#08 View Template of Component | Angular Components & Directives| A Complete Angular Course
Summary
TLDRThe lecture explains how to create and use a component in Angular, focusing on rendering its view template. It discusses two methods: the 'template' property, which embeds HTML as a string in the TypeScript file, and the 'templateUrl' property, which references an external HTML file. While the 'template' method can handle small amounts of HTML, it becomes cumbersome and error-prone with larger code. The 'templateUrl' method is preferred for separating HTML from TypeScript, making the code more maintainable. The lecture concludes by demonstrating how to implement these techniques.
Takeaways
- 📦 Angular components can be used by referring to their selectors, which are treated like HTML elements.
- 📄 The HTML rendered by a component is known as its view template.
- 📛 The template property in the component is used to define the HTML when there are only a few lines of code.
- 💻 Using template URL allows developers to specify the path to an external HTML file, making the code more manageable.
- 🗂️ It's recommended to use template URL when there are many lines of HTML to avoid cluttering the TypeScript file.
- 🔧 The template property can lead to maintainability issues since it mixes TypeScript and HTML code in one file.
- 🚫 Errors in HTML within the template property are harder to detect since they only appear at runtime, not during compilation.
- 📐 A large amount of HTML code in the template property can make the code messy and difficult to manage.
- 📏 Separating HTML into a template URL promotes better code organization and maintainability, even for small HTML snippets.
- 🖌️ The next step after defining the view template is to style it, which will be covered in the following lecture.
Q & A
What is the view template of a component in Angular?
-The view template of a component is the HTML that tells Angular how to render the component. It defines the structure of the component's HTML content.
How do you use a component's selector in Angular?
-To use a component's selector, you add the selector like an HTML element in another component's HTML file. Angular then replaces that selector with the component's view template.
What is the role of the 'template' property in a component?
-The 'template' property allows you to specify the view template of a component directly as an inline HTML string within the component’s TypeScript file.
Why is the 'template' property not suitable for large HTML content?
-Using the 'template' property for large HTML content can make the code messy and harder to maintain because the HTML is written as a string within the TypeScript file.
What is the 'templateUrl' property, and when should you use it?
-The 'templateUrl' property specifies the path to an external HTML file that contains the view template for the component. It should be used when the HTML content is large or when you want to separate the HTML from the TypeScript code for better maintainability.
What are the disadvantages of using the 'template' property?
-Disadvantages of using the 'template' property include mixing HTML with TypeScript, lack of compile-time error detection for HTML mistakes, and the potential for messy code if the HTML is large.
Why is it beneficial to use 'templateUrl' even for small HTML templates?
-Using 'templateUrl' is beneficial even for small templates because it keeps the HTML code separate from the TypeScript code, making it easier to maintain and less prone to errors.
What happens when Angular compiles the component with a selector and a view template?
-When Angular compiles the component, it replaces the selector with the HTML content specified in the view template, either defined via the 'template' or 'templateUrl' property.
What issue arises with using 'template' when an HTML tag is not closed properly?
-If an HTML tag is not closed properly while using the 'template' property, the error won't be detected at compile-time. Instead, it will only be noticed at runtime, making debugging harder.
What should be done if the HTML view template is styled improperly?
-If the view template is styled improperly, CSS or other styling methods can be applied to the component, which will be covered in a subsequent lecture.
Outlines
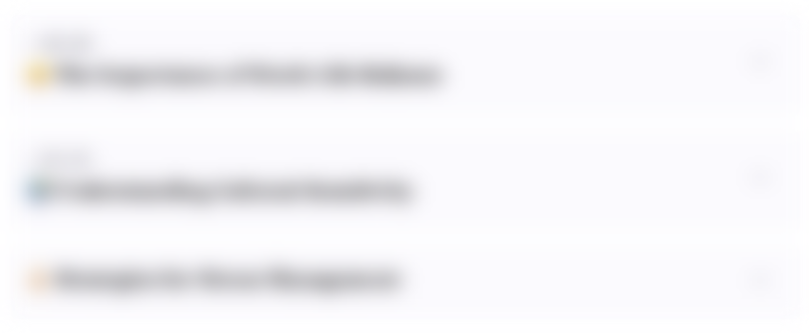
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنMindmap
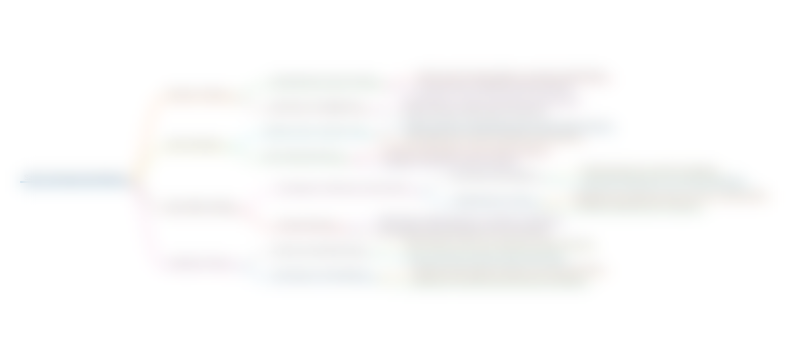
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنKeywords
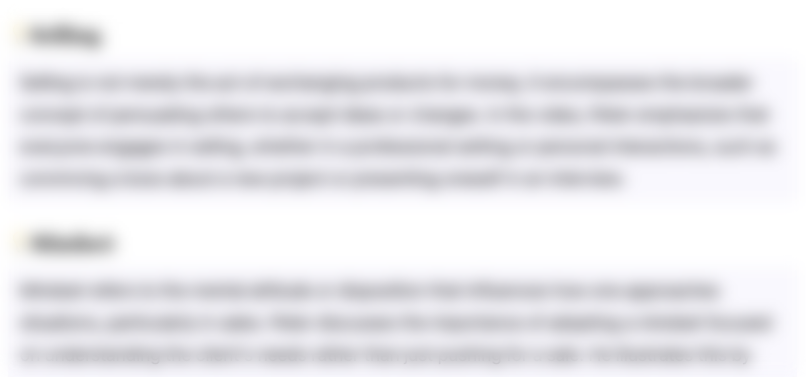
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنHighlights
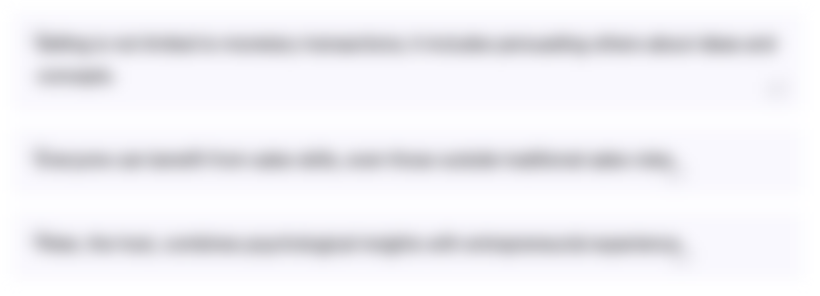
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنTranscripts
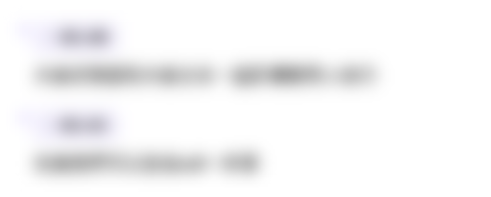
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنتصفح المزيد من مقاطع الفيديو ذات الصلة
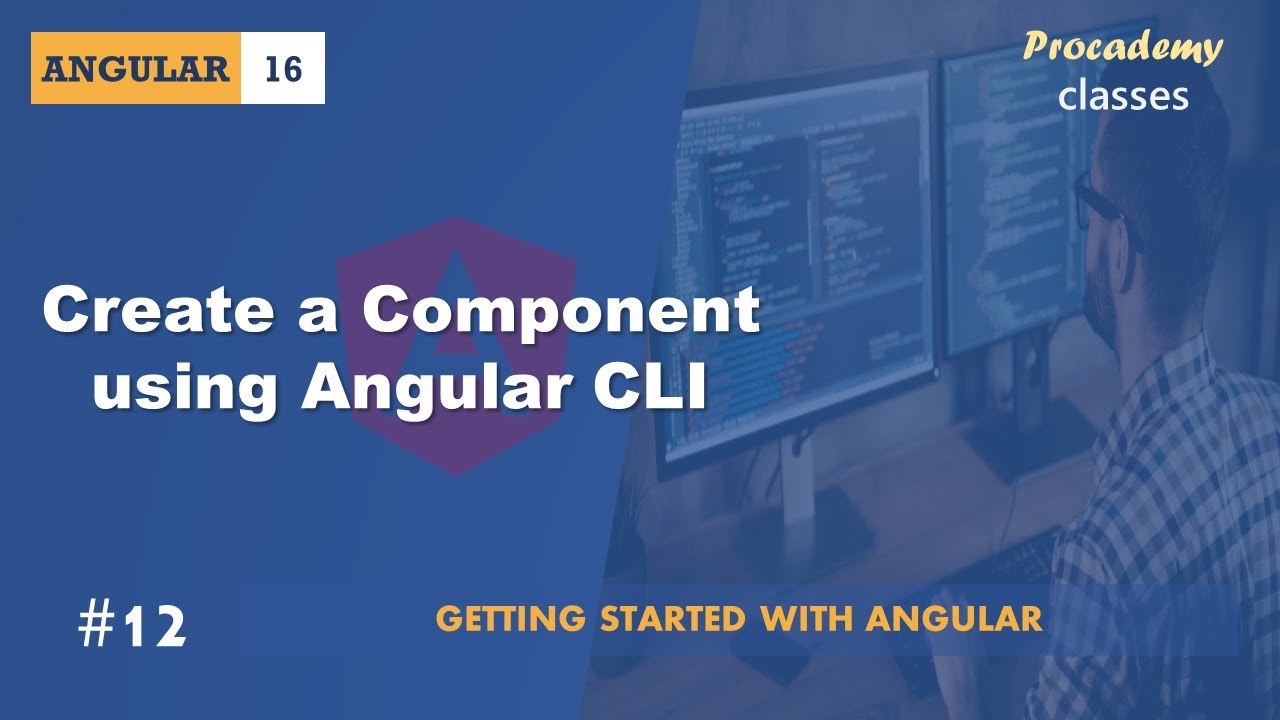
#12 Create Component using Angular CLI | Angular Components & Directives | A Complete Angular Course

#17 Event Binding | Angular Components & Directives | A Complete Angular Course

#18 Two way Data Binding | Angular Components & Directives | A Complete Angular Course
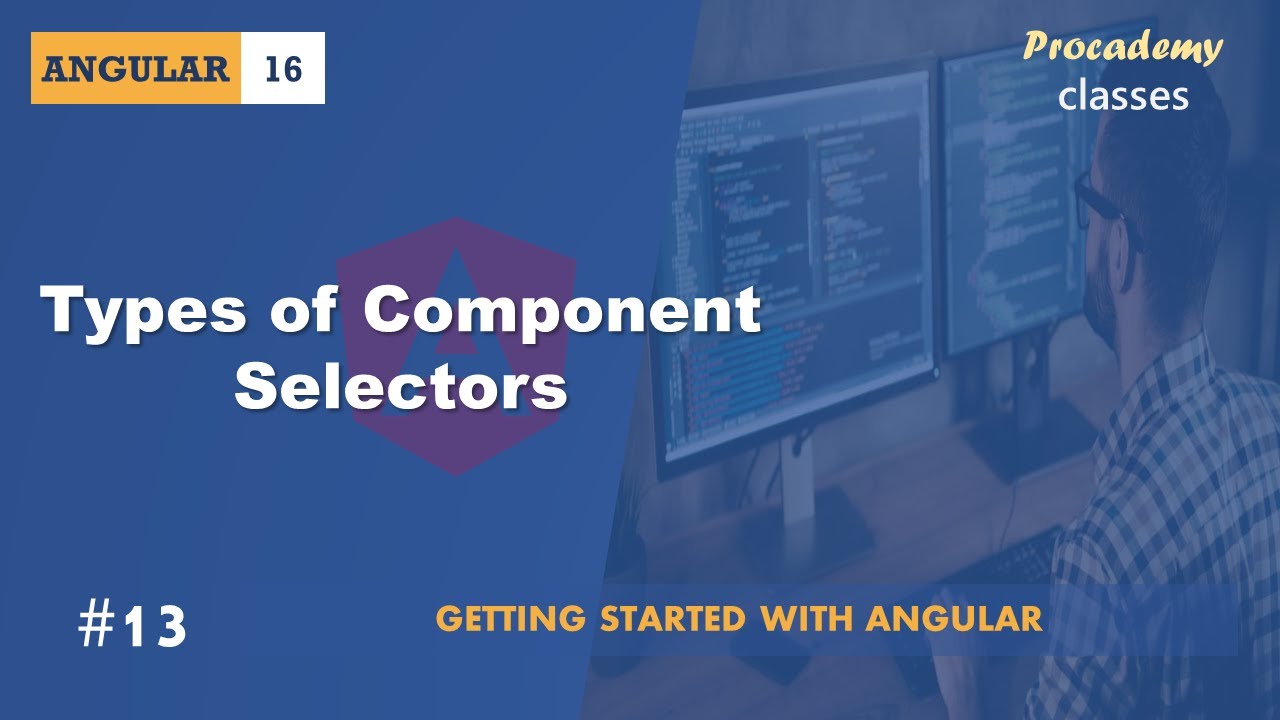
#13 Types of Component Selector | Angular Components & Directives | A Complete Angular Course
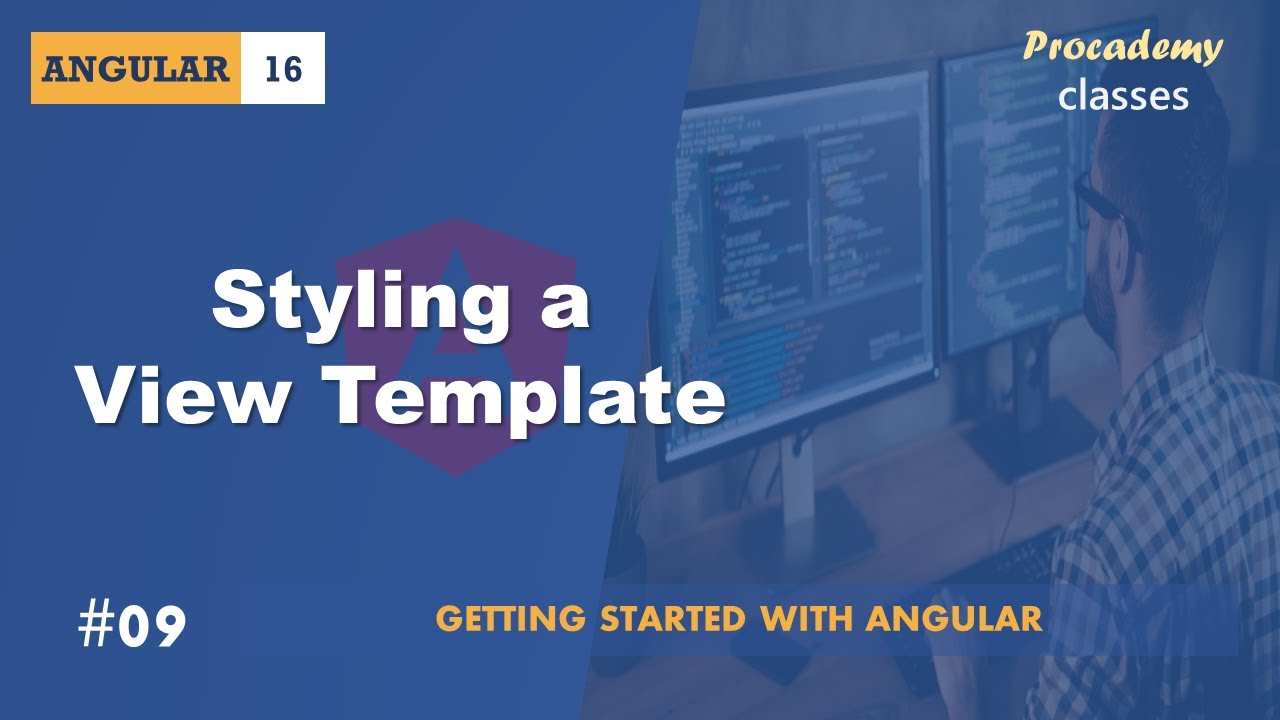
#09 Styling View Template | Angular Components & Directives| A Complete Angular Course
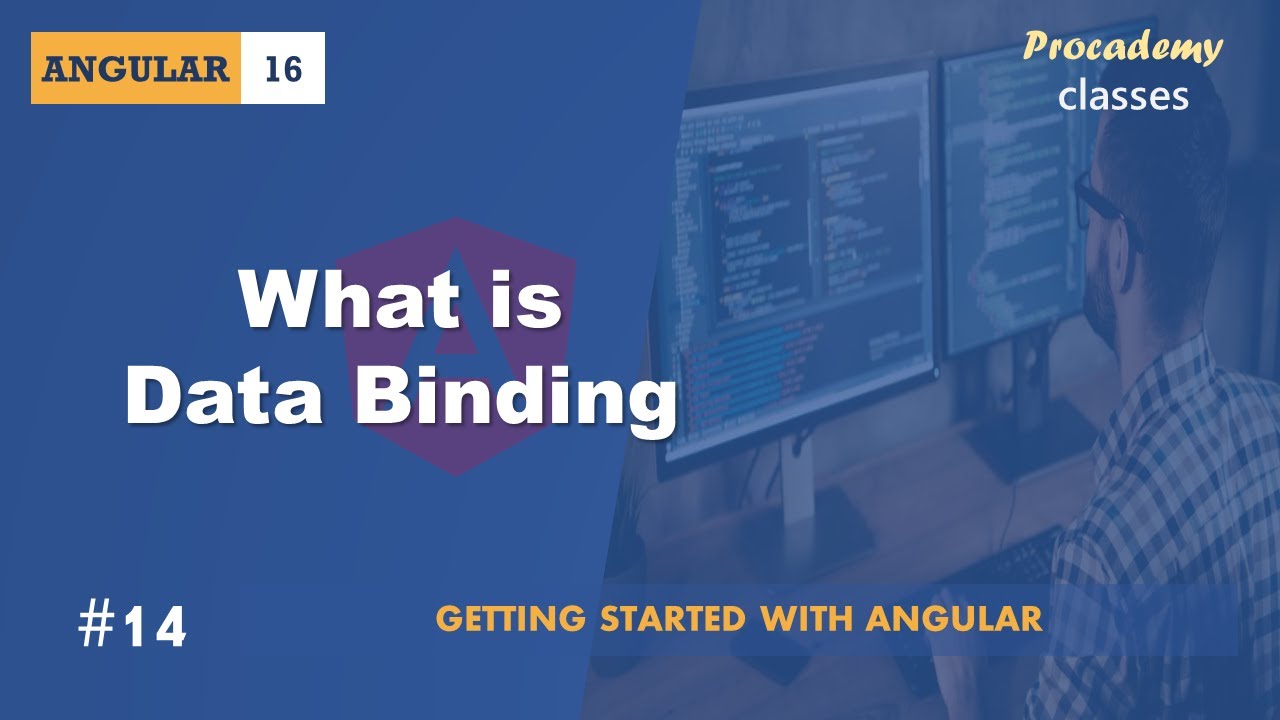
#14 What is Data Binding | Angular Components & Directives | A Complete Angular Course
5.0 / 5 (0 votes)