MERN Stack Tutorial #2 - Express App Setup
Summary
TLDRIn this tutorial, we set up the backend of a MERN stack application using Node.js and Express. We create an Express app, install necessary dependencies, and configure it to listen on a port. The script demonstrates setting up routes, handling GET requests, and utilizing environment variables for sensitive data. Tools like Postman are introduced to test different HTTP requests (GET, POST, etc.). Additionally, basic middleware for logging requests is implemented to provide visibility into the application. By the end, we have a functional backend ready for integration with a frontend React application.
Takeaways
- 😀 Set up a backend Express app using Node.js to create an API that communicates with the frontend React app and the MongoDB database.
- 😀 Create a new folder for the backend code and a `server.js` file as the entry point for the backend application.
- 😀 Initialize a `package.json` file inside the backend folder to track dependencies and custom scripts, and use `npm init -y` for automatic setup.
- 😀 Install Express using `npm install express` and require it in the `server.js` file to create the Express app.
- 😀 Use `app.listen(4000)` to have the server listen for incoming requests on port 4000, and display a message when the server is up.
- 😀 Install and use `nodemon` to automatically restart the server when code changes are made, improving the development workflow.
- 😀 Set up a `dev` script in `package.json` to easily run the backend server with `npm run dev`.
- 😀 Create route handlers using `app.get()` to handle incoming GET requests and send JSON responses to the client.
- 😀 Store sensitive information, like the port number, in a `.env` file and use the `dotenv` package to load it into the environment variables.
- 😀 Test different types of HTTP requests (GET, POST, DELETE, etc.) using Postman to simulate requests before the frontend is fully developed.
- 😀 Register global middleware to log incoming requests, including the request method and path, to help with debugging and monitoring.
Q & A
What is the purpose of setting up an Express app in this tutorial?
-The purpose of setting up an Express app is to create a backend API that can handle requests from a frontend React application and interact with a MongoDB database for data fetching and response handling.
Why is the 'npm init -y' command used during the project setup?
-'npm init -y' is used to automatically generate a 'package.json' file with default values, which helps manage project dependencies and scripts without manually answering prompts.
How do you start an Express app once the dependencies are installed?
-After installing dependencies like Express, you start the Express app by requiring Express in the 'server.js' file and invoking the function 'express()' to create the app. Then, you use 'app.listen()' to set the port the app will listen on.
What is the advantage of using 'nodemon' over 'node' for running the server?
-'nodemon' automatically restarts the server whenever changes are made to the source files, making it more efficient for development compared to using 'node' which requires manually restarting the server after changes.
What does the '.env' file do and why is it important?
-The '.env' file stores environment variables like port numbers or sensitive data (e.g., database connection strings) outside of the main codebase. This helps keep sensitive information private and makes the application more configurable.
How do you access environment variables in the code after setting up the '.env' file?
-After installing the 'dotenv' package and requiring it in the 'server.js' file, you access environment variables through 'process.env', followed by the variable name, like 'process.env.PORT'. This ensures values like the port number are fetched from the '.env' file.
What role does Postman play in testing the Express routes?
-Postman is used to simulate different HTTP requests (GET, POST, DELETE, etc.) to the server. This allows testing of various routes without needing a frontend to make those requests, helping with backend development and debugging.
Why is middleware used in Express apps?
-Middleware in Express is used to execute code between receiving a request and sending a response. It's typically used for tasks like logging, authentication, or modifying the request/response objects before passing them on.
What does the 'next()' function do in middleware?
-The 'next()' function is used to pass control to the next middleware function in the stack. If 'next()' is not called, the request will not continue and the response will not be sent back to the client.
How can you handle dynamic port numbers in your Express app?
-You can handle dynamic port numbers by using environment variables. Instead of hardcoding the port number in the 'server.js' file, store it in a '.env' file and access it via 'process.env.PORT' after loading it with the 'dotenv' package.
Outlines
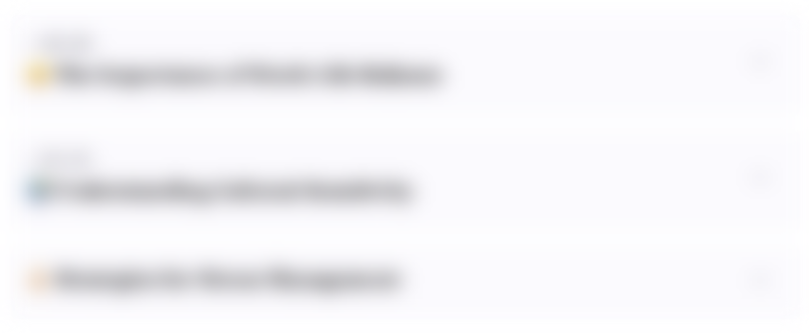
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنMindmap
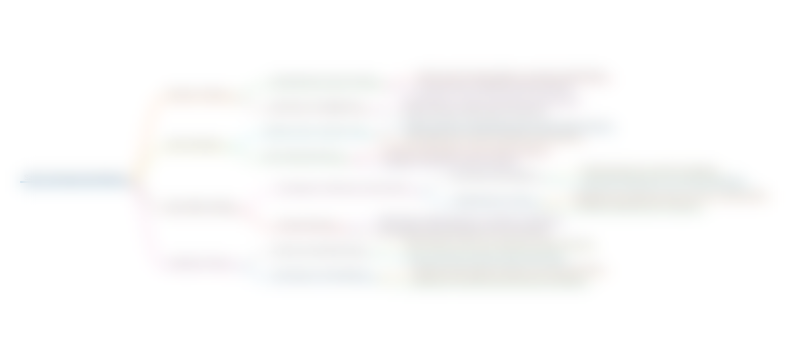
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنKeywords
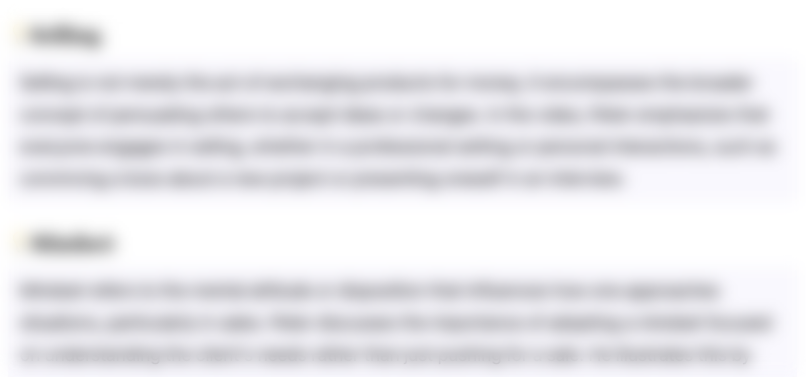
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنHighlights
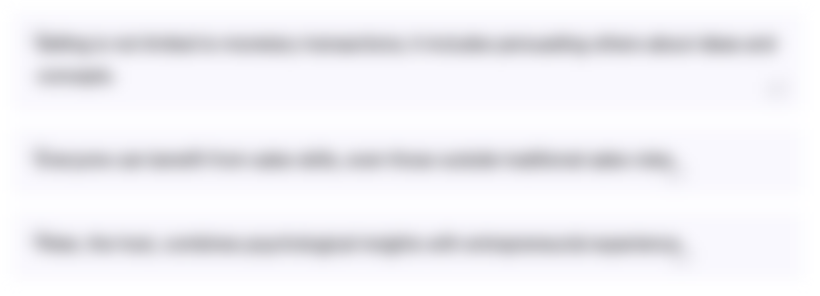
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنTranscripts
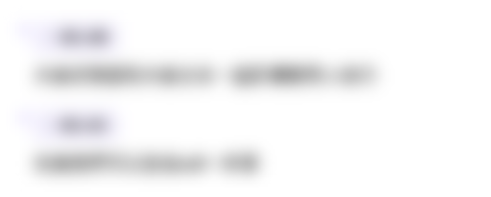
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنتصفح المزيد من مقاطع الفيديو ذات الصلة
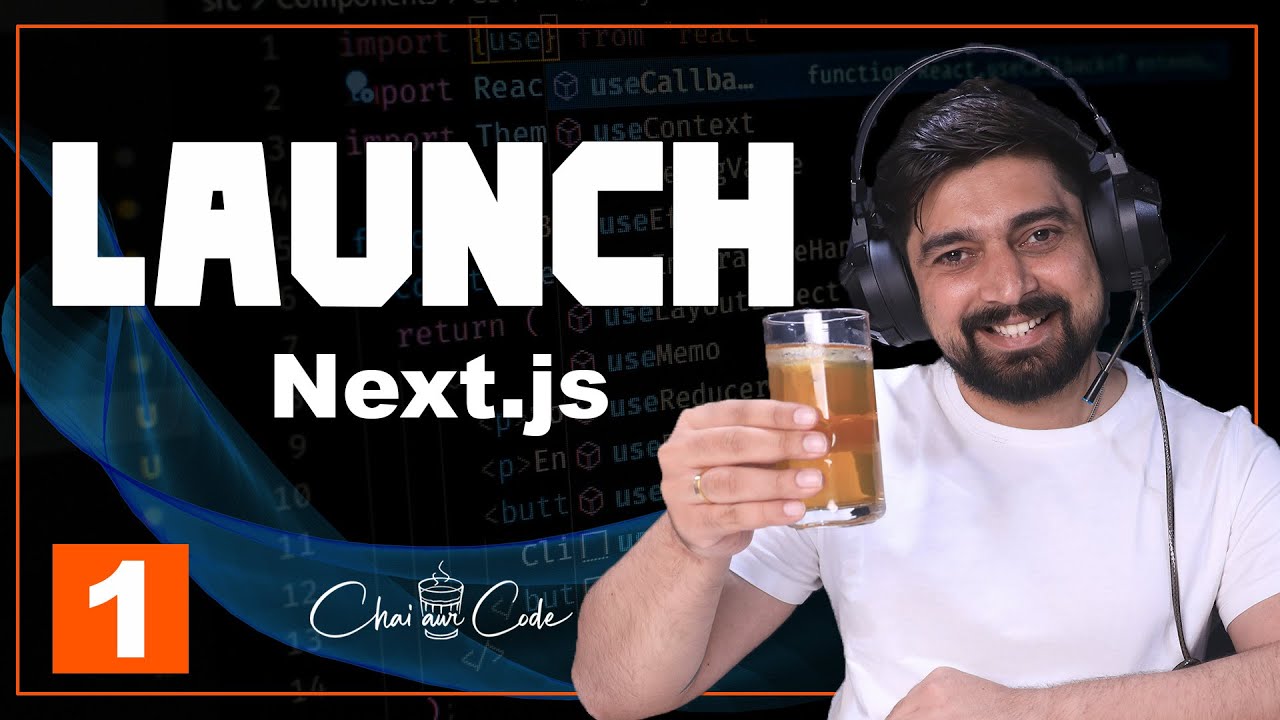
Complete full stack freelance ready course
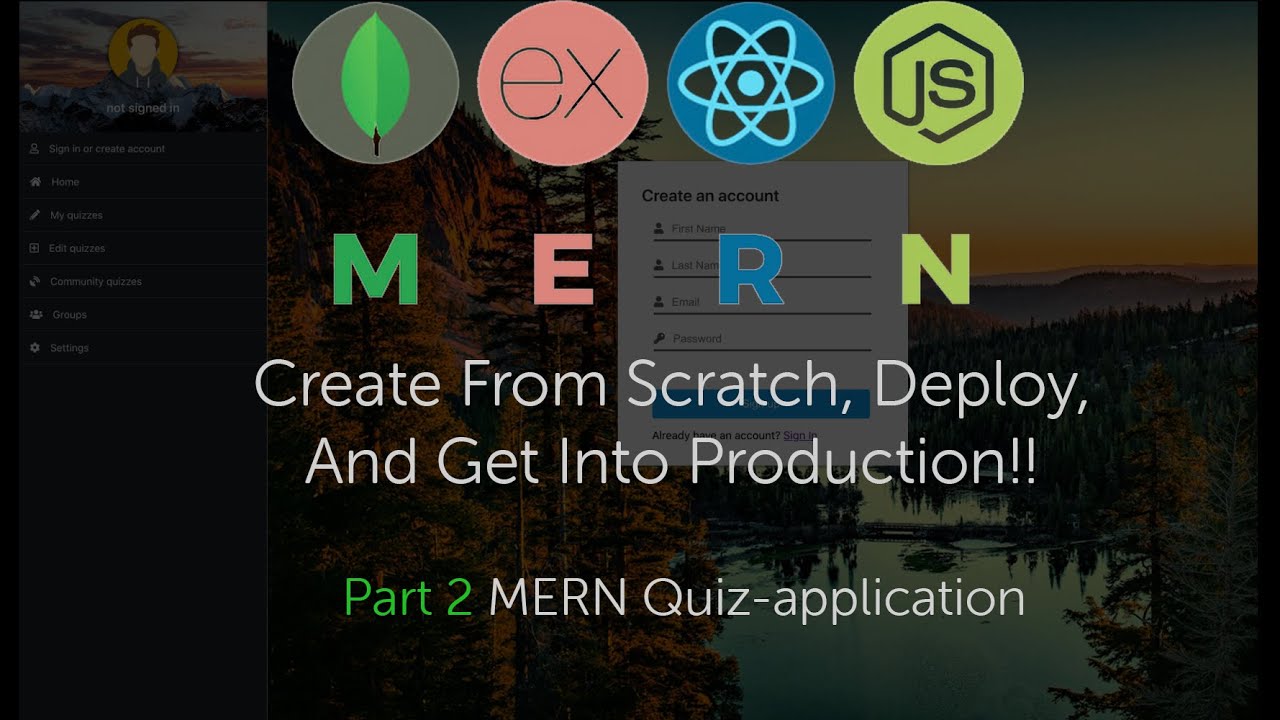
MERN quiz creator app Part 2: Creating a database, Creating a basic api, adding JWT authentication.
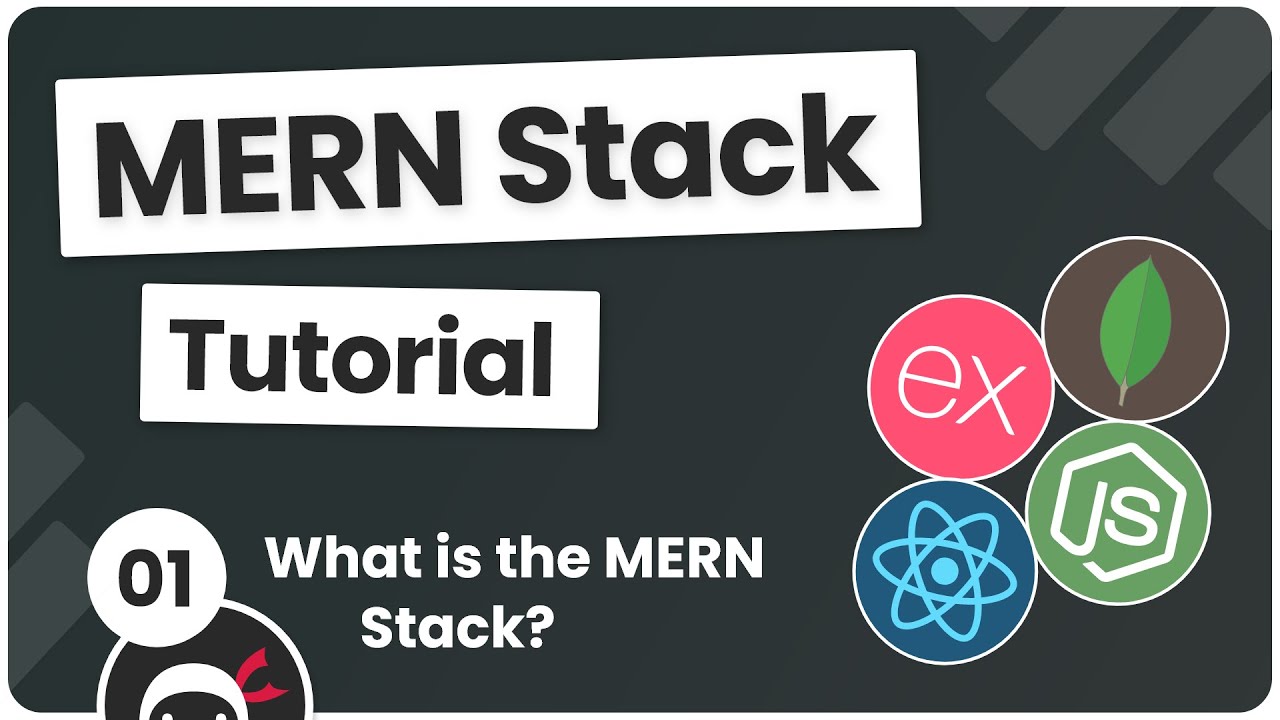
MERN Stack Tutorial #1 - What is the MERN Stack?

Getting Started with Next 14 and Strapi: project example overview
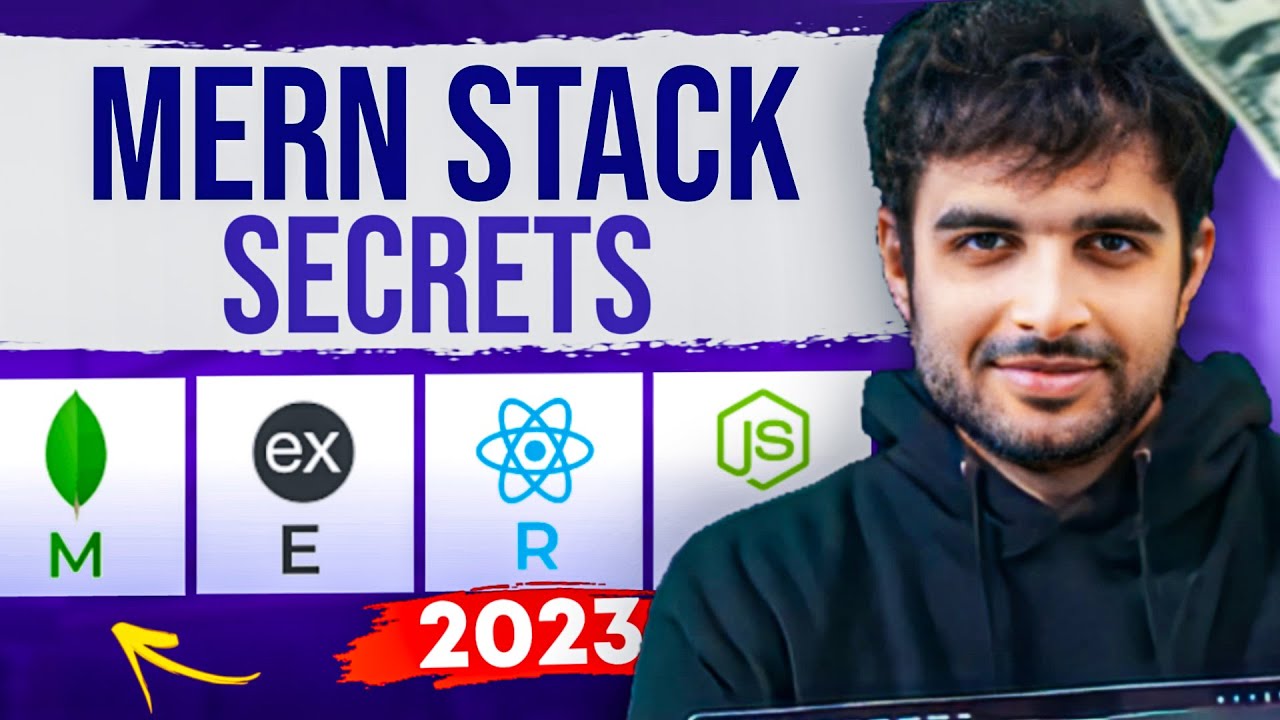
Complete MERN Stack Developer Roadmap For Beginners (2023)
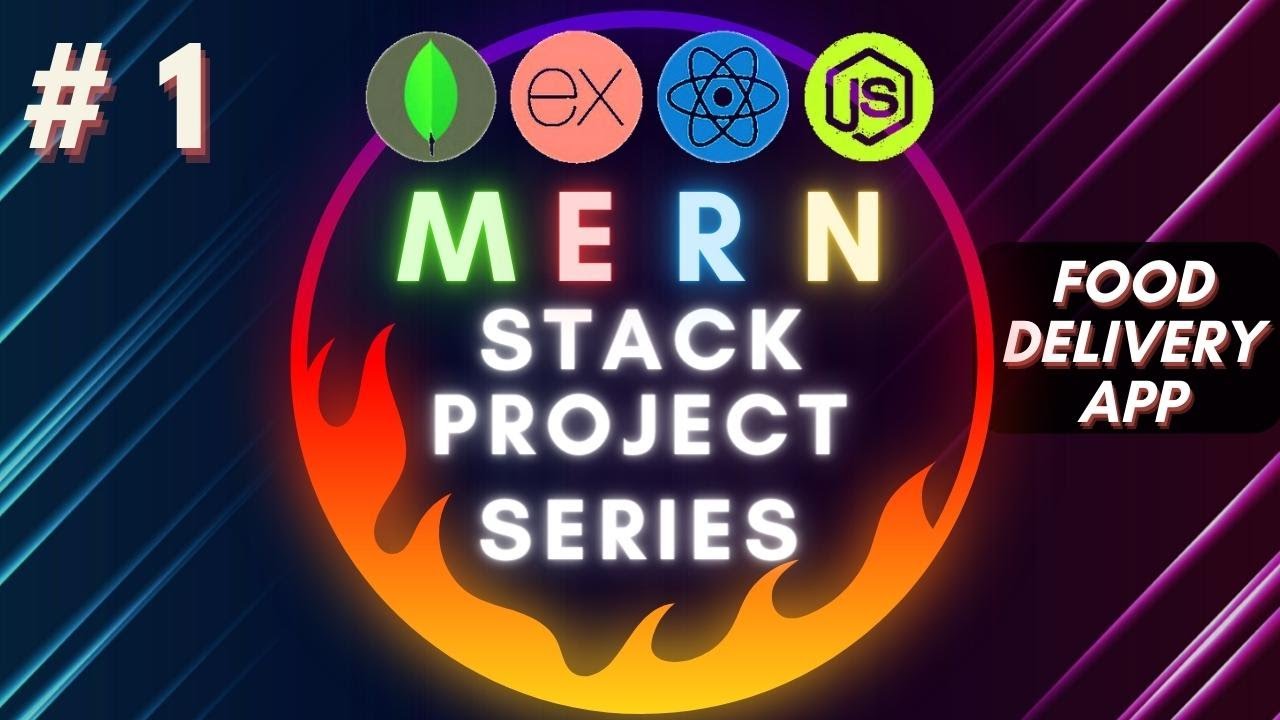
# 1 MERN Project Series | Intro to Food Delivery App & Brief on MERN Stack | Hindi 2023
5.0 / 5 (0 votes)