#32 Array of Objects in Java
Summary
TLDRIn this video, the focus is on enhancing understanding of arrays in programming. It begins with a discussion on the necessity of arrays and their creation, then delves into more complex operations such as dynamically printing array values without hardcoding the size. The instructor demonstrates the default initialization of integer arrays and the potential for runtime errors, like index out of bounds exceptions. A solution is presented using the 'length' property to avoid such errors. The tutorial progresses to creating an array of objects, specifically 'Student' objects, and iterating over them to print details. The video concludes with a preview of upcoming topics, promising to address the challenge of printing object values directly.
Takeaways
- 😀 Arrays are essential for storing multiple values in a single variable.
- 🔍 The length of an array can be dynamic, and it's crucial to know the array's length to avoid errors.
- 👀 Default values for integer arrays are zero, which is important to remember when elements are not explicitly initialized.
- ⚠️ Accessing an array index that is out of bounds will result in a runtime exception.
- 🛠️ The 'length' property of an array can be used to safely iterate over its elements without causing errors.
- 🎓 Demonstrated the creation of a 'Student' class with instance variables like roll number, name, and marks.
- 📚 Showed how to create multiple 'Student' objects and assign them to an array to manage a collection of students.
- 🔑 Explained that arrays do not create objects themselves; objects must be instantiated separately and then added to the array.
- 🖨️ Discussed how to print the details of objects stored in an array, emphasizing the need to access object properties individually.
- 🔄 Illustrated the process of iterating over an array of 'Student' objects and printing their details using a loop.
Q & A
What happens if you define an array with a size larger than the number of assigned values?
-If you define an array with a size larger than the assigned values, the unassigned indexes will be filled with default values. For an integer array, the default value is zero.
What is an exception in the context of arrays?
-An exception is a runtime error that occurs when you try to access an array index that is out of bounds. For example, if the array has six elements and you try to access the seventh, an exception will be thrown.
How can the length property of an array help avoid errors?
-The length property can help by ensuring you only access valid indexes within the array. By using the array's length property, you can iterate over the array without exceeding its bounds and causing exceptions.
How do you create an array of objects, such as students, in this example?
-To create an array of objects, you define an array of the object type (e.g., `Student[] students = new Student[3];`). Then, you manually assign objects to the array's indexes, such as `students[0] = S1;`.
What is the difference between creating an array of objects and creating the objects themselves?
-Creating an array of objects only allocates space for the object references, not the objects themselves. You need to manually create the objects and assign them to the array.
Why does printing an object directly result in an address-like value?
-When printing an object directly without overriding the `toString()` method, Java prints the memory reference (address) of the object rather than its values. This is why you need to explicitly access object fields like `S1.name` to print meaningful information.
How can you dynamically print the values of multiple student objects in an array?
-You can loop through the array using a `for` loop, accessing each object's fields dynamically. For example, `students[i].name` and `students[i].marks` will print the name and marks of each student as the loop iterates.
Why is it necessary to use object fields like `.name` and `.marks` when printing student data?
-Without explicitly accessing these fields, Java will print the object's memory reference instead of its actual data. Using `object.field` ensures that you retrieve and print the desired values stored in the object.
Can arrays hold different data types, such as integers, strings, and objects?
-Yes, arrays can hold different data types, but they must be homogeneous. For example, you can have an array of integers, an array of strings, or an array of objects like `Student`. However, you cannot mix data types in a single array.
What is the purpose of using arrays in Java programming?
-Arrays provide a way to store multiple values of the same type in a single variable, making it easier to manage and process large amounts of data. They allow for more efficient storage and retrieval compared to individual variables.
Outlines
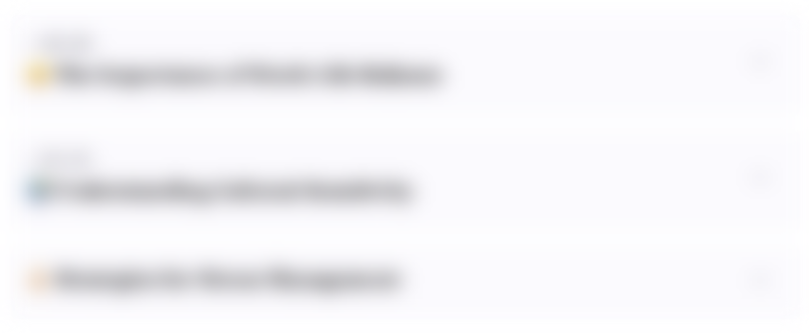
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenMindmap
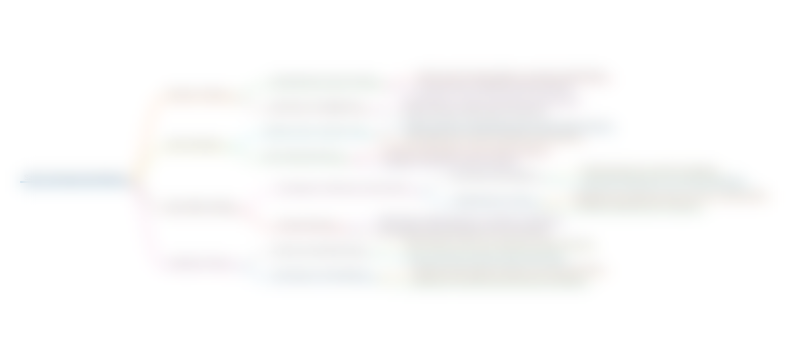
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenKeywords
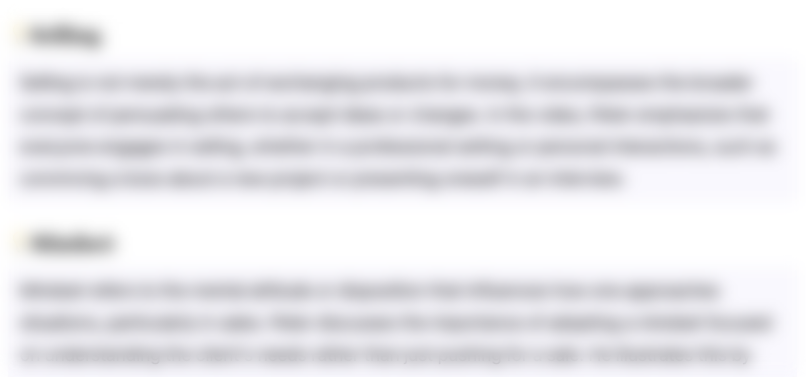
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenHighlights
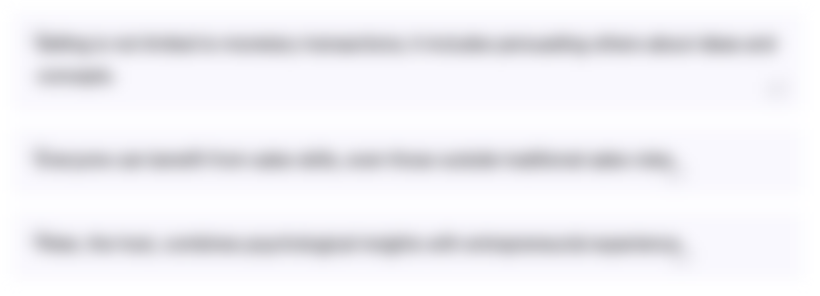
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenTranscripts
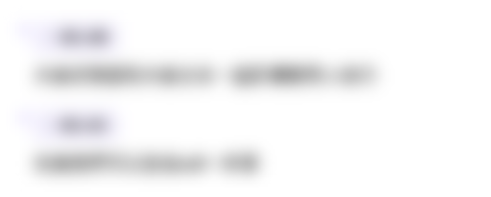
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführen5.0 / 5 (0 votes)