#20 Python Tutorial for Beginners | While Loop in Python
Summary
TLDRThis Python tutorial introduces loops, focusing on the 'while' loop to repeat code efficiently. The instructor explains the importance of loops by comparing manual repetition with the use of loops for tasks like printing statements multiple times. The video walks through the syntax and key concepts of the 'while' loop, including initialization, conditions, and incrementing or decrementing values. It also covers debugging loops and nesting loops for more complex tasks. Through hands-on examples, the video demonstrates how to structure loops in Python to save time and reduce redundancy, ensuring clearer understanding and better programming practices.
Takeaways
- 😀 Loops in programming allow you to repeat code efficiently, avoiding manual repetition and saving time.
- 😀 The `while` loop in Python continues to execute a block of code as long as a specified condition remains true.
- 😀 Instead of copying and pasting code multiple times, you can use a `while` loop to repeat statements automatically.
- 😀 The basic structure of a `while` loop in Python consists of a condition and an indented block of code to execute.
- 😀 A counter variable (e.g., `i`) is used to control the number of iterations in a `while` loop.
- 😀 To increment the counter in a `while` loop, you can use `i += 1`. This updates the counter after each loop iteration.
- 😀 A `while` loop stops when the condition becomes false, such as when the counter exceeds a certain value.
- 😀 You can reverse the iteration by using decrementing instead of incrementing, such as `i -= 1`.
- 😀 Nested `while` loops allow you to execute a loop inside another loop, enabling more complex repetitive tasks.
- 😀 The importance of initializing the counter variable, setting the loop condition, and deciding whether to increment or decrement is crucial for controlling loop behavior.
- 😀 Debugging is essential to understand how the values change during each iteration of the loop, helping to identify logic errors.
Q & A
What is the primary purpose of using loops in programming?
-The primary purpose of loops in programming is to repeat certain statements multiple times without manually writing them out each time. This helps to automate repetitive tasks and make the code more efficient.
What are the two types of loops discussed in the script?
-The two types of loops discussed in the script are the 'while loop' and the 'for loop'. The script focuses on explaining the while loop.
Why is using a loop better than manually copying and pasting code multiple times?
-Using a loop is more efficient than copying and pasting code because it allows the same block of code to be repeated a specified number of times without redundancy, making the code cleaner and easier to maintain.
How does a 'while loop' work in Python?
-A 'while loop' in Python works by repeatedly executing a block of code as long as a specified condition evaluates to true. Once the condition becomes false, the loop stops.
What are the three essential parts of a while loop as described in the video?
-The three essential parts of a while loop are initialization (starting a variable), the condition (defining when the loop should stop), and the increment/decrement (changing the variable to eventually meet the condition).
What happens if the loop condition in a while loop is never met?
-If the condition in a while loop is never met, the loop will run infinitely, leading to a 'forever loop', which can cause the program to crash or hang.
What does the 'I = I + 1' statement do in the while loop?
-The 'I = I + 1' statement increments the value of the variable 'I' by 1 after each iteration, which is necessary to eventually break the loop once the condition becomes false.
What does the 'end' parameter in the print function do in Python?
-The 'end' parameter in the print function specifies what should be printed at the end of the output. By default, it is set to a newline character, but setting it to an empty string or a different character prevents the output from going to the next line.
What issue did the presenter encounter when using nested while loops?
-The presenter encountered an issue where the inner loop did not stop after reaching its condition. This was due to forgetting to increment the inner loop variable, which caused the loop to run indefinitely.
How can you fix an issue where two print statements are not appearing on separate lines?
-To fix the issue where print statements are not appearing on separate lines, you can explicitly use a print statement with no arguments (i.e., print()) to move to the next line after the first print statement.
Outlines
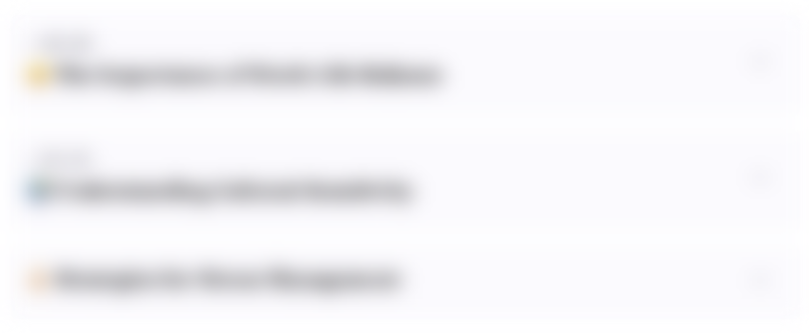
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenMindmap
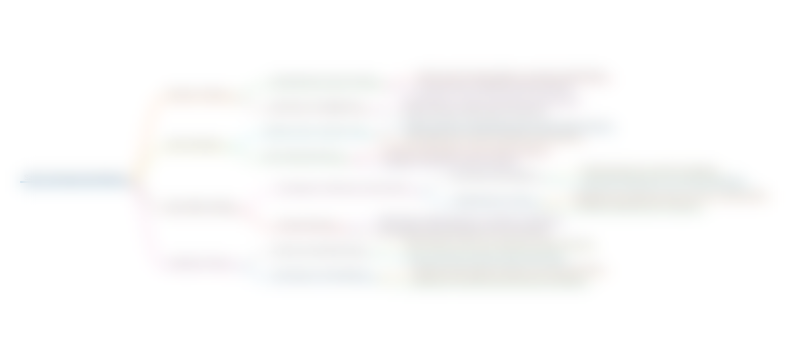
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenKeywords
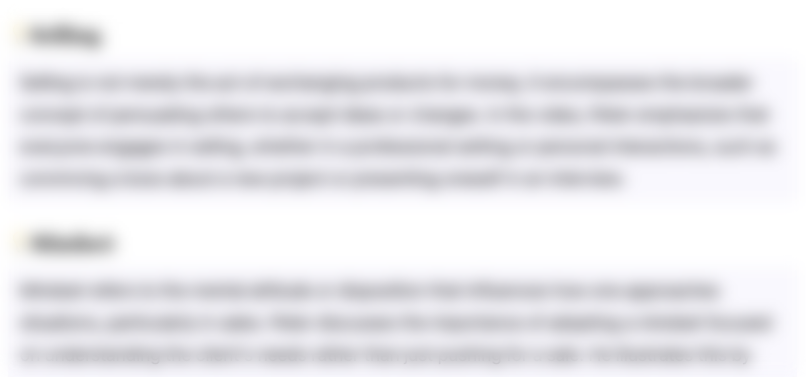
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenHighlights
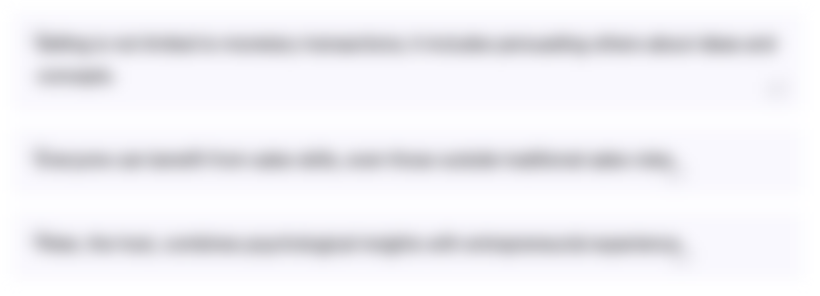
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenTranscripts
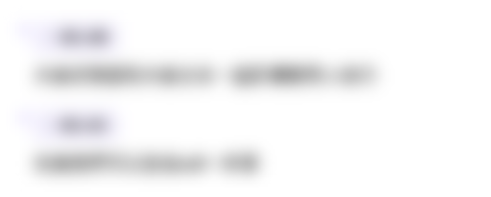
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenWeitere ähnliche Videos ansehen
5.0 / 5 (0 votes)