Asynchronous JavaScript in ~10 Minutes - Callbacks, Promises, and Async/Await
Summary
TLDRIn this video, JamesQuick dives into the intricacies of asynchronous JavaScript, a fundamental yet challenging aspect of the language. He breaks down the concept into three main categories: callbacks, promises, and async/await. Starting with the basics of callbacks and their potential pitfalls, such as 'callback hell', he then introduces promises as an improvement over callbacks, demonstrating how they handle success and error cases. Finally, he presents async/await as the preferred method for writing asynchronous code, showcasing its readability and ease of use with examples like reading files and making API requests. The video aims to clarify these concepts, making them accessible for web developers looking to master asynchronous JavaScript.
Takeaways
- 😀 Asynchronous JavaScript is a fundamental concept in JavaScript that makes the language unique and is essential for day-to-day coding.
- 🔄 There are three main categories of asynchronous JavaScript: callbacks, promises, and async/await, each with its own approach to handling asynchronous operations.
- ⏱ Callbacks are functions passed into other functions to be executed later, often used in scenarios like `setTimeout` and event listeners, but can lead to 'callback hell' with nested functions.
- 📚 Error-first callbacks are a pattern where the first parameter of a callback function is reserved for an error object, allowing error handling within asynchronous operations.
- 🔄 Promises are an improvement over callbacks, providing a more manageable way to handle asynchronous operations with `.then()` for success and `.catch()` for errors.
- 🔄 `fs.promises` in Node.js and the Fetch API are examples of built-in modules that use promises to simplify file operations and HTTP requests respectively.
- 🆕 Async/await is a newer feature that simplifies writing asynchronous code by allowing the use of `await` to wait for a promise's resolution within an `async` function.
- 👍 Async/await is the preferred method for the script's author due to its readability and ease of handling errors with try/catch blocks.
- 🛑 Unhandled promise rejections can occur if the error case is not properly caught, highlighting the importance of comprehensive error handling in asynchronous code.
- 📚 The script provides practical examples of asynchronous JavaScript, including reading files, handling HTTP requests with the Fetch API, and dealing with errors.
- 🔧 Converting older callback-based code to promises and then to async/await is a way to modernize and improve the structure and readability of JavaScript code.
Q & A
What are the three main categories of asynchronous JavaScript discussed in the video?
-The three main categories of asynchronous JavaScript discussed in the video are callbacks, promises, and async/await.
Why is asynchronous JavaScript considered an important concept to learn?
-Asynchronous JavaScript is important because it is core to how JavaScript works, especially in day-to-day coding, and it makes JavaScript unique compared to other languages.
What is a callback and how is it used in JavaScript?
-A callback is a function passed into another function to be executed later. In JavaScript, callbacks are used in scenarios like setTimeout, where the callback function is executed after a specified delay.
Can you explain the concept of 'callback hell' mentioned in the video?
-Callback hell refers to the situation where multiple callbacks are nested within each other, leading to complex and hard-to-maintain code structures that resemble a 'Christmas tree' pattern.
How does the video demonstrate the use of callbacks with events in JavaScript?
-The video demonstrates the use of callbacks with events by showing an example of using `button.addEventListener` to listen for a click event and execute a callback function when the event occurs.
What is an 'error-first callback' and why is it significant?
-An 'error-first callback' is a callback pattern where the first parameter is reserved for an error object. It is significant because it allows developers to handle errors in asynchronous operations more effectively.
How does the video explain the evolution from callbacks to promises?
-The video explains that promises evolved from callbacks to provide a cleaner and more manageable way to handle asynchronous operations. Promises use the `resolve` and `reject` functions to handle success and failure paths, respectively.
What is the main advantage of using promises over callbacks in JavaScript?
-The main advantage of using promises over callbacks is that promises allow for better error handling with `.catch()` and make the code more readable and maintainable by avoiding deeply nested callback structures.
How does the 'async/await' syntax simplify writing asynchronous code in JavaScript?
-The 'async/await' syntax simplifies asynchronous code by allowing developers to write code that looks and behaves more like synchronous code. It uses the `await` keyword to pause the execution of an async function until the promise is resolved or rejected.
What is the purpose of the 'try/catch' block when using async/await?
-The 'try/catch' block is used with async/await to handle any errors that occur during the execution of the asynchronous operations. It allows for error handling similar to synchronous code.
Can you provide an example of how the video uses async/await with the fetch API?
-The video demonstrates using async/await with the fetch API by defining an async function that awaits the fetch request, awaits the JSON response, and then processes the data. If an error occurs, it is caught and handled in a try/catch block.
Outlines
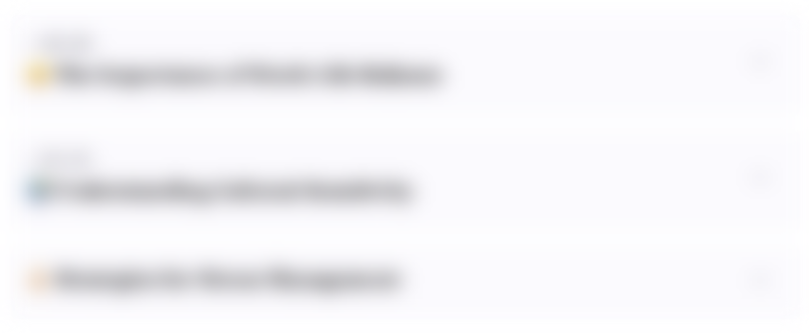
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードMindmap
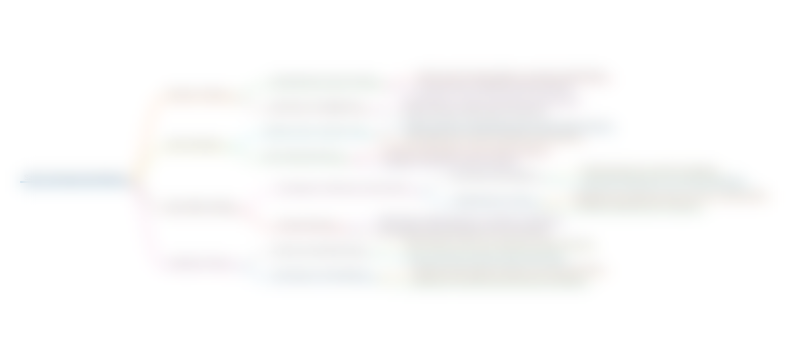
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードKeywords
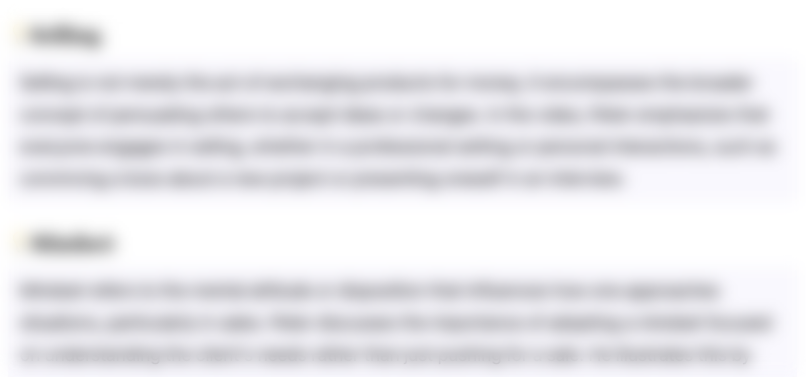
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードHighlights
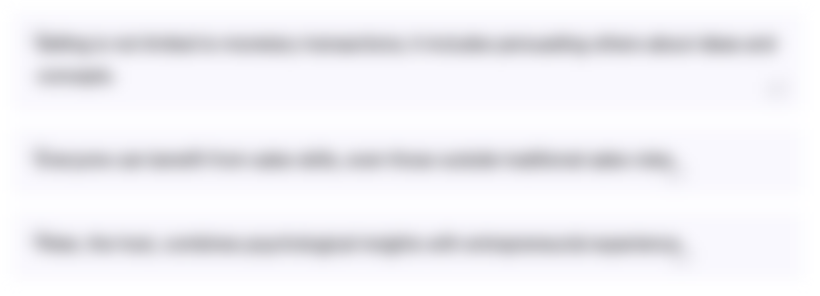
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードTranscripts
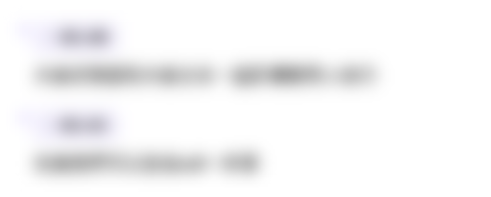
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレード関連動画をさらに表示
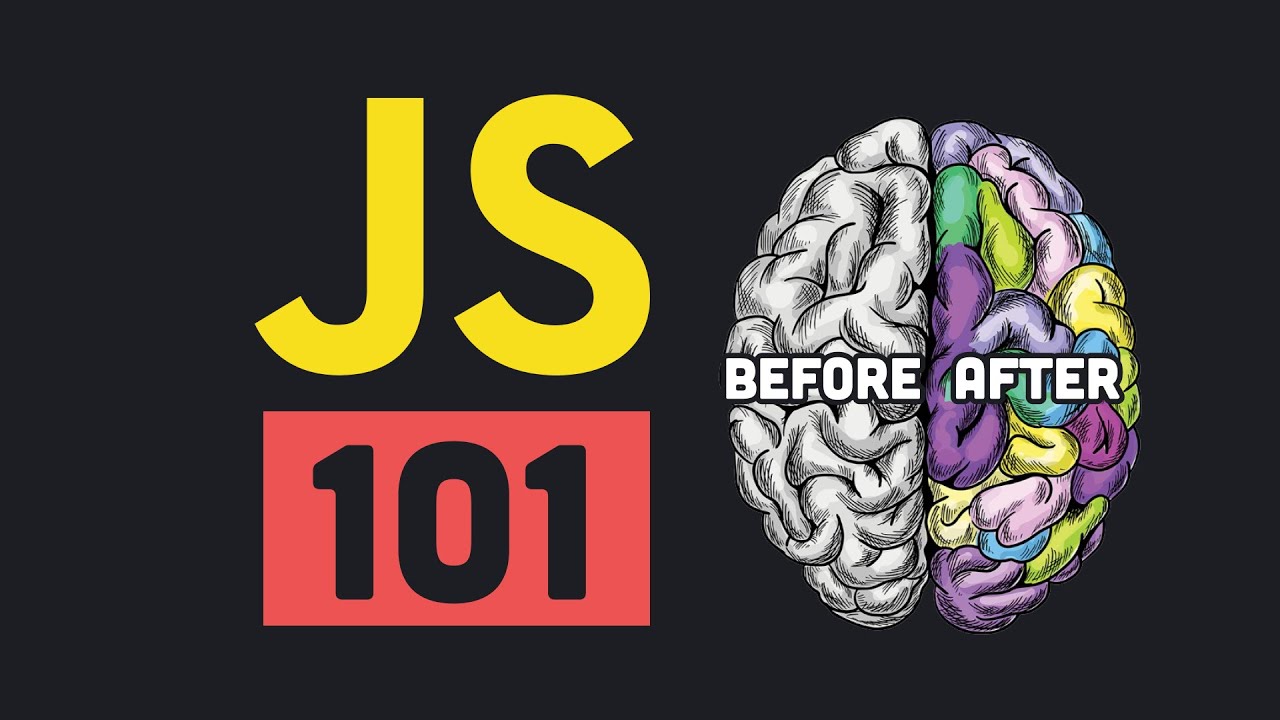
100+ JavaScript Concepts you Need to Know

What the heck is the event loop anyway? | Philip Roberts | JSConf EU

How JavaScript Works 🔥& Execution Context | Namaste JavaScript Ep.1

Aula: Imunologia - Sistema Complemento | Imunologia #8
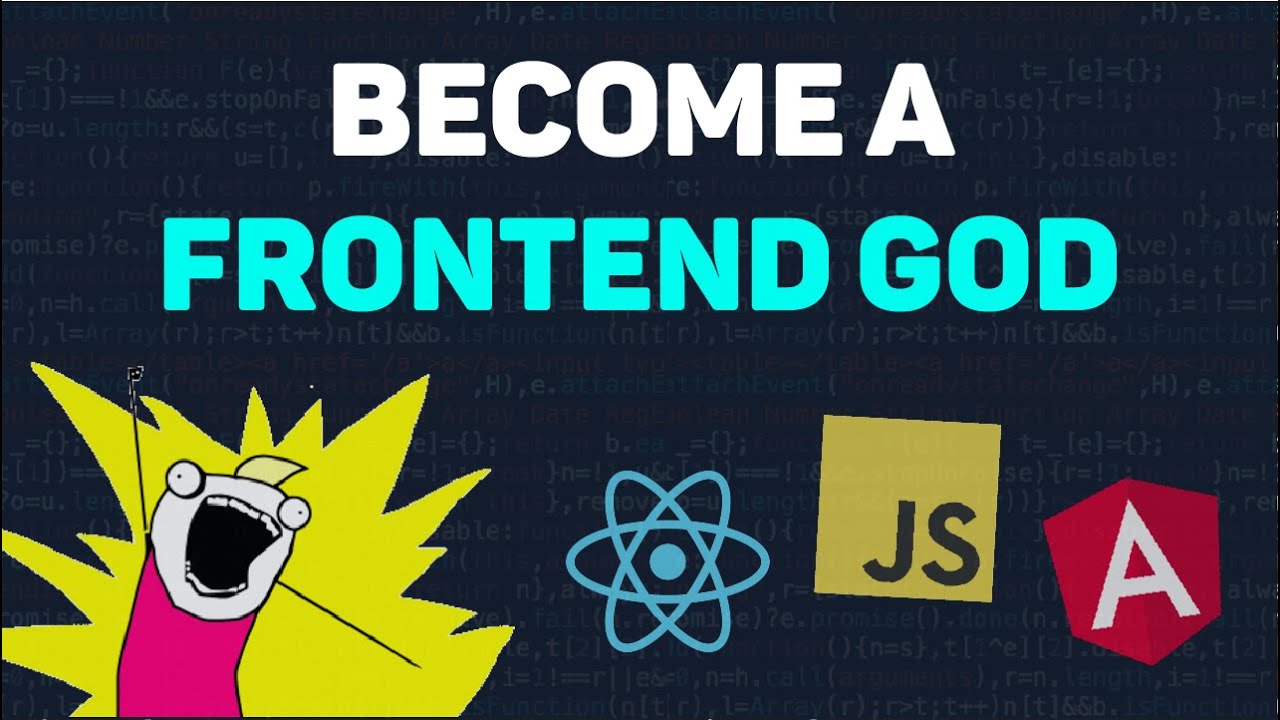
No-Nonsense Frontend Engineering Roadmap

[Introduction to Linguistics] Phonological Features
5.0 / 5 (0 votes)