Python Programming - Basic Input and Output - print and input functions
Summary
TLDRThis video tutorial provides a comprehensive introduction to basic input and output functions in Python, focusing on the `print` and `input` functions. Viewers learn how to display text and variables using `print()`, manage output formatting with string literals and newline characters, and capture user input with `input()`. The tutorial emphasizes the importance of converting input strings to integers for calculations and demonstrates practical examples to solidify understanding. Overall, it serves as a foundational guide for beginners looking to enhance their programming skills in Python.
Takeaways
- 😀 The `print()` function is used to display output on the screen.
- 😀 Strings can include various characters and symbols, and they must be enclosed in single or double quotes.
- 😀 The newline character (`\n`) can be used to print text on a new line without multiple `print()` statements.
- 😀 The `input()` function reads user input as strings, which can be assigned to variables.
- 😀 To format multiple items in `print()`, use commas to separate them; this automatically adds spaces between them.
- 😀 If you want to suppress the newline after a `print()` statement, use `end=''` to control the output format.
- 😀 Whitespace in the code does not affect the output, but it's best to maintain readability with consistent spacing.
- 😀 The `int()` function converts string input to integers for mathematical operations.
- 😀 Ensure proper handling of quotation marks when including apostrophes in strings to avoid syntax errors.
- 😀 Example code demonstrates capturing user input for name and age and performing arithmetic operations.
Q & A
What is the purpose of the print function in Python?
-The print function is used to display output to the screen, allowing you to print strings, numbers, and other data types.
How do you print a string literal in Python?
-You can print a string literal by enclosing it in either single quotes (' ') or double quotes (' '), such as print('Hello, World!').
What is a newline character and how is it used?
-A newline character, represented as '\n', is used to move the output to a new line when printed. For example, print('Hello,\nWorld!') prints 'Hello,' and 'World!' on separate lines.
How can you control the end character in a print statement?
-You can control the end character in a print statement using the 'end' parameter. For instance, print('Hello', end=' ') will print 'Hello' without moving to a new line and will continue on the same line.
What does the input function do in Python?
-The input function reads a line of input from the user as a string. You can use it to prompt the user for data, such as their name or age.
How do you handle quotes in input prompts?
-If your input prompt contains an apostrophe, you can use double quotes to enclose the entire prompt. For example, input("What's your name?") avoids conflicts with the apostrophe.
Why is it necessary to convert input data types in Python?
-Input data is read as a string, so to perform calculations (like adding numbers), you need to convert the input to the appropriate data type, such as using int() to convert a string to an integer.
What will happen if you try to add one to a variable holding input without converting it to an integer?
-If you attempt to add one to a variable holding input without converting it to an integer, you will receive a TypeError because you cannot perform arithmetic operations on strings.
Can you give an example of using both print and input functions together?
-Yes, for instance: name = input('What's your name? '); print(name, 'is', age, 'years old.') combines both functions to greet the user with their name and age.
How does whitespace affect the print function's output?
-Whitespace in the print function does not affect the output; items separated by commas automatically include a space. However, excessive whitespace may make the code harder to read.
Outlines
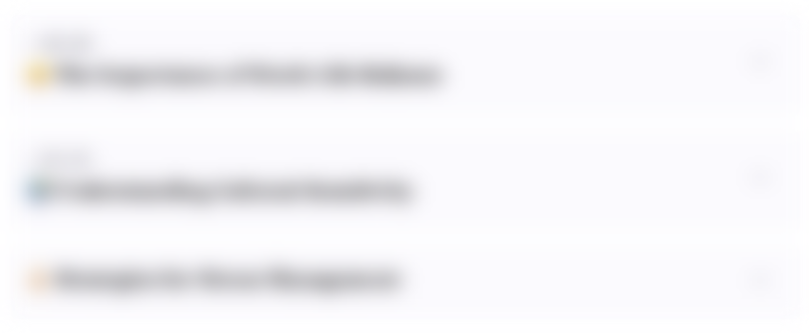
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenMindmap
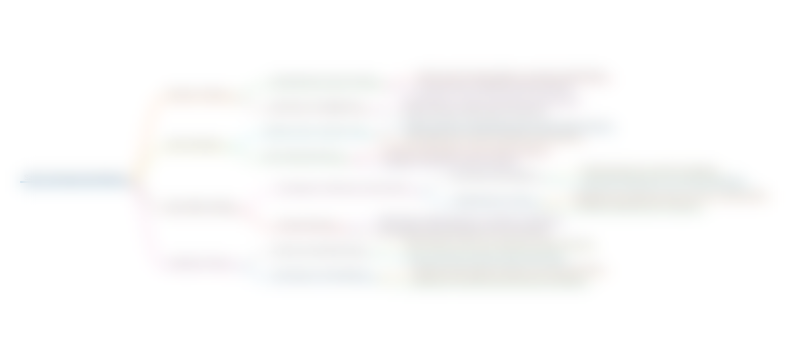
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenKeywords
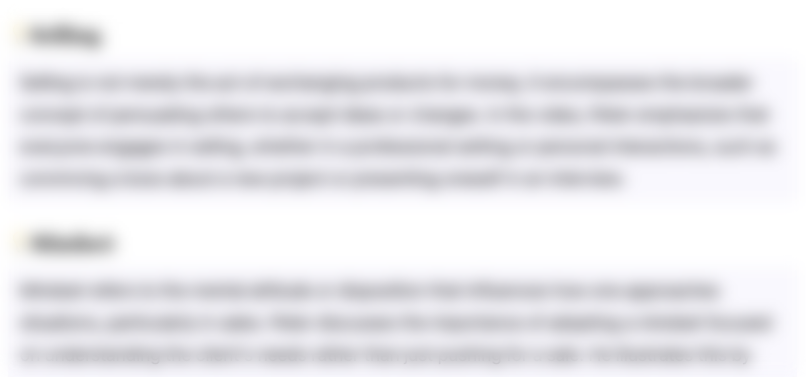
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenHighlights
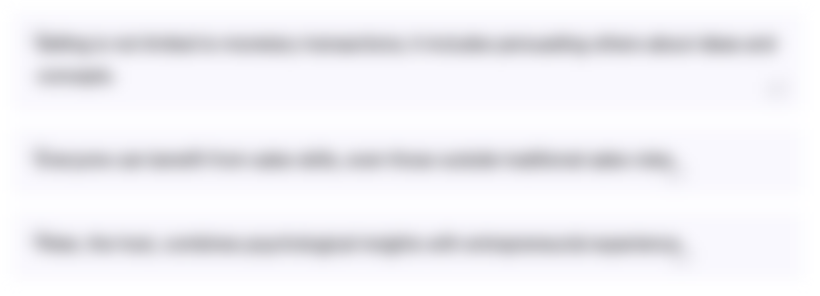
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenTranscripts
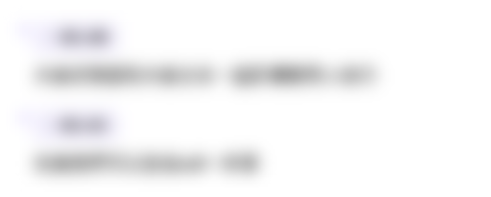
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenWeitere ähnliche Videos ansehen
5.0 / 5 (0 votes)