Strategy Design Pattern Tutorial with Java Coding Example | Strategy Pattern Explained
Summary
TLDRIn this episode of Goldman Digest, the focus is on the Strategy Design Pattern, a software design pattern that allows the behavior of an object to change at runtime. The video explains the concept, provides a Java implementation, and discusses its benefits, including adherence to the Open/Closed Principle and the ability to switch algorithms dynamically. Examples like transportation strategies and a navigation app illustrate the pattern's utility. The host encourages viewers to subscribe and engage with the content, promising a follow-up on the Observer Design Pattern in the next installment.
Takeaways
- 😀 The video introduces the Strategy Design Pattern, explaining its concept and applications.
- 🔍 It discusses where to use the Strategy Design Pattern in projects, highlighting its utility in software development.
- 💻 The presenter provides a Java code implementation of the Strategy Design Pattern, demonstrating its practical application.
- 🚀 The video emphasizes the benefits of using the Strategy Design Pattern, such as the ability to change algorithms at runtime.
- 📚 A real-world example is given to illustrate the Strategy Design Pattern, making it easier to understand its use cases.
- 🔗 The video links to a GitHub repository where viewers can access the code for the Strategy Design Pattern discussed.
- 📈 The presenter outlines scenarios where the Strategy Design Pattern is particularly useful, such as when multiple algorithms are needed.
- 🛠️ The video explains the Open/Closed Principle in the context of the Strategy Design Pattern, emphasizing code maintainability.
- 📝 The script encourages viewers to engage by subscribing, liking, and sharing the video, and to comment on their experiences with the pattern.
- ⏭️ The video concludes with a teaser for the next video, which will cover the Observer Design Pattern.
Q & A
What is a strategy design pattern?
-A strategy design pattern is a behavioral design pattern that enables an algorithm's behavior to be selected at runtime. It allows the definition of a family of algorithms, encapsulates each one, and makes them interchangeable. Strategy lets the algorithm vary independently from clients that use it.
What is the benefit of using the strategy design pattern?
-Using the strategy design pattern allows you to swap algorithms used inside an object at runtime, supports the open/closed principle by being open for extension but closed for modification, isolates the implementation detail of an algorithm from the code that uses it, and can replace inheritance with composition.
How does the strategy design pattern relate to the open/closed principle?
-The strategy design pattern exemplifies the open/closed principle by allowing new functionalities to be added (open for extension) without modifying existing code (closed for modification). This is achieved by adding new algorithm strategies without altering the context or other strategies.
Can you provide a real-world example of the strategy design pattern mentioned in the script?
-Yes, a real-world example given in the script is choosing a mode of transportation to the airport. Depending on factors like budget or time constraints, one might choose between a bus, a cab, or a bicycle, each representing a different strategy.
What is the context class in the strategy design pattern?
-The context class in the strategy design pattern is the driver class that encapsulates the strategy. It maintains a reference to one of the strategy objects and delegates the work to the linked strategy object instead of executing its own algorithms.
How does the strategy design pattern help in maintaining a navigation app as described in the script?
-The strategy design pattern helps in maintaining a navigation app by allowing the app to add new routing algorithms (like car, cyclist, or walking routes) without significantly increasing the complexity of the main class. It does this by delegating the routing logic to strategy objects, keeping the main class clean and manageable.
What is the role of the strategy interface in the strategy design pattern?
-The strategy interface in the strategy design pattern defines a common interface for all supported algorithms. It allows the context to work with all strategies because they are derived from the same interface, enabling the context to switch between different algorithms at runtime.
How can you add a new algorithm to the strategy design pattern without modifying existing code?
-To add a new algorithm without modifying existing code, you create a new class that implements the strategy interface and then instantiate this new strategy class when needed. The context can then use this new strategy object, adhering to the open/closed principle.
What is the difference between strategy pattern and state pattern as mentioned in the script?
-The strategy pattern is about defining a family of algorithms and making them interchangeable, whereas the state pattern allows an object to alter its behavior when its internal state changes. The strategy pattern is used to switch algorithms at runtime, and the state pattern is used to manage state transitions internally.
How does the script demonstrate the strategy design pattern with a calculator example?
-The script demonstrates the strategy design pattern with a calculator example by defining a strategy interface with a calculate method and various strategy implementations for addition, subtraction, multiplication, and division. The calculator context then uses these strategies to perform operations on numbers based on the selected strategy.
Outlines
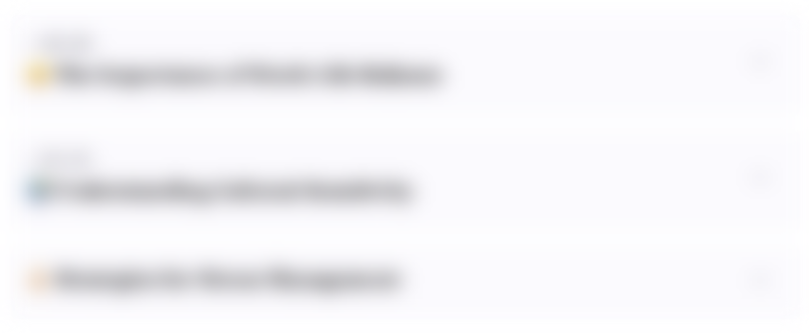
此内容仅限付费用户访问。 请升级后访问。
立即升级Mindmap
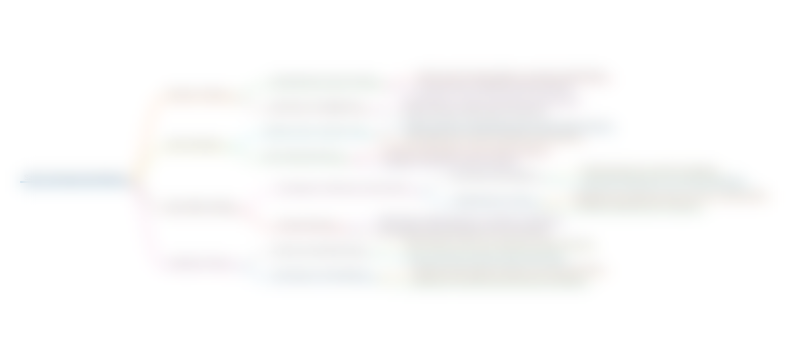
此内容仅限付费用户访问。 请升级后访问。
立即升级Keywords
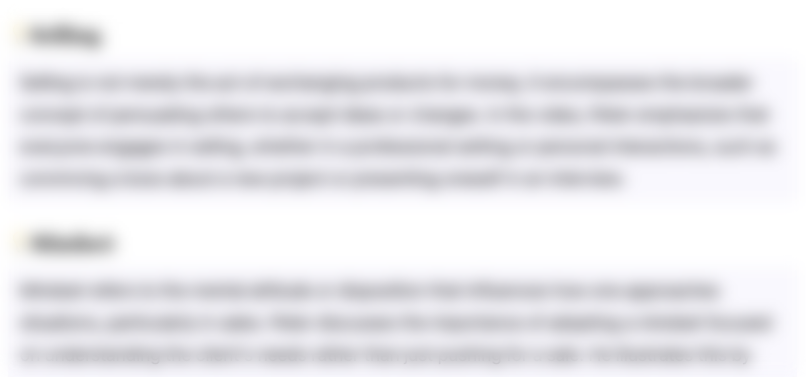
此内容仅限付费用户访问。 请升级后访问。
立即升级Highlights
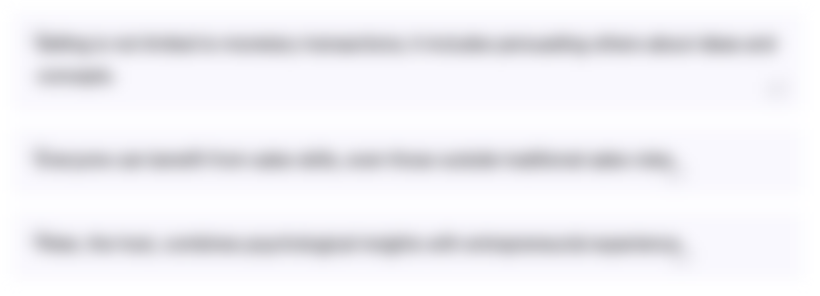
此内容仅限付费用户访问。 请升级后访问。
立即升级Transcripts
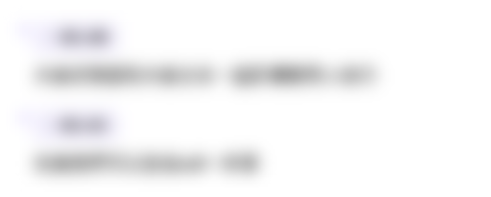
此内容仅限付费用户访问。 请升级后访问。
立即升级浏览更多相关视频

Builder Design Pattern Explained in 10 Minutes
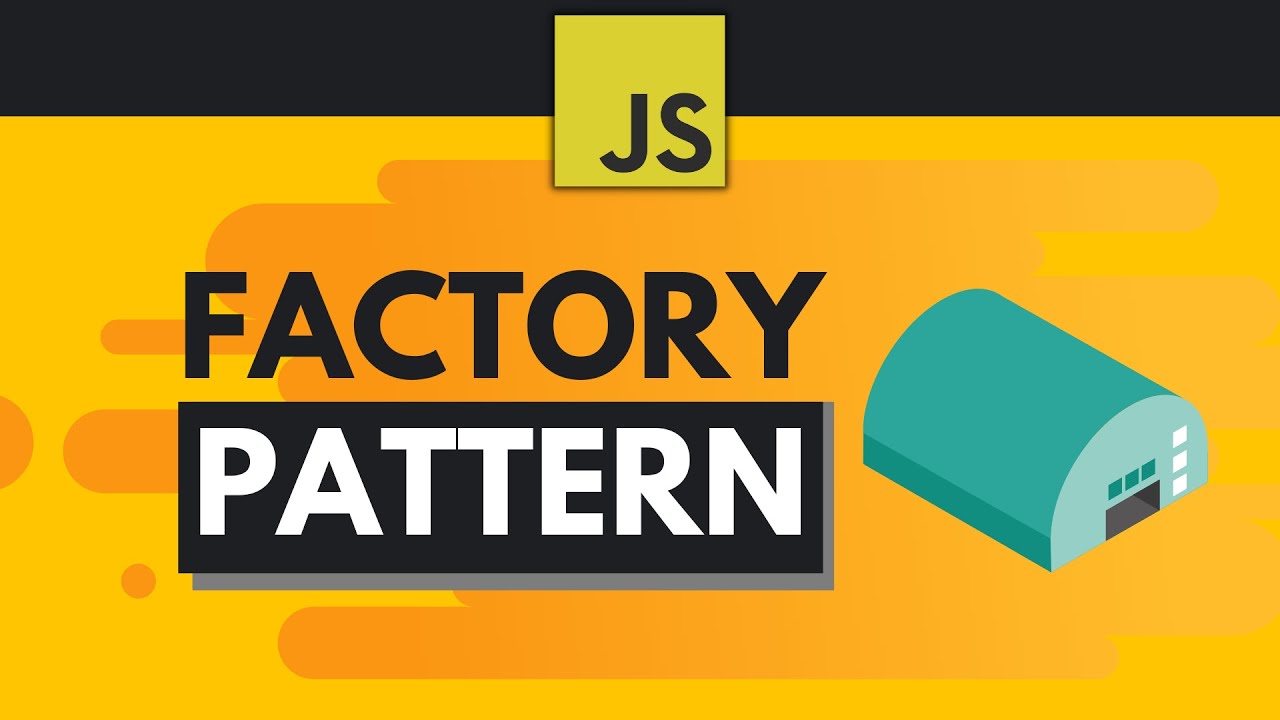
Javascript Design Patterns #1 - Factory Pattern
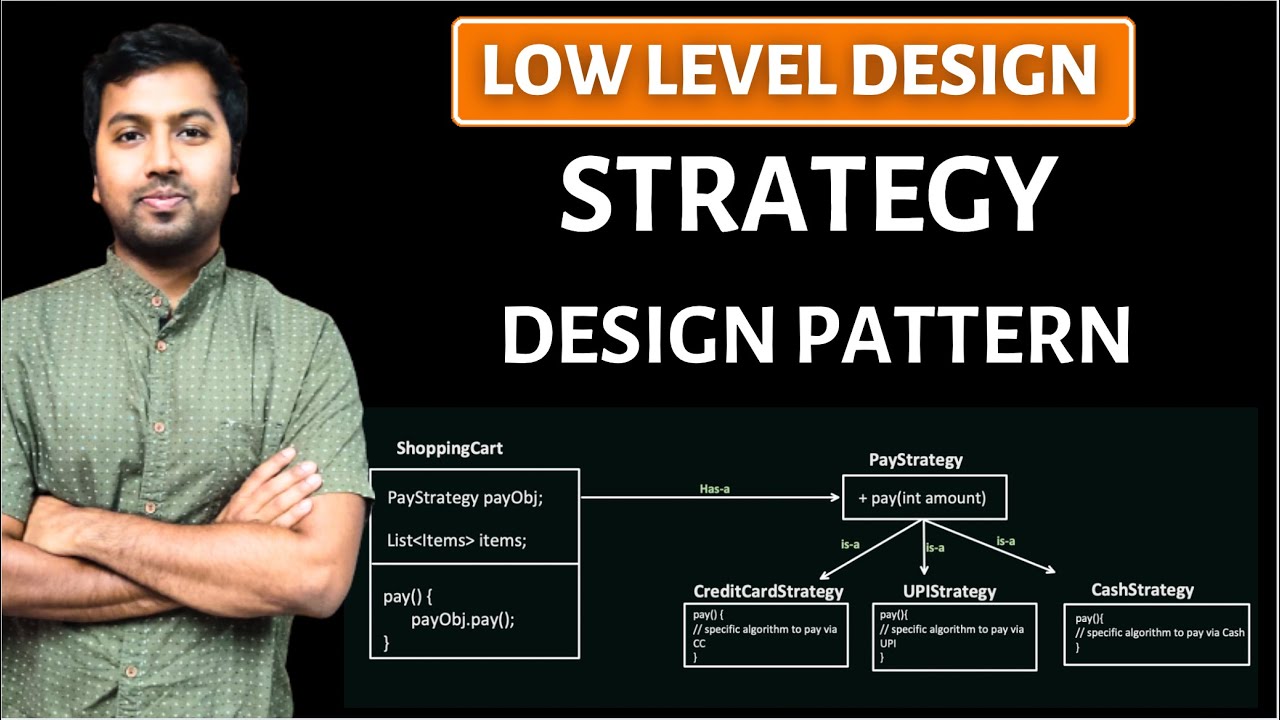
2. Strategy Design Pattern explanation (Hindi) | LLD System Design | Design pattern in Java
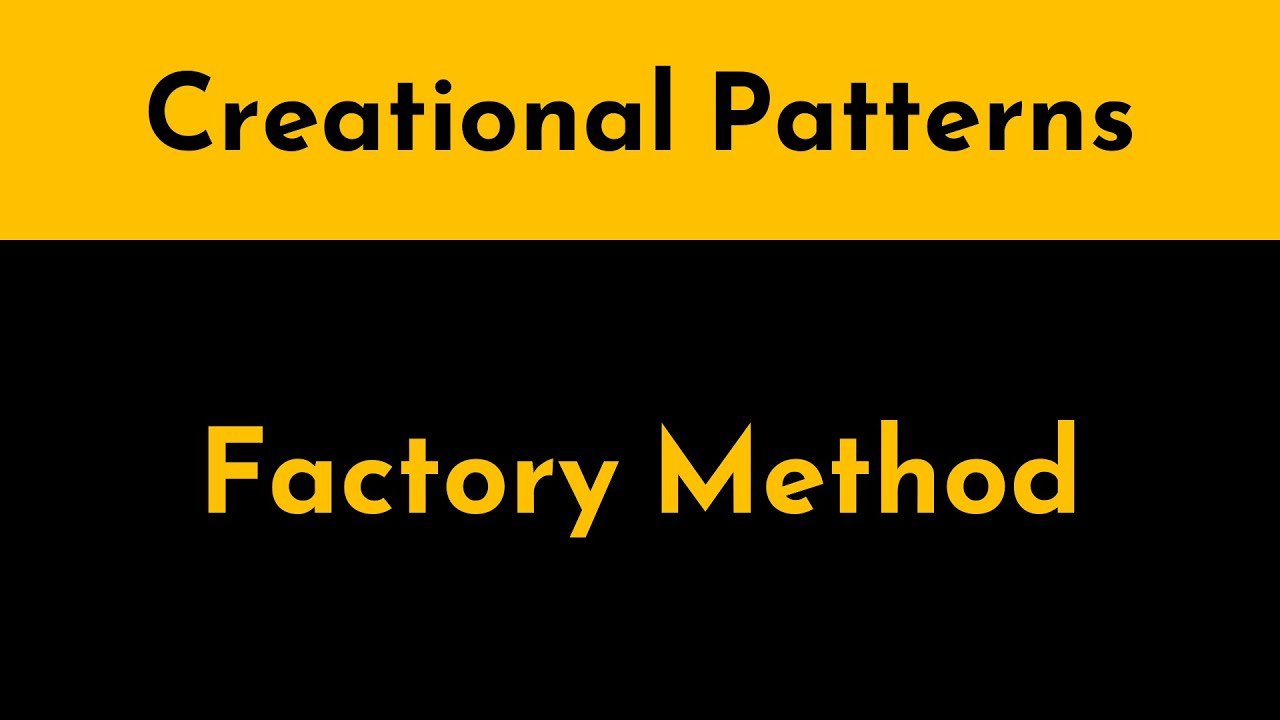
The Factory Method Pattern Explained and Implemented in Java | Creational Design Patterns | Geekific
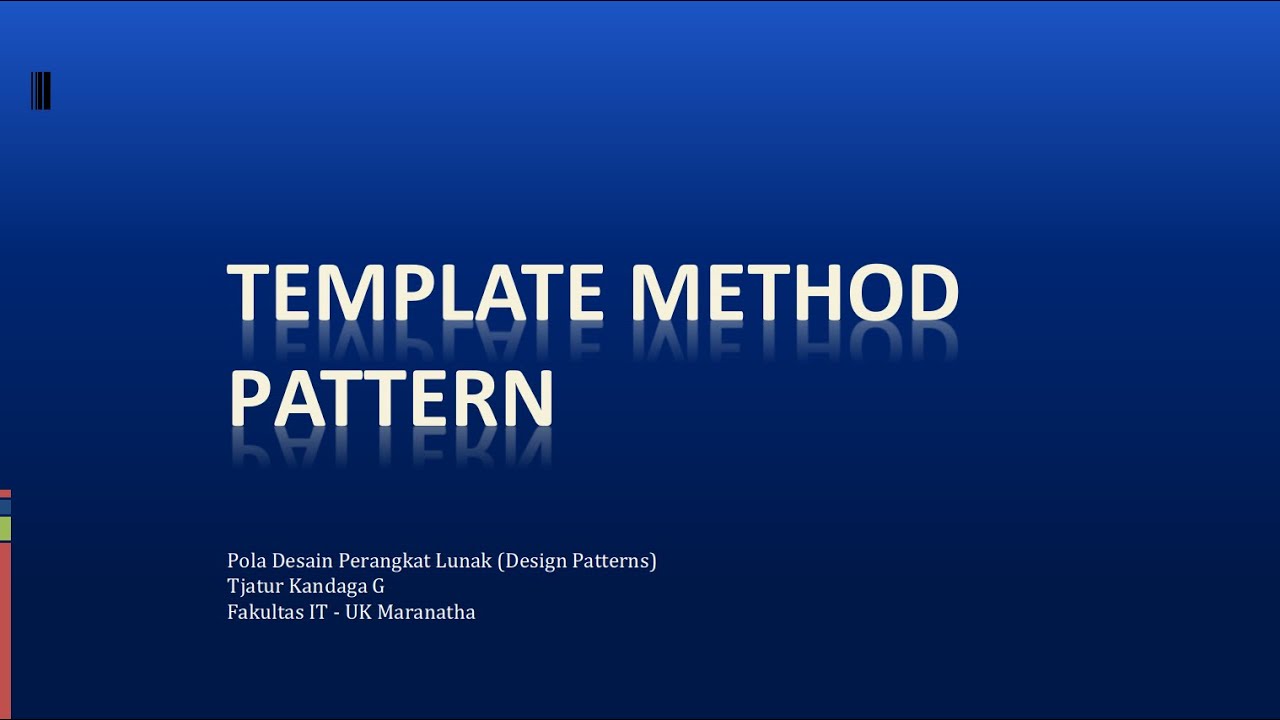
Template Method Pattern

The Chain of Responsibility Pattern Explained & Implemented | Behavioral Design Patterns | Geekific
5.0 / 5 (0 votes)