Template Method Pattern
Summary
TLDRThis video delves into the Template Method design pattern, a crucial behavioral pattern in software development. It establishes a framework for algorithms while allowing subclasses to implement specific steps. Through the example of a `RobotTemplate`, viewers learn how different types of robots—like an automotive robot and a baking robot—can share a common algorithm while differing in details. The pattern's flexibility and structure empower developers to create diverse implementations without altering the overarching process, making it a valuable tool for efficient software design.
Takeaways
- 😀 The Template Method design pattern is a behavioral pattern that establishes a framework for an algorithm, allowing subclasses to redefine specific steps.
- 😀 It provides a global structure for executing an algorithm while enabling flexibility in its implementation.
- 😀 The Template Method is useful when creating various objects with differing behaviors but similar overall processes.
- 😀 An abstract class typically defines the template method, where common behaviors are outlined, and specific implementations are deferred to subclasses.
- 😀 Subclasses can implement the details of certain steps, providing varied behaviors while maintaining the overall algorithm structure.
- 😀 A practical example includes an abstract class like 'InstantMessage,' which specifies how to send messages, with subclasses defining how to handle different message types.
- 😀 The pattern encourages code reuse and helps maintain a clear separation of concerns, especially in complex algorithms.
- 😀 The class diagram consists of an abstract class defining the template method and concrete classes implementing specific behaviors.
- 😀 Example implementations include 'RobotTemplate' for different types of robots, where subclasses execute unique tasks within a defined algorithm.
- 😀 The Template Method pattern enhances the clarity and manageability of complex systems by encapsulating varying behaviors within a consistent framework.
Q & A
What is the Template Method design pattern?
-The Template Method design pattern is a behavioral pattern that defines the skeleton of an algorithm in a base class, allowing subclasses to redefine specific steps of the algorithm without changing its overall structure.
What type of design patterns does the Template Method belong to?
-The Template Method belongs to the behavioral design patterns category.
How does the Template Method pattern benefit object creation?
-It allows for the creation of different types of objects that share a common process framework, making it easier to implement variations without altering the global algorithm.
Can you explain how subclasses interact with the Template Method?
-Subclasses can override specific steps defined in the Template Method, providing their own implementations while adhering to the overall algorithm structure established by the base class.
What role does the abstract class play in the Template Method pattern?
-The abstract class contains the template method, which defines the sequence of steps for the algorithm, and specifies any required methods that subclasses need to implement.
What is an example of using the Template Method pattern in messaging applications?
-In messaging applications, an abstract class like `InstantMessage` can define the steps to send messages, while subclasses implement the specifics for different message types, such as text, video, or images.
What are the main steps typically defined in a Template Method?
-The main steps usually include an initial setup, the core process, any variations based on subclass implementations, and a finalization step.
How does the Template Method improve code maintenance?
-It centralizes the algorithm definition in one place, making it easier to update or modify the overall structure without having to change every individual subclass.
What are some advantages of using the Template Method pattern?
-Advantages include code reuse, simplified maintenance, enhanced flexibility for subclass behavior, and a clear separation of common and variable parts of the algorithm.
What specific examples of robots were discussed in relation to the Template Method pattern?
-The examples included `AutomotiveRobot`, which follows a specific process for car manufacturing, and `CookieRobot`, which follows a different process for baking cookies, both using the same algorithm framework defined by `RobotTemplate`.
Outlines
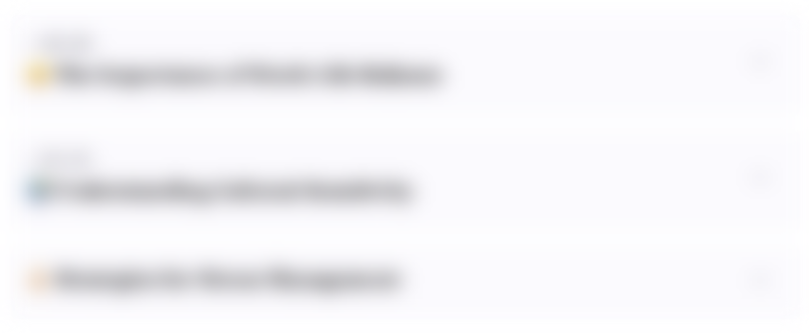
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنMindmap
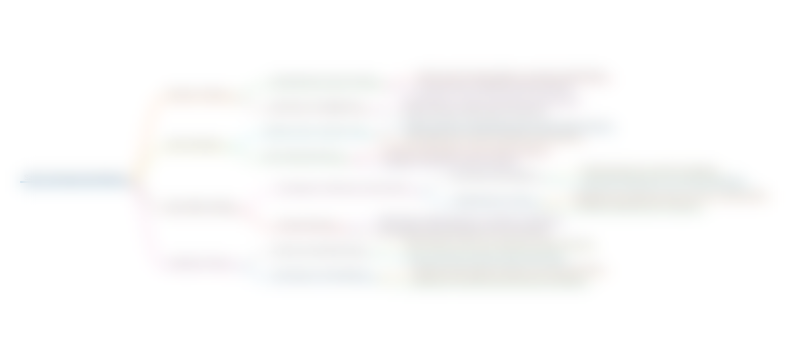
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنKeywords
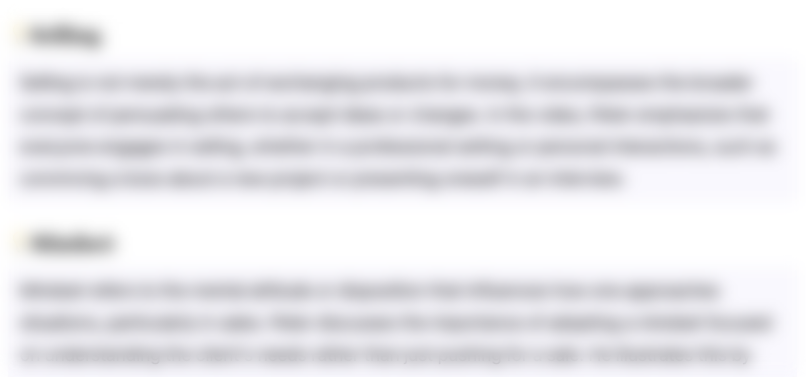
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنHighlights
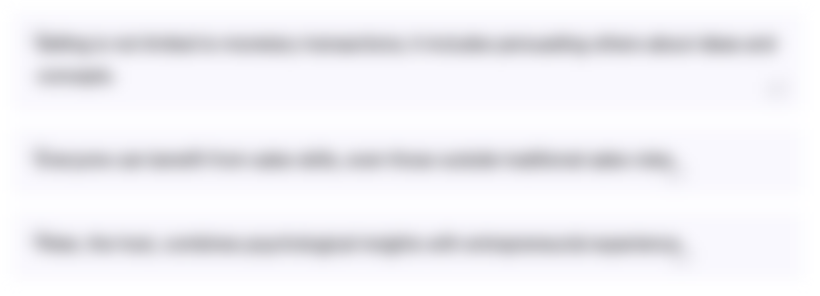
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنTranscripts
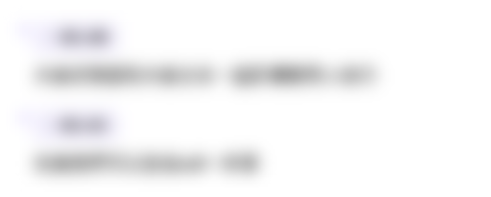
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنتصفح المزيد من مقاطع الفيديو ذات الصلة

The Chain of Responsibility Pattern Explained & Implemented | Behavioral Design Patterns | Geekific
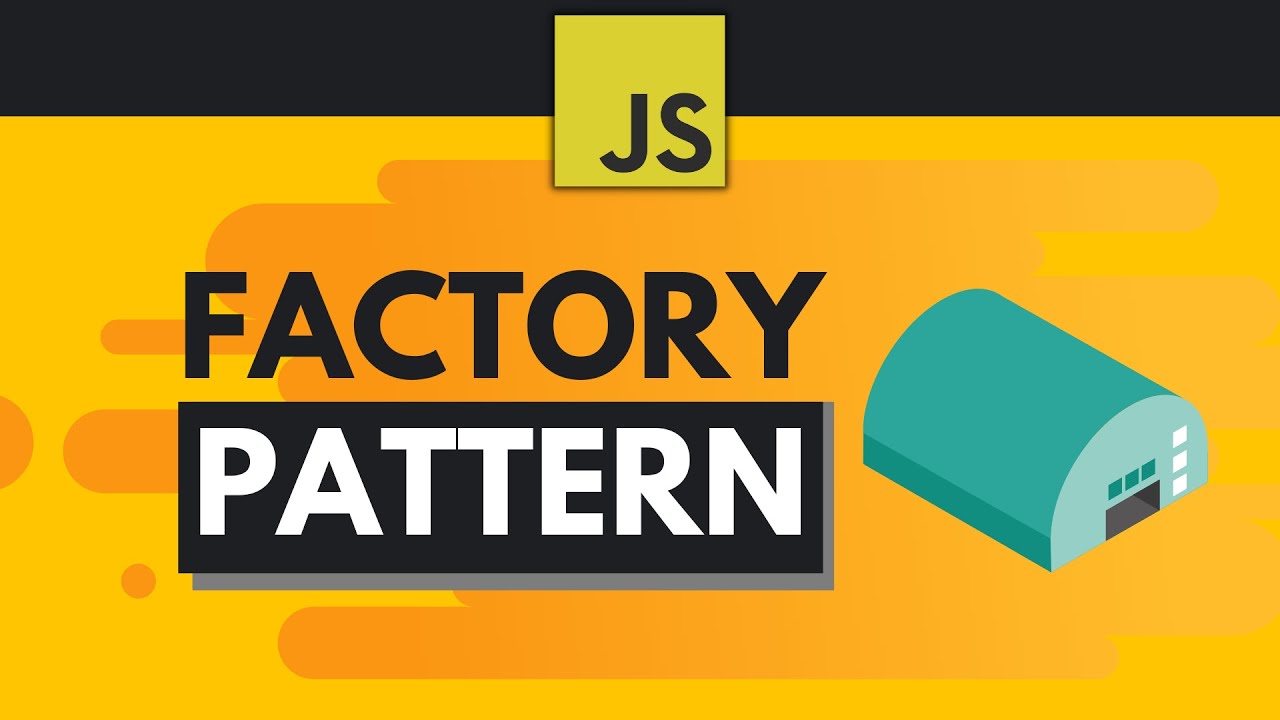
Javascript Design Patterns #1 - Factory Pattern
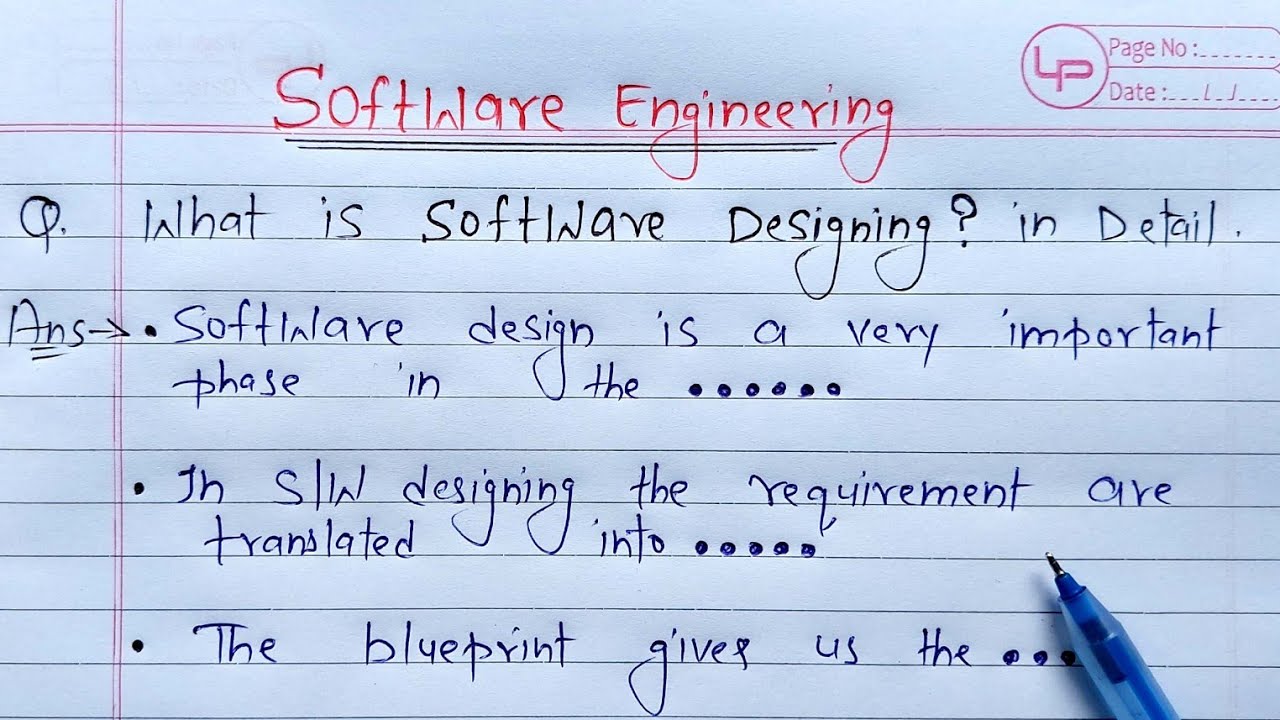
what is software designing? full Explanation | Learn Coding

Strategy Design Pattern Tutorial with Java Coding Example | Strategy Pattern Explained

DOMINE O EVENT STORMING!

The Singleton Pattern Explained and Implemented in Java | Creational Design Patterns | Geekific
5.0 / 5 (0 votes)