#20 C Multidimensional Arrays | C Programming For Beginners
Summary
TLDRIn this instructional video, Padma from Programmers introduces multi-dimensional arrays in C programming, emphasizing their structure as arrays of arrays. Viewers learn about the syntax, initialization, and how to access and modify elements within these arrays. The video demonstrates practical examples, including the use of nested for loops for iteration and matrix multiplication. Engaging viewers with a question about array indexing, Padma encourages comments and interaction. Additionally, she promotes a mobile app offering a comprehensive C programming course, enhancing the learning experience with practical coding exercises.
Takeaways
- 😀 A multi-dimensional array is an array of arrays, allowing storage of multiple elements together in a structured way.
- 😀 The syntax for declaring a two-dimensional array includes the data type, array name, and its dimensions (e.g., int arr[2][3]).
- 😀 A two-dimensional array can be visualized as a table with rows and columns, where each row is an inner array.
- 😀 To initialize a multi-dimensional array, values are assigned using nested curly brackets, e.g., int arr[2][3] = {{1, 3, 5}, {2, 4, 6}}.
- 😀 Accessing elements in a multi-dimensional array requires two indexes: one for the row and one for the column (e.g., arr[0][0] accesses the first element).
- 😀 Elements in a multi-dimensional array can be modified using their indexes, allowing for dynamic changes to the data stored.
- 😀 Nested loops can be used to iterate through each element of a multi-dimensional array, facilitating operations on all array elements.
- 😀 The outer loop runs for the number of rows, while the inner loop iterates through the columns for each row.
- 😀 Proper formatting of output can enhance readability when printing multi-dimensional arrays to the console.
- 😀 The video encourages viewer engagement through likes and comments, highlighting the importance of community interaction in learning.
Q & A
What is a multi-dimensional array?
-A multi-dimensional array is an array that contains other arrays as its elements, allowing for the storage of data in a structured format, like a table.
How is a two-dimensional array declared in C?
-A two-dimensional array in C can be declared using the syntax: `int arr[2][3];`, which creates an array with 2 arrays of 3 elements each.
What does the size '2' and '3' represent in a two-dimensional array?
-The size '2' represents the number of inner arrays, while '3' represents the number of elements in each inner array, allowing for a total of 6 elements.
How do you initialize a two-dimensional array?
-A two-dimensional array can be initialized using nested curly brackets, like this: `int arr[2][3] = {{1, 3, 5}, {2, 4, 6}};`.
How can you access elements in a multi-dimensional array?
-Elements in a multi-dimensional array can be accessed using multiple indexes, for example, `arr[0][0]` accesses the first element, while `arr[1][2]` accesses the third element of the second array.
How do you modify an element in a multi-dimensional array?
-You can modify an element using its index, such as `arr[0][2] = 7;`, which changes the value at that position.
What is the purpose of using nested loops with multi-dimensional arrays?
-Nested loops allow you to iterate through each element in a multi-dimensional array, making it easy to access and manipulate all elements in structured formats.
Can you provide an example of a nested loop for a two-dimensional array?
-Yes, a typical nested loop for accessing a two-dimensional array looks like this: `for (int i = 0; i < 2; i++) { for (int j = 0; j < 3; j++) { printf("%d ", arr[i][j]); } printf("\n"); }`.
What should viewers do if they want to support the channel?
-Viewers are encouraged to leave comments, like the video, and subscribe to enhance engagement and help more people discover the programming courses.
Where can viewers find additional resources for learning C programming?
-Viewers can find additional programming resources and examples on the GitHub repository linked in the video description.
Outlines
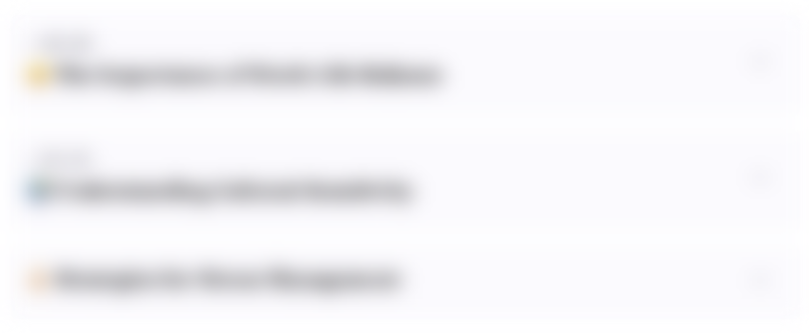
此内容仅限付费用户访问。 请升级后访问。
立即升级Mindmap
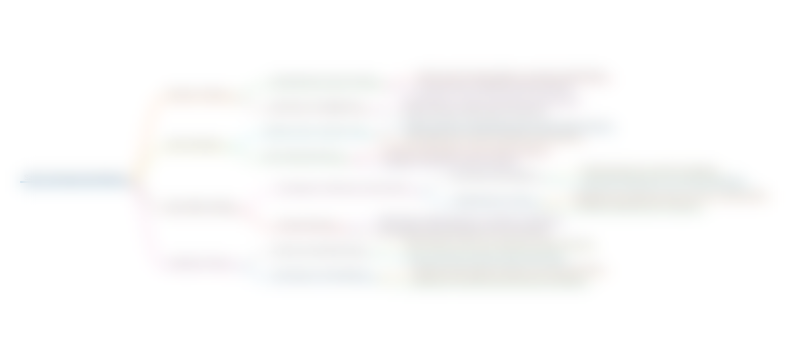
此内容仅限付费用户访问。 请升级后访问。
立即升级Keywords
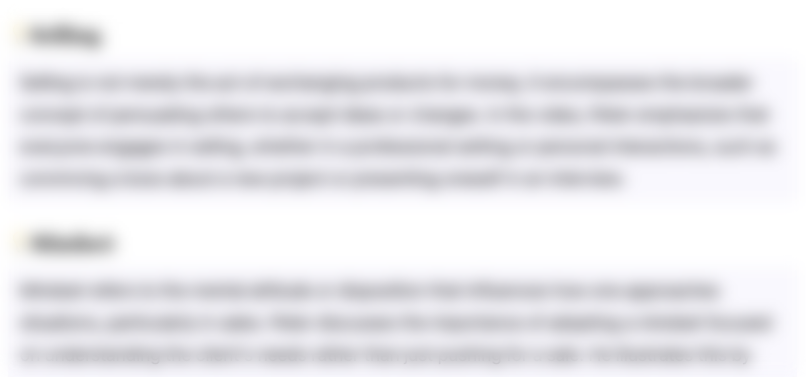
此内容仅限付费用户访问。 请升级后访问。
立即升级Highlights
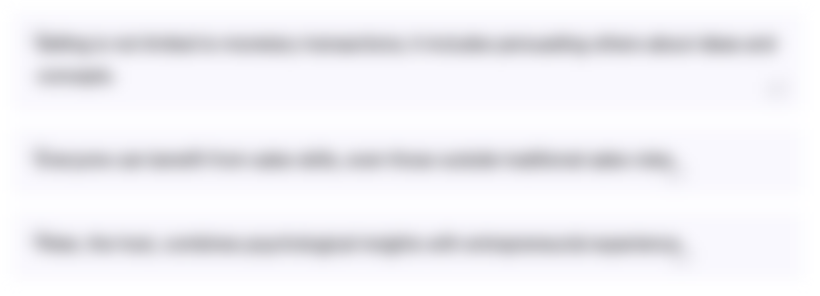
此内容仅限付费用户访问。 请升级后访问。
立即升级Transcripts
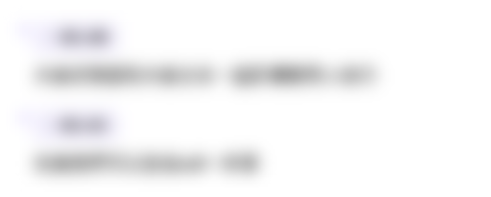
此内容仅限付费用户访问。 请升级后访问。
立即升级浏览更多相关视频
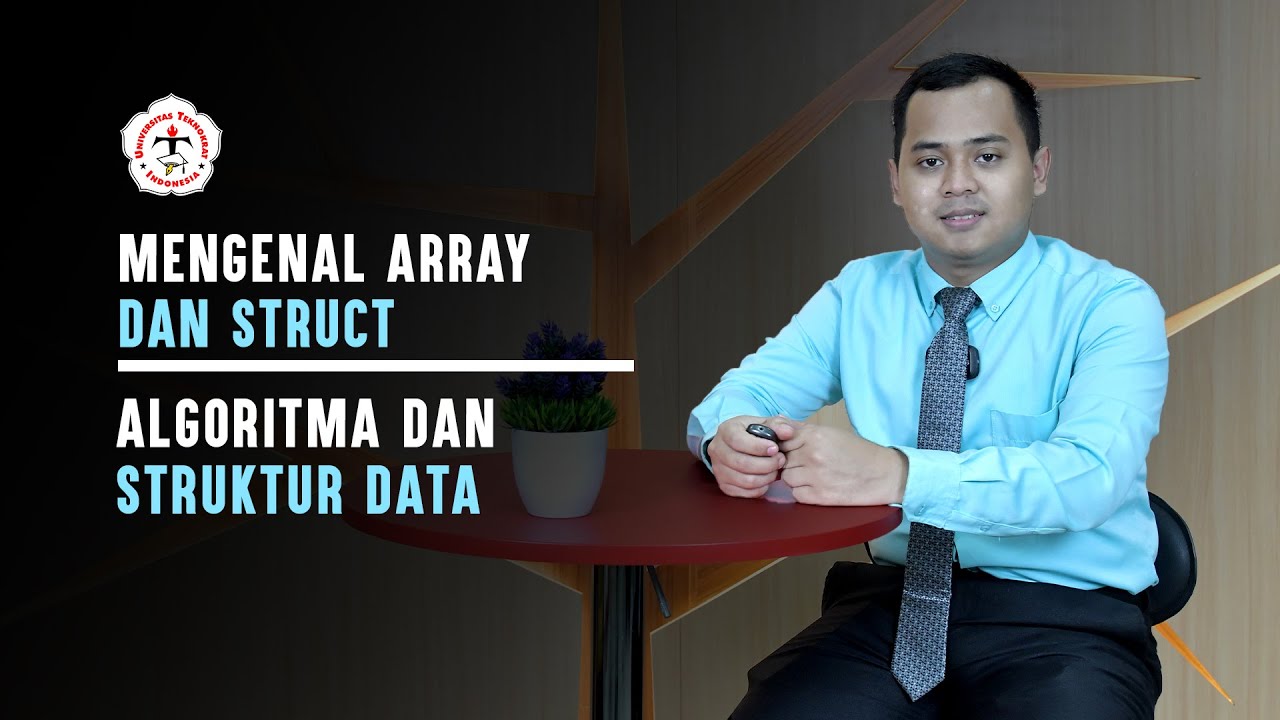
Mengenal Array dan Struct Dalam Algoritma Struktur Data
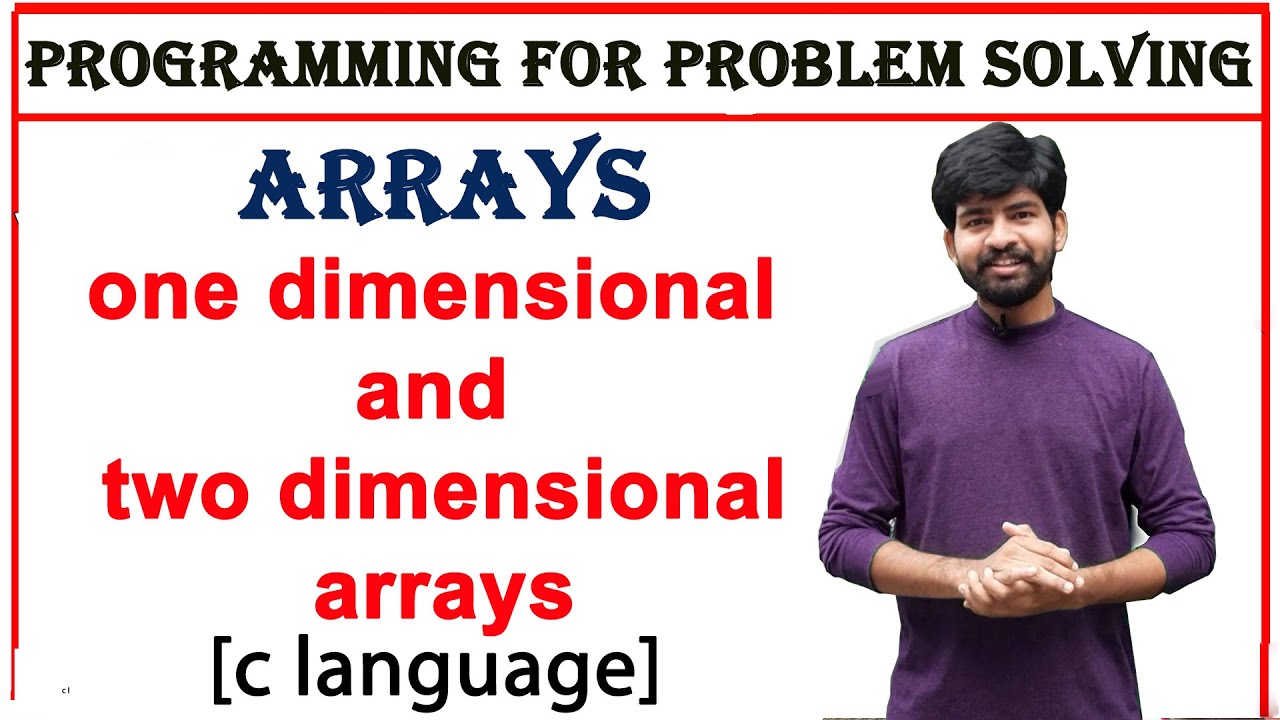
arrays in c, one dimensional array, two dimensional array |accessing and manipulating array elements
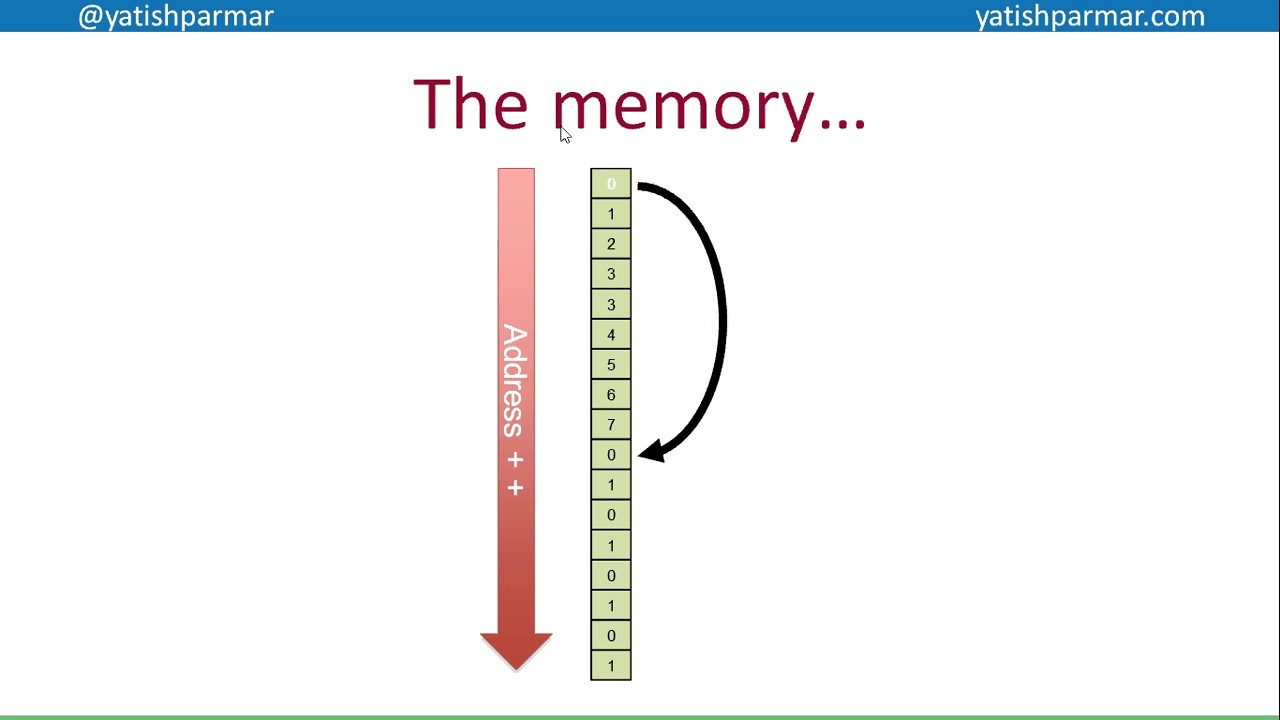
2D arrays - A Level Computer Science
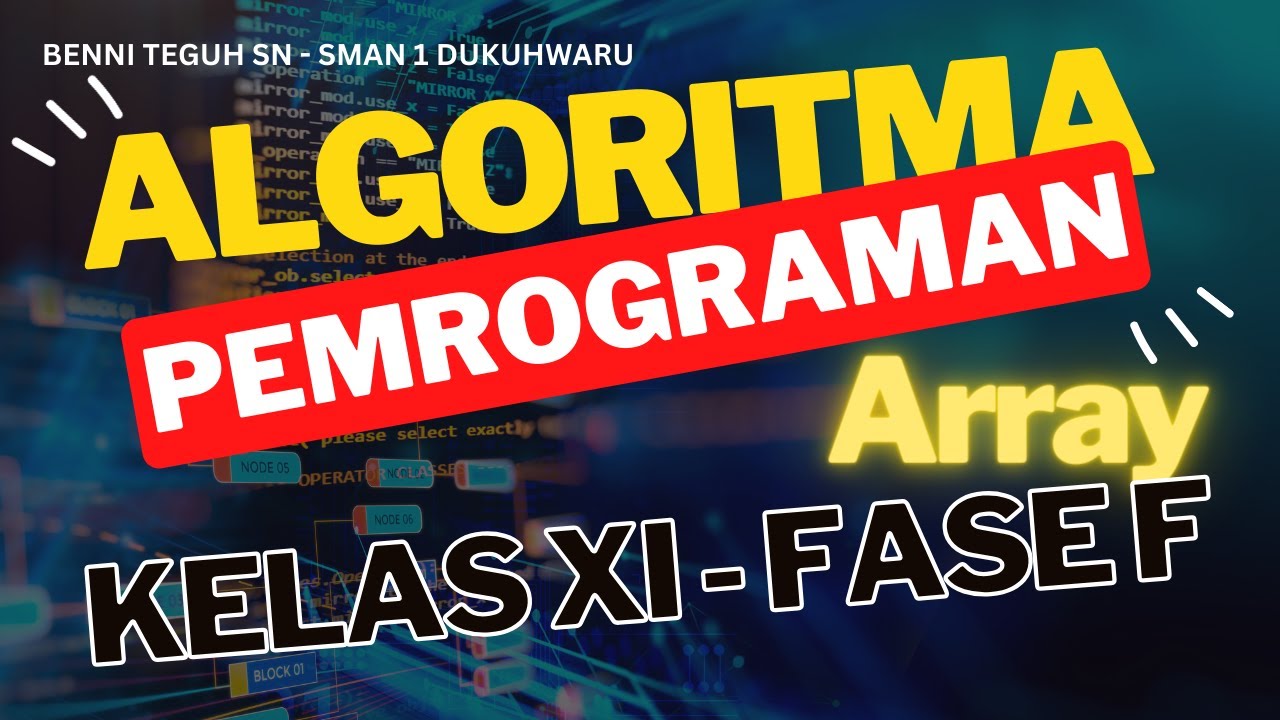
Larik (Array) - Algoritma dan Pemrograman

NumPy Python - What is NumPy in Python | Numpy Python tutorial in Hindi
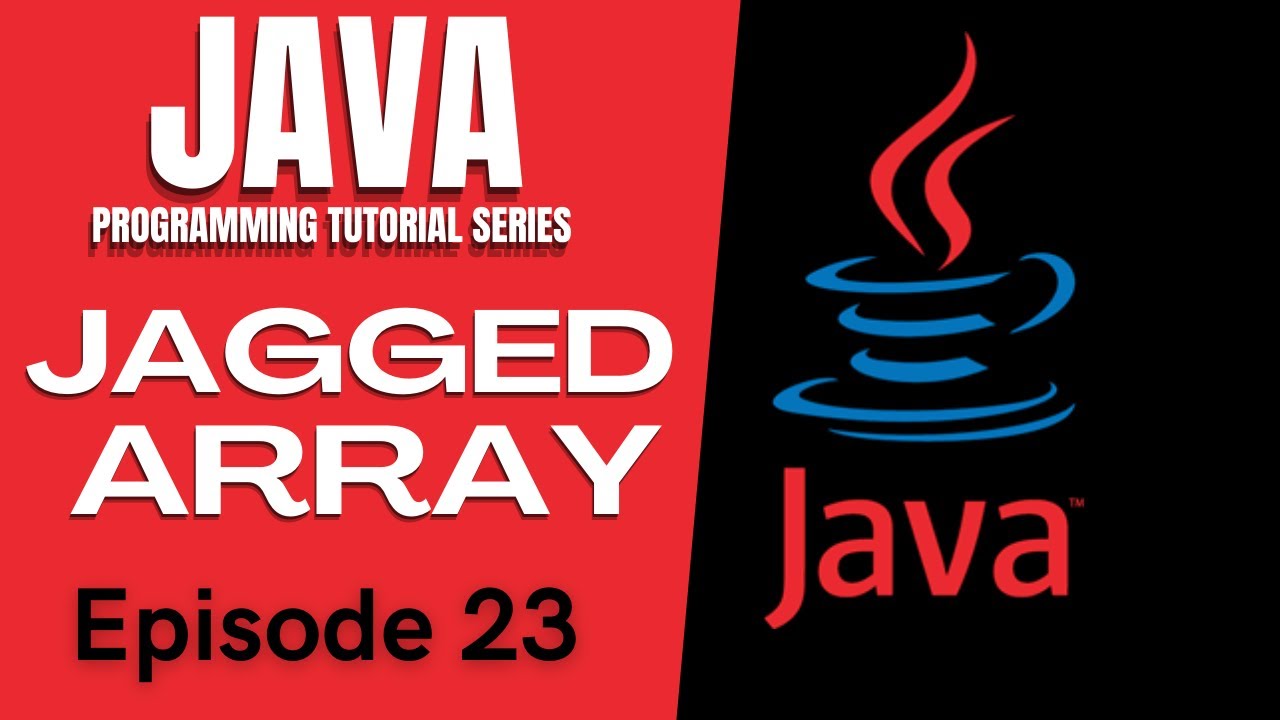
Java Tutorial #23 | 2D JAGGED ARRAYS IN JAVA | RAGGED |Tagalog | English | Filipino | 2021
5.0 / 5 (0 votes)