Master Go Programming With These Concurrency Patterns (in 40 minutes)
Summary
TLDRThis video explores the fundamentals of concurrency in Go, covering key concepts such as unbuffered and buffered channels, goroutines, and the select statement. The speaker explains how to use channels to enable synchronization between concurrent tasks, prevents goroutine leaks using the done channel, and demonstrates building a simple pipeline to process data across multiple stages. The video offers practical examples of concurrency patterns, highlighting Go's ability to manage complex, concurrent operations efficiently with minimal risk of resource issues.
Takeaways
- 😀 Unbuffered channels in Go provide synchronous communication, blocking the sending goroutine until the data is received.
- 😀 Buffered channels allow for asynchronous communication, enabling the sender to continue even if the receiver hasn't picked up the data yet, as long as the buffer isn't full.
- 😀 The `select` statement allows a goroutine to wait on multiple channels and react when one is ready, making it essential for managing concurrent operations.
- 😀 The `done` channel is used for gracefully stopping goroutines by sending a signal to indicate when they should terminate.
- 😀 Proper management of goroutines and channels is crucial to prevent goroutine leaks, ensuring efficient resource usage.
- 😀 The pipeline pattern in Go allows for processing data in multiple stages, where each stage performs an operation and passes the result to the next.
- 😀 Channels are used to communicate data between goroutines in a pipeline, ensuring that each stage is completed before moving on to the next.
- 😀 In a pipeline, each stage is typically implemented as a goroutine, allowing for concurrent processing of data.
- 😀 The use of unbuffered channels in a pipeline guarantees synchronous communication, which helps maintain the correct order of operations.
- 😀 The pipeline example demonstrates how Go routines can square a slice of integers, with each stage performing a different task (converting, squaring, and printing).
Q & A
What is the purpose of channels in Go?
-Channels in Go are used for communication between goroutines, enabling them to synchronize their execution and share data safely. They can be either buffered or unbuffered, determining the synchronization behavior of the communication.
What is the difference between buffered and unbuffered channels?
-Unbuffered channels synchronize the sender and receiver. The sender blocks until the receiver reads the data. Buffered channels allow asynchronous communication, where the sender can continue if there is space in the buffer; if full, it blocks until space is available.
What role does the select statement play in Go concurrency?
-The select statement in Go allows a goroutine to wait on multiple channel operations simultaneously. It blocks until one of the channels is ready, making it useful for handling multiple channels or timeouts. It can also include a 'default' case to perform other work when no channels are ready.
What is a Done channel and why is it important?
-A Done channel is used to signal the cancellation or termination of a goroutine. It allows a parent goroutine to signal its child goroutines to stop, preventing resource leaks and managing long-running or indefinite tasks effectively.
What is a 'goroutine leak'?
-A goroutine leak happens when a goroutine is not terminated as expected, causing it to continue running indefinitely and consuming resources. This can occur if the Done channel is not properly handled or closed.
How does Go handle synchronization in a pipeline pattern?
-Go handles synchronization in the pipeline pattern using channels. Each stage in the pipeline is a separate goroutine, and channels pass data between these stages. The communication between stages is typically synchronous, ensuring that data flows in the correct order and is processed properly.
What are the advantages of using Go routines in a pipeline pattern?
-Go routines in a pipeline pattern allow for concurrent processing of data, improving performance and scalability. Each stage can run in parallel, and the channels provide the synchronization needed to pass data through each stage without issues.
Why is the order of processing important in Go's pipeline pattern?
-The order of processing is crucial because it ensures that the data is processed correctly in a sequence of stages. In the given example, the squared value of each number is printed in the same order as the original slice, maintaining the expected output.
How does the pipeline example in the transcript work in practice?
-In the pipeline example, an integer slice is passed through multiple stages. Each stage is handled by a goroutine that processes the data and passes it to the next stage via channels. In the example, the data is squared, and the results are printed in order, demonstrating how data flows through the pipeline.
How do Go routines interact with channels in the provided example?
-In the example, Go routines interact with channels by sending and receiving data. The data is sent to the channel from one goroutine, processed by another, and passed to subsequent stages. The channels ensure that the operations are synchronized, maintaining the correct flow of data through the pipeline.
Outlines
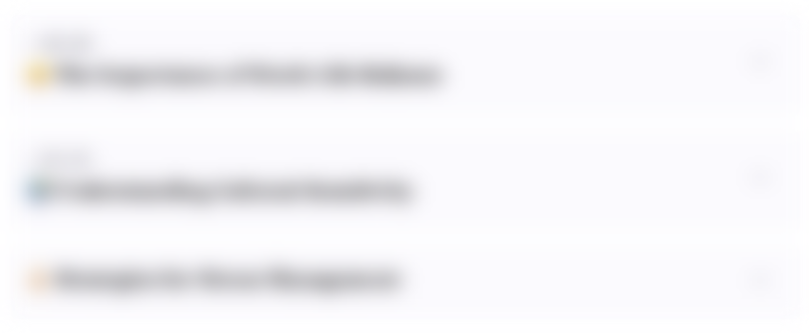
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードMindmap
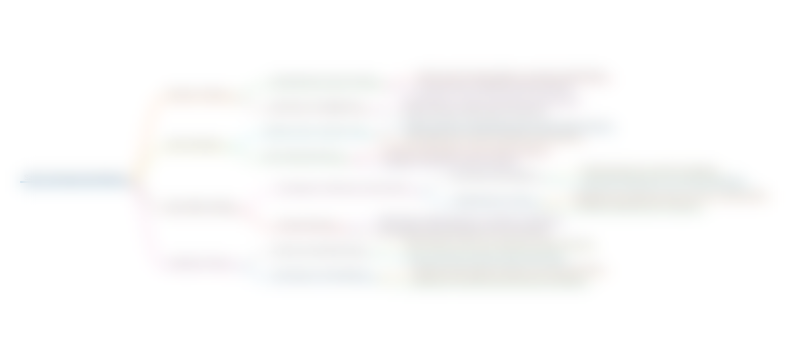
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードKeywords
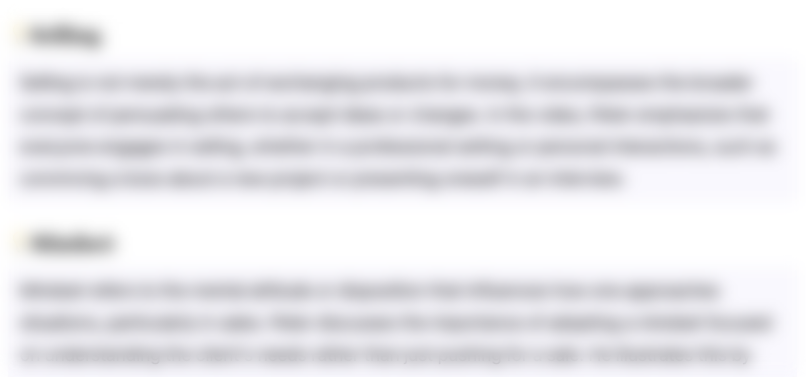
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードHighlights
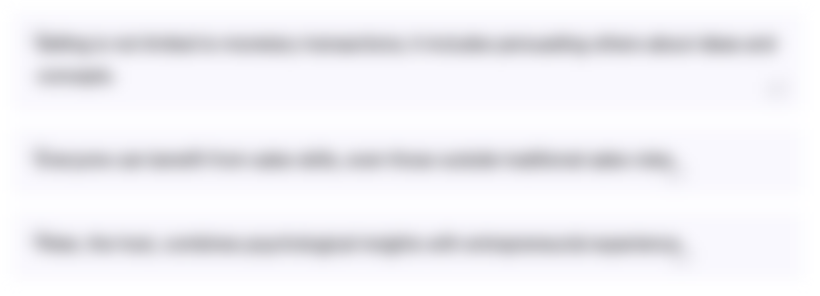
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードTranscripts
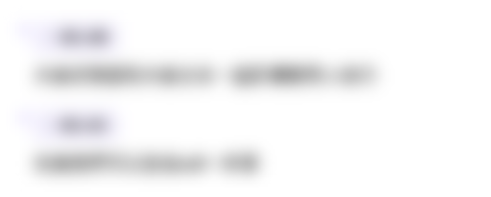
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレード5.0 / 5 (0 votes)