Beginning C# with Unity (2023 Edition) - Operators
Summary
TLDRIn this educational video, Vegetarian Zombie guides viewers through the basics of C# operators in Unity, emphasizing the importance of celebrating small coding successes. The tutorial begins with an assignment operator, then explores incrementing and decrementing variables, and introduces equality checks. Sponsored by cadeco.com, the video also includes a practical demonstration of creating a button in Unity and responding to clicks using a script. The lesson covers arithmetic operations, the modulus operator, string concatenation, and the order of operations. It concludes with a challenge to create a tip calculator, encouraging viewers to apply their newfound knowledge.
Takeaways
- 🎓 The video is an educational tutorial aimed at beginners in C# and Unity, focusing on the use of operators.
- 👏 It encourages learners to celebrate small successes and be patient with themselves as they progress in coding.
- 🔢 The video introduces the assignment operator, explaining how to use it to increment variables like hit points and experience points in a game.
- 💻 Sponsored by cadeco.com, a platform for developers offering learning resources on various development topics, including game development in Unity and Unreal.
- 🛠️ The tutorial demonstrates adding interactivity to a Unity project by creating a button and a script to respond to button clicks.
- 📝 It discusses the importance of understanding operators for game development, such as increment, decrement, addition, subtraction, multiplication, division, and modulus.
- 🧠 The video presents a brain teaser to illustrate the difference between postfix and prefix increment operators, highlighting a common source of confusion for new programmers.
- 🔄 The concept of integer division is explained, showing how it truncates decimal values, and the modulus operator is introduced as a tool for remainder calculations.
- 📑 The tutorial covers string concatenation and the need for manual spacing when combining strings with the addition operator.
- 🧮 Emphasis is placed on the order of operations in arithmetic, advising viewers to write clear and simple code to avoid confusion.
- 🔄 The cast operator is introduced for type conversion, with a cautionary note about data loss when converting from a larger to a smaller data type.
- 💡 The video concludes with a challenge to create a tip calculator, providing a practical application of the concepts discussed.
Q & A
What is the main focus of the video?
-The main focus of the video is to introduce the viewer to the world of operators in C# programming within the context of Unity game development.
Why is it important to celebrate small successes in coding?
-Celebrating small successes in coding is important because coding is hard and it takes time to understand concepts. Recognizing and rewarding progress helps maintain motivation and patience.
What is the assignment operator in programming?
-The assignment operator in programming, denoted by the equal sign, is used to assign the value of one variable to another, rather than to check for equality as in mathematics.
What is the purpose of the 'my operators' script created in the video?
-The 'my operators' script is created to demonstrate how to respond to button presses in Unity and to show how to increment variables using different operators.
How does the video explain the difference between prefix and postfix operators?
-The video explains that the prefix operator increments the value before the operation, while the postfix operator increments the value after the operation. It also cautions that the postfix operator can be confusing and recommends using the 'plus equals' operator for clarity.
Why does the video recommend avoiding the use of postfix operators when starting out?
-The video recommends avoiding postfix operators for beginners because they can be easily misunderstood, leading to bugs and confusion, especially when the order of operations is not clear.
What is the modulus operator and how is it demonstrated in the video?
-The modulus operator returns the remainder of a division operation. In the video, it is demonstrated by dividing 5 by 2, which results in a remainder of 1.
How does the video handle the addition of strings in C#?
-The video shows that when adding strings in C#, it is necessary to manually add spaces between the strings to avoid them being concatenated without any separation.
What is the importance of the order of operations in coding as discussed in the video?
-The video emphasizes the importance of the order of operations to ensure that calculations are performed correctly. It reminds viewers to follow the standard order (parentheses, exponents, multiplication/division, addition/subtraction) to avoid errors.
What is the cast operator and how is it used in the video?
-The cast operator is used to convert a variable from one data type to another. In the video, it is used to convert an integer to a float and vice versa, demonstrating how data loss can occur when converting from a larger to a smaller data type.
What challenge is presented at the end of the video?
-The challenge presented at the end of the video is to create a tip calculator that calculates the total amount including a tip based on the bill total and a tip percentage.
Outlines
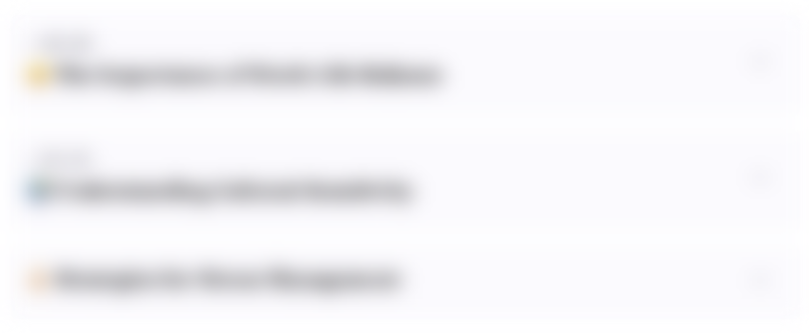
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードMindmap
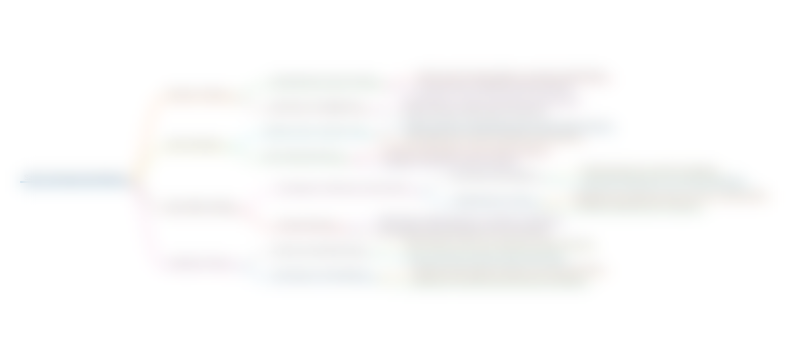
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードKeywords
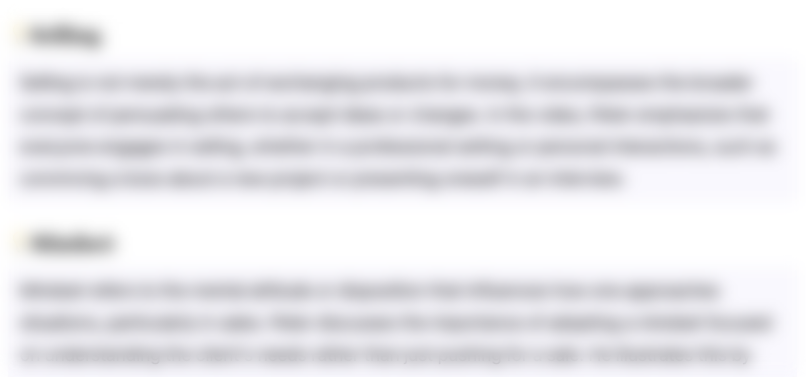
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードHighlights
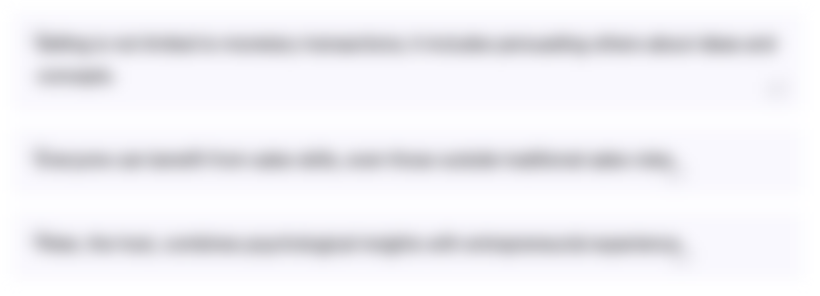
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードTranscripts
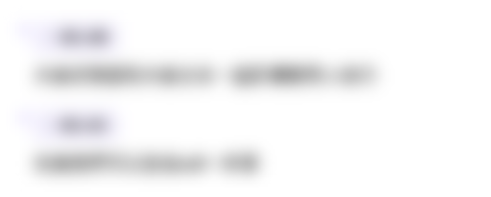
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレード関連動画をさらに表示
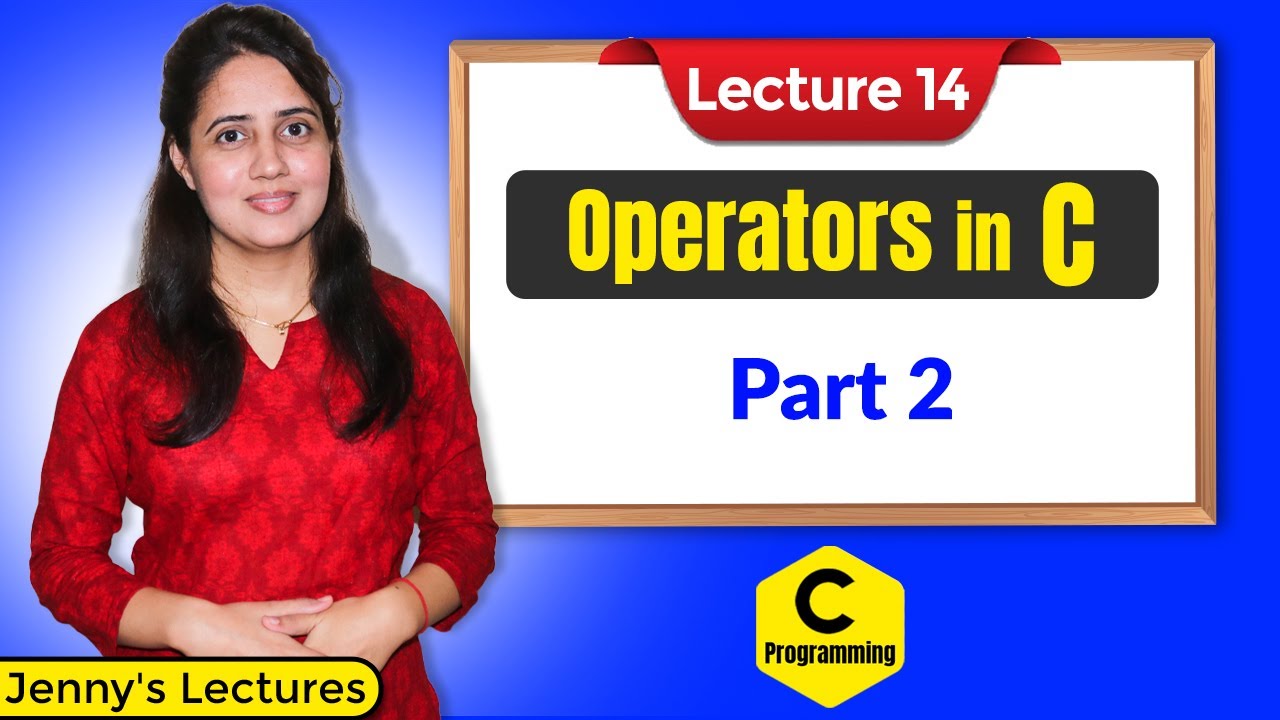
C_14 Operators in C - Part 2 | Arithmetic & Assignment Operators | C Programming Tutorials
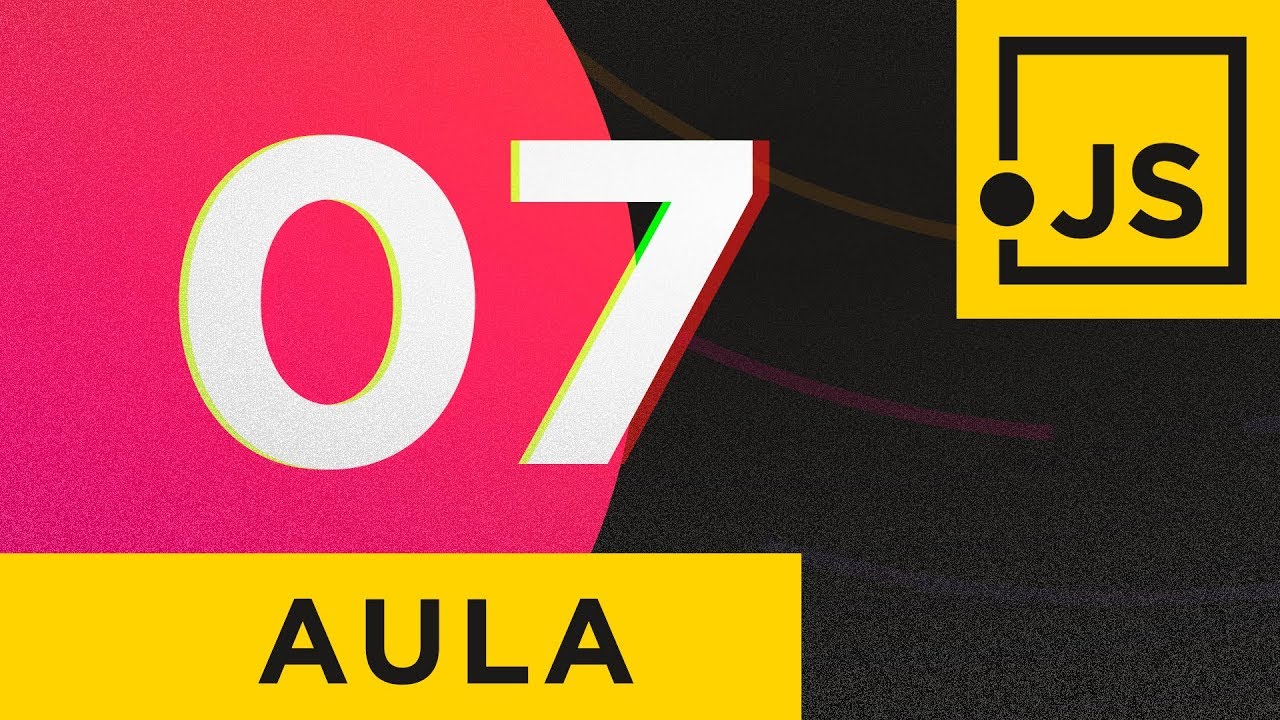
Operators (Part1) - JavaScript Course #07
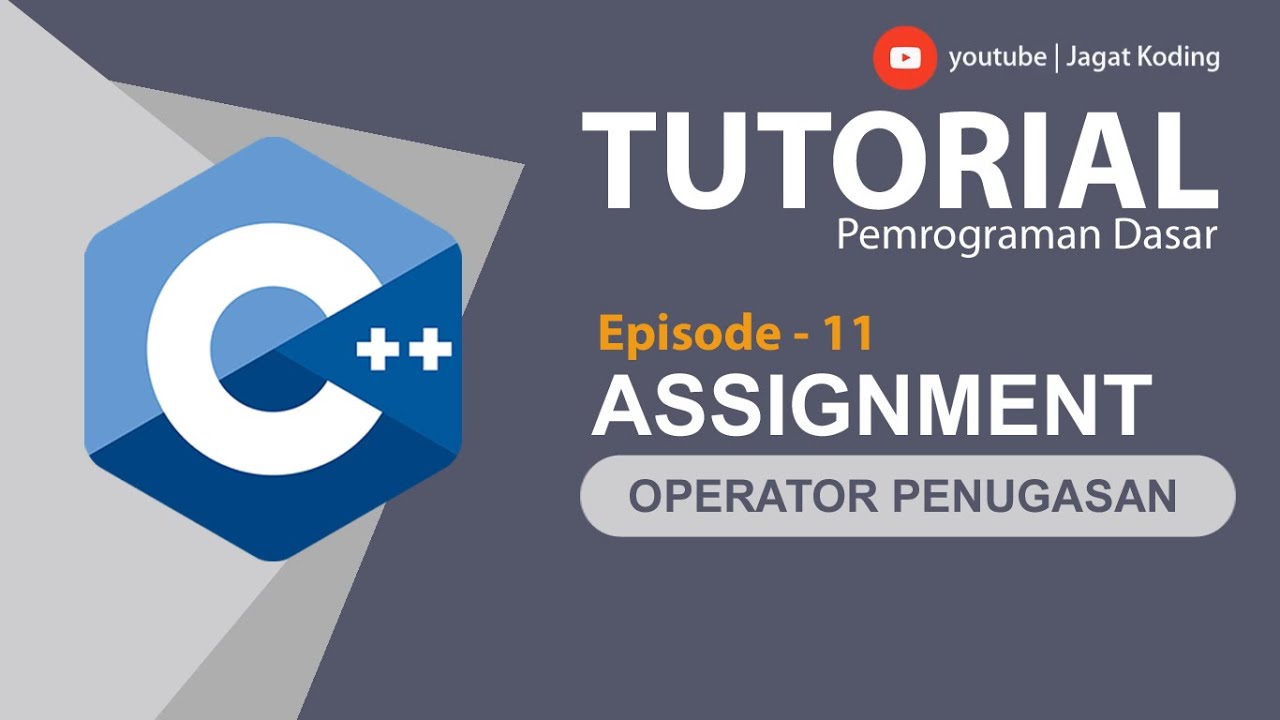
C++ 11 | Operator Assignment | Tutorial Pemrograman C++
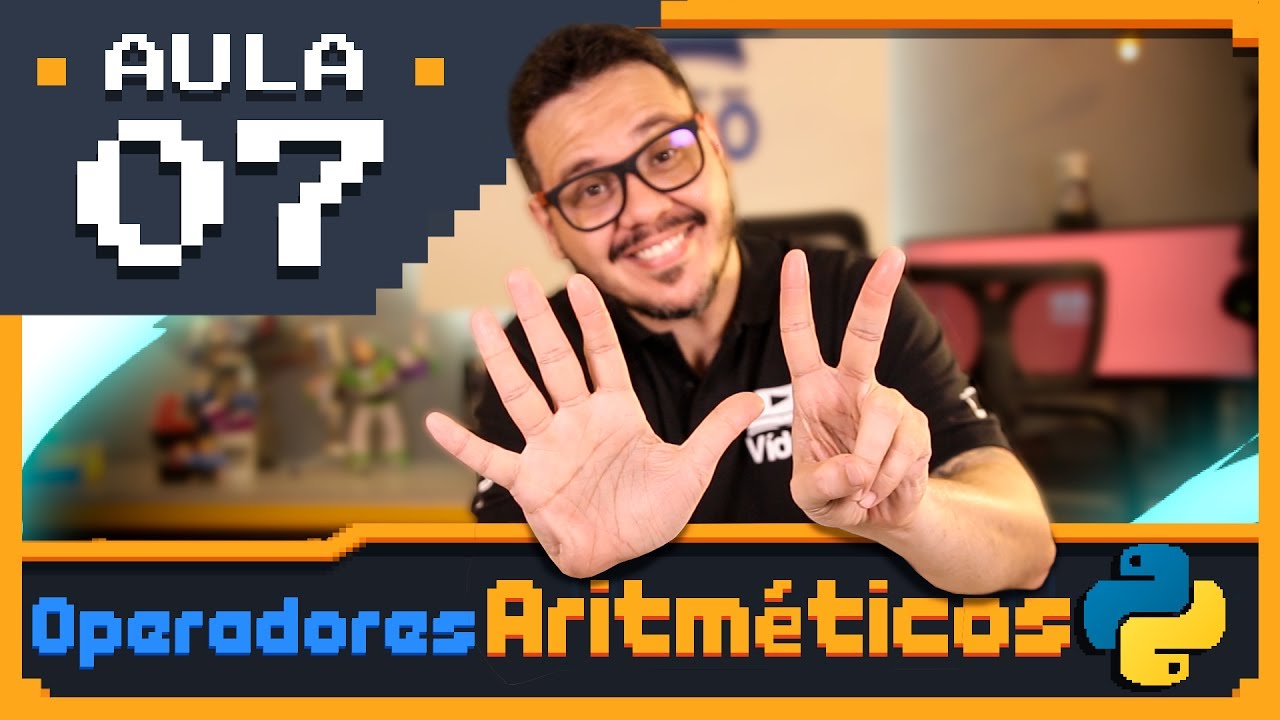
Curso Python #07 - Operadores Aritméticos
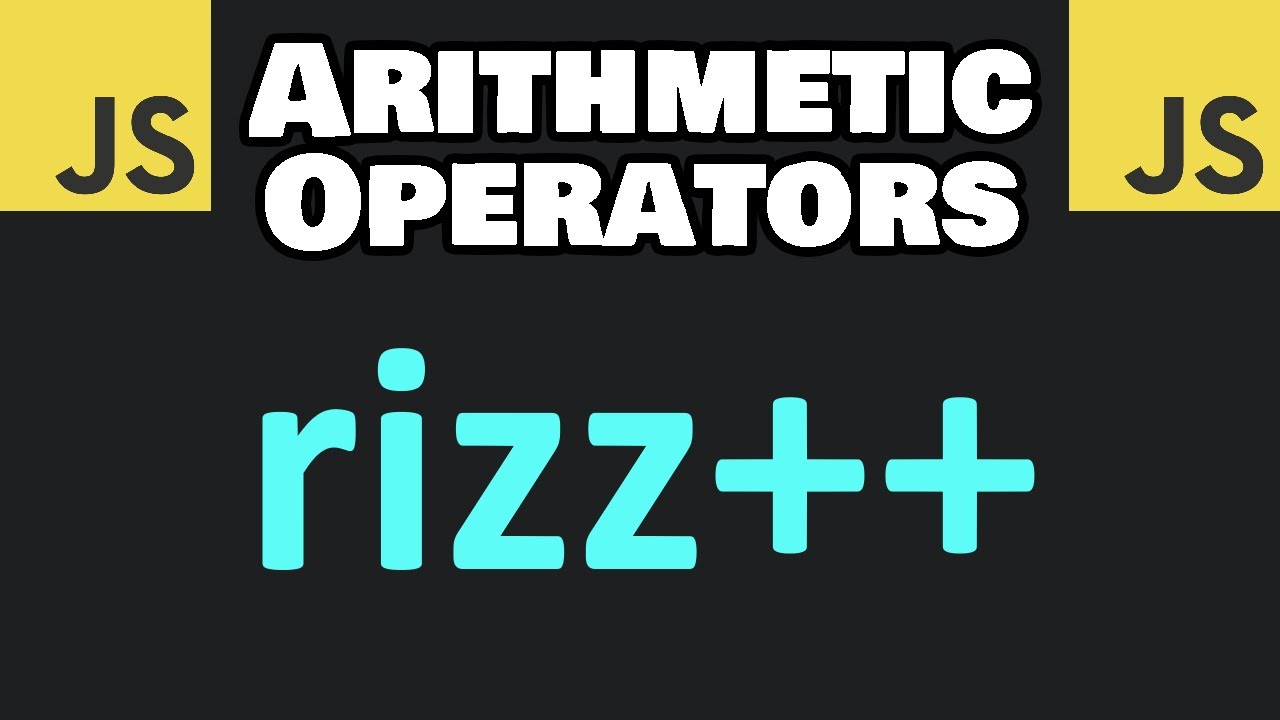
JavaScript ARITHMETIC OPERATORS in 8 minutes! ➕
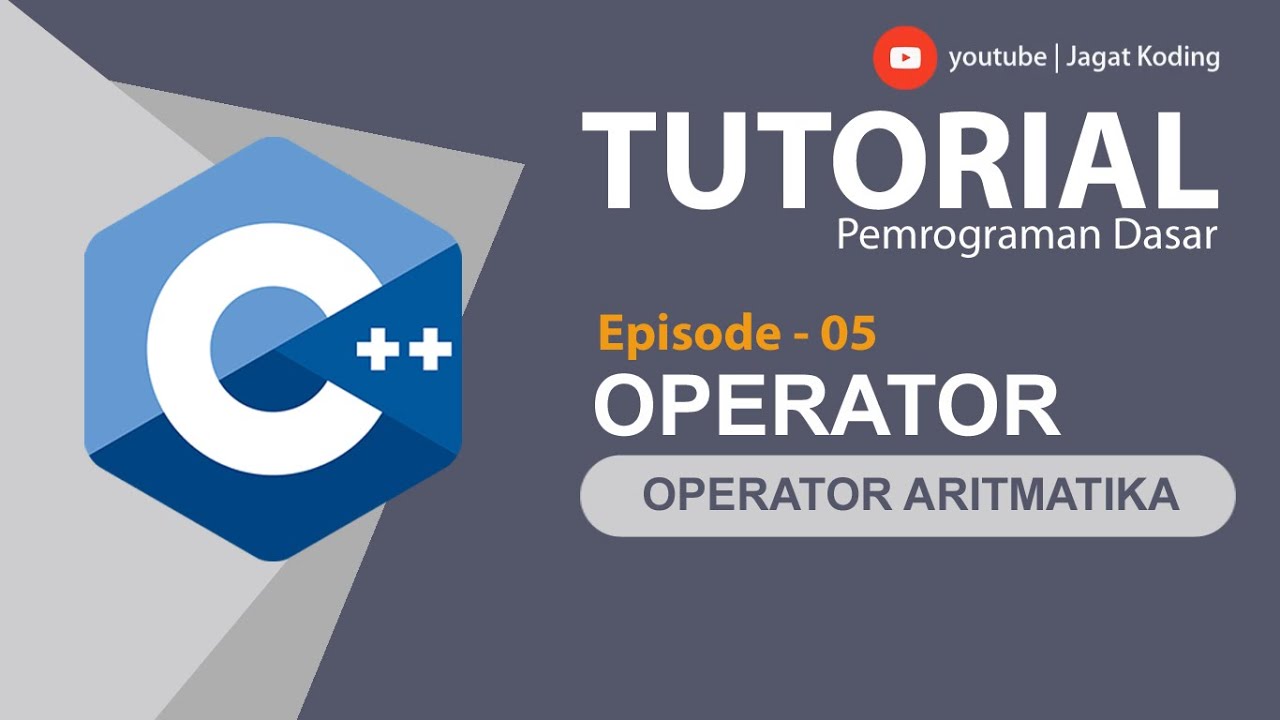
C++ 05 | Operator Aritmatika | Tutorial Dev C++ Indonesia
5.0 / 5 (0 votes)