Overloading dan Overriding Kotlin
Summary
TLDRThis video discusses the key differences between method overloading and method overriding in object-oriented programming. Method overloading occurs when a class has multiple methods with the same name but different parameters, allowing flexibility in input types. In contrast, method overriding involves a subclass using the same method name as its parent class, but altering its behavior. The video explains these concepts with practical code examples, showcasing how method signatures and inheritance affect functionality. It also hints at future discussions on access modifiers, like private and protected, to deepen understanding.
Takeaways
- 📚 Overloading allows methods with the same name in a class to have different parameters (e.g., different data types or number of parameters).
- 🔢 Overloading works by differentiating methods based on the number and type of input parameters, even if they share the same name.
- 🔄 Overriding occurs when a subclass has a method with the same name and parameters as its parent class, but it provides its own implementation.
- 📏 The script explains overloading using a 'Rectangle' class example, where multiple 'calculate' methods take different parameter types (integer vs. double).
- 💡 Overloading is flexible, allowing methods with the same name but different input types or parameter counts, as long as they don't create ambiguity.
- 🚫 Two methods cannot have the same name and the same number/type of parameters in the same class because it would cause a conflict.
- 🧱 The script illustrates overriding using a 'Block' class that inherits from the 'Rectangle' class, where the child class redefines a 'display' method.
- ⚙️ The 'super' keyword is used to call the parent class's constructor in the child class when overriding.
- 🔍 When overriding, if a method exists in both the parent and child classes, the child class's version will be called unless specified otherwise.
- 🔑 The script also mentions that access modifiers like 'open', 'private', and 'protected' affect method visibility, which will be explained later.
Q & A
What is method overloading?
-Method overloading is a feature in object-oriented programming where a class has multiple methods with the same name but different parameters (different number, type, or order of parameters). The method to be executed is determined by the argument list during the call.
What is method overriding?
-Method overriding occurs when a subclass provides a specific implementation for a method that is already defined in its parent class. The method in the child class must have the same name, return type, and parameters as the method in the parent class.
What is the main difference between method overloading and method overriding?
-The main difference is that overloading happens within a single class and deals with multiple methods having the same name but different parameters. Overriding, on the other hand, involves a child class redefining a method that is already defined in its parent class, keeping the same name and parameters.
Can method overloading have different return types?
-Yes, method overloading can have different return types, as long as the parameter lists are different. However, the return type alone cannot be used to distinguish overloaded methods.
In the provided script, how does the `hitung` method demonstrate method overloading?
-The `hitung` method in the `PersegiPanjang` class is overloaded by creating multiple methods with the same name (`hitung`) but different parameter types (e.g., `int` vs. `double`) and different numbers of parameters.
How does the `display` method demonstrate method overriding in the script?
-The `display` method is defined in both the parent class (`PersegiPanjang`) and the child class (`Balok`). The method in the child class overrides the parent class method to provide a more specific implementation that includes additional attributes (e.g., height).
What happens if two overloaded methods have the same number and type of parameters?
-If two overloaded methods have the same number and type of parameters, the compiler will not be able to differentiate between them, causing an error. Overloaded methods must differ either in the number, order, or type of parameters.
Why is the `override` keyword used in method overriding?
-The `override` keyword explicitly indicates that a method is meant to override a method from its parent class. This helps in ensuring that the method signature matches the parent's method, reducing the risk of errors.
What is the purpose of the `super` keyword in the script?
-The `super` keyword is used to call the constructor of the parent class from the child class (`Balok`). This ensures that the parent class attributes (length and width) are initialized before additional attributes are set in the child class.
How does the script illustrate the difference between method overriding and method overloading?
-The script uses the `hitung` method to illustrate overloading by defining multiple `hitung` methods with different parameters within a single class. It demonstrates overriding by defining the `display` method in both the `PersegiPanjang` and `Balok` classes, showing how the child class method takes precedence when called from a child class instance.
Outlines
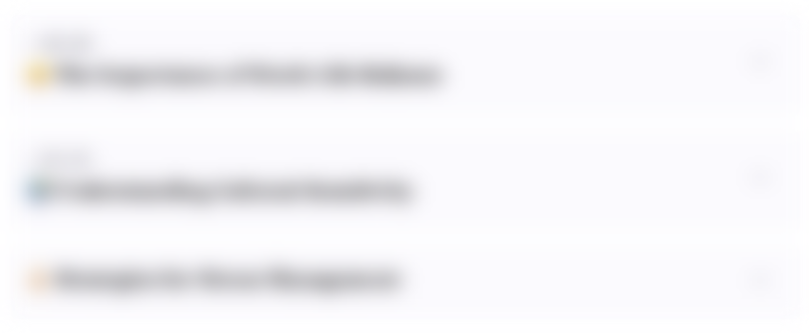
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنMindmap
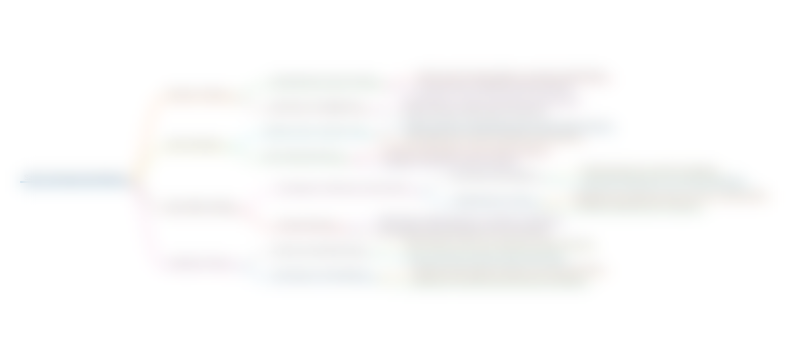
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنKeywords
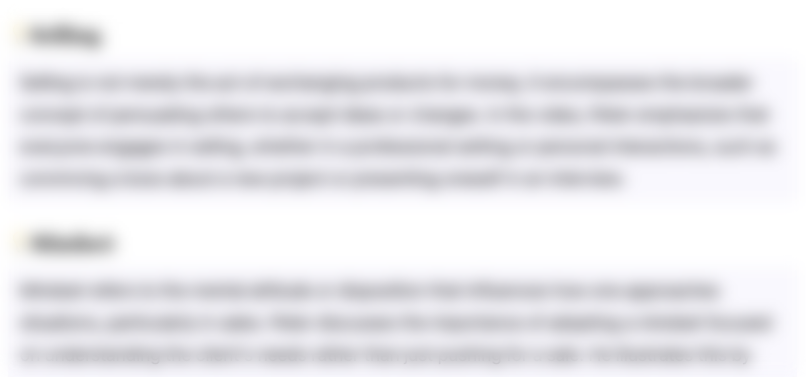
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنHighlights
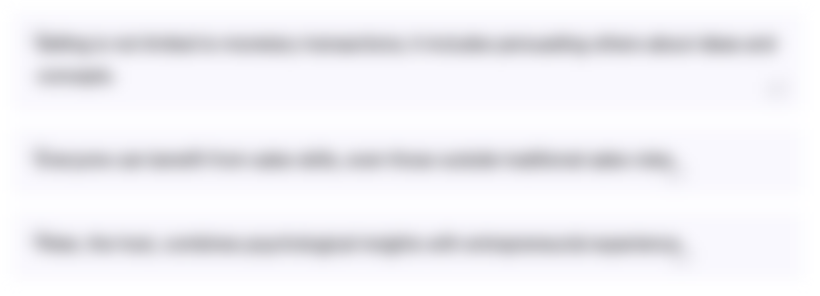
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنTranscripts
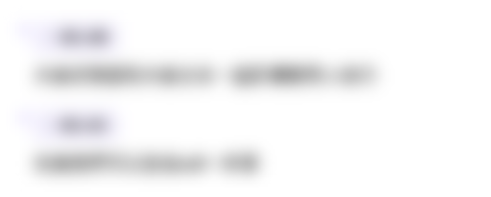
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنتصفح المزيد من مقاطع الفيديو ذات الصلة
5.0 / 5 (0 votes)