Java Collections Framework-Part2 | Interfaces And Classes
Summary
TLDRThis video script offers an in-depth exploration of Java's Collection Framework, emphasizing the foundational Collection interface and its derived interfaces: List, Set, and Queue. It distinguishes between them based on their handling of insertion order and duplicates. The script also introduces the distinct Map interface, which stores data as key-value pairs with unique keys. It briefly mentions utility classes like Collections and outlines various collection classes such as ArrayList, LinkedList, HashSet, and HashMap, setting the stage for实操 demonstrations in upcoming videos.
Takeaways
- 📚 The Java Collection Framework is a set of interfaces and classes that allow for the manipulation and storage of groups of objects.
- 🔑 The root interface in the Collection Framework is the Collection interface, which provides a set of common methods for all collection objects.
- 🧩 The Collection interface is extended by several child interfaces including List, Set, and Queue, each with specific characteristics for different use cases.
- 📝 List interface maintains the order of elements and allows duplicates, suitable for scenarios where insertion order is important.
- 🚫 Set interface does not maintain the order of elements and does not allow duplicates, ideal for unique element storage without order concerns.
- 📬 Queue interface follows the First-In-First-Out (FIFO) principle, used for processing elements in the order they were added.
- 🔄 The Collections class in Java provides utility methods to manipulate any collection, such as sorting, searching, and shuffling.
- 🔗 The Map interface is distinct from the Collection interface and is used for storing data in key-value pairs, with keys being unique.
- 📦 Classes like ArrayList, LinkedList, HashSet, and PriorityQueue implement various collection interfaces, providing different functionalities and performance characteristics.
- 🔍 The choice of collection interface and implementing class depends on the specific requirements of data storage and manipulation, such as order preservation, uniqueness, and access patterns.
Q & A
What is the primary role of the Collection interface in Java's Collection Framework?
-The Collection interface serves as the root interface for all collection-related classes and interfaces in Java. It provides a set of common methods that can be used across various collection objects, such as adding, removing, and checking for the presence of elements within the collection.
How does the Collections class in Java differ from the Collection interface?
-The Collections class is a utility class within the java.util package that contains static methods to operate on or return various collection types, such as sorting a list. It is not an interface but a class that provides additional functionality to manipulate collections, whereas the Collection interface defines the contract for collection types.
What is the significance of the List interface in the Java Collection Framework?
-The List interface is a child of the Collection interface and is used when the order of elements is important. It allows duplicate elements and maintains the insertion order, meaning elements are preserved in the order they were added.
Which Java classes implement the List interface and why would you use them?
-Classes that implement the List interface include ArrayList, LinkedList, Vector, and Stack. You would use these classes when you need to maintain the insertion order of elements and allow duplicates, with each class offering different performance characteristics and capabilities.
What is the Set interface and under what conditions should it be used?
-The Set interface is also a child of the Collection interface and is used when you want to store unique elements without maintaining any order. It does not allow duplicates, and the insertion order is not preserved.
Can you name the classes that implement the Set interface in Java?
-The classes that implement the Set interface in Java are HashSet and LinkedHashSet. These classes are used when you need to ensure that all elements are unique and the order of elements is not a concern.
How does the Queue interface differ from the List and Set interfaces?
-The Queue interface is different from List and Set interfaces as it represents a collection designed for holding elements prior to processing, following a first-in, first-out (FIFO) order. It is typically used for managing tasks in the order they were received.
What is the primary use case for the PriorityQueue class in Java?
-The PriorityQueue class is used when you need to manage a collection of objects where each object has a priority associated with it. It ensures that the highest priority object is always at the front of the queue and is served first.
What is the Map interface and how does it differ from the Collection interface?
-The Map interface is a separate and independent interface in the Java Collection Framework. It stores elements in key-value pairs, where each key is unique but values may not be. It is not a child of the Collection interface and is used when you need to associate values with unique keys.
Which classes in Java implement the Map interface and what are their主要用途?
-The classes that implement the Map interface in Java are HashMap and LinkedHashMap. HashMap is used for fast access to values based on keys, while LinkedHashMap maintains the insertion order of the keys.
Outlines
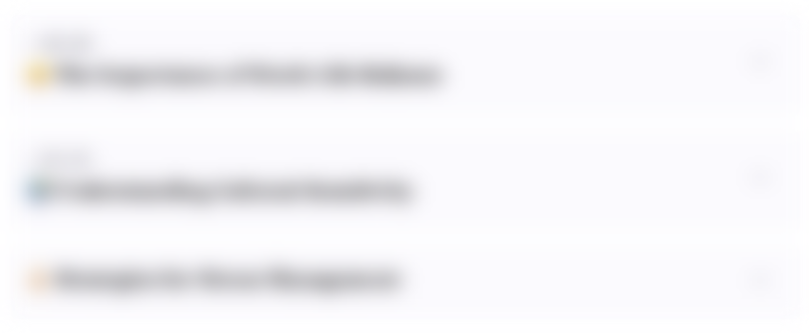
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنMindmap
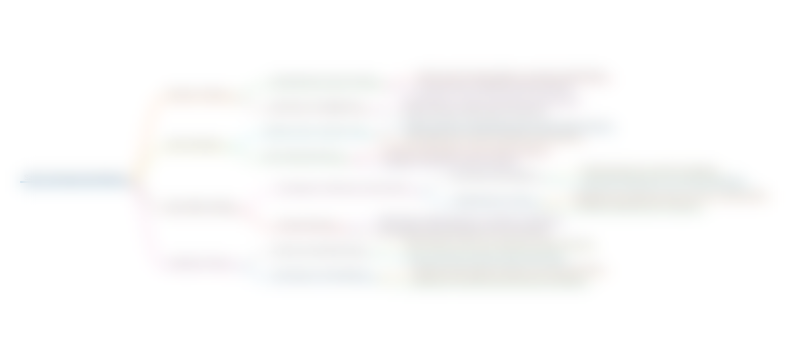
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنKeywords
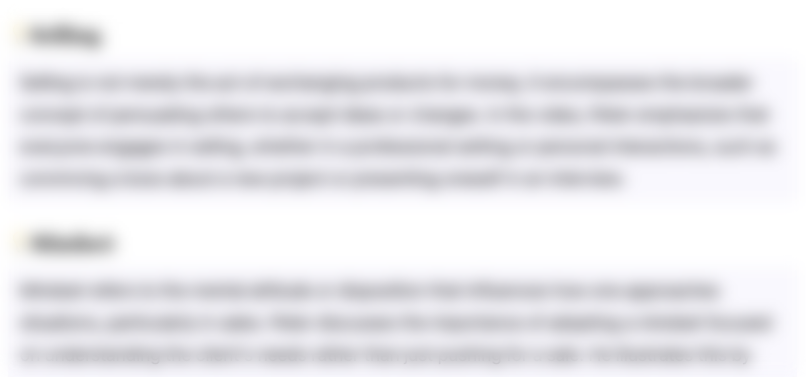
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنHighlights
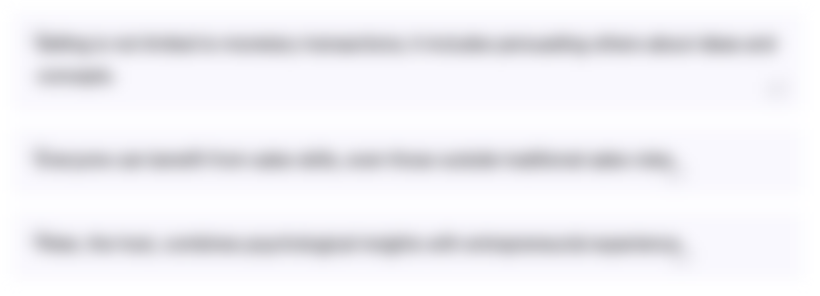
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنTranscripts
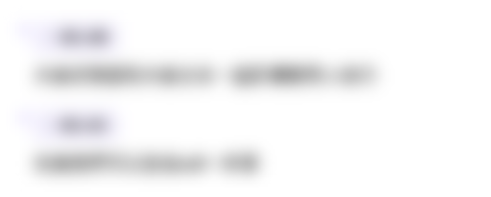
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنتصفح المزيد من مقاطع الفيديو ذات الصلة
5.0 / 5 (0 votes)