TOPCIT Software | 07. Software Detail Design
Summary
TLDRThis script delves into the concept of software modularity, emphasizing its benefits like reduced complexity and improved reliability. It explores coupling and cohesion as measures of module independence and outlines structured design methods for creating control structures and refining them incrementally. The script also covers class design in object-oriented programming, detailing the transition from class diagrams to implementation, including operation signatures and associations. Lastly, it introduces software design patterns, categorizing them into creational, structural, and behavioral, highlighting their role in solving common software design issues.
Takeaways
- π§© Software Modularity: The script discusses the concept of software modularity, which involves breaking down software into functional modules to enhance independence, manageability, and efficiency.
- π Coupling and Cohesion: It explains the importance of low coupling and high cohesion for module independence, with various types of coupling and cohesion described to measure this independence.
- π οΈ Modular Benefits: The advantages of software modularity include reduced complexity, easier changes, efficient memory management, and improved reliability, leading to time and cost savings in development.
- ποΈ Design Time Considerations: Software developers must consider modularity during the design phase to ensure functional independence and effective development.
- π Cohesion and Coupling Details: The script delves into the specifics of different types of coupling (e.g., data, stamp, control) and cohesion (e.g., functional, sequential, logical) and their impact on module independence.
- π Structured Design Method: It outlines the steps of structured design, starting with control structures and data flow diagrams, to create a structured chart that defines the call order between functions.
- π Incremental Development: The script suggests an incremental approach to development and design review, allowing for the refinement of control structures and the improvement of code quality.
- π Class Design in OOD: The process of refining class diagrams in object-oriented design (OOD) by determining operation signatures, attributes, and visibility within classes is explained.
- π Association Implementation: The script covers how associations between classes in OOD are translated into reference attributes during the detailed design phase, with examples of one-to-many and many-to-many associations.
- π¨ Design Patterns: The concept of software design patterns as reusable solutions to common software design problems is introduced, with a distinction between creational, structural, and behavioral patterns.
- π Pattern Classification: It differentiates between class patterns, which are applied to classes and determined at compile time, and object patterns, which deal with runtime object relationships and are more dynamic.
Q & A
What is software modularity and why is it important?
-Software modularity refers to the practice of breaking down software into smaller, separate components called modules based on functionality. It is important because it reduces software complexity, making changes easier, facilitating program implementation, and improving reliability, which in turn saves development time, effort, and cost.
How is the independence of a module measured in software modularity?
-The independence of a module is measured by the degree of coupling and cohesion. Lower coupling indicates fewer dependencies on other modules, while higher cohesion means that the elements within a module are closely related to each other.
What are the default properties of a module in software modularity?
-The default properties of a module include IO elements for accepting and exporting data, functional elements for converting input to output, procedural codes for performing a function, and internal data elements that the module itself refers to.
What are the types of coupling in software modularity and which is the weakest?
-Types of coupling include data coupling, stamp coupling, control coupling, external coupling, common coupling, and content coupling. Data coupling is the weakest as it involves the least interdependence between modules.
What does cohesion in software modularity represent and what are its types?
-Cohesion represents the degree to which elements within a module are related to each other, indicating how well a module is defined as an independent function. Types of cohesion include functional, sequential, exchange, procedural, temporal, logical, and coincident cohesion, with functional cohesion being the strongest and coincident cohesion the weakest.
Can you explain the structured method in software detailed design?
-In the structured method of software detailed design, a control structure is designed to implement the processes of the data flow diagram assigned to each task and module. This involves creating a structure chart that defines the call order between functions and helps in identifying duplicated call relationships, leading to a more concise control structure.
How does incremental implementation and review in detailed design improve code quality?
-Incremental implementation and review allow developers to implement functionality in small, manageable pieces, review them through a structure chart, and then refine the code based on the improvements identified. This iterative process leads to higher quality code that reflects the refinements made.
What is the purpose of creating a structure chart in detailed design?
-The purpose of creating a structure chart is to visualize the relationships between functions, helping to identify duplicated call relationships and to ensure that derived functions have an appropriate size for implementation. It also aids in determining the specific control structure corresponding to the programming language used.
What is the role of class detailed design in object-oriented design?
-The role of class detailed design in object-oriented design is to refine the class diagram by determining the properties, operations, and associated visibility within the class. It involves defining exact operation signatures and attributes based on inputs such as class diagrams and sequence diagrams.
How are associations between classes implemented during the detailed design phase?
-Associations between classes are implemented by replacing them with reference attributes that match the selected object-oriented language. This transformation turns associations shown in the class diagram into reference attributes of the class, making them suitable for implementation.
What are software design patterns and how are they classified?
-Software design patterns are common, reusable solutions to problems that can arise in software design. They can be converted into source code and represent best practices for programmers. Design patterns are classified into creational, structural, and behavioral patterns, with further distinction between class patterns, which apply mainly to classes, and object patterns, which apply mainly to objects.
Outlines
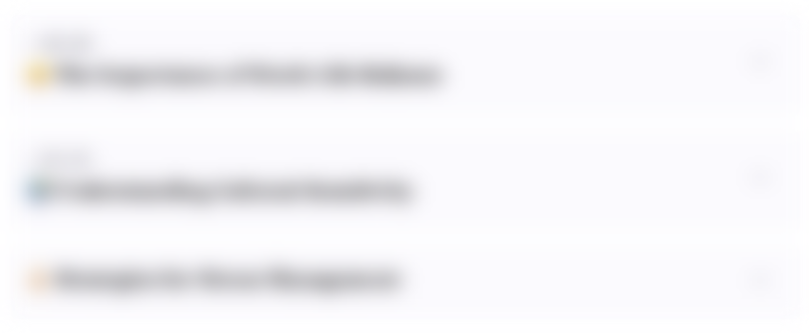
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
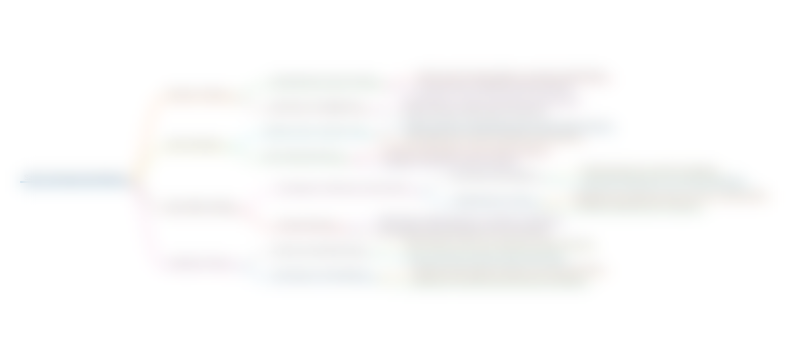
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
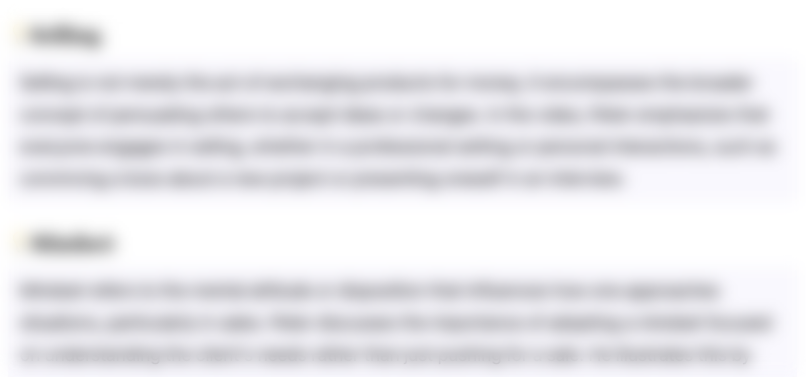
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
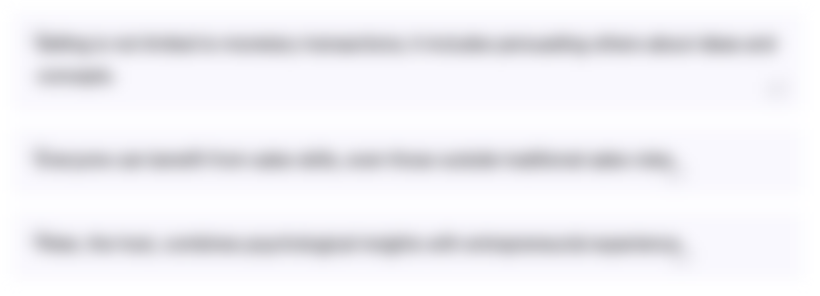
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
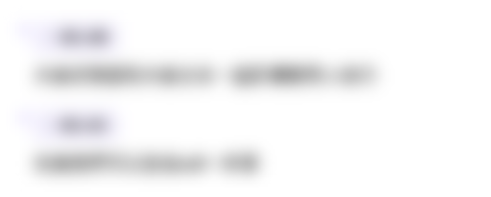
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
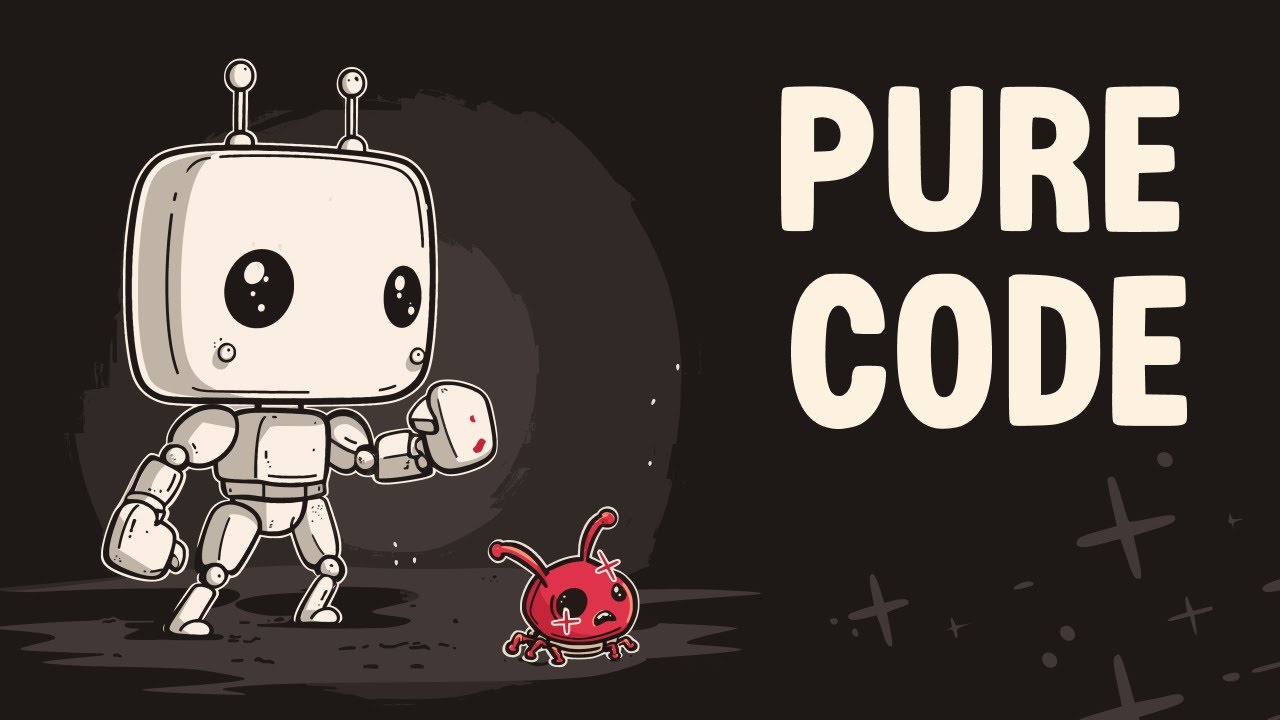
The purest coding style, where bugs are near impossible
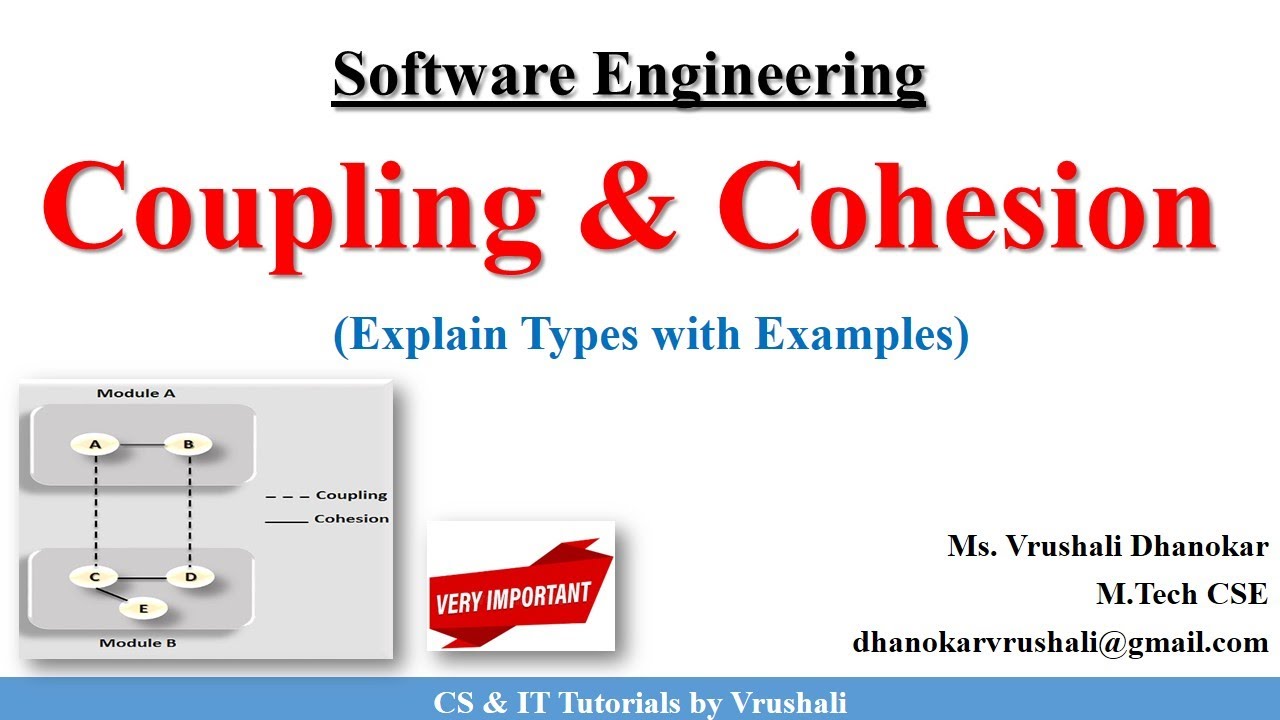
SE 23: Coupling & Cohesion with Examples | Software Engineering
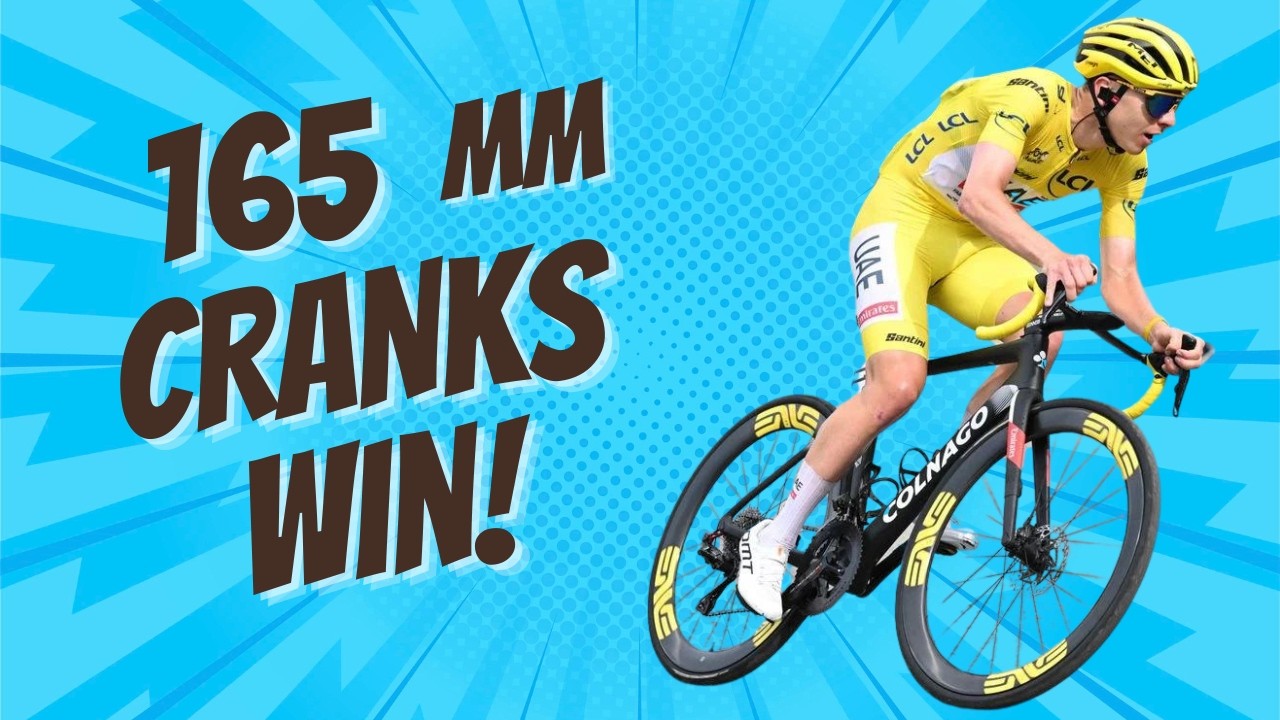
How Tadej Pogacar Won the Tour de France Riding 165mm Cranks
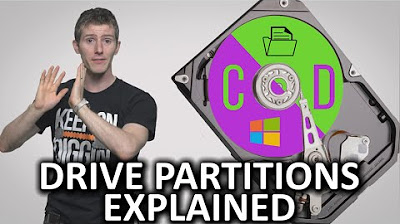
What are Drive Partitions?
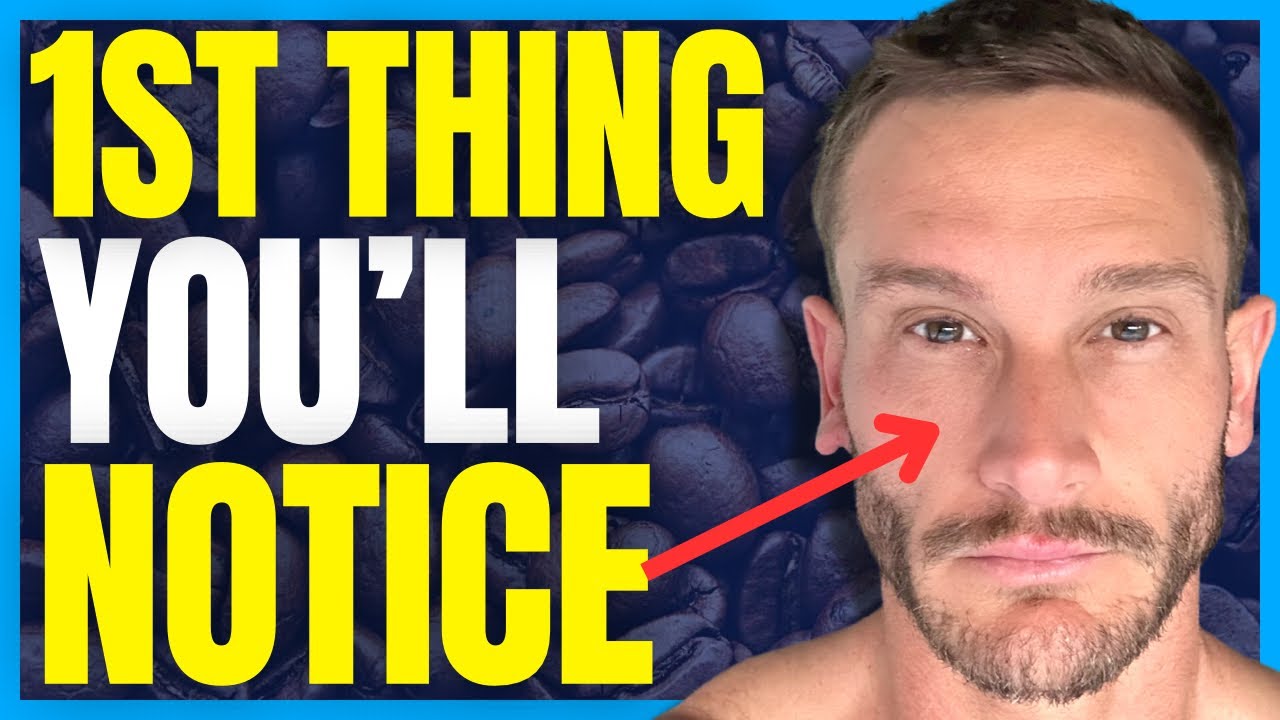
30 Days of NO CAFFEINE has Surprising Effects

Big Data In 5 Minutes | What Is Big Data?| Big Data Analytics | Big Data Tutorial | Simplilearn
5.0 / 5 (0 votes)