Operators in Python | Bitwise Operators | Python Tutorials for Beginners #lec17
Summary
TLDRIn this video, we explore bitwise operators in Python, covering the AND, OR, XOR, NOT, left shift, and right shift operators. Each operator is explained with simple, practical examples, showing how they manipulate binary numbers at the bit level. Viewers are introduced to concepts such as binary representation, two's complement, and how bitwise operations are performed on integers. The video also includes interactive exercises to encourage self-practice and understanding. By the end, viewers will have a solid grasp of bitwise operations and their applications in Python programming.
Takeaways
- 😀 Bitwise operators in Python work directly on the binary representation of numbers.
- 😀 The main bitwise operators in Python are AND (&), OR (|), XOR (^), NOT (~), left shift (<<), and right shift (>>).
- 😀 Bitwise AND (&) returns 1 only if both corresponding bits are 1; otherwise, it returns 0.
- 😀 Bitwise OR (|) returns 1 if at least one corresponding bit is 1.
- 😀 Bitwise XOR (^) returns 1 if the corresponding bits are different, otherwise, it returns 0.
- 😀 Bitwise NOT (~) inverts all bits of a number, and in Python, it uses two's complement to represent negative numbers.
- 😀 Left shift (<<) shifts bits to the left, multiplying the number by 2 raised to the power of the shift count.
- 😀 Right shift (>>) shifts bits to the right, dividing the number by 2 raised to the power of the shift count.
- 😀 For bitwise NOT (~), Python uses two's complement representation, which may lead to negative results.
- 😀 A good approach to practicing bitwise operations is to predict the result before testing it in Python, to better understand how bits are manipulated.
Q & A
What are bitwise operators and how do they work in Python?
-Bitwise operators in Python work directly on the bits of binary numbers. They perform operations like AND, OR, XOR, and NOT at the bit level. For example, bitwise AND (`&`) compares corresponding bits of two numbers and returns 1 only if both bits are 1, otherwise it returns 0.
What is the difference between the bitwise AND and bitwise OR operators?
-The bitwise AND operator (`&`) returns 1 only when both bits being compared are 1. The bitwise OR operator (`|`) returns 1 if at least one of the bits is 1. So, `5 & 4` would return `4` (binary `0100`), whereas `5 | 4` would return `5` (binary `0101`).
How does the bitwise XOR operator work?
-The bitwise XOR operator (`^`) compares two bits and returns 1 if the bits are different, and 0 if they are the same. For example, `5 ^ 4` would return `1` because in binary, `0101` (5) and `0100` (4) differ at the last bit.
What is the bitwise NOT operator and how does it work?
-The bitwise NOT operator (`~`) inverts each bit of a number. It flips all the bits, changing 0s to 1s and vice versa. For example, `~5` returns `-6` because the binary complement of `5` results in `-6` due to how negative numbers are stored in two’s complement representation.
What is the significance of two's complement in the bitwise NOT operation?
-Two's complement is used to represent negative numbers in binary. When you apply the bitwise NOT operator to a number, it inverts the bits and then adds 1 to the result. This is how negative numbers like `-6` are represented in memory.
How does the left shift operator (`<<`) work in Python?
-The left shift operator (`<<`) shifts the bits of a number to the left by a specified number of positions. Zeros are added on the right side. For example, `5 << 2` shifts the bits of `5` (binary `0101`) to the left by 2 positions, resulting in `20` (binary `10100`).
What happens when we use the right shift operator (`>>`) in Python?
-The right shift operator (`>>`) shifts the bits of a number to the right by the specified number of positions, discarding the rightmost bits and filling the leftmost positions with zeros. For example, `5 >> 2` shifts `0101` to the right by 2, resulting in `1` (binary `0001`).
What is the formula for calculating the result of a left shift operation?
-The formula for a left shift operation is: `X << n` results in `X * (2^n)`, where `X` is the number being shifted and `n` is the number of positions shifted. For example, `5 << 2` results in `5 * 2^2 = 20`.
Can bitwise operations be performed on floating point numbers in Python?
-No, bitwise operations in Python are only valid for integer values. They operate directly on the binary representation of integers, so attempting to perform bitwise operations on floating-point numbers will result in an error.
How can bitwise operators be useful in programming beyond simple calculations?
-Bitwise operators are useful for low-level programming tasks such as manipulating individual bits in flags, encryption algorithms, optimizing performance (e.g., using shifts for multiplication or division by powers of 2), and working with data structures that require precise control over binary representations.
Outlines
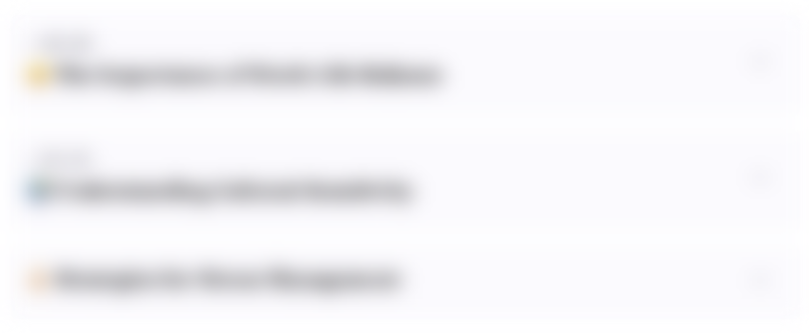
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードMindmap
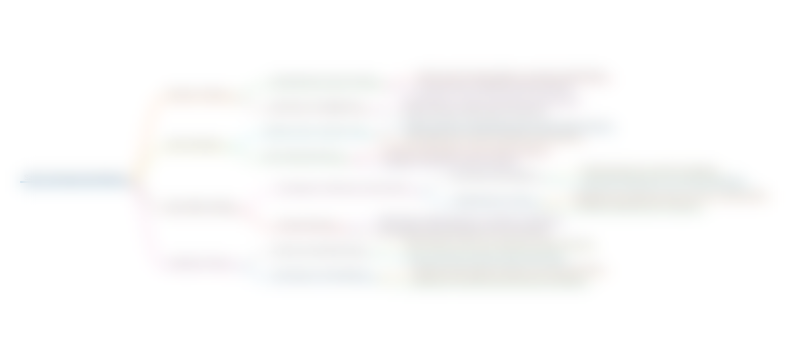
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードKeywords
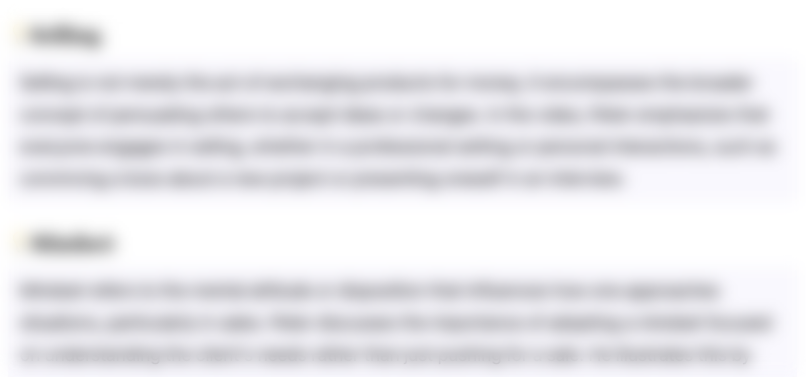
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードHighlights
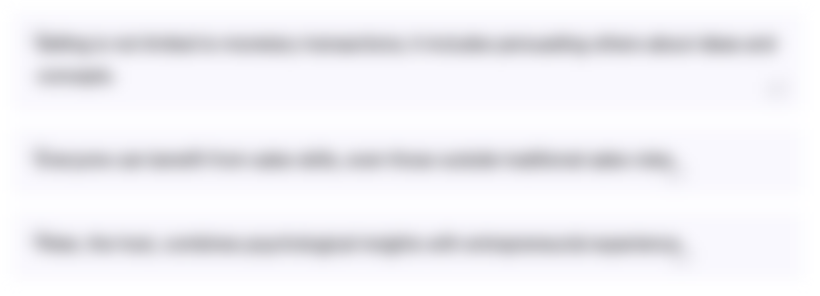
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードTranscripts
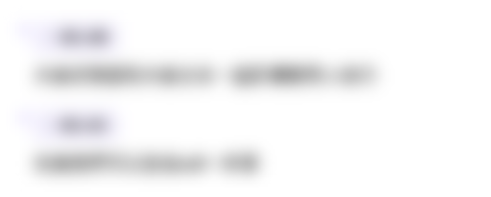
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレード関連動画をさらに表示
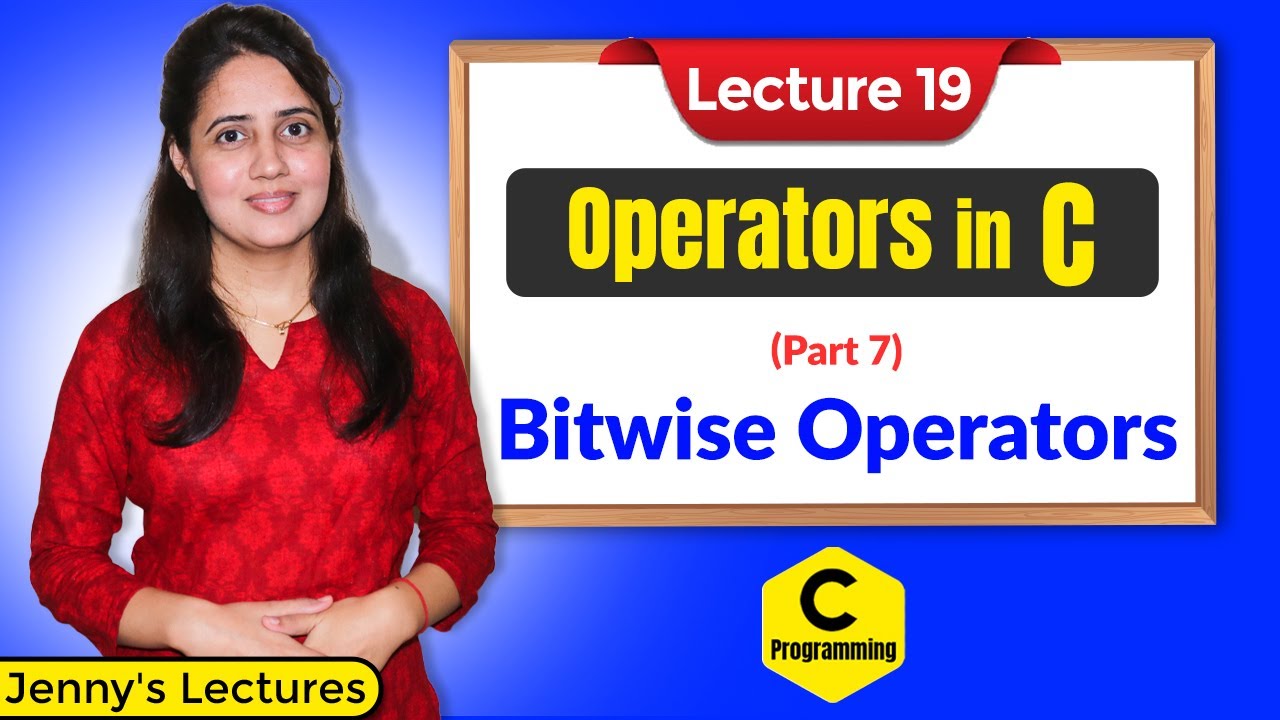
C_19 Operators in C - Part 7 (Bitwise Operators-II) | C Programming Tutorials
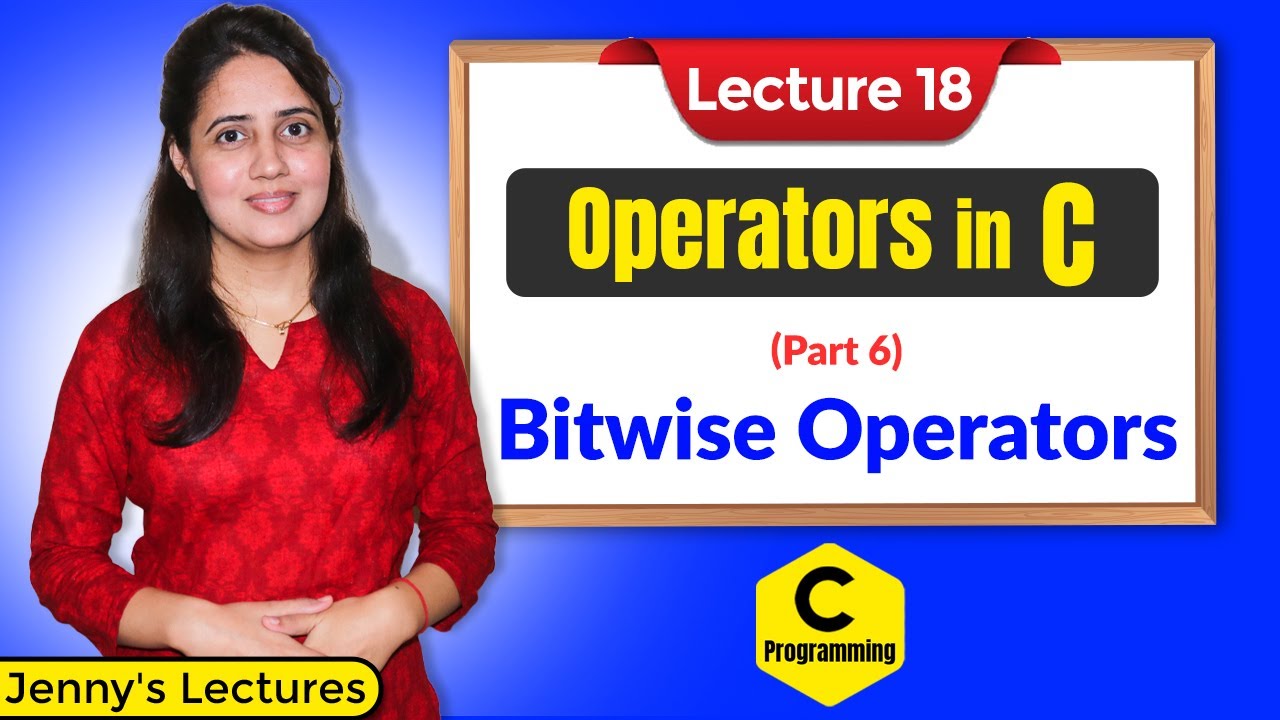
C_18 Operators in C - Part 6 | Bitwise Operators | C Programming Tutorials
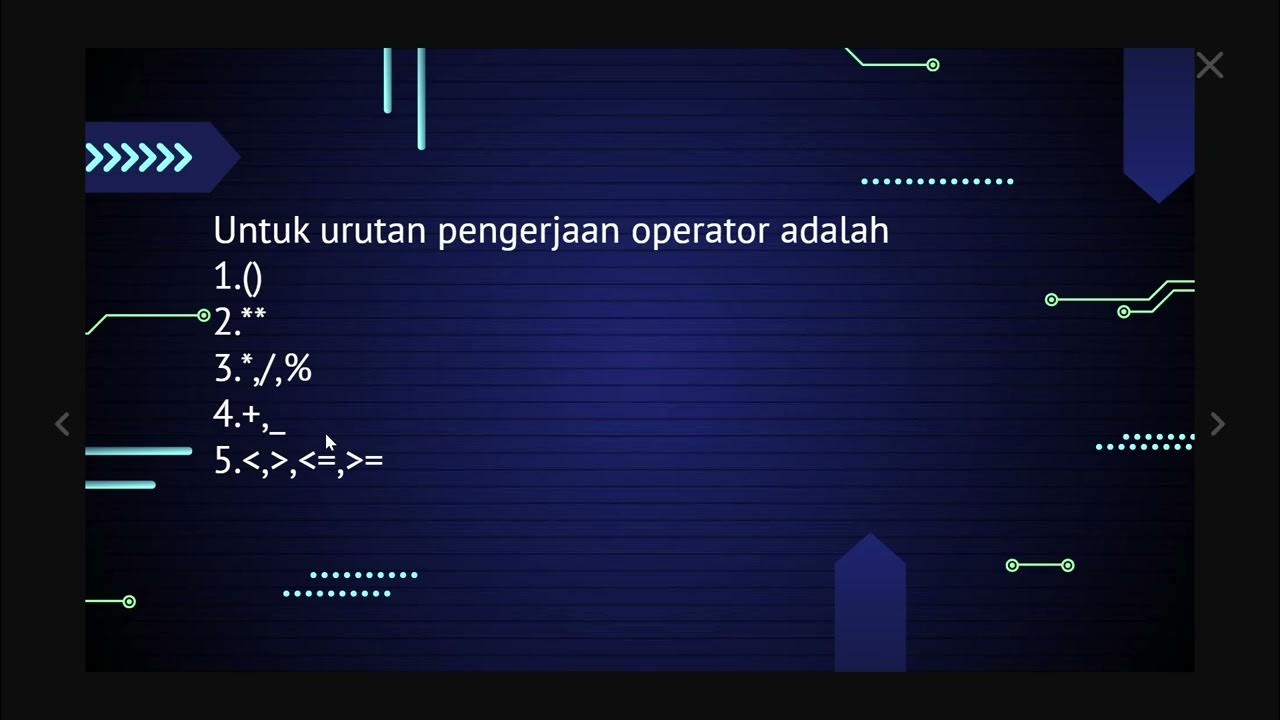
ALGORITMA dan PEMROGRAMAN || OPERATOR

Comparison, Logical, and Membership Operators in Python | Python for Beginners
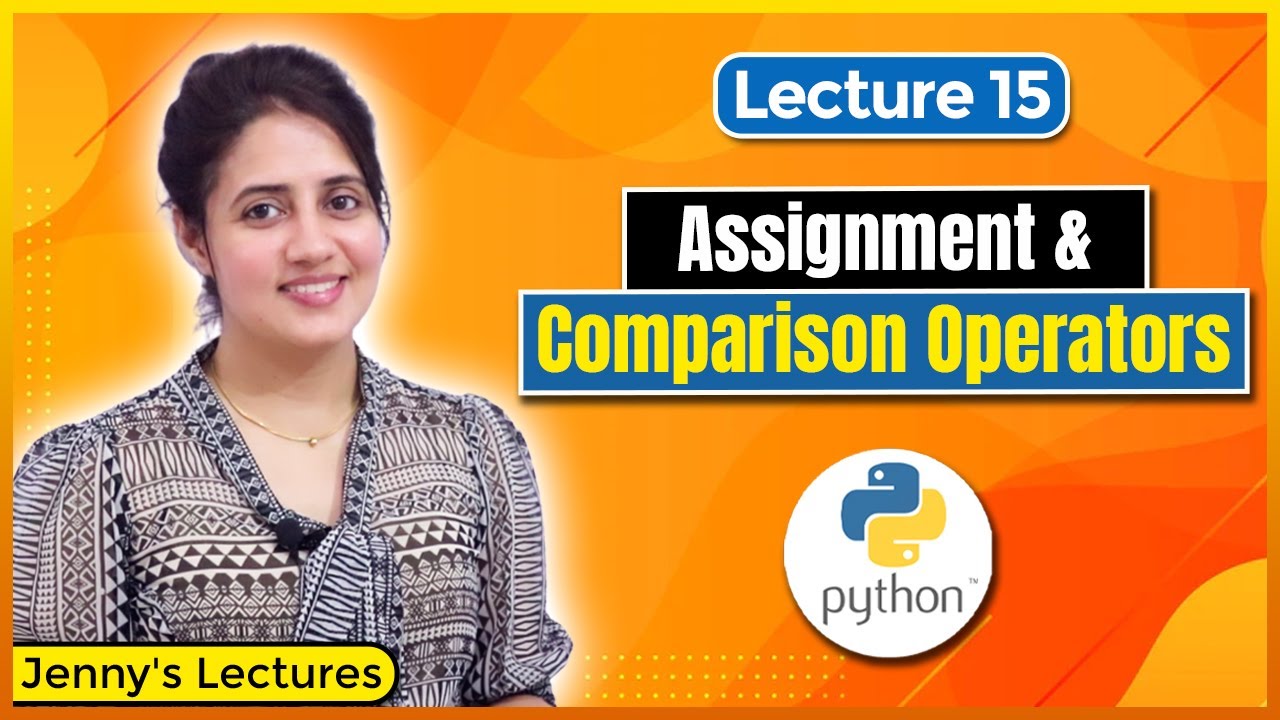
P_15 Operators in Python | Assignment and Comparison Operators | Python Tutorials for Beginners
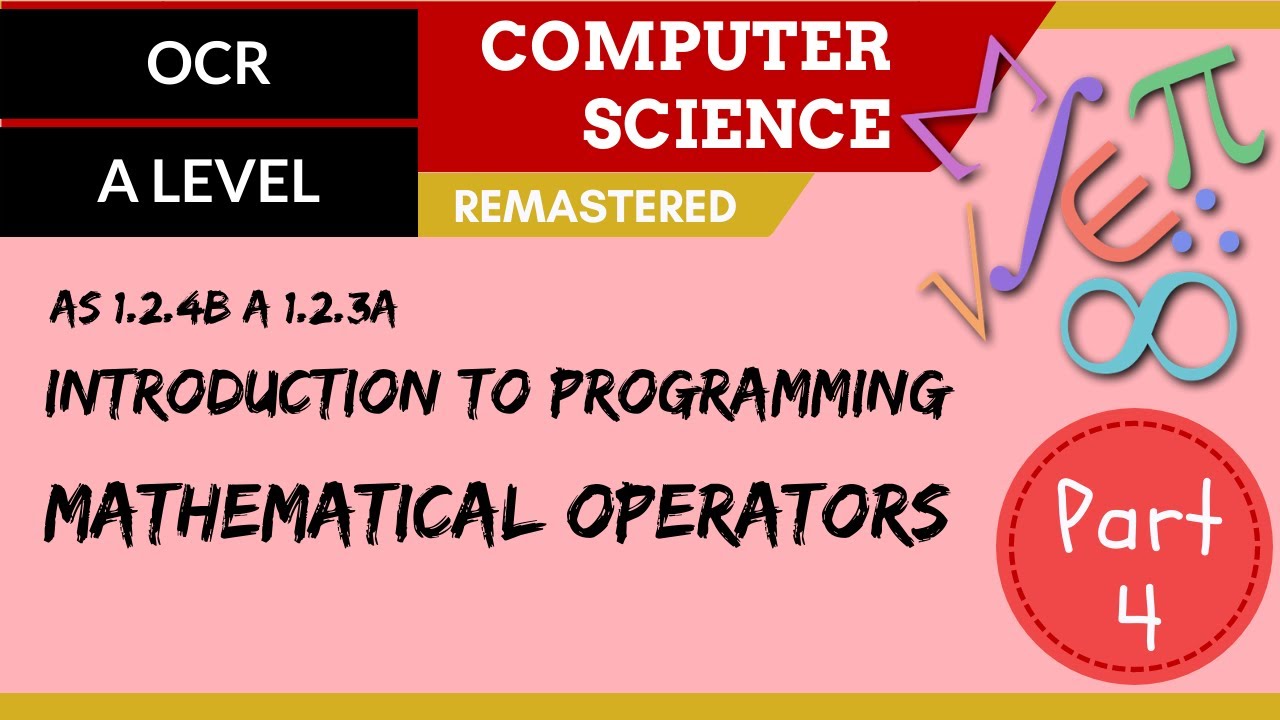
43. OCR A Level (H046-H446) SLR8 - 1.2 Introduction to programming part 4 mathematical operators
5.0 / 5 (0 votes)