Testing Entity Framework Core Correctly in .NET
Summary
TLDRIn this video, Nick discusses the common mistake of using an in-memory database for integration tests with EF Core, which can lead to unreliable tests and missed critical application flow issues. He demonstrates a simple API example and shows how replacing the real database provider with an in-memory version during testing can be problematic. Nick then presents a solution using test containers to ensure tests are run against a real database, maintaining the integrity of the integration testing process. The video also promotes a new course on messaging in .NET with MassTransit, highlighting its importance for developers.
Takeaways
- 🚫 Avoid using the in-memory provider for integration tests with EF Core as it does not accurately test the database integration.
- 🔍 Integration tests should test the critical component of the application's flow, including the conversion of the DbContext code to the database queries.
- 💡 The in-memory provider can lead to flaky tests that do not reflect the real-world failures of the application.
- 🛠️ Demonstrated a simple customers API with integration tests, showing how to properly set up and run tests.
- 🔗 Highlighted the importance of injecting the app DbContext into services to ensure that the database context is tested correctly.
- 📝 Discussed two schools of thought on validating tests: validating API responses and validating database objects directly.
- 🔄 Showed how to override the configure web host in tests to replace the real database provider with the in-memory provider.
- 📚 Provided a step-by-step guide on how to add the in-memory package and configure services to use it for testing.
- 🚀 Introduced Testcontainers as a solution to use the real database in integration tests by running a containerized version of the database.
- 🛑 Explained how to configure Testcontainers to start and stop a database container asynchronously for each test run.
- 🔄 Showed how to pass the connection string from the Testcontainers database to the application for real database testing in CI/CD pipelines.
Q & A
What is the single biggest mistake people make when writing integration tests for an application using EF Core?
-The biggest mistake is replacing the real database provider with the in-memory version during testing. This can lead to flaky tests that do not accurately represent the application's behavior with the actual database.
Why are integration tests that use the in-memory provider not considered good tests?
-They are not good because they remove the critical component of the application's data flow, which includes the conversion of the DbContext code and query generations, leading to tests that do not fail in the same way the application would in real scenarios.
What is the purpose of the video presented by Nick?
-The purpose of the video is to explain the common mistake made in EF Core integration testing and to show how to solve the problem by using real databases with the help of test containers.
What is the role of the DbContext in the integration tests discussed in the video?
-The DbContext is a critical component that needs to be tested as it is responsible for the conversion of the application's data flow to what the database accepts. Testing it with an in-memory provider does not accurately reflect its behavior with a real database.
How does Nick demonstrate the mistake in the integration tests?
-Nick demonstrates the mistake by showing how developers replace the real database provider with an in-memory provider in their integration tests, which leads to unreliable tests.
What is the alternative solution to using an in-memory provider for integration tests presented in the video?
-The alternative solution is to use test containers to run integration tests against a real database, ensuring that the tests are more reliable and accurately represent the application's behavior.
What is the significance of using the same database as the production environment in integration tests?
-Using the same database ensures that the tests are testing the actual integration points of the application, including query generations and database interactions, which are crucial for identifying potential issues before deployment.
How does Nick use test containers to run integration tests with a real database?
-Nick uses test containers by installing a Postgres container and configuring it to work with the application's DbContext. This allows the integration tests to run against a real database environment.
What is the benefit of using Docker containers in the CI pipeline for integration tests?
-Docker containers in the CI pipeline ensure that the integration tests run in a consistent and isolated environment, similar to production, which helps in identifying issues early in the development cycle.
What is the course being promoted in the video, and what does it cover?
-The course being promoted is 'From 0 to Hero Messaging in .NET with MassTransit'. It covers advanced concepts and production-ready patterns for messaging in .NET, including the Outbox pattern and the Saga pattern, using MassTransit, a popular library for messaging in .NET applications.
How does Nick ensure that the integration tests are not just functional tests but also test the integration points?
-Nick ensures this by using test containers to run the tests against a real database, which includes the actual database interactions and query generations, making the tests more meaningful and representative of the application's behavior.
Outlines
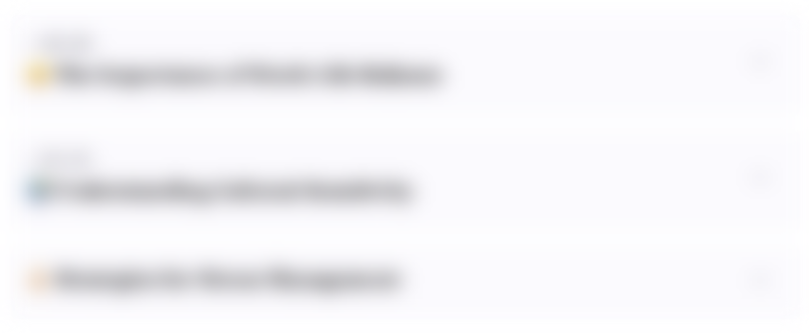
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードMindmap
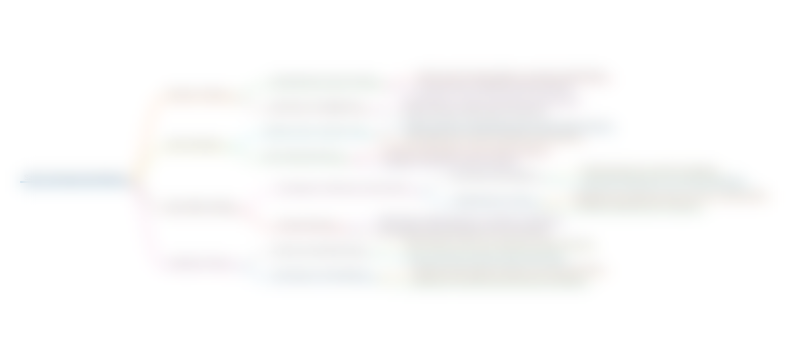
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードKeywords
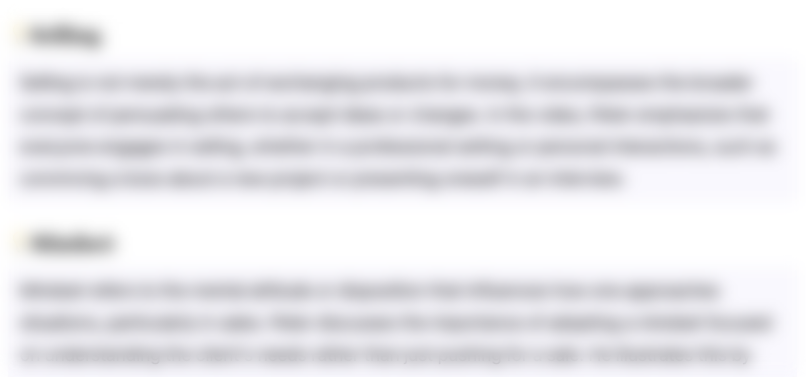
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードHighlights
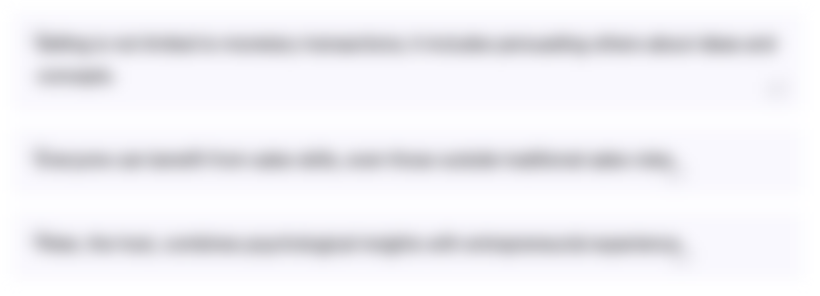
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードTranscripts
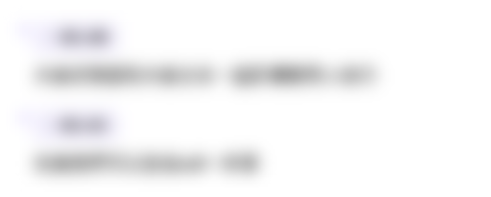
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレード関連動画をさらに表示
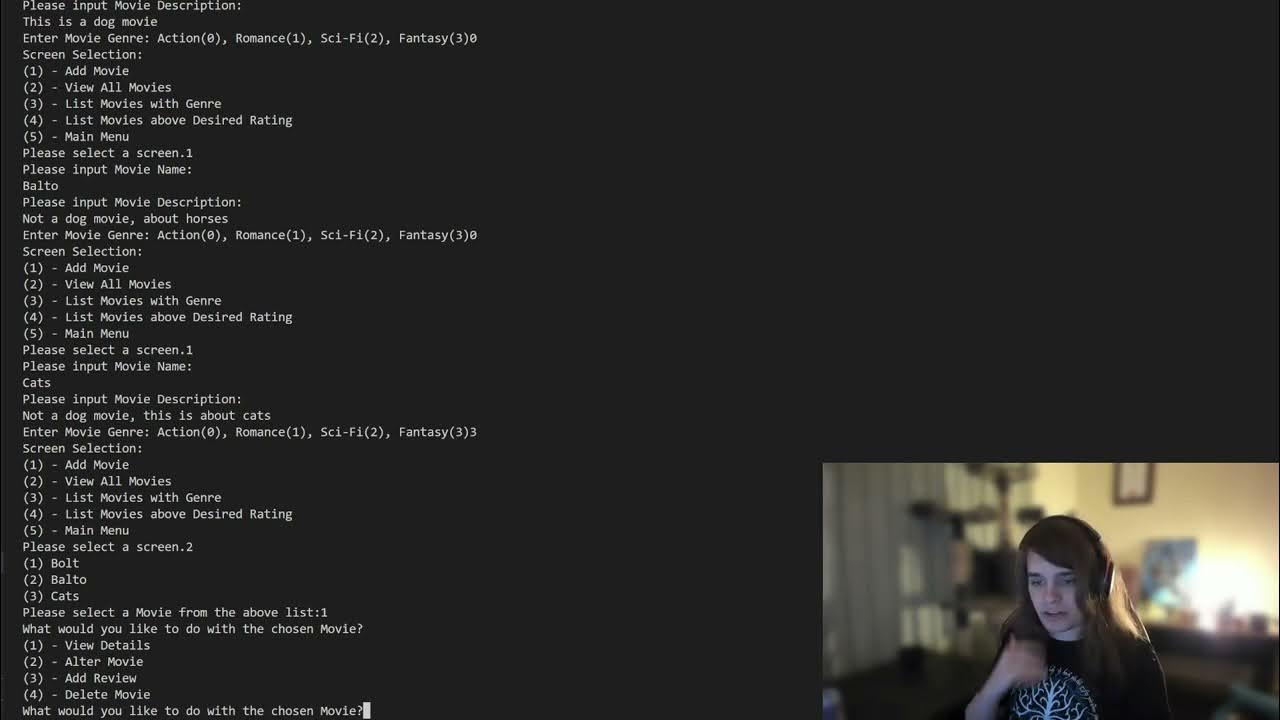
Film and TV Show Database - Project - Test Your Knowledge
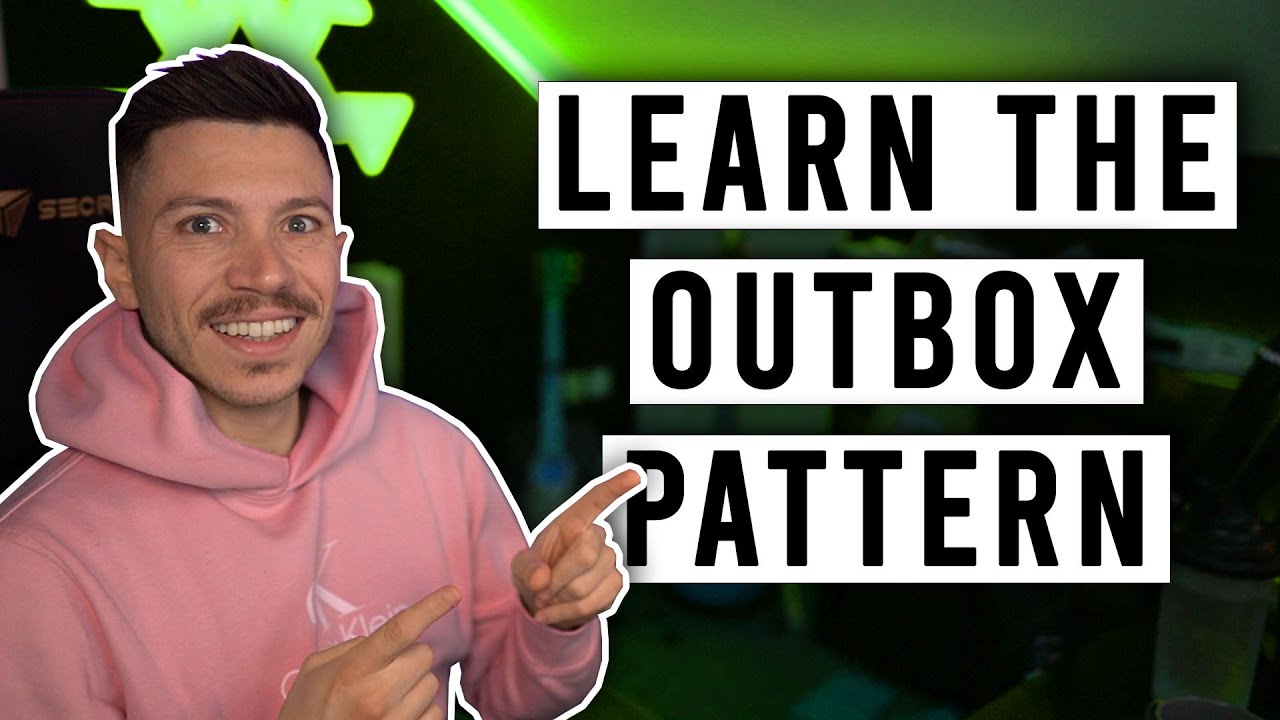
The Pattern You MUST Learn in .NET

GPT-4 Makes Old ChatGPT Look Like a JOKE!
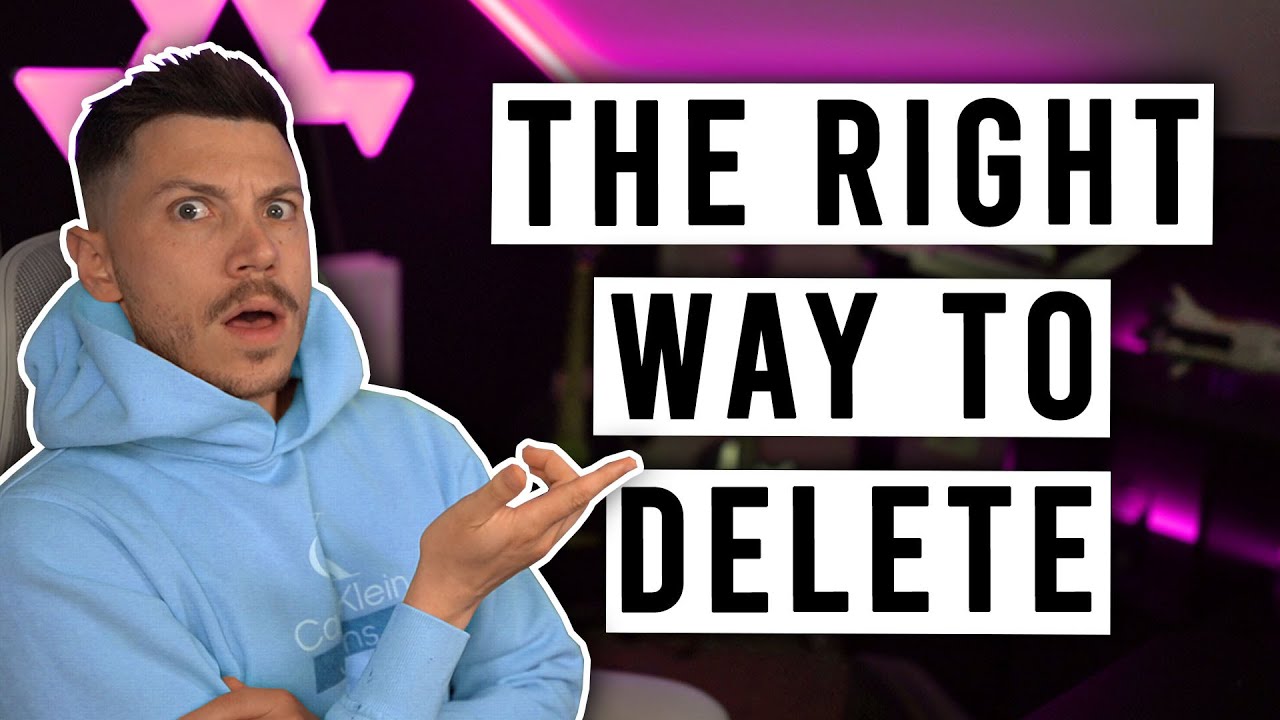
The Alternative to Deleting Data in .NET
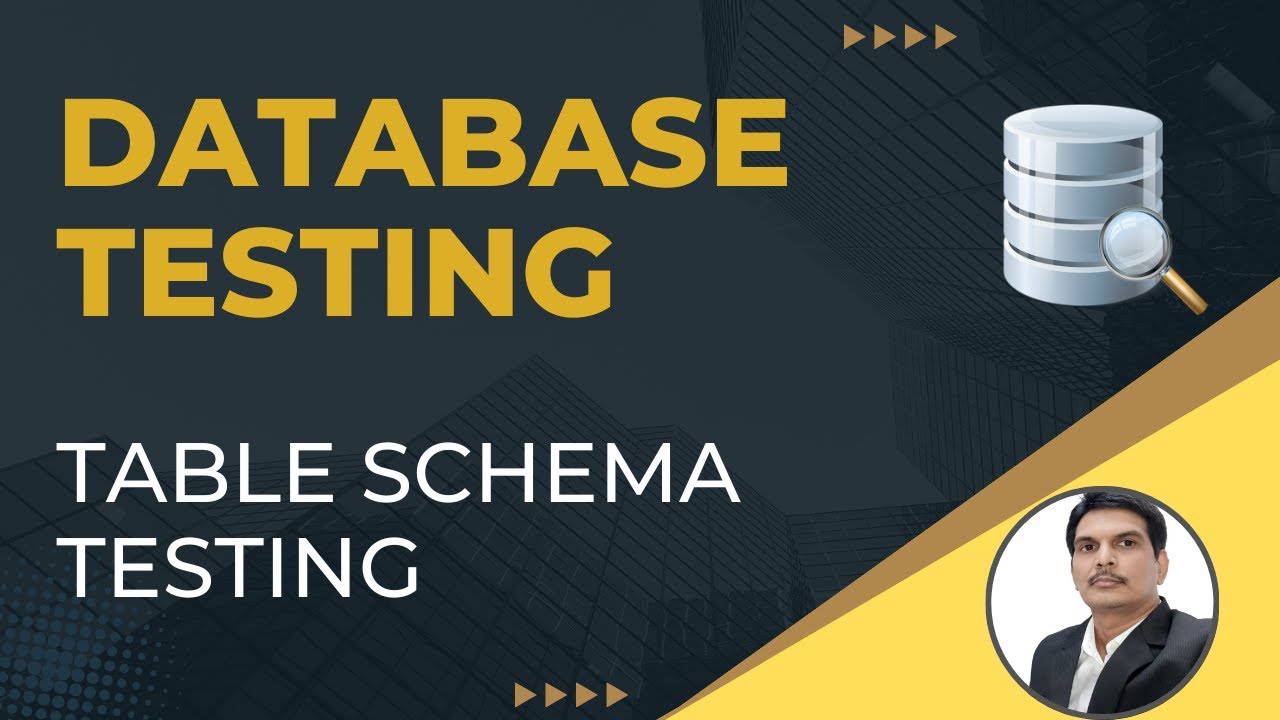
Part3 : Database Testing | How To Test Schema of Database Table | Test Cases
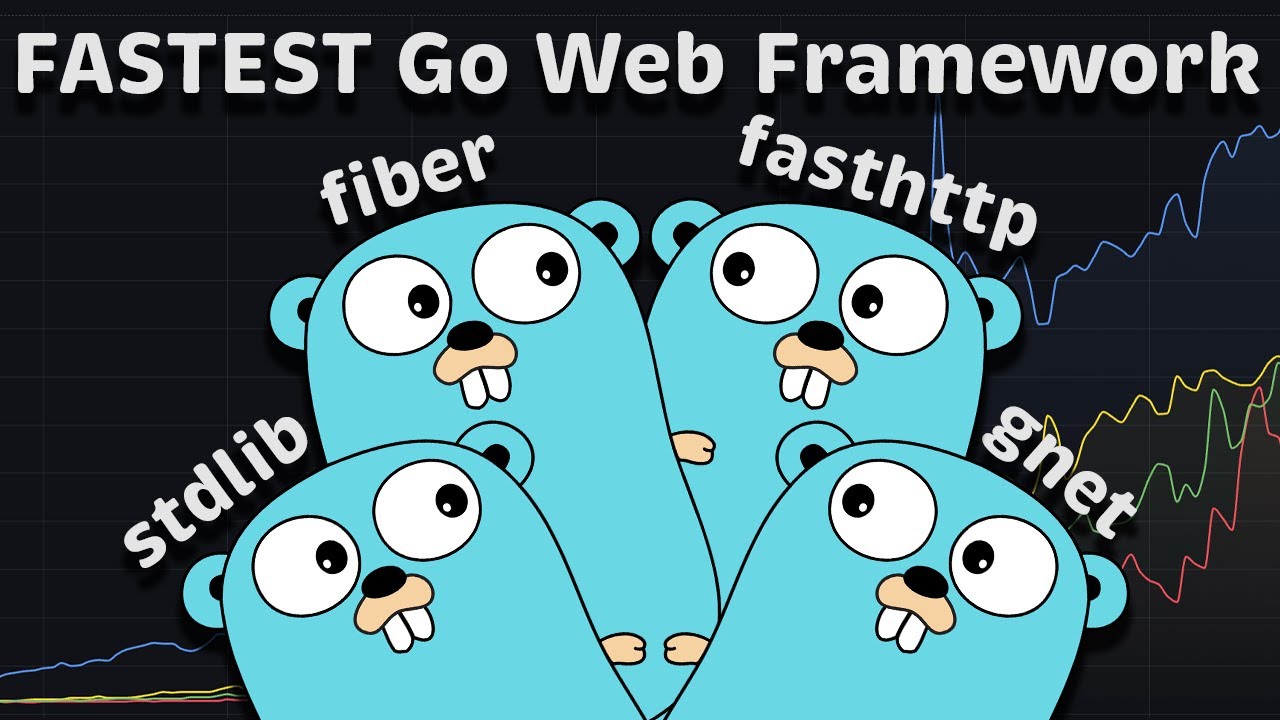
FASTEST Go Web Framework: gnet vs fiber vs fasthttp vs net/http?
5.0 / 5 (0 votes)