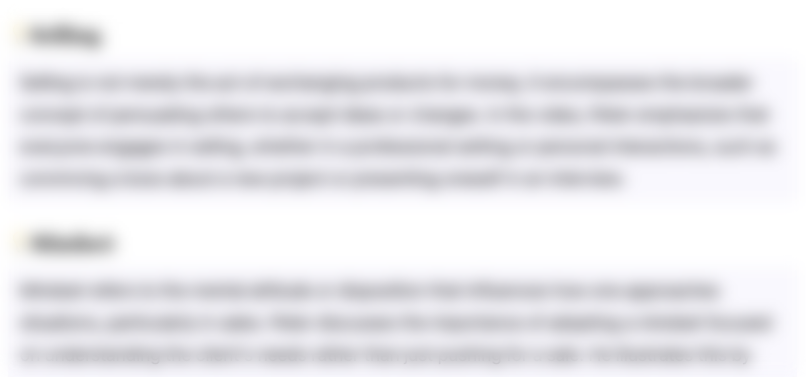
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنHighlights
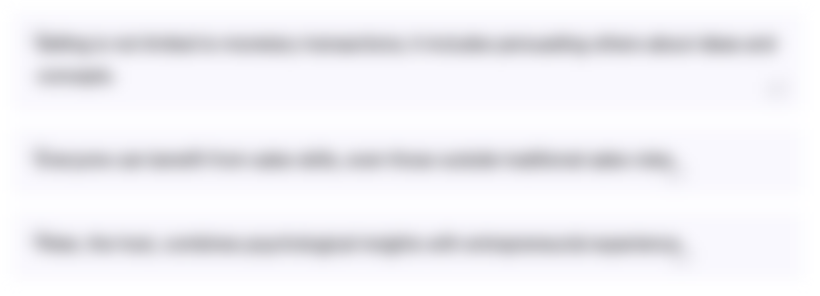
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنTranscripts
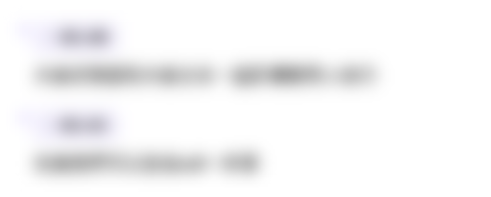
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنتصفح المزيد من مقاطع الفيديو ذات الصلة
5.0 / 5 (0 votes)