Object Methods | JavaScript 🔥 | Lecture 041
Summary
TLDRThe lecture discusses adding functions called methods to JavaScript objects, allowing objects to have capabilities beyond just storing data. Methods are created by assigning a function expression as an object property value. The 'this' keyword gives methods access to other data in the object. An example method called calcAge() calculates a person's age from their birth year. Another method getSummary() returns a string summarizing the person's data. This demonstrates that arrays are also objects that have built-in methods, foreshadowing more complex topics ahead about why arrays can have methods.
Takeaways
- 😀 Objects can contain functions as values, called methods
- 👍 Methods can access the object's properties using the 'this' keyword
- 💡 'this' refers to the object calling the method
- 📝 Methods can calculate and store data in the object for later use
- 🚦 Arrays are actually a special type of object with built-in methods
- 🔑 Writing methods keeps code DRY by avoiding repetition
- ✏️ Challenges help reinforce learning of new concepts
- 🎯 Object methods enable efficient data access and manipulation
- 🚀 There is an exciting programming journey ahead to dive deeper
- 🧠 Understanding function expressions enables writing methods
Q & A
What are object methods?
-Object methods are functions that are attached to an object. They are also called method properties on objects.
How do you add a method to an object in JavaScript?
-To add a method to an object, you define a function expression as the value for a property in the object. The property name is the name of the method and the function expression becomes the method definition.
What is the 'this' keyword in a method?
-The 'this' keyword in a method refers to the object on which the method is being called. So 'this' points to the object calling the method.
Why use the 'this' keyword instead of hard-coding the object name?
-Using the 'this' keyword allows the code to work automatically if the object name changes, following the DRY (Don't Repeat Yourself) principle by avoiding hard-coding the name.
How can an object method access other properties on the same object?
-By using the 'this' keyword, a method can access other properties defined on the same object, for example with 'this.propertyName'.
What are built-in array methods?
-Built-in array methods are functions that allow manipulation of arrays, like push(), pop(), shift(), unshift() etc. Arrays have these because arrays are also objects.
What is method chaining?
-Method chaining is calling one method after another sequentially by chaining them together with the dot notation, for example array.push().pop().
Should calculation logic go directly in object property values?
-No, any complex logic should go in methods instead, while property values can cache results, avoiding repeat calculations.
Why define a getSummary() method instead of a summary property?
-Defining a getSummary() method allows dynamically generating the summary string when needed, ensuring it stays up-to-date if properties change.
What is the main advantage of using OOP in JavaScript?
-The main advantage of OOP in JavaScript is better organization by grouping related properties and behaviors into objects, keeping code modular and reusable.
Outlines
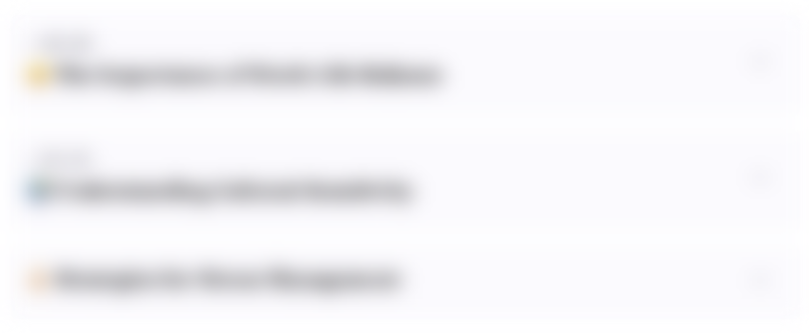
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنMindmap
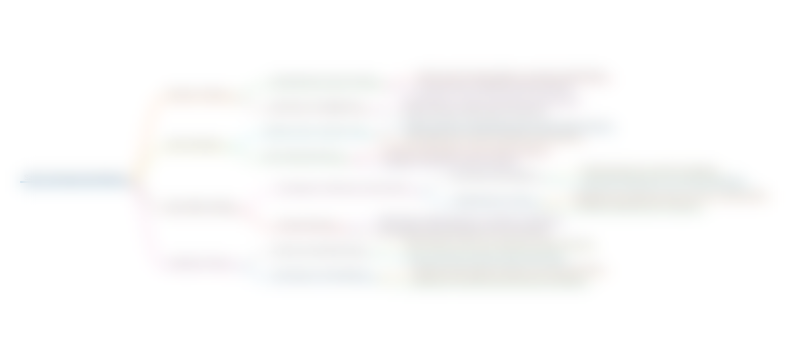
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنKeywords
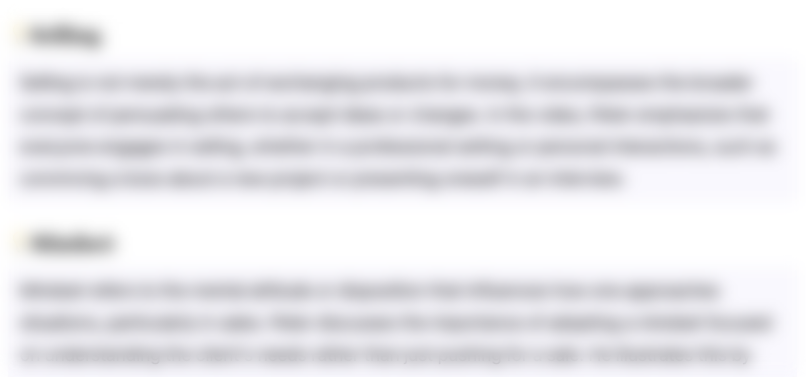
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنHighlights
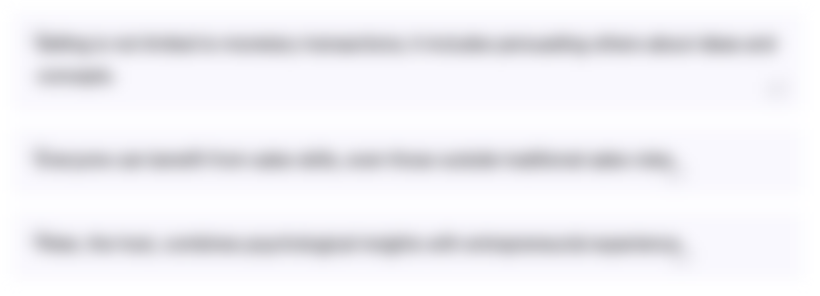
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنTranscripts
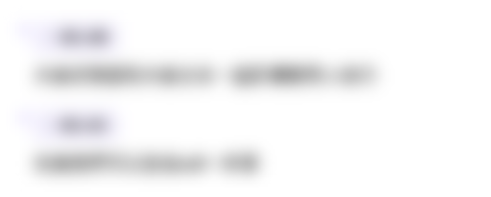
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنتصفح المزيد من مقاطع الفيديو ذات الصلة
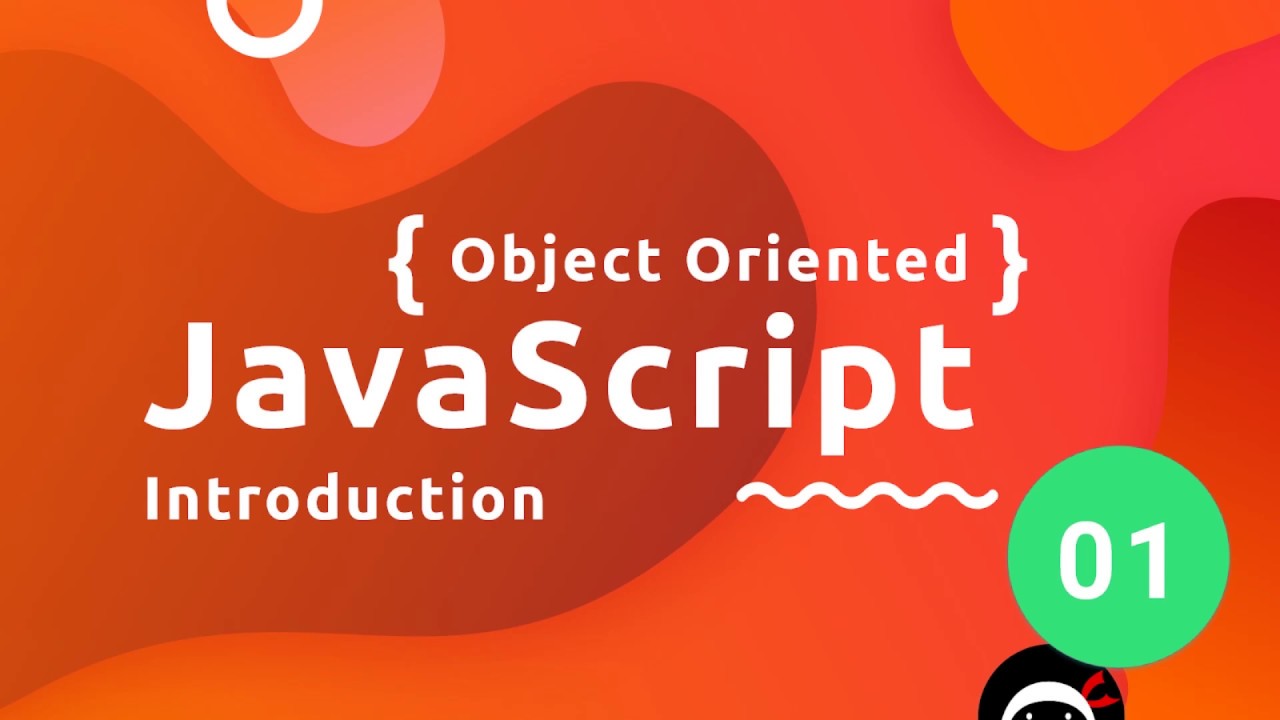
Object Oriented JavaScript Tutorial #1 - Introduction
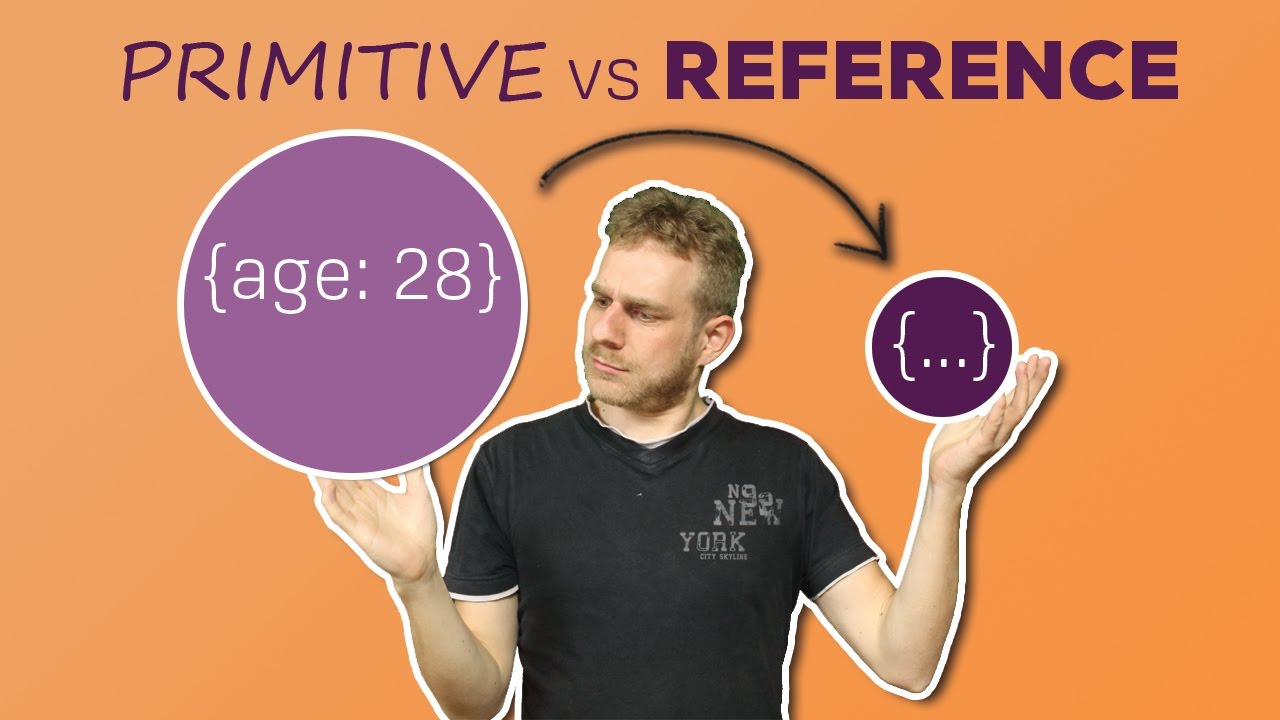
JavaScript - Reference vs Primitive Values/ Types
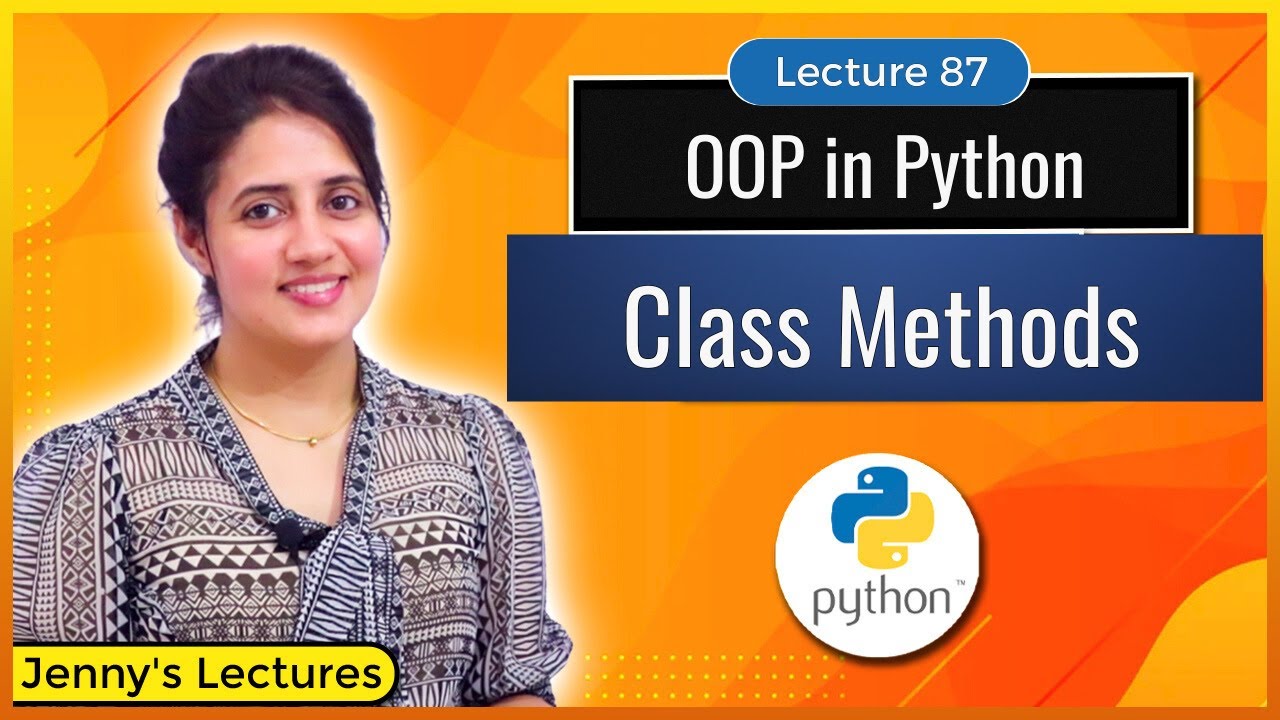
Class Methods in Python | How to add Methods in Class | Python Tutorials for Beginners #lec87

AP Computer Science A - Unit 3: Boolean Expressions And if Statements

Classes and Objects in Python | OOP in Python | Python for Beginners #lec85
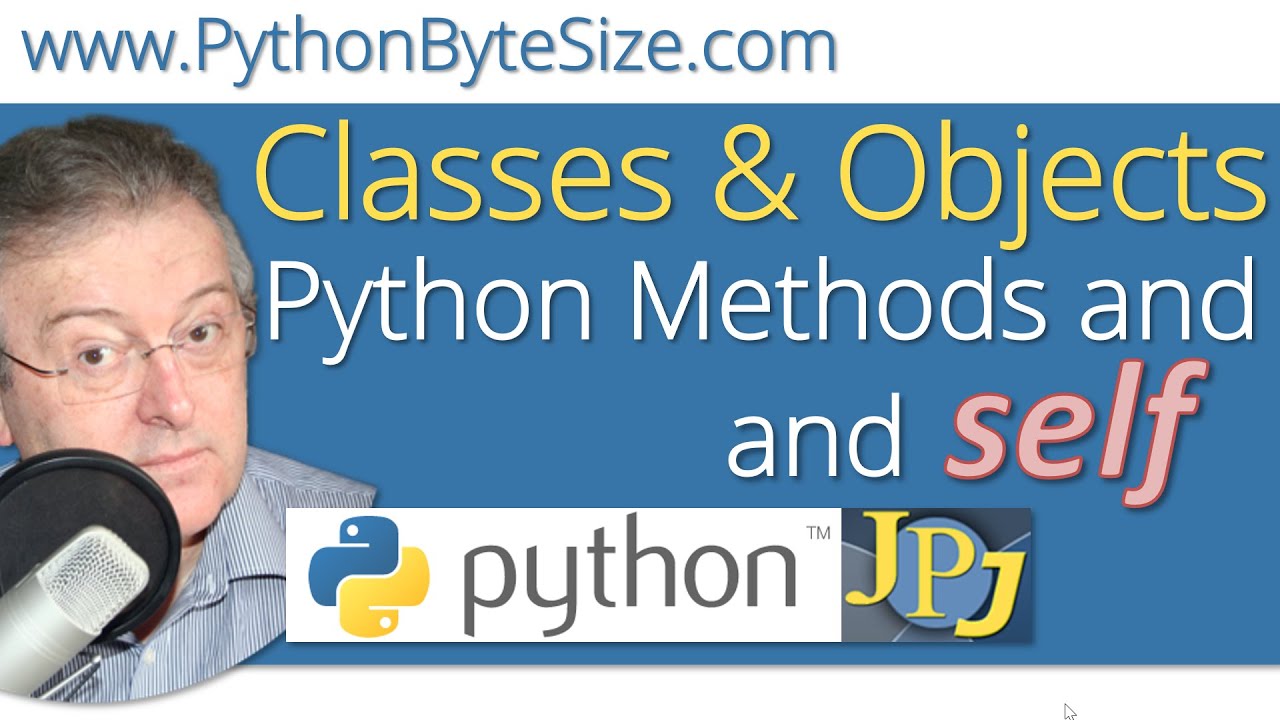
Python Methods and self
5.0 / 5 (0 votes)