Variables in Java - Practice
Summary
TLDRIn this instructional video, the presenter guides viewers through Java programming basics, focusing on variable declaration, initialization, and value assignment. They demonstrate how to declare a string variable, assign it a value, and print it using System.out.println. The lesson also covers variable copying, showing that changes to the original variable do not affect the copied value. Additionally, the presenter explains how to access command-line arguments in Java, illustrating with an example that prints the first argument provided. The video is designed to solidify understanding of Java fundamentals and practical application.
Takeaways
- 💻 The lecture focuses on practicing Java programming concepts learned in a previous video.
- 🔑 The importance of declaring variables with a type and a name is emphasized, following the camelCase convention.
- ⚠️ Java requires variables to be initialized before they can be used, as demonstrated by the error when trying to print an uninitialized variable.
- 📝 The process of assigning values to variables using the assignment operator '=' is shown.
- 🔄 The difference between declaring and initializing a variable in one statement is explained.
- 🔗 The concept of variable copying is introduced, where a new variable is initialized with the value of an existing variable.
- 🛠️ The script demonstrates how to change the value of a variable and shows that the copy remains unaffected.
- 🗣️ The use of command-line arguments in Java programs is discussed, with a focus on accessing the first argument using `args[0]`.
- 📌 The script shows how to print command-line arguments using `System.out.println()` and how to handle additional unused arguments.
- 🚫 The potential error of trying to access an argument that hasn't been provided is highlighted, emphasizing proper error handling.
Q & A
What is the purpose of the lecture in the transcript?
-The purpose of the lecture is to practice what was learned in the previous video, focusing on working with variables in Java within the IntelliJ IDE.
How is a variable of type string declared in Java?
-A variable of type string is declared by specifying the type 'String', followed by a space, the variable name using camelCase convention, and ending with a semicolon.
Why is it necessary to initialize a variable in Java before using it?
-In Java, variables must be initialized before use because the language requires that a value is placed inside the variable to avoid errors and ensure proper memory allocation.
What is the error that occurs if a string variable is declared without initialization?
-The error that occurs is that the variable has not been initialized, meaning no value has been assigned to it yet, which is required in Java before the variable can be used.
How can a string variable be initialized with a value at the time of declaration?
-A string variable can be initialized by assigning a value to it immediately after the declaration using the assignment operator '='.
What is the output when the program is run after initializing the variable 'myName'?
-The output will be the value assigned to the 'myName' variable, which is printed to the console.
What is the difference between declaring a variable and initializing it?
-Declaring a variable involves specifying its type and name, while initializing a variable involves assigning an initial value to it. Initialization can be done at the time of declaration.
Why does creating a variable named 'myNameCopy' and assigning it the value of 'myName' result in a copy of the value?
-Assigning the value of 'myName' to 'myNameCopy' creates a copy of the value, meaning any changes to 'myName' will not affect 'myNameCopy' as they are separate variables with separate memory allocations.
How can command-line arguments be accessed in a Java program?
-Command-line arguments can be accessed using the 'args' array, where 'args[0]' represents the first argument passed to the program.
What happens if a program tries to access an argument that was not provided?
-Attempting to access an argument that was not provided will result in an ArrayIndexOutOfBoundsException, indicating that the index is out of bounds for the size of the 'args' array.
Why is it okay to have unused command-line arguments in a Java program?
-It is okay to have unused command-line arguments because they do not affect the execution of the program unless specifically accessed and used in the code.
Outlines
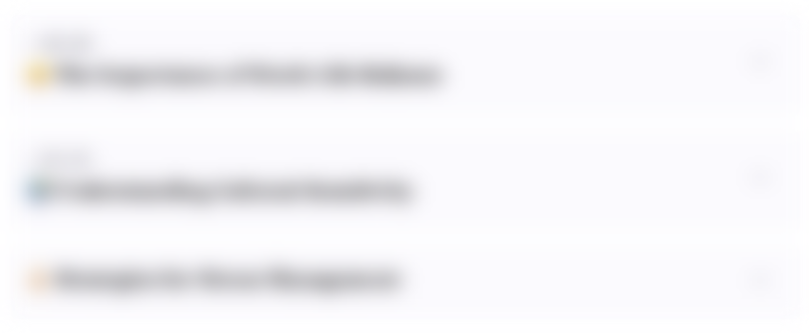
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنMindmap
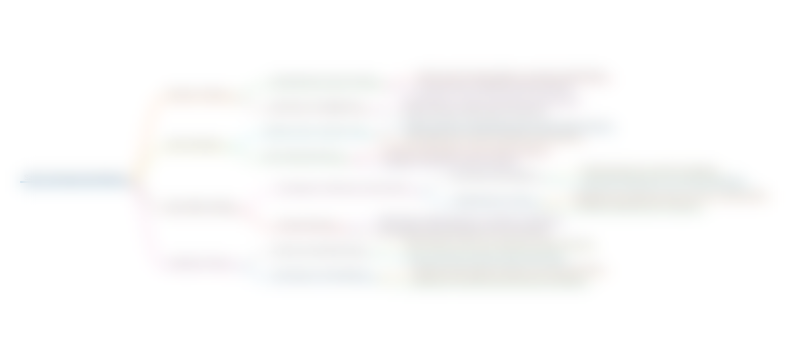
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنKeywords
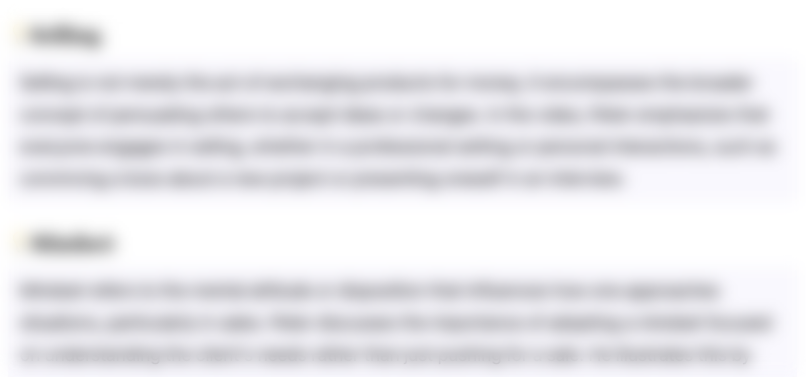
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنHighlights
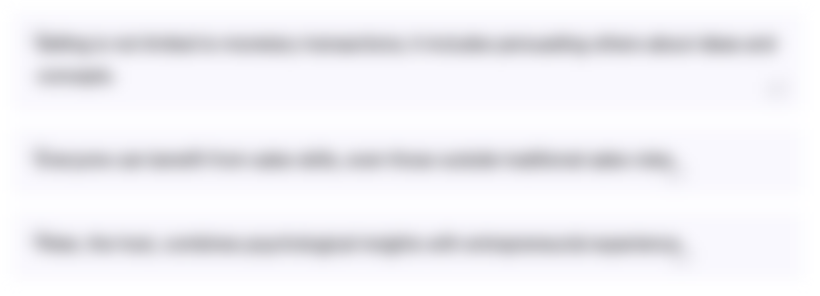
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنTranscripts
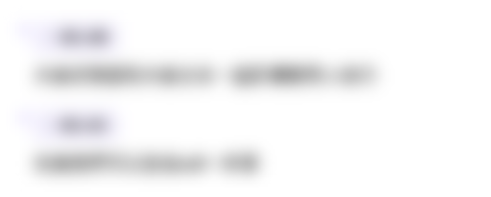
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآن5.0 / 5 (0 votes)