Depth First Search (DFS) Graph Traversal in Data Structures
Summary
TLDRThis video introduces Depth First Search (DFS), a method for graph traversal. It contrasts DFS with Breadth First Search (BFS), explaining how DFS explores nodes deeply before backtracking. The speaker outlines the procedure, starting from a node and using a stack to keep track of visited nodes. As the traversal progresses, the video illustrates how to add connected vertices to the stack and visit them sequentially. The speaker emphasizes the simplicity of DFS while hinting at a forthcoming detailed coding tutorial in C. Overall, the video provides a clear and engaging explanation of DFS.
Takeaways
- 😀 Depth First Search (DFS) is a method for traversing graphs, just like Breadth First Search (BFS).
- 🌐 In DFS, you start at a node and explore its connected vertices, going as deep as possible before backtracking.
- 🔄 If you reach a dead end while traversing, you backtrack to the last visited node to explore other paths.
- 📊 DFS and BFS are two major graph traversal techniques, each with different approaches.
- 📚 The procedure for DFS involves using a stack to keep track of the nodes to visit.
- ✅ When implementing DFS, nodes are marked as visited to avoid revisiting them.
- 🗂️ The stack in DFS is crucial, as it follows the Last In, First Out (LIFO) principle.
- 💻 The programming implementation of DFS can be done in languages like C, utilizing function call stacks.
- 🔍 The traversal order in DFS can vary based on the order nodes are pushed onto the stack.
- 📝 Pseudocode for DFS is provided to assist with coding the algorithm in the next video.
Q & A
What is Depth First Search (DFS) and how is it different from Breadth First Search (BFS)?
-Depth First Search (DFS) is a graph traversal method where you start at a node and explore as deeply as possible along each branch before backtracking. In contrast, Breadth First Search (BFS) explores all neighboring nodes at the current level before moving on to nodes at the next level.
How does DFS explore a graph?
-In DFS, the exploration starts from a node, and the algorithm moves to its connected vertices, going deeper into the graph. When a dead end is reached, it backtracks and explores other suspended paths. This continues until all nodes are visited.
What is the role of a stack in DFS?
-A stack is used in DFS to manage the nodes to be visited. It follows the Last In, First Out (LIFO) principle, where the last node added to the stack is the first one to be visited. This structure helps DFS explore deeper nodes before backtracking.
Can DFS be implemented without a stack?
-While DFS can be conceptually described without a stack, in computer programming, a stack is essential to manage the traversal process efficiently. The stack ensures that DFS explores nodes correctly and backtracks when necessary.
What happens when DFS encounters a dead end?
-When DFS encounters a dead end, meaning a node has no unvisited neighbors, it backtracks by popping the last node from the stack and continues exploring from there.
How does DFS work programmatically?
-Programmatically, DFS begins by pushing the starting node onto a stack. Then, nodes are popped from the stack, visited, and any unvisited connected nodes are pushed onto the stack. This process continues until all nodes are visited.
Why does the speaker mention that DFS is simple in layman terms?
-The speaker mentions that DFS is simple in layman terms to emphasize the straightforward nature of the algorithm. It involves going deep into a graph and backtracking when necessary, which is easy to grasp without technical details.
What is the key difference between how DFS is described informally and how it is implemented in programming?
-Informally, DFS is described as a process of exploring a graph deeply before backtracking. In programming, DFS is implemented using a stack to track nodes to be visited and manage backtracking efficiently.
What does the pseudocode provided in the video illustrate?
-The pseudocode provided in the video illustrates the step-by-step procedure for implementing DFS in a programming language, showing how nodes are added to the stack, popped from it, and visited until all nodes are explored.
How does DFS compare to BFS in terms of traversal order?
-DFS explores a graph by going deep along one branch before backtracking, whereas BFS explores nodes level by level, visiting all neighboring nodes before moving deeper. DFS can lead to a different traversal order compared to BFS, depending on the graph structure and node connections.
Outlines
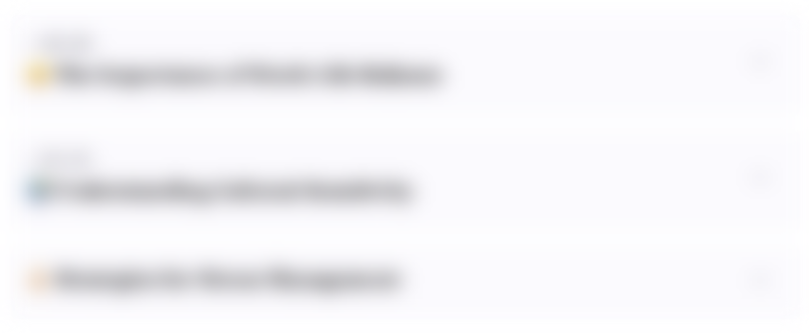
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنMindmap
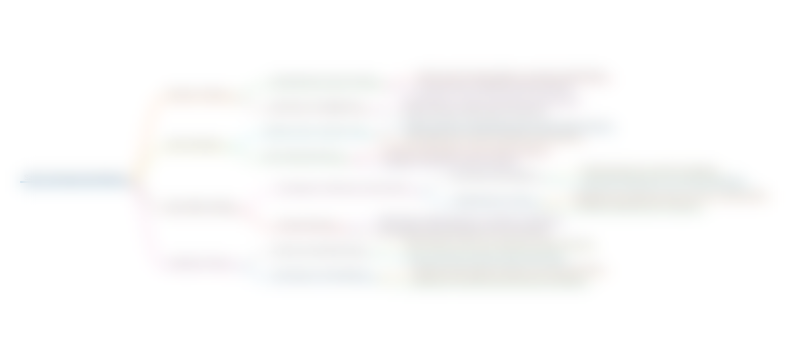
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنKeywords
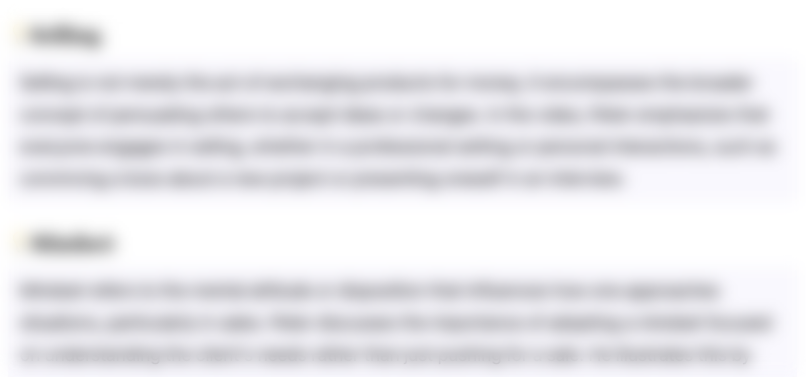
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنHighlights
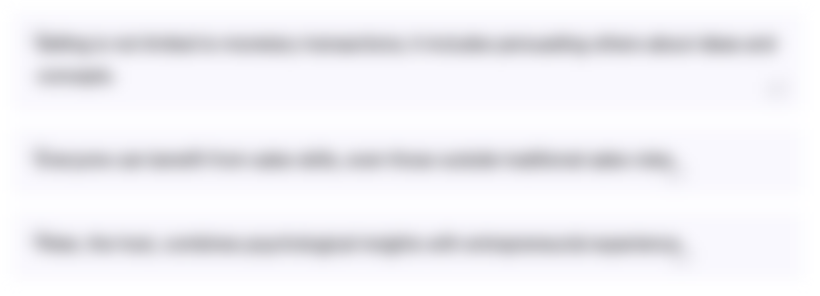
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنTranscripts
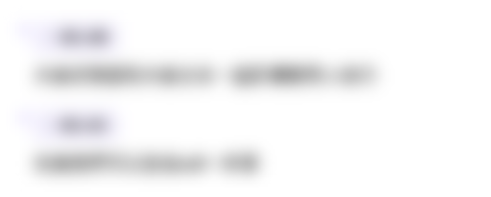
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنتصفح المزيد من مقاطع الفيديو ذات الصلة
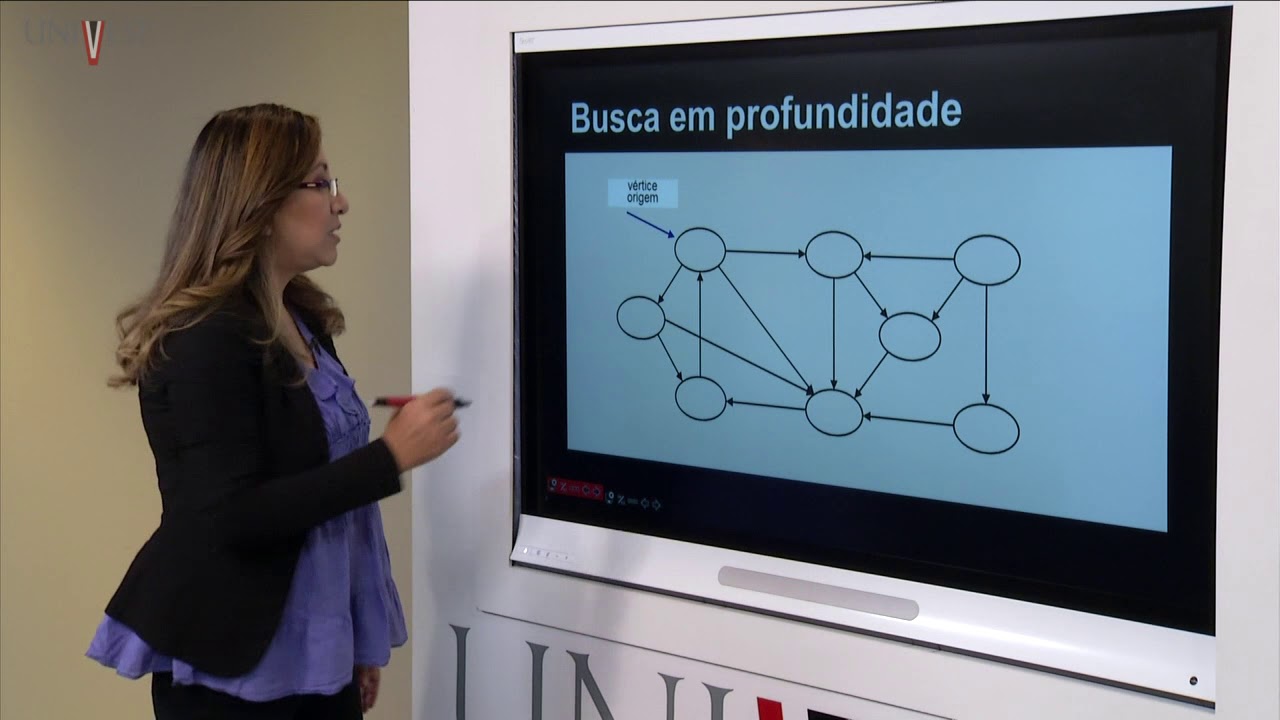
Projeto e Análise de Algoritmos - Aula 12 - Algoritmos de busca em largura e profundidade em grafos

G-19. Detect cycle in a directed graph using DFS | Java | C++
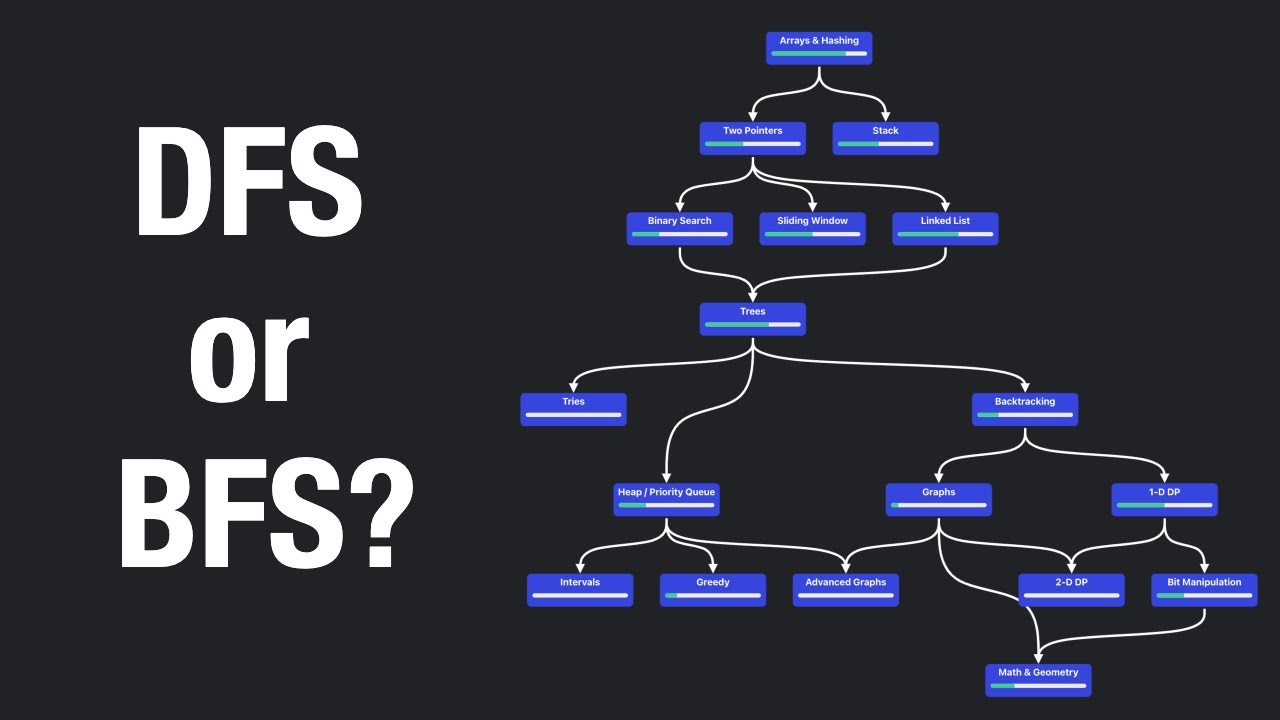
The Best Order to learn Algorithms & Data Structures?

1 Backtracking Course Overview
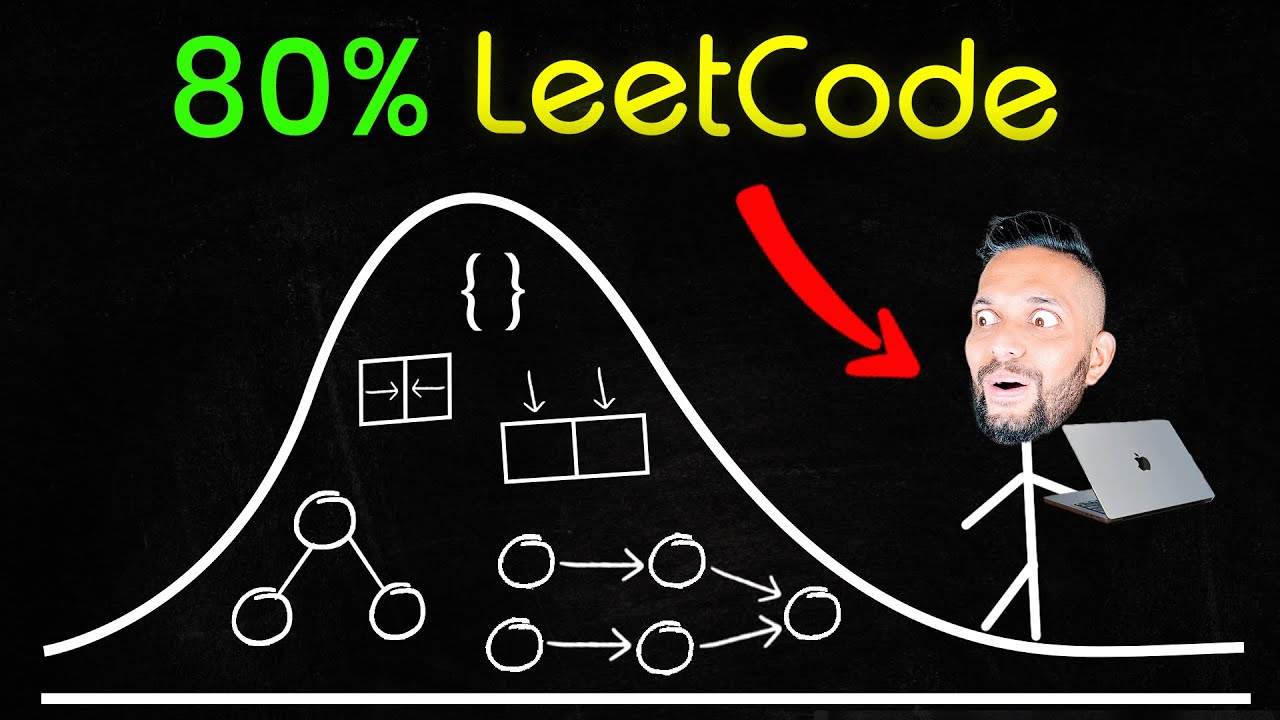
8 patterns to solve 80% Leetcode problems
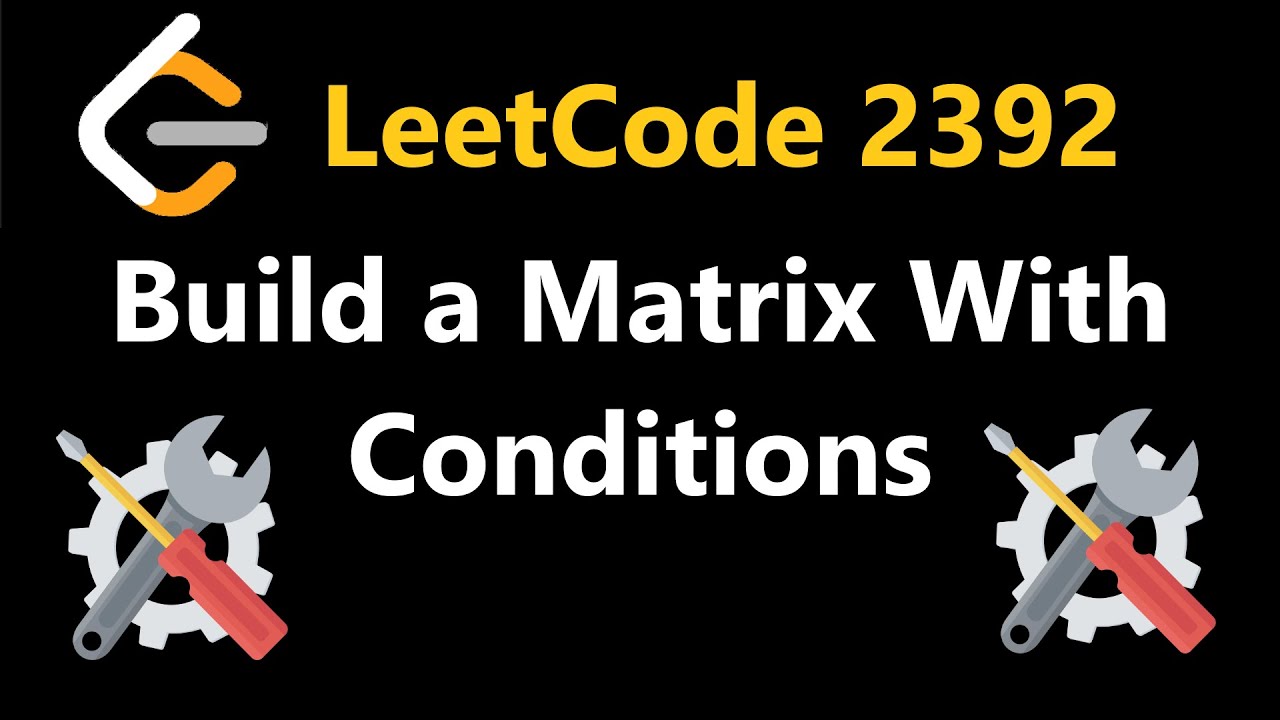
Build a Matrix With Conditions - Leetcode 2392 - Python
5.0 / 5 (0 votes)