C++ State Machines
Summary
TLDRThis video demonstrates two approaches for implementing state machines: one using simple `if` statements with enumerated types, and the other using an object-oriented design. The script focuses on modeling a state machine for a weapon and a light bulb, exploring the advantages and drawbacks of both methods. The object-oriented solution offers scalability and clearer management of states and transitions through classes like `State`, `Transition`, `FSM`, and `Bulb`. The video also includes a detailed walkthrough of coding these classes, ensuring the correct transition logic is applied based on specific conditions, such as voltage levels for the light bulb.
Takeaways
- 😀 A state machine consists of states, transitions, and conditions, and is used to model systems with distinct states and changes over time.
- 😀 In a state machine diagram, the initial state is denoted by a black circle, and the final state is a black circle with an outer ring.
- 😀 The non-object-oriented approach to state machines uses enumerated types and `if` statements to handle state transitions.
- 😀 In the non-object-oriented approach, callback functions are often used to execute additional logic during state transitions, such as decrementing rounds in a weapon example.
- 😀 As state machines grow more complex with multiple states and transitions, the non-object-oriented approach becomes harder to maintain due to a lack of centralization and scalability issues.
- 😀 An object-oriented approach models states and transitions as objects, making the system more scalable and easier to manage as complexity increases.
- 😀 In the object-oriented approach, guard functions are implemented as methods that return a boolean value to determine whether a transition can occur based on certain conditions.
- 😀 A key benefit of object-oriented design is centralization: guard functions and other transition logic are contained within the objects, making the system more maintainable and flexible.
- 😀 An example is given with a light bulb state machine, where the bulb can be in `On`, `Off`, or `Broken` states, and transitions depend on voltage conditions.
- 😀 The object-oriented approach uses a finite state machine (FSM) class to manage state transitions and maintains linked lists of transitions for each state, which makes it easier to handle dynamic and complex state changes.
Q & A
What are state machines, and how are they represented in the video?
-State machines are systems that model different states and the transitions between them based on certain conditions. In the video, they are represented using UML diagrams, with states shown as rectangles and transitions as arrows. The initial state is marked by a black circle, and the final state is shown with a black circle and an outer ring.
What are the main components of a state machine as described in the video?
-The main components of a state machine include states (represented by rectangles), transitions (represented by arrows), and guards (conditions that must be met for a transition to occur). Additionally, callback functions may be used to execute extra code during transitions.
What are the two approaches to implementing state machines discussed in the video?
-The two approaches discussed are the non-object-oriented approach, which uses an enumerated type and `if` statements for state transitions, and the object-oriented approach, which models states and transitions as objects and uses functions to handle guard conditions and state changes.
Why does the non-object-oriented approach become problematic as the state machine scales?
-The non-object-oriented approach becomes problematic as the state machine grows because it lacks centralization. As the number of states and transitions increases, managing them with a large number of `if` statements becomes difficult to maintain, prone to errors, and hard to read.
How does the object-oriented approach address the scalability issues of the non-object-oriented approach?
-The object-oriented approach models states and transitions as objects, making it easier to manage as the system grows. It centralizes transition logic in guard functions, which are located in specific objects. This structure improves readability, maintainability, and scalability.
In the light bulb example, what are the three states that the bulb can be in?
-The light bulb can be in one of three states: 'on,' 'off,' or 'broken.' The transitions between these states depend on the voltage supplied to the bulb.
What are the transition conditions for the light bulb's state machine?
-The conditions for state transitions in the light bulb example are based on voltage. The bulb transitions from 'on' to 'broken' if the voltage exceeds 300V, from 'on' to 'off' if the voltage is 0V, from 'off' to 'on' if the voltage is 240V, and from 'off' to 'broken' if the voltage exceeds 300V.
What role do guard functions play in the object-oriented state machine implementation?
-Guard functions check whether the conditions for a transition are met. They return a boolean value (true or false) to determine if a state transition should occur. These guard functions centralize the logic for transitions and make the system more maintainable.
How does the FSM (Finite State Machine) class manage states and transitions?
-The FSM class manages the states and transitions by holding a reference to the current state and a list of all possible states. It handles state changes by evaluating the guard functions for each transition and invoking the appropriate transition if the guard condition is true.
In the object-oriented implementation, what is the purpose of the Bulb class?
-The Bulb class manages the specific logic for the light bulb's state transitions based on the voltage. It defines the behavior for the 'on,' 'off,' and 'broken' states and contains guard functions that check the voltage conditions to trigger the appropriate state transitions.
Outlines
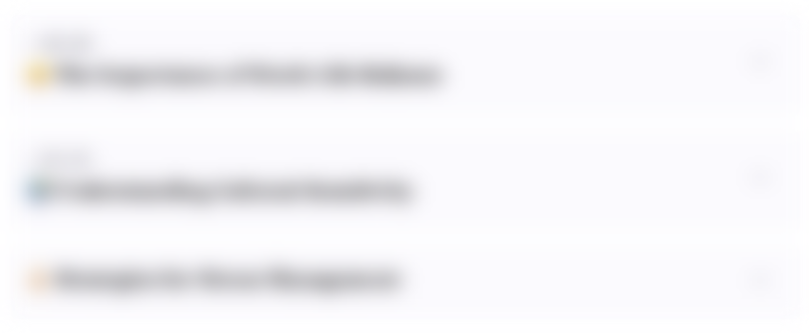
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنMindmap
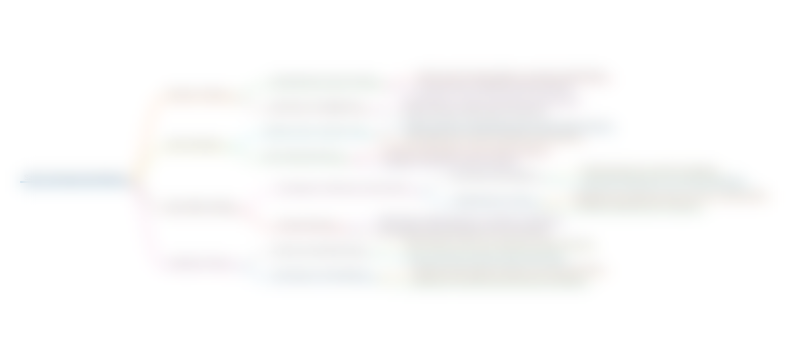
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنKeywords
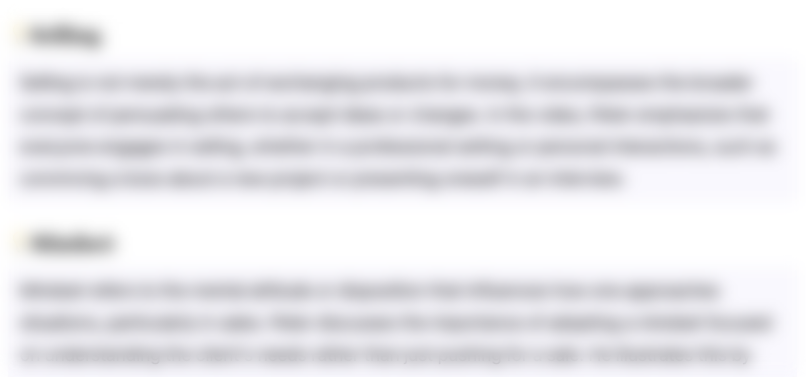
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنHighlights
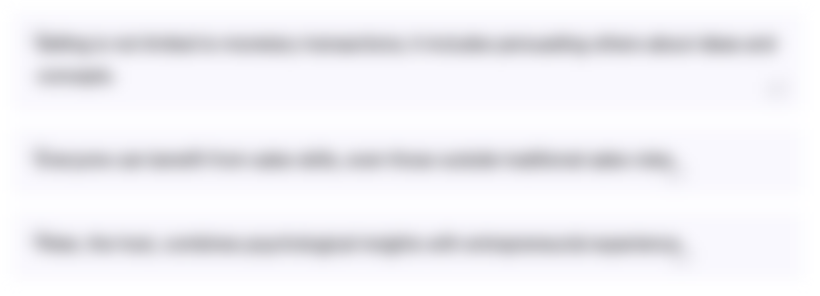
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنTranscripts
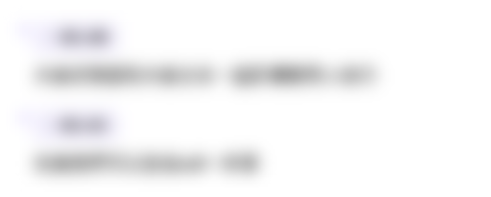
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنتصفح المزيد من مقاطع الفيديو ذات الصلة

BAB 4 SISTEM KOMPUTER | MESIN KONSEPTUAL SEDERHANA | INFORMATIKA SMA KELAS 10 | KEMENDIKBUD
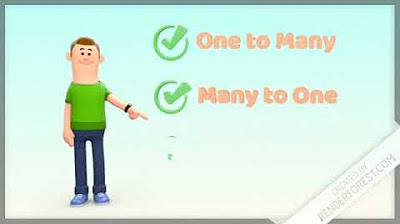
Model & Prediksi Data | Model data berdasarkan objek [2.1/3]
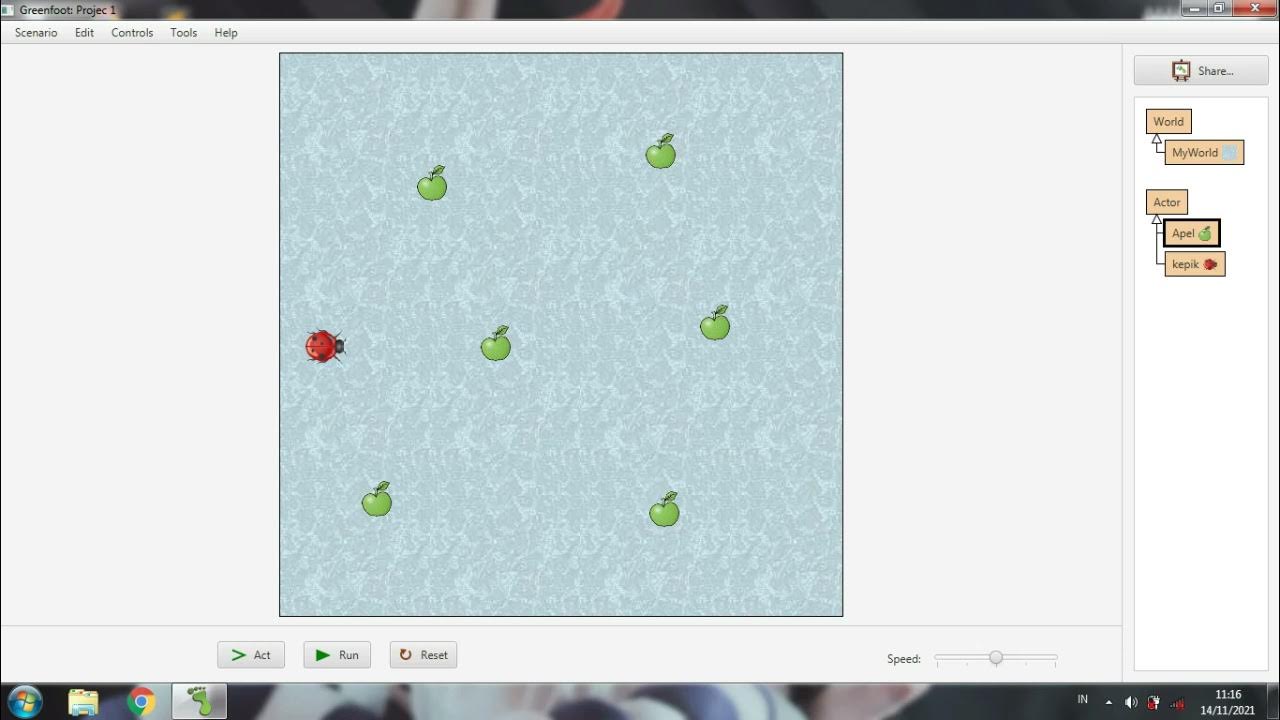
Membuat game sederhana menggunakan greenfoot #Greenfoot
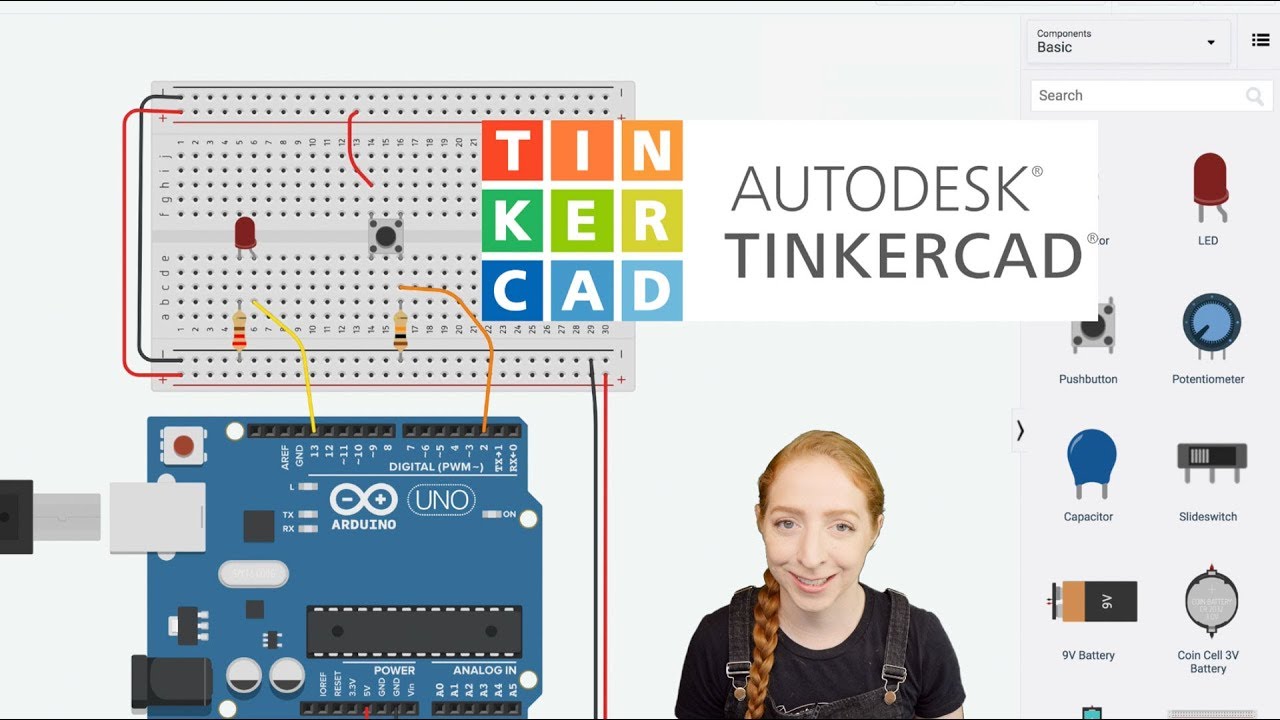
Pushbutton Digital Input With Arduino in Tinkercad
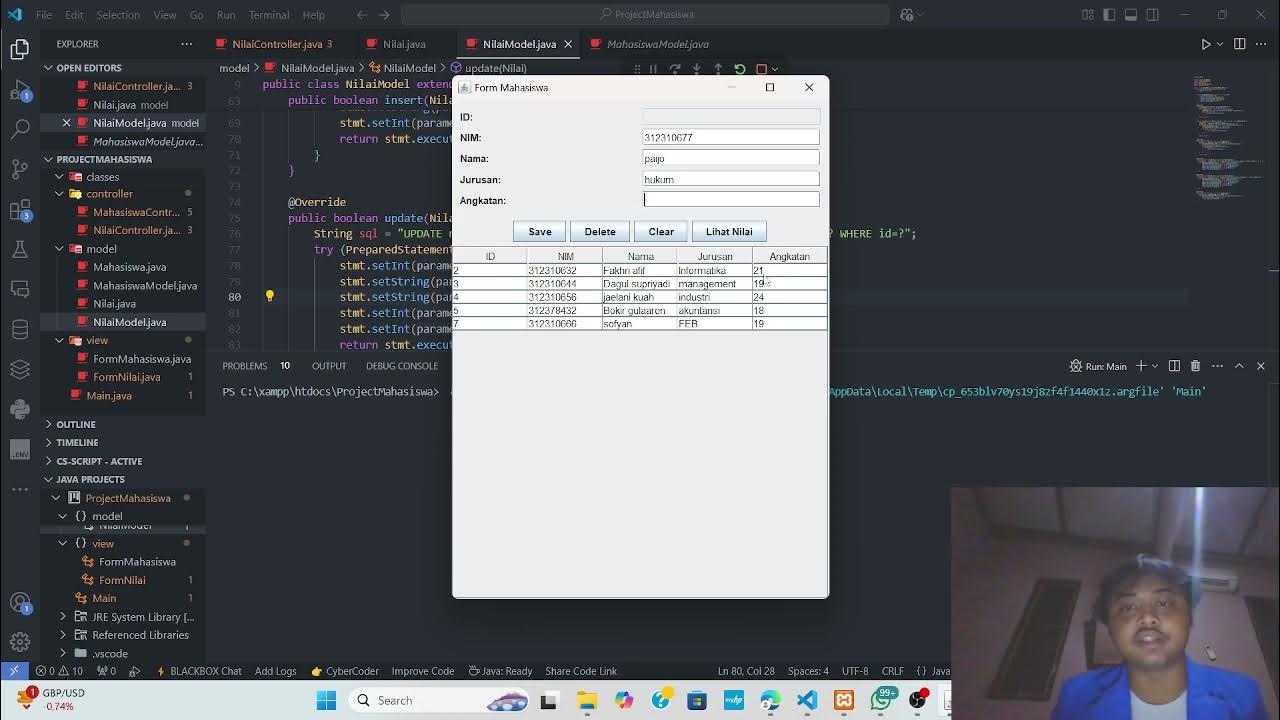
Langkah-Langkah Membuat Aplikasi CRUD Mahasiswa Berbasis MVC dengan Java
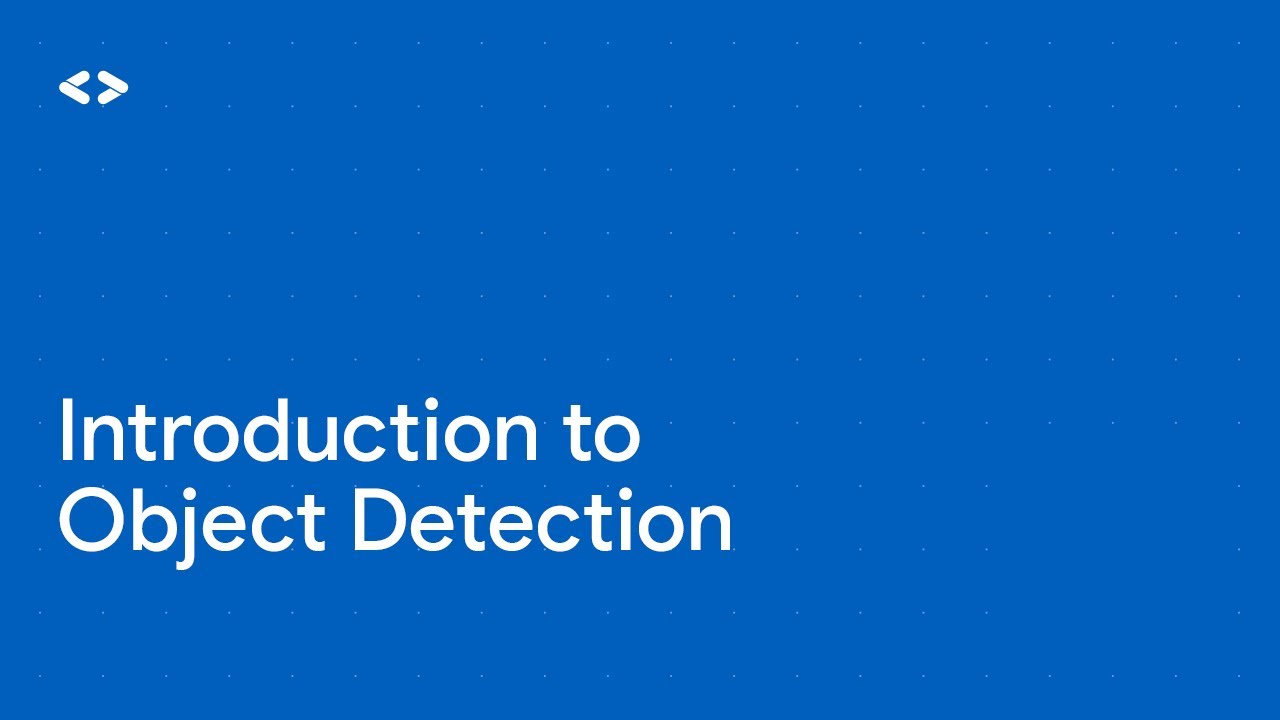
On-device object detection: Introduction
5.0 / 5 (0 votes)