Vectors in C++
Summary
TLDRThis video provides an introduction to vectors in C++, explaining their role as dynamic container objects that hold elements of a specific type. It covers the process of declaring, initializing, and modifying vectors, including how to access elements using indexing or functions like `front()` and `back()`. The video also highlights the ability to resize vectors dynamically with `push_back()`, making them more flexible than traditional arrays. Examples demonstrate these concepts in action, showcasing vector operations such as element manipulation and printing vector contents. Overall, it offers a foundational understanding of how to work with vectors in C++.
Takeaways
- 😀 Vectors in C++ are container objects that hold a collection of elements of the same type.
- 😀 Vectors can hold basic data types (e.g., int, double, string) or user-defined types (e.g., Student, Employee).
- 😀 The type of data in a vector must be specified when declaring it (e.g., vector<double> or vector<int>).
- 😀 A vector is initialized with a specific size, and each element in it is set to a default value (e.g., 0 for numeric types).
- 😀 Each element in a vector is accessed using its index, with the first element starting at index 0.
- 😀 You can dynamically change the size of a vector using the `push_back()` method to add elements at the end.
- 😀 The `front()` method retrieves the first element, while the `back()` method retrieves the last element of the vector.
- 😀 To access specific elements of a vector, you can use either direct indexing or the `at()` method, which includes bounds checking.
- 😀 Vectors are stored in contiguous memory locations, making them efficient for accessing elements directly by index.
- 😀 You can create a function to print all the elements in a vector, which helps avoid repetitive `cout` statements for large vectors.
Q & A
What is a vector in C++?
-A vector in C++ is a container object that holds a collection of elements of a specific type. Vectors can dynamically resize themselves, allowing for flexibility in managing data.
How does a vector differ from an array in C++?
-Unlike arrays, vectors in C++ can dynamically grow or shrink in size. Arrays have a fixed size that must be defined at the time of declaration, while vectors allow for more flexibility in handling elements.
What does it mean that a vector is a 'container object'?
-A container object is a data structure that stores a collection of elements. In the case of vectors, the container holds elements of a specified type, such as integers or user-defined objects.
Can vectors hold different data types?
-No, vectors in C++ can only hold elements of a single type. You cannot have a vector that contains a mix of different types such as books and CDs; it must be a collection of the same type.
How do you declare a vector in C++?
-To declare a vector, you use the syntax: `vector<Type> vectorName(size);`. The type represents the data type of the elements, and the size specifies the number of elements initially in the vector.
What happens if you declare a vector of integers without specifying initial values?
-If you declare a vector of integers without specifying initial values, each element will be initialized to zero by default.
How do you access an element in a vector?
-You access an element in a vector by using the vector's name followed by the index of the element in square brackets. For example, `vectorName[index]` retrieves the element at that index.
What is the significance of the index in a vector?
-The index in a vector is used to refer to the position of an element. Indices start at 0, meaning the first element is at index 0, the second at index 1, and so on.
How do you add elements to the end of a vector?
-To add elements to the end of a vector, you use the `push_back()` method. For example, `vectorName.push_back(value);` adds the specified value to the end of the vector.
What are some useful functions associated with vectors in C++?
-Some useful functions include `front()` (returns the first element), `back()` (returns the last element), and `size()` (returns the number of elements in the vector).
Outlines
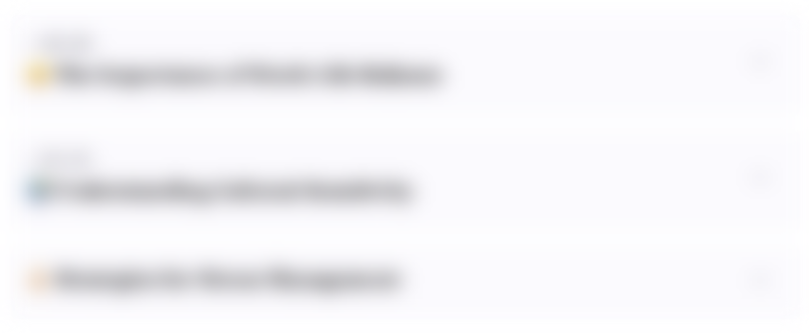
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنMindmap
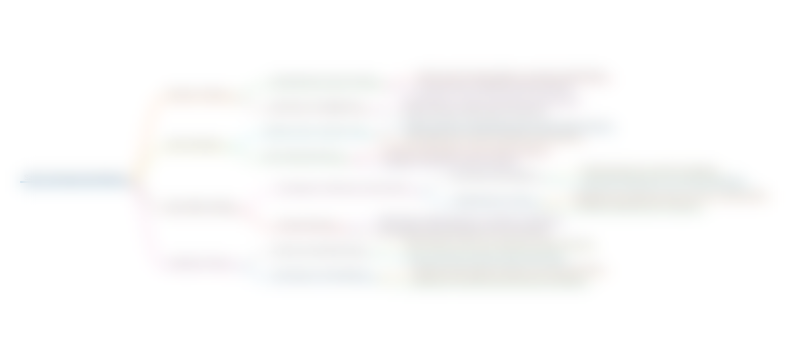
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنKeywords
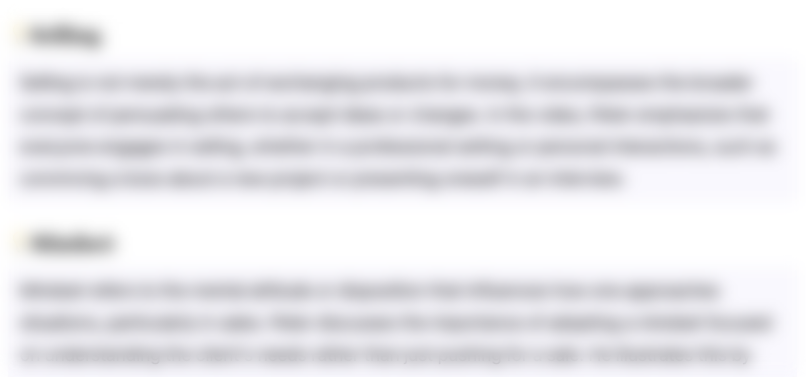
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنHighlights
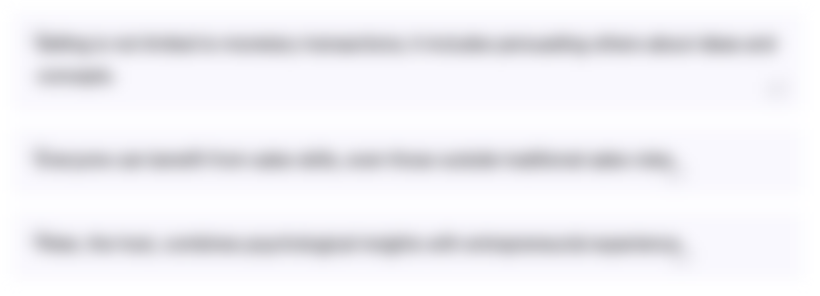
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنTranscripts
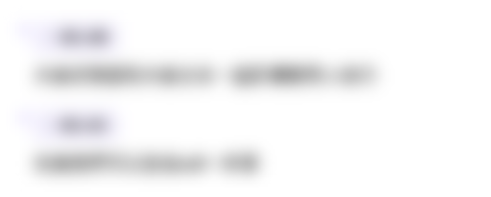
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنتصفح المزيد من مقاطع الفيديو ذات الصلة
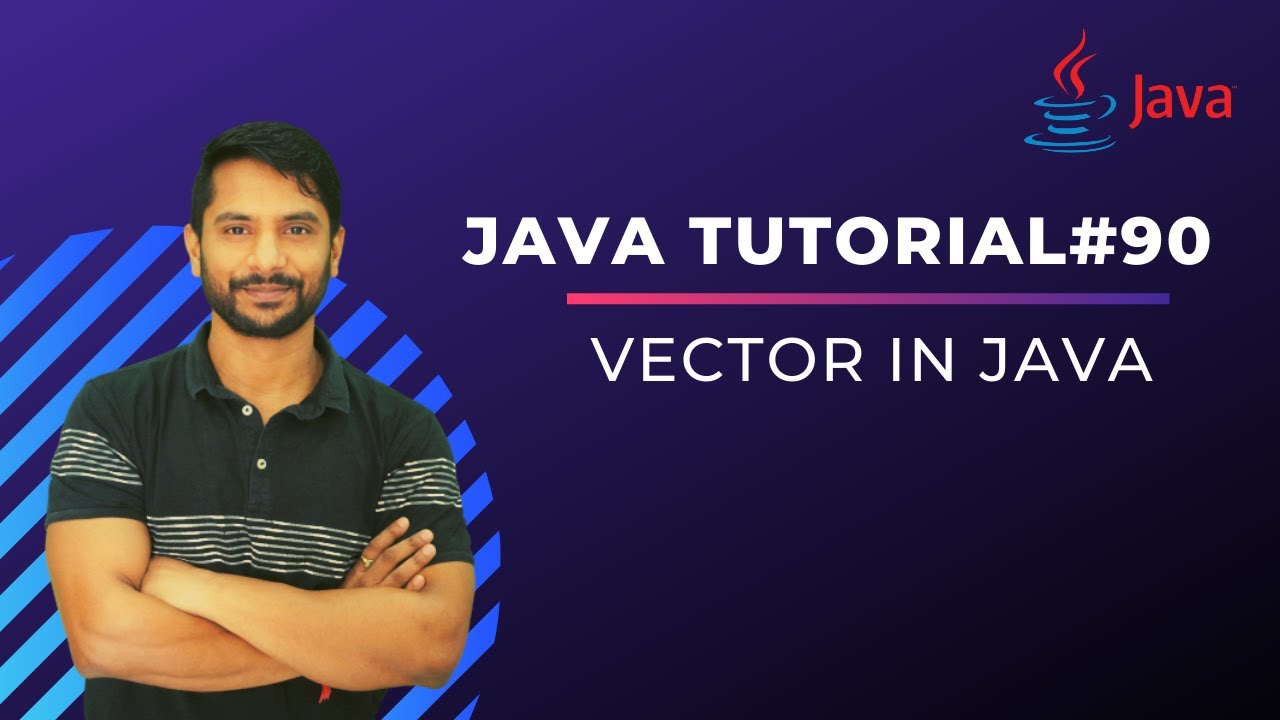
Introduction to Vector | Java Programming | In Hindi

Iterators in c++ | STL | Part-1/2 | OOPs in C++ | Lec-58 | Bhanu Priya
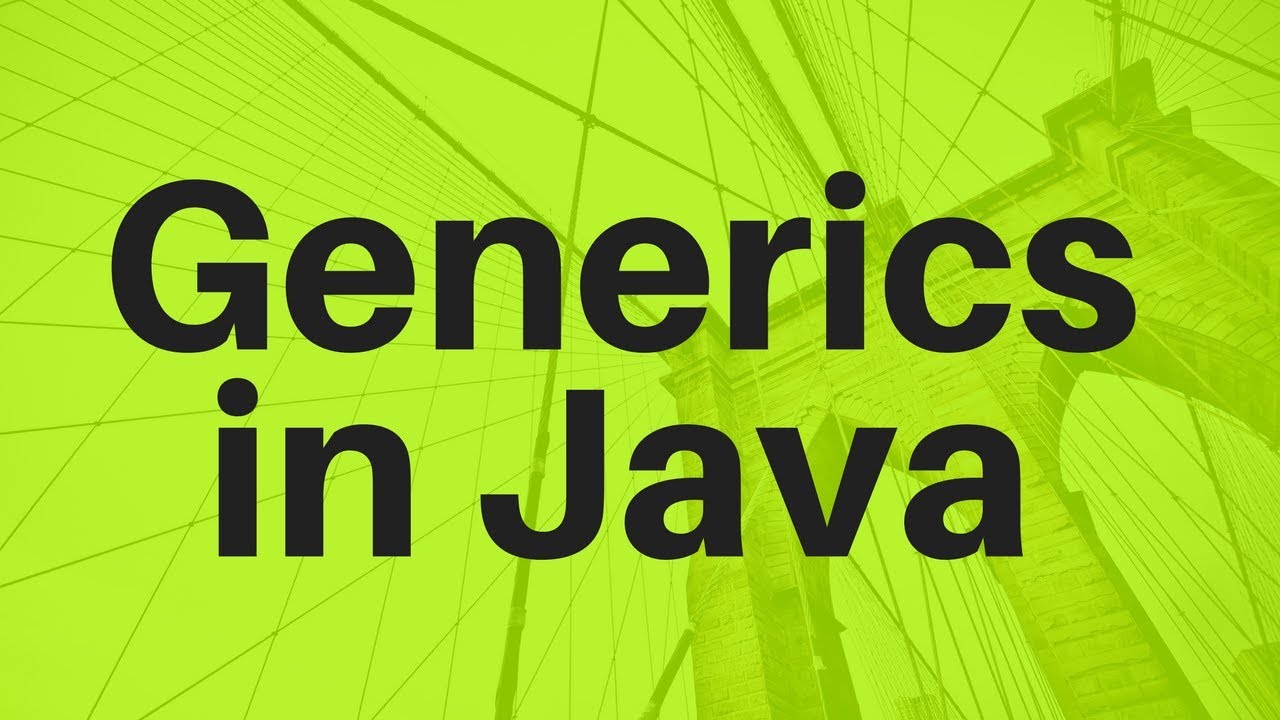
Generics in Java

How variables works in Python | Explained with Animations
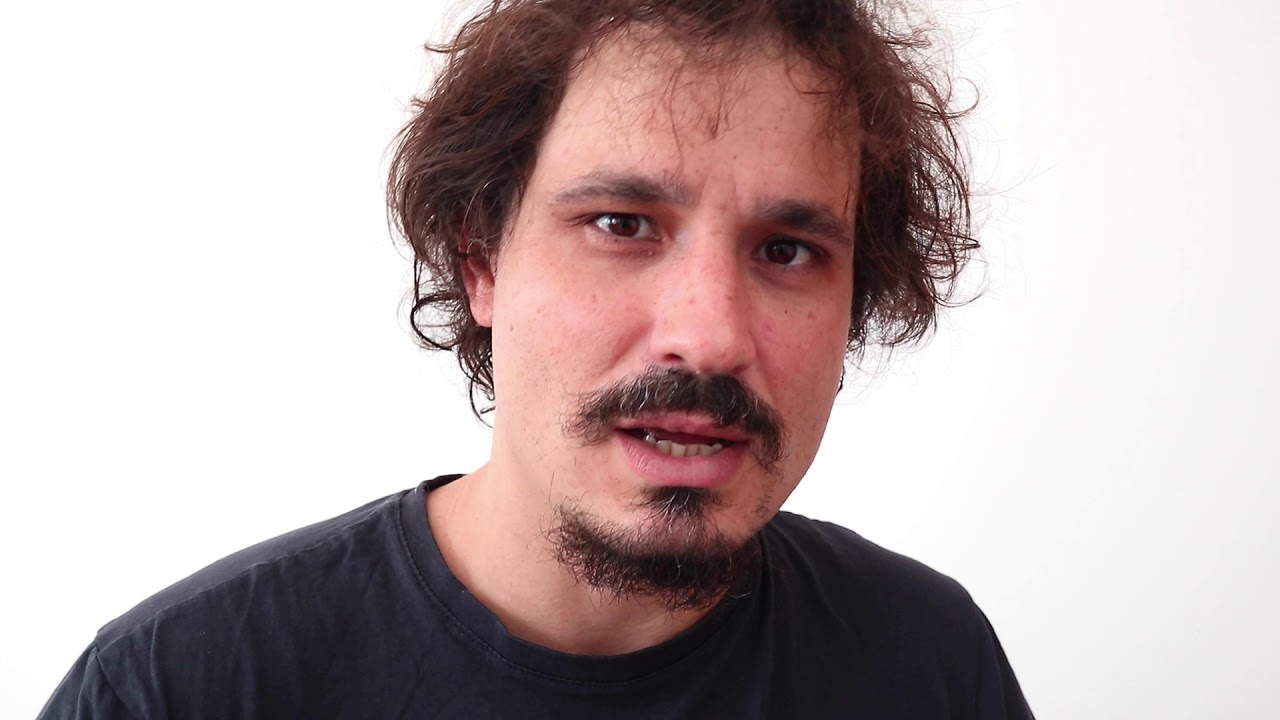
EDB1 IMD UFRN : Introdução a TAD Sequência ED Vetor
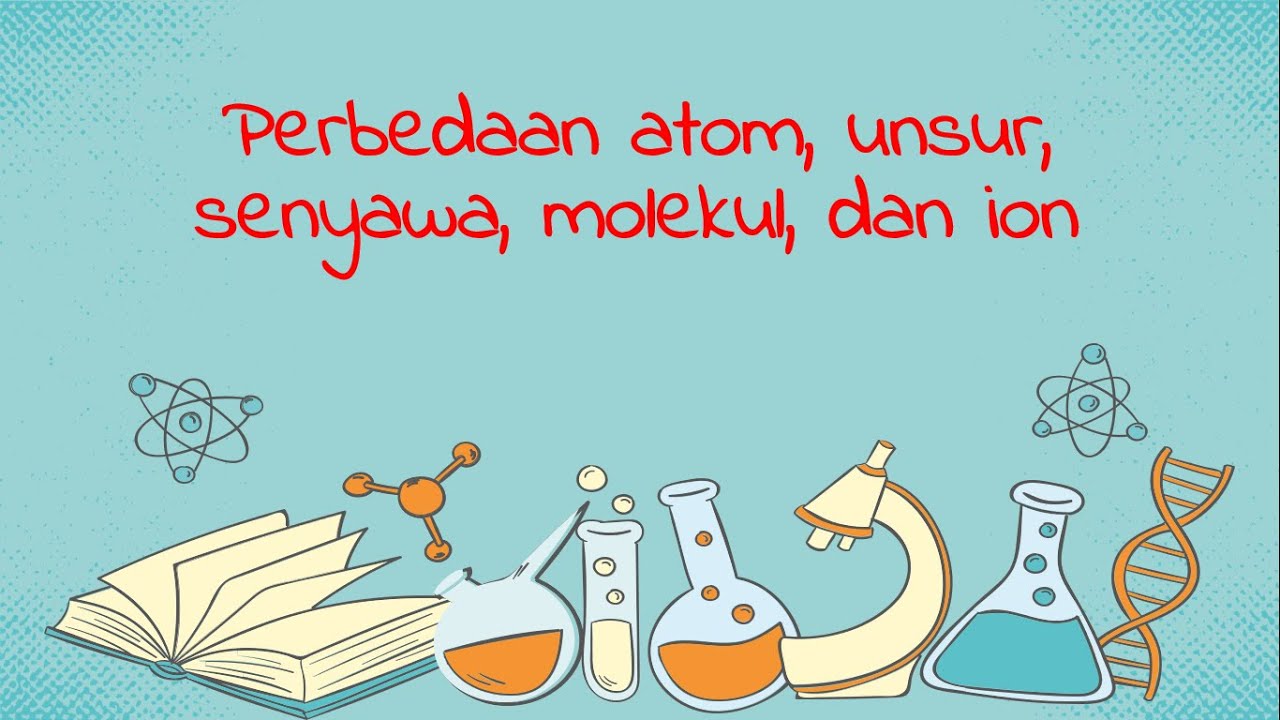
Perbedaan Atom, Unsur, Senyawa, Molekul dan Ion | KIMIA KELAS 10
5.0 / 5 (0 votes)