JavaScript Visualized - Promise Execution
Summary
TLDRThis script demystifies JavaScript Promises by explaining their creation, execution, and handling behind the scenes. It clarifies how Promises work with async tasks, the event loop, and microtask queue, using a practical example with setTimeout. The explanation simplifies Promise chaining and the non-blocking nature of asynchronous JavaScript, encouraging viewers to understand and utilize Promises effectively in their coding.
Takeaways
- 😎 Promises in JavaScript can seem intimidating but are not as complicated once you understand their mechanics.
- 🛠 A Promise is created using the `new Promise` constructor, which takes an Executor function and creates a Promise object with internal slots for state, result, and reactions.
- 🔄 The Executor function has access to `resolve` and `reject` to change the state of the Promise to 'fulfilled' or 'rejected', respectively.
- 🔗 Promises can be chained using `.then()` and `.catch()` methods, which add Promise reaction records to the Promise object.
- 📂 The microtask queue is where the asynchronous part of Promises is handled, and it gets priority over the task queue when the call stack is empty.
- 🔄 When a Promise is resolved, its reaction records' handlers are added to the microtask queue, allowing for non-blocking execution.
- 🕒 The `setTimeout` function is used in the script to demonstrate asynchronous behavior within a Promise constructor.
- 🔄 The `.then()` method not only creates a reaction record but also returns a new Promise, enabling Promise chaining.
- 🔢 The script demonstrates how Promises can be chained to incrementally process results, such as multiplying numbers in sequence.
- 📈 Real-world applications of Promises might involve image processing, where operations can be chained in a non-blocking manner.
- 📝 The script concludes with a challenge for viewers to understand the order in which numbers are logged, reinforcing the concept of Promise chaining and execution.
Q & A
What is a Promise in JavaScript?
-A Promise in JavaScript is an object that may produce a single value some time in the future. It represents a proxy for a value not necessarily known when the promise is created. It allows you to associate handlers to be called in the future, when the promise is fulfilled or rejected.
How is a Promise created in JavaScript?
-A Promise is created by using the `new Promise` constructor, which takes an Executor function as an argument. This Executor function itself takes two arguments, typically named `resolve` and `reject`, which are functions used to resolve or reject the promise respectively.
What are the internal states of a Promise?
-A Promise has three possible states: pending, fulfilled, and rejected. The 'pending' state is the initial state. 'Fulfilled' means that the operation completed successfully, and 'rejected' means that the operation failed.
What is the purpose of the resolve function in a Promise?
-The resolve function is used to resolve a Promise, setting its state to 'fulfilled' and assigning it a resulting value. This function is called when the asynchronous operation completes successfully.
What does the reject function do in a Promise?
-The reject function is used to reject a Promise, setting its state to 'rejected' and assigning it a resulting value that represents the reason for the rejection. This function is called when the asynchronous operation fails.
What are Promise reaction records?
-Promise reaction records are objects that are created when you chain a `then` or `catch` method to a Promise. They contain information about the handlers that will be called when the Promise is fulfilled or rejected.
How does the event loop handle Promises?
-The event loop plays a crucial role in handling Promises. When a Promise is resolved or rejected, its handlers are added to the microtask queue. Once the call stack is empty, the event loop checks the microtask queue and executes any pending microtasks.
What is the difference between the microtask queue and the task queue?
-The microtask queue is where Promise reaction handlers are placed and is processed before the task queue. The task queue, also known as the callback queue or macro task queue, is where callbacks from tasks like timers, network responses, and I/O operations are placed.
How can you chain Promises in JavaScript?
-You can chain Promises by using the `then` method. Each call to `then` returns a new Promise, which can then be followed by another `then`, allowing you to create a sequence of asynchronous operations.
What is the significance of the microtask queue in asynchronous JavaScript programming?
-The microtask queue is significant because it allows for the execution of Promise handlers as soon as possible, without blocking the main thread. This helps in keeping the script responsive and efficient.
How does the `setTimeout` function interact with Promises?
-The `setTimeout` function can be used within a Promise's Executor function to schedule an asynchronous task. When the timer completes, the callback passed to `setTimeout` is executed, which can then resolve or reject the Promise based on the result of the asynchronous task.
Outlines
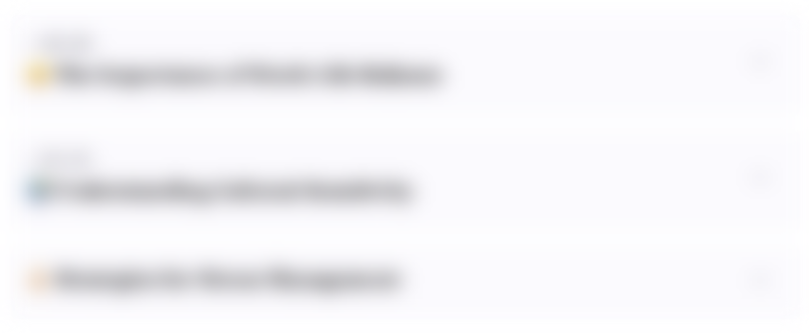
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنMindmap
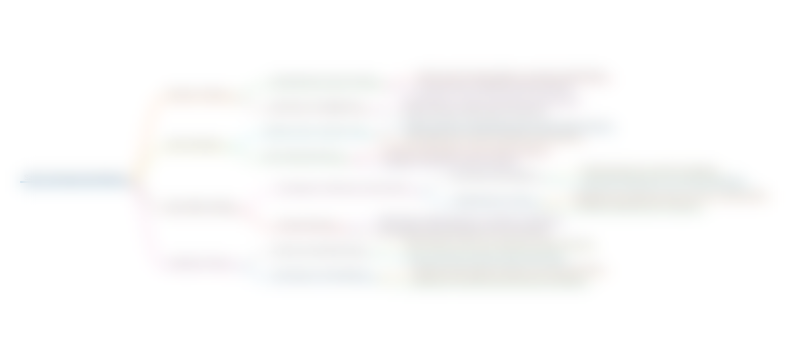
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنKeywords
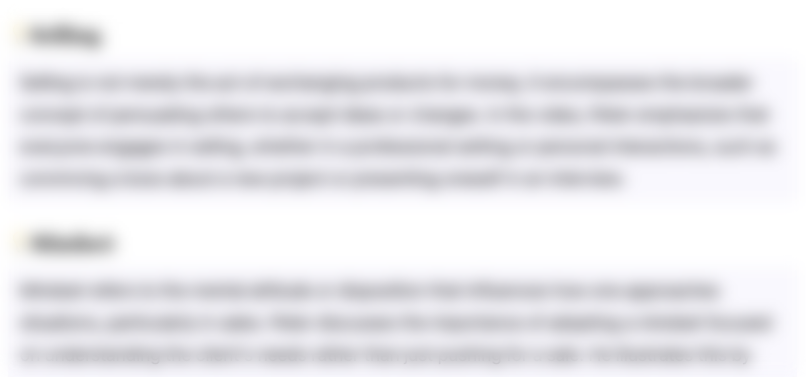
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنHighlights
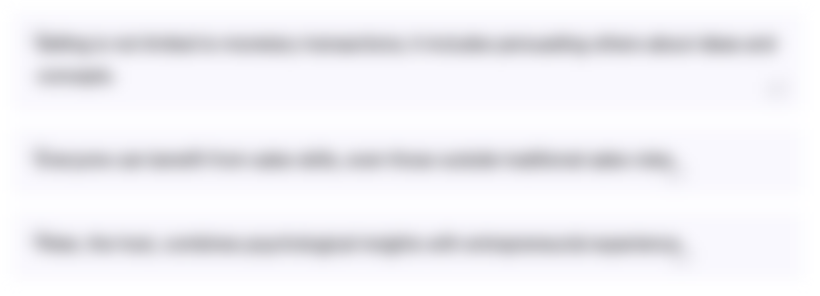
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنTranscripts
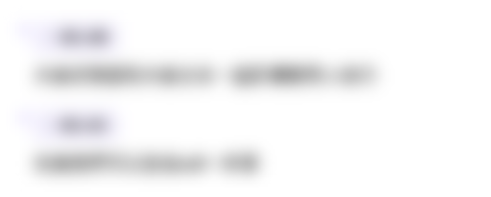
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنتصفح المزيد من مقاطع الفيديو ذات الصلة
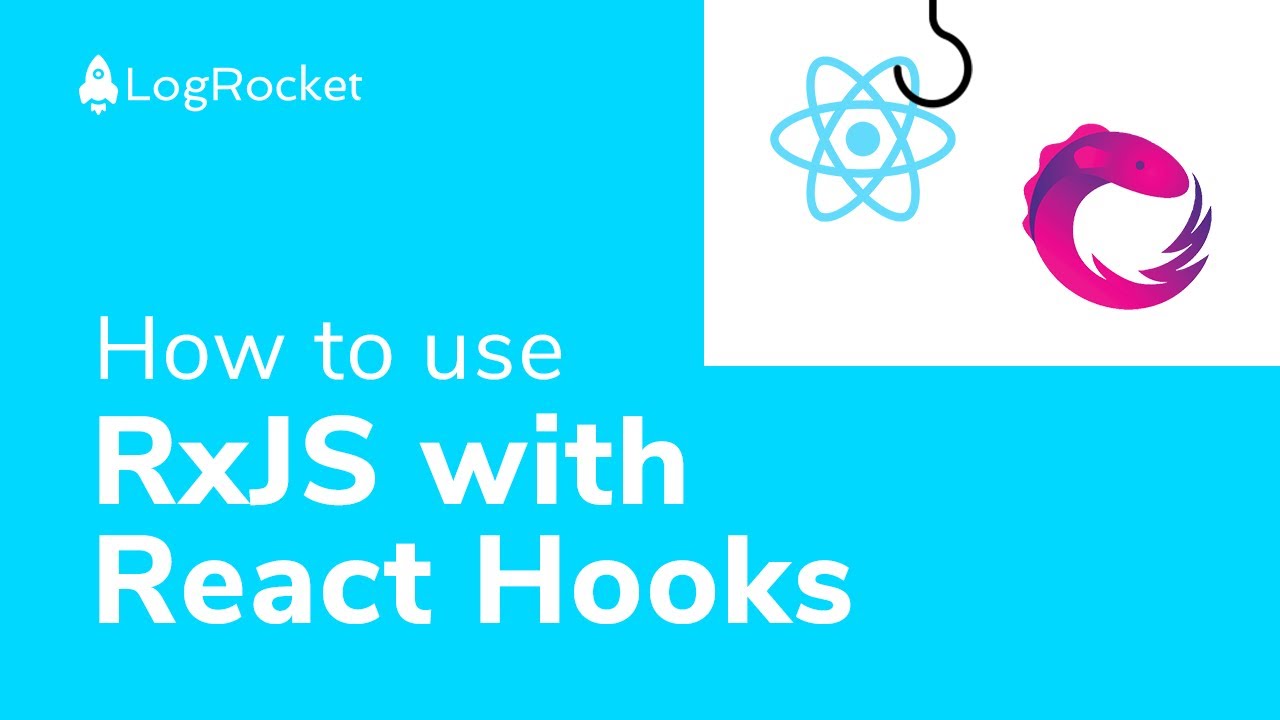
How to use RxJS with React Hooks
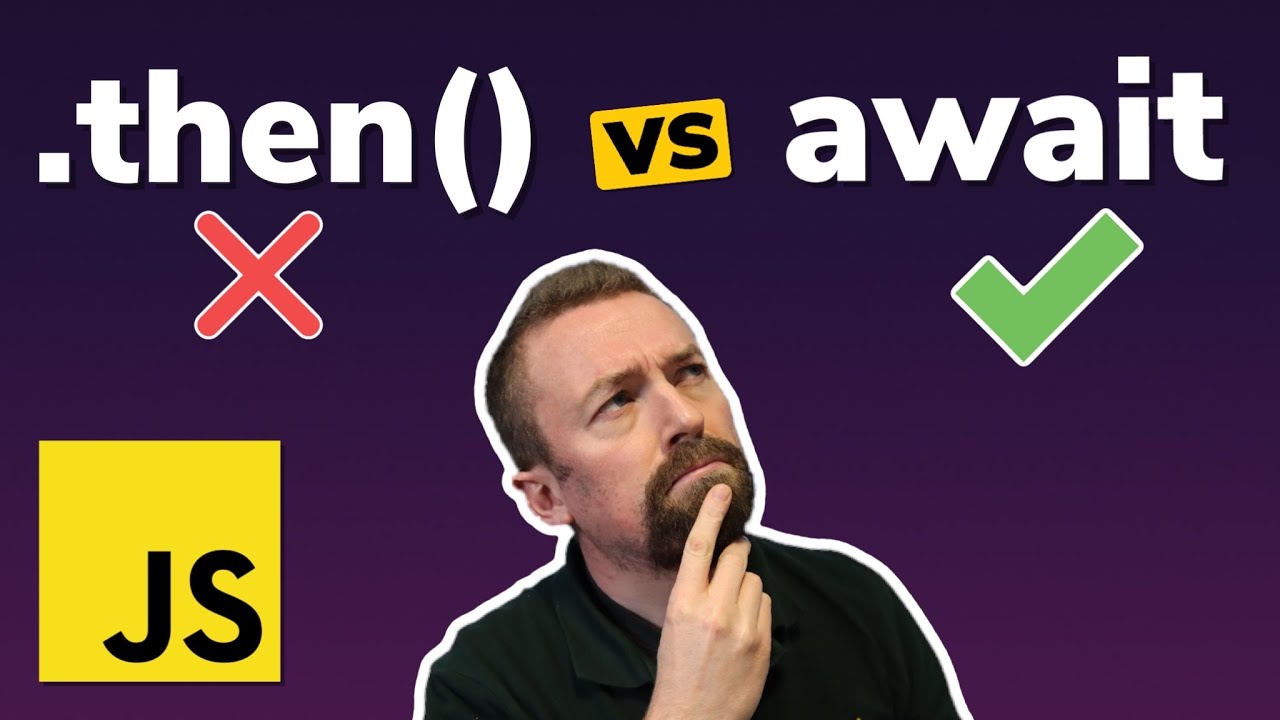
Javascript Promises vs Async Await EXPLAINED (in 5 minutes)
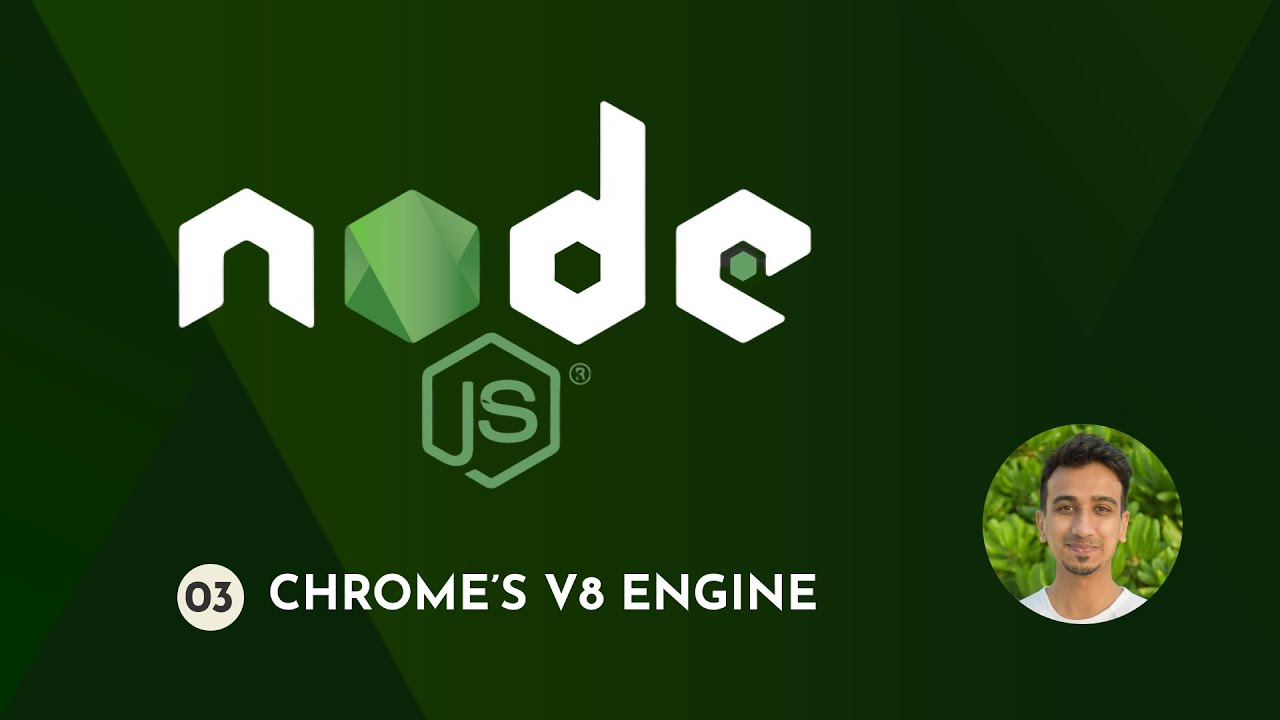
Node.js Tutorial - 3 - Chrome's V8 Engine

Closures | JavaScript 🔥 | Lecture 128

How JavaScript Code is executed? ❤️& Call Stack | Namaste JavaScript Ep. 2

How JavaScript Works 🔥& Execution Context | Namaste JavaScript Ep.1
5.0 / 5 (0 votes)