Software Engineering: Crash Course Computer Science #16
Summary
TLDRThis episode of CrashCourse Computer Science explores the discipline of Software Engineering, emphasizing the tools and practices that allow programmers to build large, complex programs like Microsoft Office. The video discusses object-oriented programming, APIs, Integrated Development Environments (IDEs), source control, and the importance of documentation and debugging in collaborative software development. It highlights how these techniques help manage the immense complexity of modern software, enabling teams to work together efficiently while maintaining code quality and stability.
Takeaways
- 😀 Sorting algorithms are often a small part of a much larger program, highlighting the need for efficient coding practices in software engineering.
- 🔍 The discipline of Software Engineering was coined by Margaret Hamilton, emphasizing the importance of preventative measures in software development.
- 🛠️ Breaking down large programs into smaller, manageable functions allows multiple programmers to work concurrently on different aspects of a project.
- 📚 Microsoft Office, as an example, contains approximately 40 million lines of code, illustrating the scale of modern software projects.
- 🧩 Packaging functions into hierarchies and related 'objects' is a method to manage complexity in large codebases, as seen in Object-Oriented Programming (OOP).
- 🔑 OOP uses public and private access modifiers to control the visibility and interaction between different parts of a program, encapsulating complexity.
- 🏗️ In large projects, teams may focus on specific subsystems, like cruise control in a car's software, relying on well-documented APIs for interaction with other parts of the system.
- 🛠️ Integrated Development Environments (IDEs) provide tools for writing, organizing, compiling, and debugging code, streamlining the development process.
- 🔍 Documentation is crucial for understanding and reusing code, with comments serving as in-code explanations and 'read-me' files offering external guidance.
- 🔄 Source Control systems, like version control, allow multiple developers to work on the same project without conflicts, tracking changes and enabling rollbacks if needed.
- 🔍 Quality Assurance (QA) testing is a rigorous process to identify and fix bugs before software is released, ensuring reliability and performance.
- 🌐 The script concludes with a teaser for the next episode, which will discuss how computers have become incredibly fast, hinting at the underlying hardware advancements.
Q & A
What is the main topic discussed in the CrashCourse Computer Science video?
-The main topic discussed in the video is the concept of Object Oriented Programming (OOP) and the practices of Software Engineering, particularly in the context of building large and complex software systems like Microsoft Office.
Who coined the term 'Software Engineering'?
-The term 'Software Engineering' was coined by engineer Margaret Hamilton, who worked on the Apollo missions for NASA.
How does breaking a program into smaller functions help in collaborative software development?
-Breaking a program into smaller functions allows many people to work simultaneously on different parts of the program without worrying about the whole system. This modular approach enables teams to focus on specific components or features, improving efficiency and manageability.
What is the purpose of packaging functions into hierarchies within objects?
-Packaging functions into hierarchies within objects helps in organizing related code together, making it easier to manage and navigate large codebases. It also promotes reusability and maintainability of the code.
Can you explain the concept of Object Oriented Programming using the example of a car's software?
-Object Oriented Programming involves creating objects that can contain other objects, functions, and variables. In the context of a car's software, you might have an 'Engine' object that contains 'children' objects like 'CruiseControl', 'IgnitionControl', etc., each with their own related functions and variables. This allows for a structured and organized approach to programming complex systems.
What is the role of an API in collaborative software development?
-An API (Application Programming Interface) defines how different parts of the code interact with each other. It allows programmers to access certain functions and data while restricting access to others, ensuring that only the right people can modify or use specific parts of the code.
Why is it important for functions to be marked as 'public' or 'private' in OOP?
-Marking functions as 'public' or 'private' is crucial for encapsulation in OOP. 'Private' functions can only be called within the object they belong to, while 'public' functions can be accessed by other objects. This helps in hiding the internal workings of an object and exposing only what is necessary for interaction.
What is an Integrated Development Environment (IDE) and what are its benefits?
-An Integrated Development Environment (IDE) is a software application that provides a comprehensive set of tools for writing, organizing, compiling, and testing code. Benefits of using an IDE include improved readability through features like color-coding, syntax error checking, efficient navigation of large codebases, and integrated debugging support.
How does documentation aid in software development and maintenance?
-Documentation, both in the form of standalone files and in-code comments, helps programmers understand the purpose and functionality of the code. It is crucial for revisiting old code, onboarding new developers, and promoting code reuse, thus reducing redundancy and improving efficiency.
What is Source Control and how does it facilitate collaborative coding projects?
-Source Control, also known as version control or revision control, is a system that tracks changes to the code over time and allows multiple developers to work on the same project without conflicts. It enables programmers to check out, modify, and commit code changes back to a central repository, while also providing a way to revert to previous versions if necessary.
What are the differences between alpha, beta, and release versions of software?
-Alpha versions are the earliest stage of software development, typically rough and buggy, and are only tested internally. Beta versions are more complete but still not fully tested; they may be released to the public to help identify issues. The final release version is the software that has undergone thorough testing and is ready for public use.
Outlines
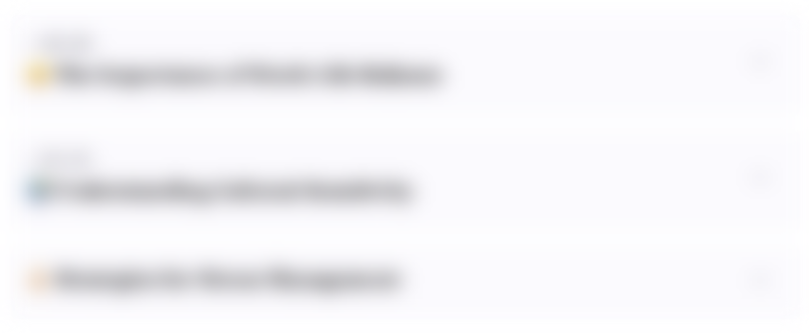
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنMindmap
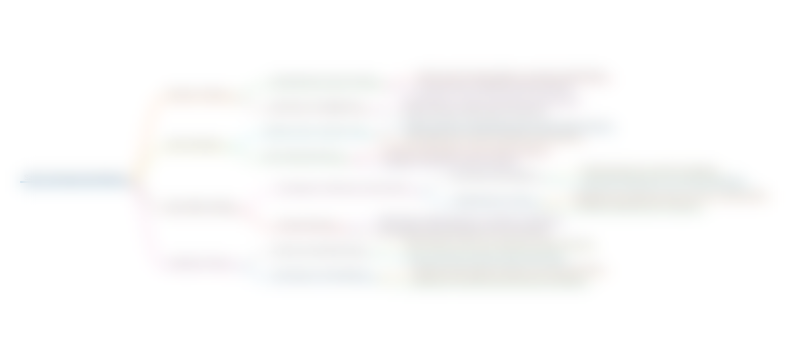
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنKeywords
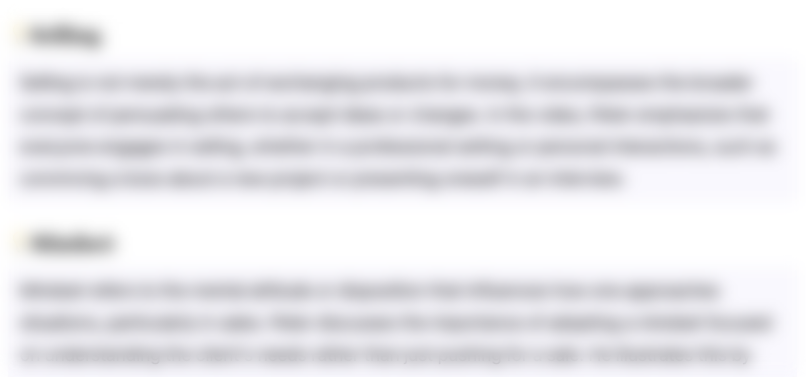
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنHighlights
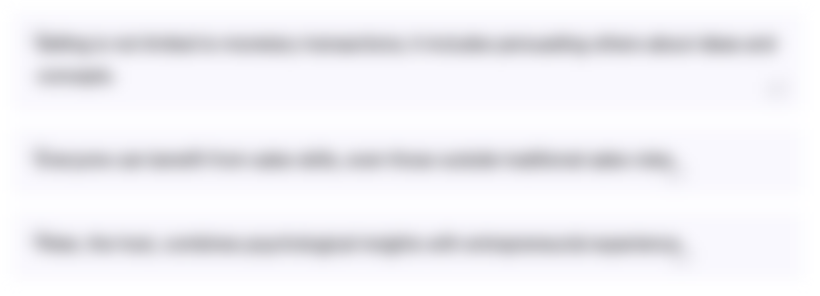
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنTranscripts
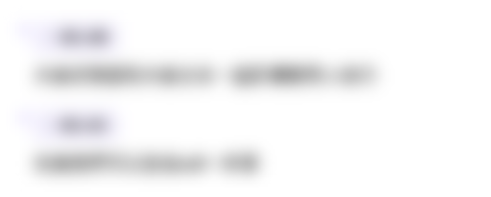
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنتصفح المزيد من مقاطع الفيديو ذات الصلة
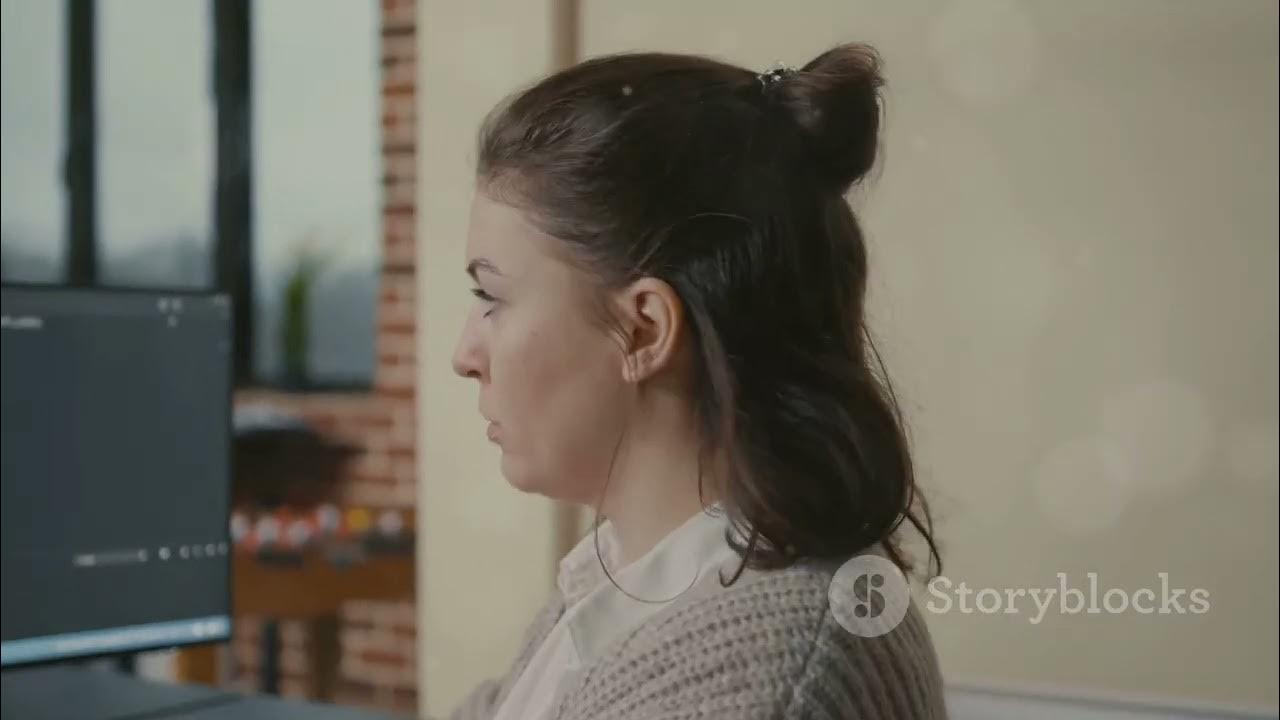
Mastering IDE's: Your Ultimate Guide to Software Development
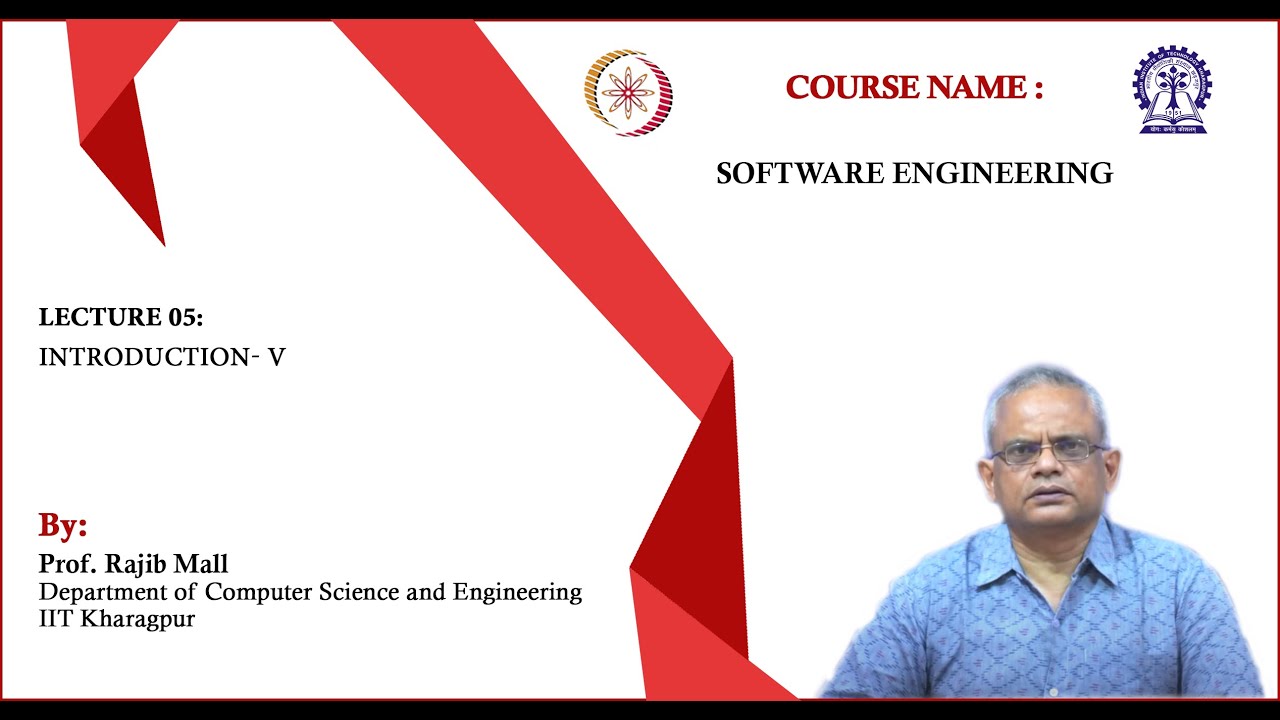
Lecture 05: Introduction- V
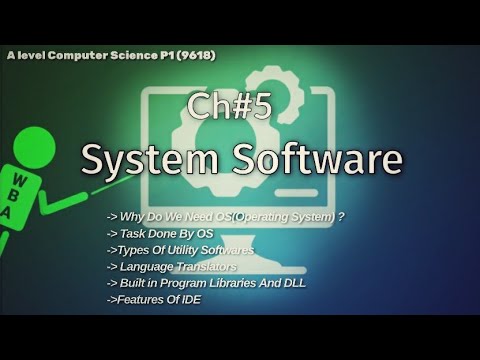
A level Computer Science (9618)P1||Ch#5 System Software||@wbaatz
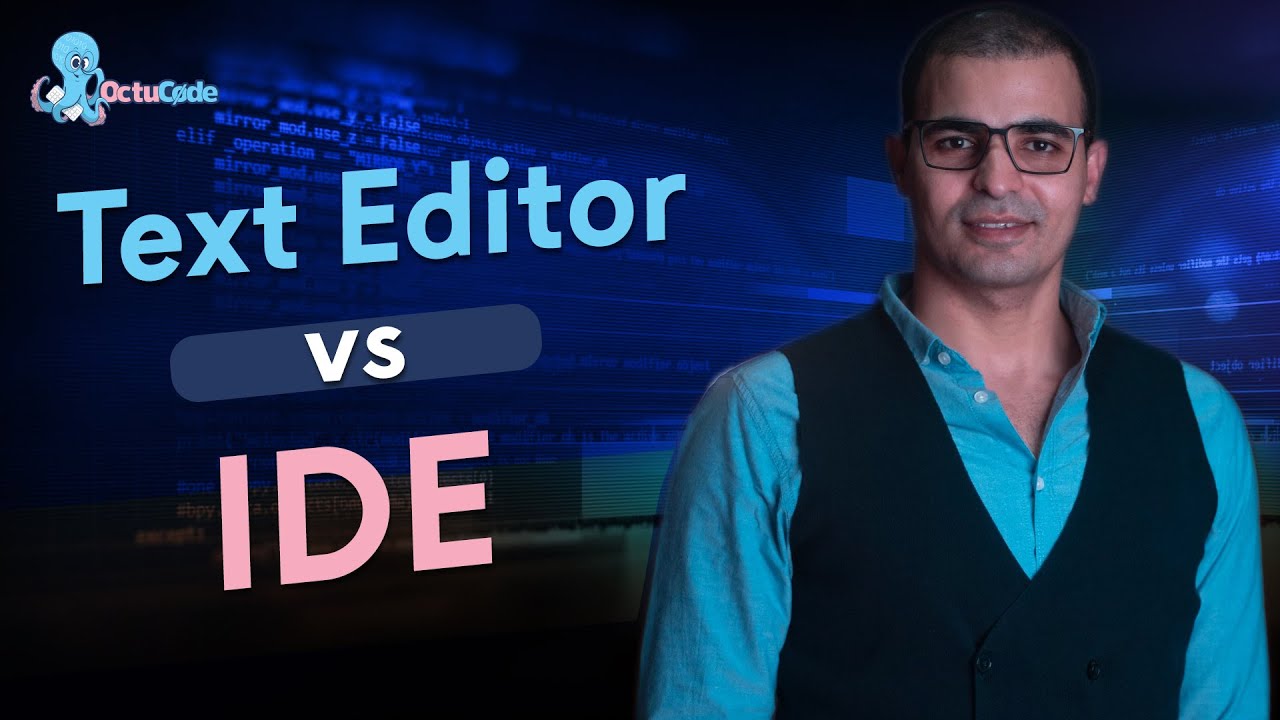
The difference between IDE and text editors : learn programming
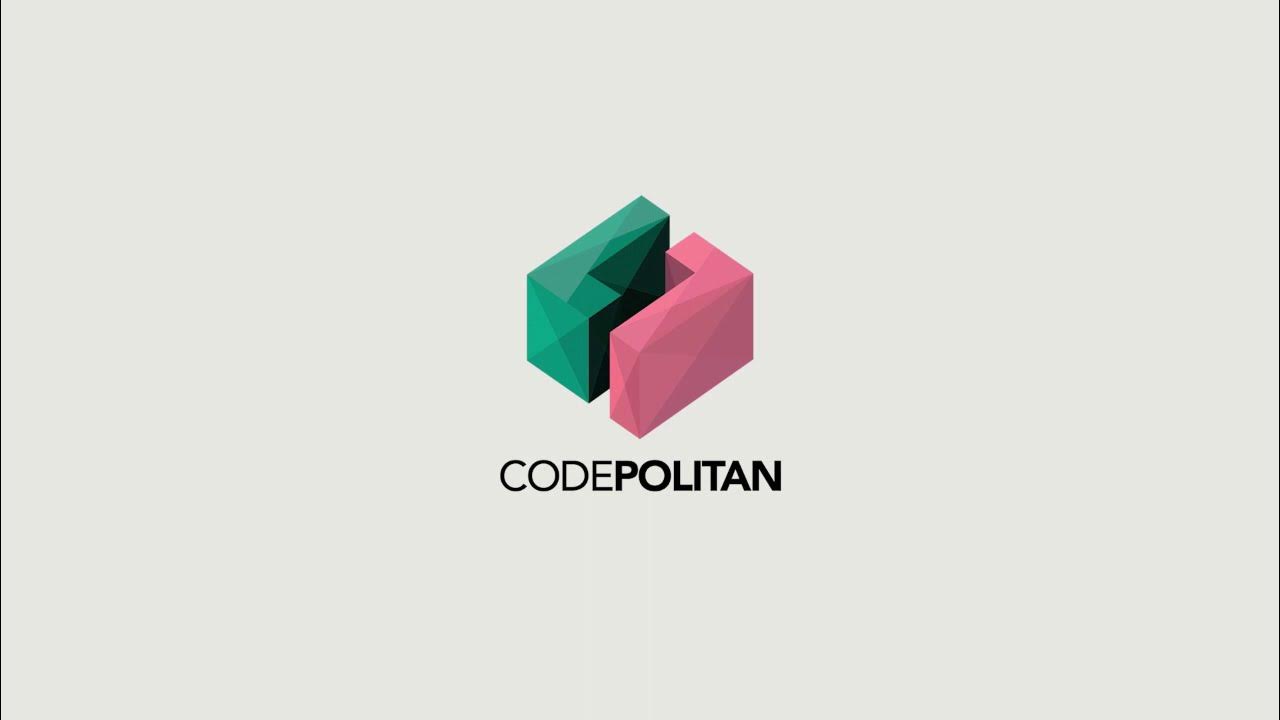
01 Apa itu IDE dan Text Editor

Will AI replace programmers? | Cursor Team and Lex Fridman
5.0 / 5 (0 votes)