Recursive Backtracking - DSA Course in Python Lecture 14
Summary
TLDRIn this video, Greg explains recursive backtracking, a powerful algorithmic technique used for exhaustive searches. He illustrates the concept using the subsets problem, where the goal is to find all possible subsets of a given array. By making decisions to include or exclude elements, the algorithm explores various paths and backtracks as necessary. The process is structured through recursive calls, leading to a time complexity of O(2^N) and a space complexity of O(N). Greg emphasizes the importance of this technique in solving combinatorial problems, making it an essential topic in computer science.
Takeaways
- 😀 Recursive backtracking is a method that combines recursion with backtracking to solve problems by making decisions and exploring their outcomes.
- 😀 The primary use of backtracking is for exhaustive searches, similar to brute force techniques, particularly when generating all possible solutions.
- 😀 The subsets problem is a key example of recursive backtracking, where the goal is to find all subsets of a given set of unique integers.
- 😀 For an array with 'n' unique elements, there are 2^n possible subsets, including the empty set.
- 😀 In the subsets problem, decisions are made at each step to either include or exclude each element from the current subset.
- 😀 The backtracking process involves undoing previous decisions, allowing the algorithm to explore alternative paths in the decision tree.
- 😀 A depth-first search (DFS) approach is commonly used in recursive backtracking to navigate through the possible solutions.
- 😀 Two global lists are typically utilized: one for storing all solutions and another for keeping track of the current subset being constructed.
- 😀 The time complexity for the recursive backtracking solution to the subsets problem is O(2^n), while the space complexity is O(n) due to the recursion depth.
- 😀 The implementation of backtracking requires careful management of the state of the current subset to ensure accurate results are generated.
Q & A
What is recursive backtracking?
-Recursive backtracking is a technique that uses recursion to explore all possible solutions to a problem by making decisions, then undoing those decisions to allow for alternative paths.
How does backtracking relate to brute force techniques?
-Backtracking is often considered a brute force technique because it explores all potential solutions to find every possibility, especially when the problem requires generating all solutions.
Can you explain the subsets problem in the context of backtracking?
-The subsets problem involves generating all possible subsets of a given array of unique integers. For an array of size n, the total number of subsets is 2^n, including the empty set and the set itself.
What are the two main branches when making a decision in the backtracking process?
-The two main branches are: 1) choosing to include the current element in the subset, and 2) choosing not to include the current element.
What is the significance of the base case in recursive backtracking?
-The base case occurs when the index goes out of bounds, at which point a copy of the current subset is added to the results list. It signifies that a complete solution has been reached.
Why is it important to pop elements from the current subset during backtracking?
-Popping elements is crucial to undoing the last decision made, ensuring that when exploring other branches, the current subset reflects only the decisions made up to that point.
What are the global lists used in the implementation of the backtracking algorithm?
-The two global lists are: 'res', which stores all the subsets generated, and 'sol', which serves as a temporary storage for the current subset being constructed.
How does the time complexity of recursive backtracking for generating subsets manifest?
-The time complexity is O(2^n) because each element leads to two branches, resulting in an exponential growth of the decision tree as more elements are added.
What is the space complexity of the recursive backtracking approach?
-The space complexity is O(n) due to the depth of the recursive call stack, which corresponds to the maximum number of elements in the array being processed.
How does the example of the array [1, 2, 3] illustrate the subsets problem?
-For the array [1, 2, 3], all possible subsets include combinations of choosing or not choosing each element, leading to a total of eight subsets: [], [1], [2], [3], [1, 2], [1, 3], [2, 3], and [1, 2, 3].
Outlines
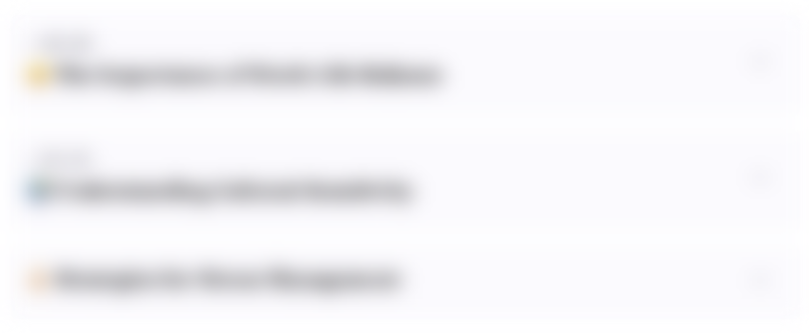
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنMindmap
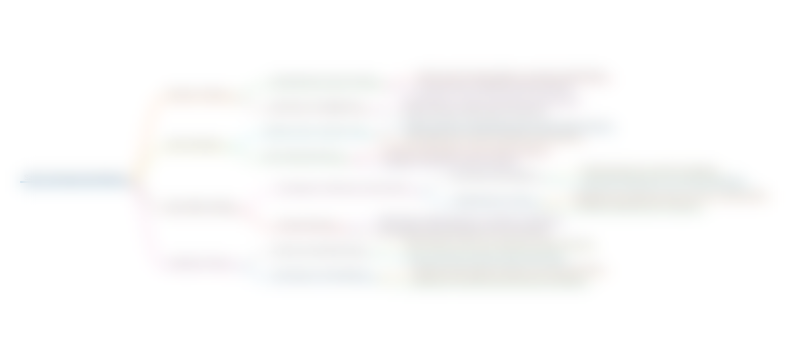
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنKeywords
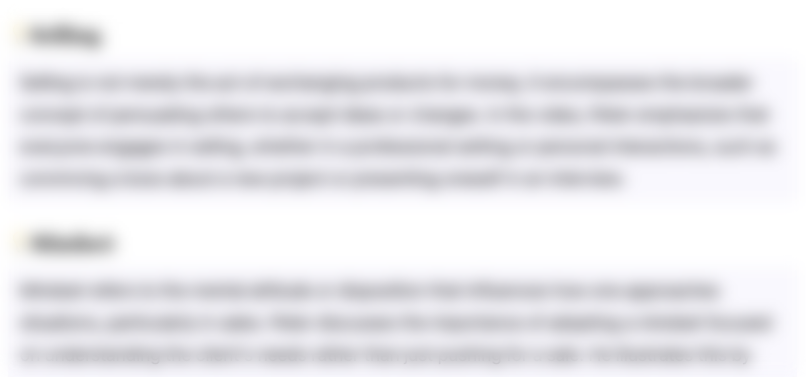
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنHighlights
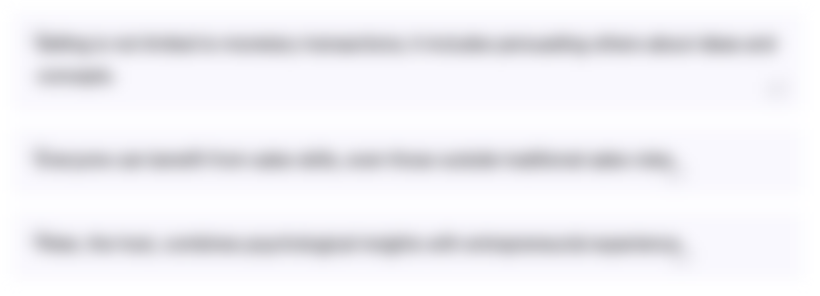
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنTranscripts
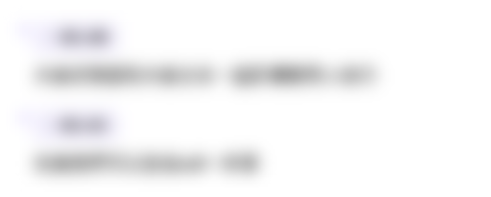
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنتصفح المزيد من مقاطع الفيديو ذات الصلة
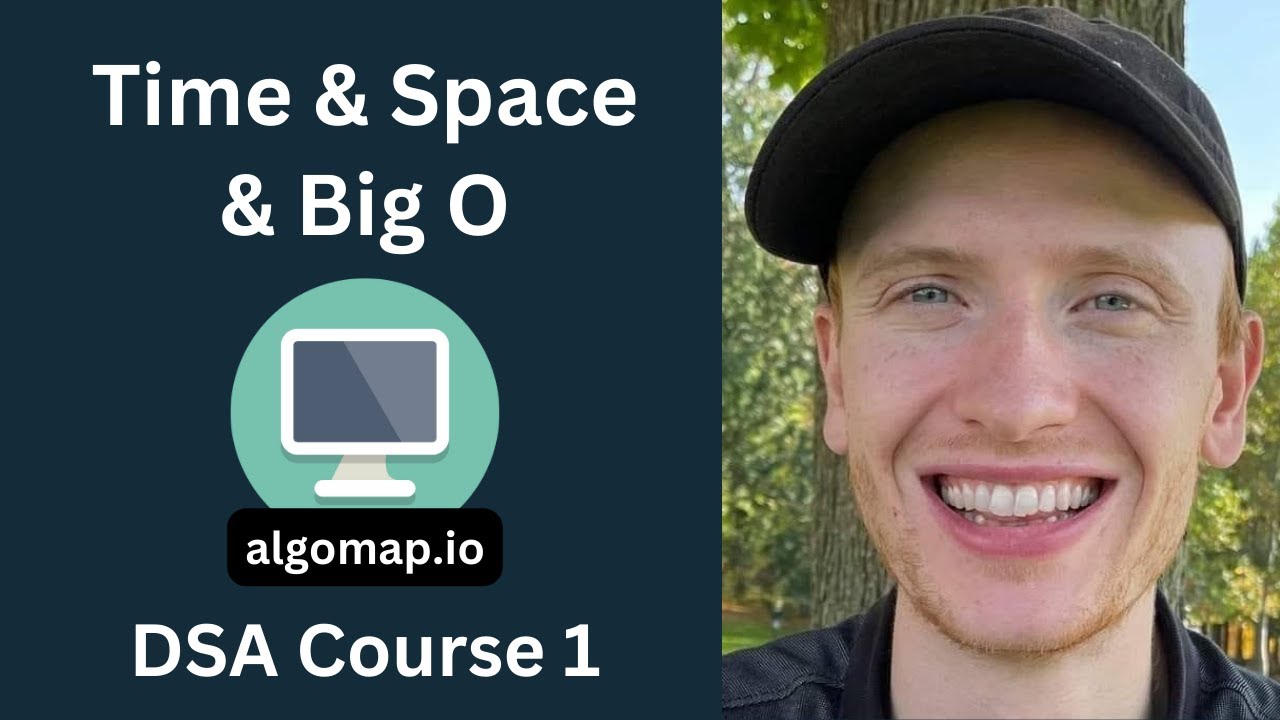
Time & Space Complexity - Big O Notation - DSA Course in Python Lecture 1

Lec-10: Two 2️⃣ Pointer👆 Technique | Two 2️⃣ Sum Problem in Data Structure

1.4 Frequency Count Method
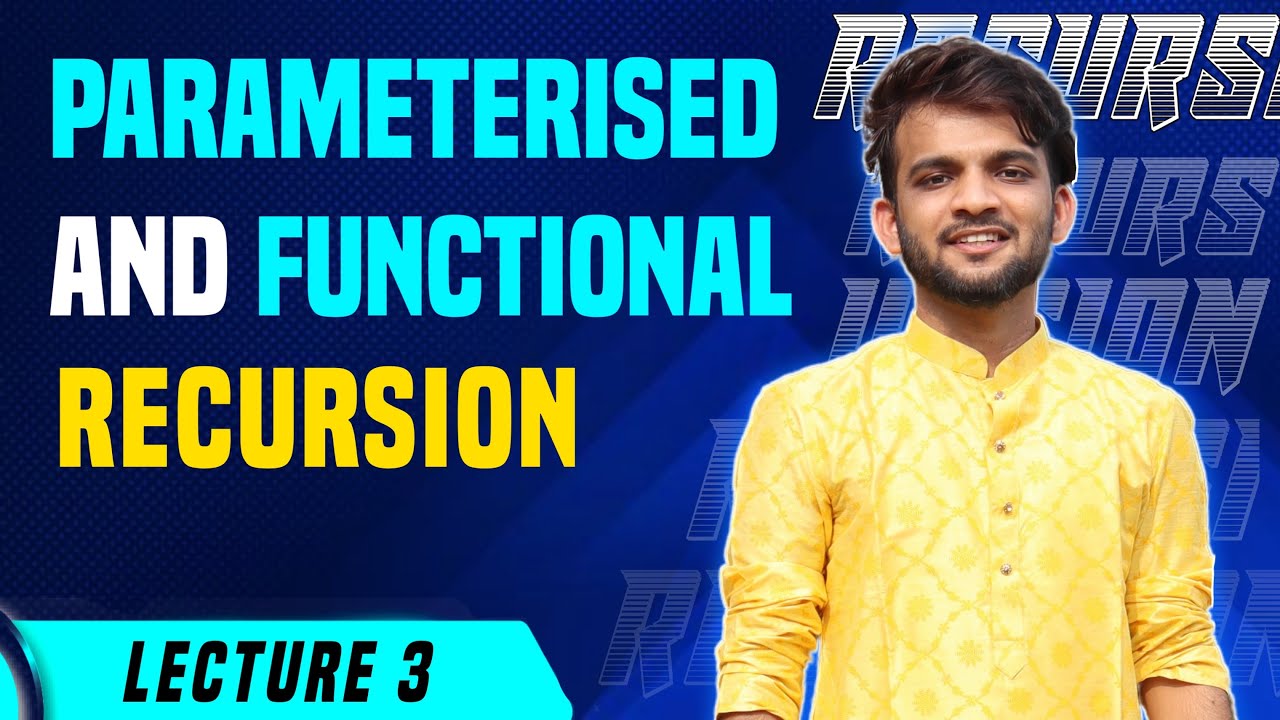
Re 3. Parameterised and Functional Recursion | Strivers A2Z DSA Course

Balanced Binary Tree - Leetcode 110 - Python
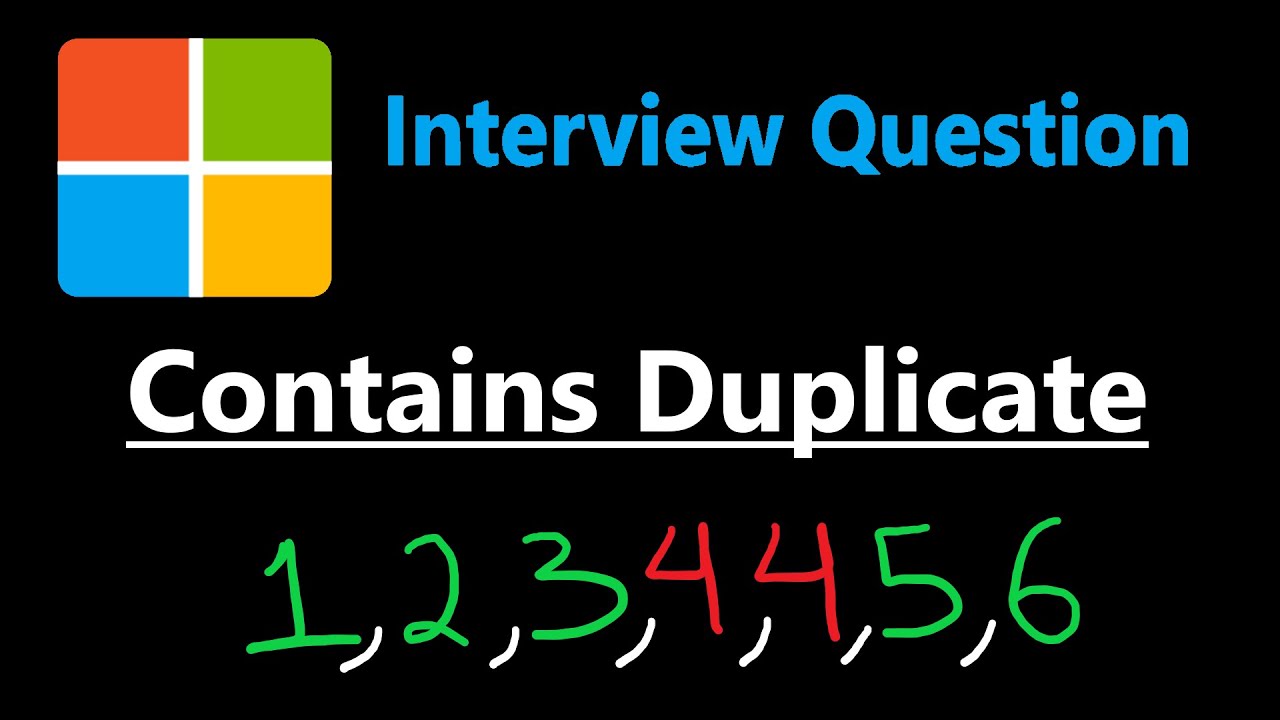
Contains Duplicate - Leetcode 217 - Python
5.0 / 5 (0 votes)