How To Make ANY Function Asynchronous In Python 3.12
Summary
TLDRこのビデオチュートリアルでは、Pythonで非同期関数に変換する方法を学びます。特に、同期モジュールであるrequestsモジュールを例に、APIからJSONをリクエストする際にコードの実行をブロックしないようにする技術を紹介します。asyncioモジュールとasyncio.to_threadを使用して、任意の同期関数を非同期に実行する方法を実践的に説明します。さらに、複数のウェブサイトのステータスを非同期にチェックするサンプルスクリプトを作成する過程も解説します。このビデオは、非同期プログラミングの基礎を学びたい方にとって価値あるリソースです。
Takeaways
- 🐍 Pythonの非同期プログラミングの重要性を説明しています。
- 🌐 'requests'モジュールは同期的であり、コードのブロックを防ぎたい場合があると述べています。
- ⚙️ 'pip install requests'コマンドを使って、'requests'モジュールをインストールする方法を紹介しています。
- 🔍 'requests'から'response'タイプをインポートする方法を説明しています。
- 🖥️ 複数のウェブサイトのステータスを非同期でチェックするサンプルスクリプトを作成する過程を示しています。
- 🛠️ 'asyncio'と'from asyncio import task'をインポートすることで非同期関数を作成する方法を説明しています。
- 📡 'await asyncio.to_thread()'を使用して、任意の同期関数を非同期関数に変換する方法を説明しています。
- 🌍 特定のURLからステータスコードを非同期に取得する具体的な方法を示しています。
- 🏃 'async def main()'を使用して非同期タスクを実行し、結果を表示する方法を説明しています。
- ✨ Pythonで非同期プログラミングを活用することの便利さとパワフルさを強調しています。
Q & A
Pythonで非同期関数に変換する方法は何ですか?
-非同期IO(asyncio)モジュールの`to_thread`関数を使用して、同期関数を非同期関数に変換することができます。
`requests`モジュールを非同期で使用するにはどうすればよいですか?
-`asyncio.to_thread`を使って、`requests.get`関数を非同期に実行できます。
非同期関数で戻り値の型を指定するにはどうすればよいですか?
-関数の定義時に型注釈を使って戻り値の型を指定できます。例えば、`async def fetch_status(url: str) -> dict:`のようにします。
複数のウェブサイトのステータスを非同期でチェックするにはどうすればよいですか?
-非同期関数を定義し、`asyncio.create_task`を使ってそれぞれのウェブサイトのステータスを非同期にフェッチするタスクを作成します。
`asyncio.run`の役割は何ですか?
-`asyncio.run`は、非同期プログラムのメインエントリポイントを実行するために使用されます。
`asyncio.to_thread`の引数には何を指定しますか?
-実行したい関数、その関数に渡す引数、およびキーワード引数を指定します。
非同期関数の中で`await`キーワードの役割は何ですか?
-`await`キーワードは、非同期実行を待機し、その結果を取得するために使用されます。
非同期プログラミングで`Task`型の重要性は何ですか?
-`Task`型は、非同期に実行されるコルーチンを管理し、その結果を後で取得できるようにするために使用されます。
同期関数を非同期関数に変換するメリットは何ですか?
-ブロッキング操作を避け、アプリケーションのレスポンス性を向上させることができます。
非同期関数を使う際に、なぜ型注釈を使うのが良いとされていますか?
-型注釈を使用することで、関数の入力と戻り値の型が明確になり、コードの可読性と保守性が向上します。
Outlines
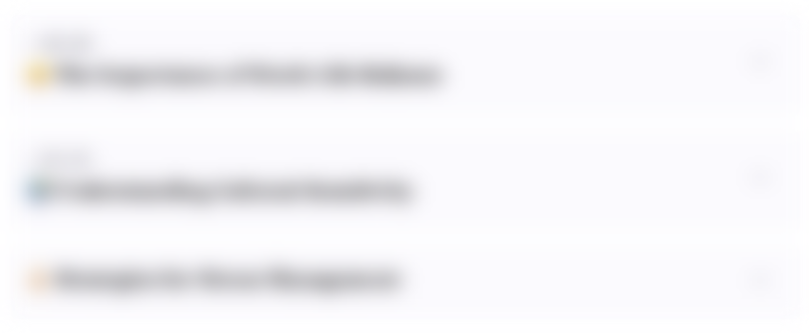
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
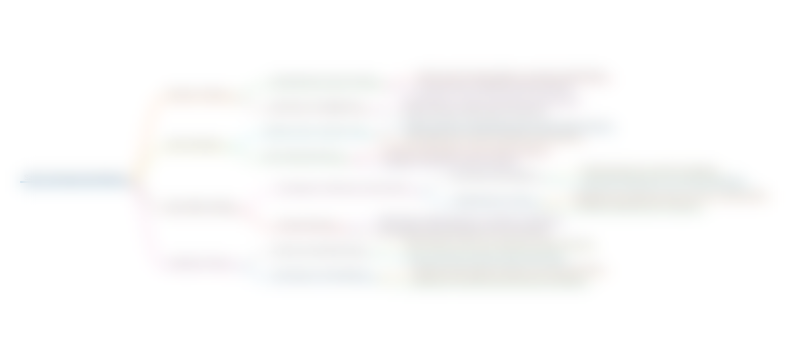
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
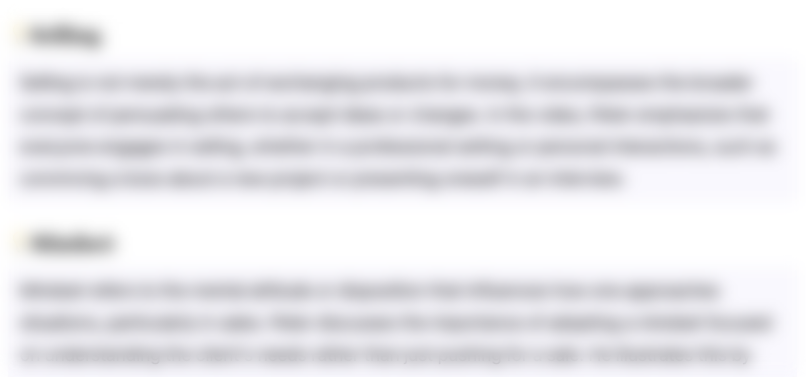
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
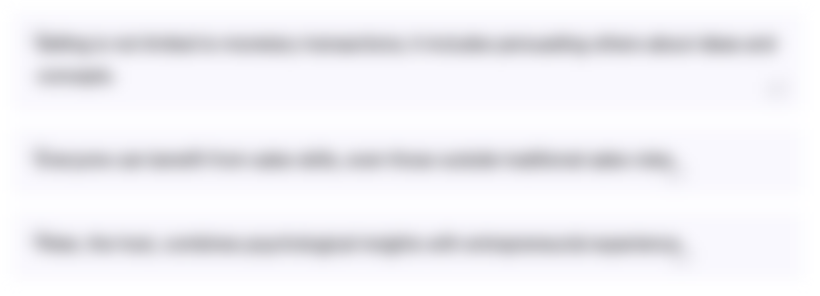
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
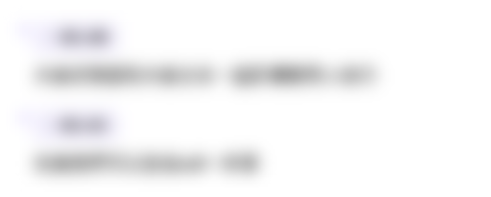
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)