Modules in Python Explained | Python Built in Modules | Python Tutorial for Beginners | Simplilearn
Summary
TLDRThis session offers an in-depth explanation of Python modules, emphasizing the importance of reusability and efficient code management. The speaker covers what modules are, why they are useful, and how to import them in various ways. The session explores built-in modules like `math` and `random`, alongside user-defined modules, demonstrating examples such as addition, remainder calculation, and checking prime numbers. The speaker also highlights the advantages of modular coding for large projects, making debugging easier and organizing code logically. The session concludes with practical examples using Jupyter Notebook.
Takeaways
- 📂 Python modules enable code reusability by allowing developers to write a piece of code once and use it across different programs.
- 📄 A Python module is simply a file with a .py extension that contains definitions and statements that can be reused in various projects.
- 💡 Built-in modules (like `random`, `datetime`, `sys`) come with Python and don’t require explicit importing, while user-defined modules are created for specific projects.
- 🔀 Python modules help organize code logically, making it easier to maintain and debug, especially in large team settings.
- 🔧 User-defined modules allow developers to write complex functions and share them with others, reducing the need for rewriting code across projects.
- ⚙️ There are five main ways to import modules in Python: `import module_name`, `import module_name as alias`, `from module_name import specific_function`, `from module_name import specific_function as alias`, and `from module_name import *`.
- 📝 Example: A file named `calculations.py` can contain functions like `add()`, `multiply()`, `remainder()`, and can be imported using any of the five import methods.
- ✅ Python provides a built-in function `dir()` that displays all available functions and variables within a module.
- 📊 Python’s standard library includes useful in-built modules such as `math`, which provides mathematical functions, and `random`, which provides functionalities for generating random values.
- 🎯 Importing and using Python modules, whether built-in or user-defined, enhances code reusability, reduces duplication, and simplifies the coding process.
Q & A
What is a Python module?
-A Python module is a simple Python file with a `.py` extension that contains Python statements and definitions. It allows code to be reused across various programs by importing functions or classes.
Why should we use Python modules?
-Python modules enable reusability and organization of code. They help avoid rewriting code, make debugging easier, and allow different developers to work on various parts of a project while using shared utilities.
What are the two types of Python modules?
-There are two types of Python modules: built-in modules and user-defined modules. Built-in modules come with Python's standard library, like `random` and `datetime`. User-defined modules are created by users to address specific project needs.
How does importing a module help in a team environment?
-By using a shared module, common functions like utility methods or business rules are centralized. This allows different team members to use the same code without each developer needing to write or modify it, improving collaboration and consistency.
What are some of the ways to import Python modules?
-There are five main ways to import Python modules: (1) `import module_name`, (2) `import module_name as alias`, (3) `from module_name import specific_function_or_class`, (4) `from module_name import specific_function_or_class as alias`, and (5) `from module_name import *`.
How do built-in modules differ from user-defined modules?
-Built-in modules come pre-installed with Python and are part of the standard library, while user-defined modules are custom-created by developers for specific tasks and projects.
What is an example of a user-defined Python module?
-An example of a user-defined Python module is a file named `calculations.py` that contains functions like `add`, `multiply`, `divide`, and custom logic, such as checking for prime numbers or anagrams.
How can you list all functions and variables in a Python module?
-You can use Python's built-in `dir()` function to list all functions, variables, and other attributes defined in a module. For example, `dir(calculations)` will list all the functions in the `calculations.py` module.
What is the purpose of using an alias when importing modules?
-Using an alias shortens the module's name, making it easier and faster to reference within your code. For example, `import calculations as cal` allows you to use `cal.add()` instead of `calculations.add()`.
What are some commonly used built-in Python modules?
-Commonly used built-in Python modules include `math` (for mathematical functions), `datetime` (for date and time manipulation), and `random` (for generating random numbers or selections).
Outlines
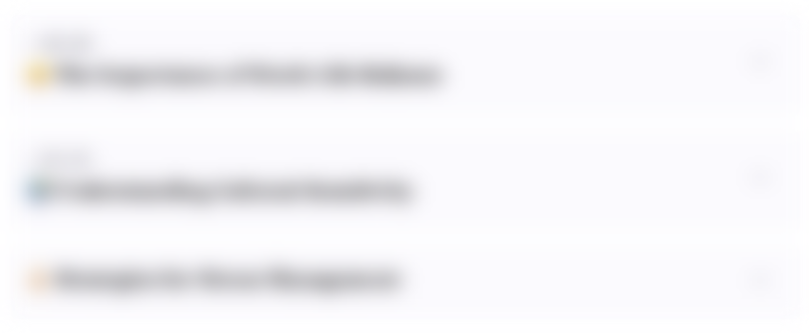
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифMindmap
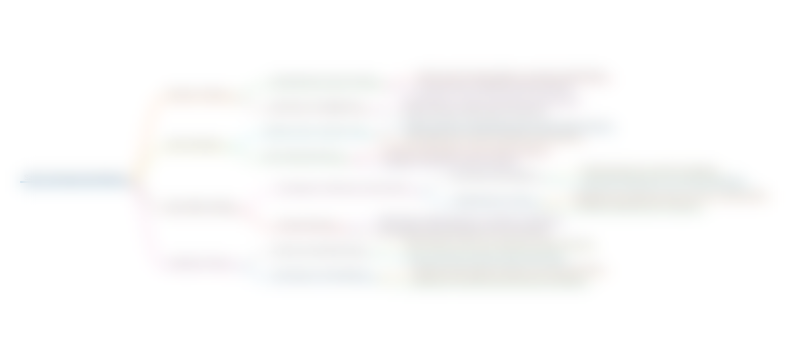
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифKeywords
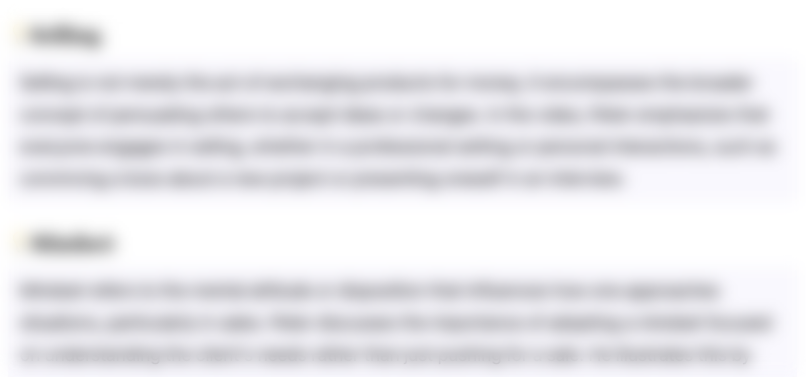
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифHighlights
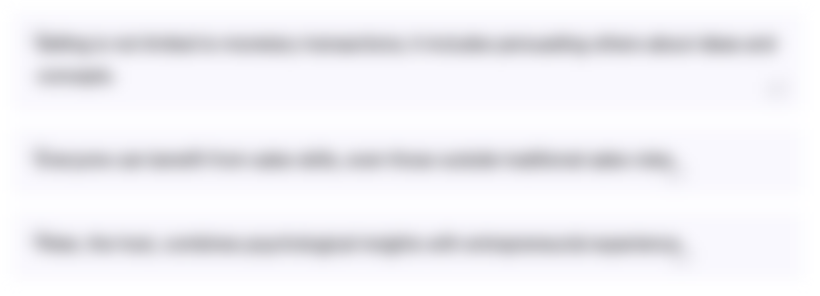
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифTranscripts
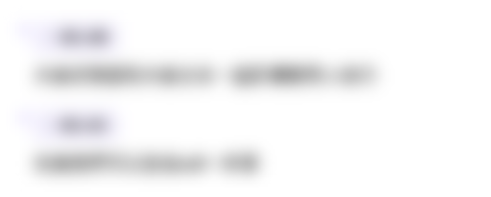
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тариф5.0 / 5 (0 votes)